How to use Higher-Order Components in React ?
Last Updated :
04 Mar, 2024
Higher-Order Component is an advanced function, which reuses component logic in a React Component and returns an enhanced new component. It wraps commonly used processes to allow their use by different components, such as state modification or props change. Components are wrapped in HOCs, whose goal is to improve them without changing their original constructions. In React applications, they serve code reusability and abstraction purposes. To compose related components, HOCs are powerful tools.
Reasons to use Higher-Order Components:
- Code Reusability: Higher components encapsulate to reuse the common logic here. This we do across many components to reduce code duplication.
- Abstraction of Logic: Higher Order Component often removes the repetitive tasks. Such as data fetching or authentication. This makes our code to have more code readability.
- Composability: Several behaviors or features can be combined into single components using several HOCs hence providing granular control over component behavior and allowing for the creation of sophisticated UIs.
Approach to Create a Higher-Order Component:
- Traditional Higher-Order Components (HOCs): This method is a bit different in that you create a function which takes a component as its argument and then returns an enhanced version of that component. The HOC wraps common logic like data fetching or authentication around the wrapped component.
- Render Props: In this case, the key difference between using render props instead of HOC is that the former introduces a function prop on it so that it can share code or state with other components. Comparing to HOCs Render Props are more flexible than they have greater composability property in some cases.
- Hooks: React has introduced hooks which let users write custom reusable hooks. For some use cases, hooks offer much shorter and easier to understand inter-component communication without resorting to more common HOC styled APIs being used today.
Higher-Order Component Structure:
Step 1: The Higher Order Component (HOC) function is defined as a function that takes a component as its input and returns a new component imbued with additional functionality.
const HigherOrderCompnent = (WrappedComponent) => {
// other code
}
Step 2: The creation of the new component involves defining a class component that wraps the WrappedComponent
and introduces supplementary functionality.
const NewComponent = (props) => {
// code
return (
// ...
);
};
Step 3: In the render()
method of NewComponent
, ensure the seamless passing of all props, including those added by the Higher Order Component (HOC), to the WrappedComponent
.
return <WrappedComponent {...props} additionalProp={additionalProp} />;
Step 4: To complete the Higher Order Component (HOC) function, ensure it returns the NewComponent
so that it can be seamlessly integrated into the application.
const HigherOrderCompnent
= (WrappedComponent) => {
const NewComponent = (props) => {
// other code
};
return NewComponent;
};
When to Use HOCs in your React Code:
Authentication:
In the context of an application featuring multiple routes, where certain routes demand user authentication, a more efficient approach involves the creation of a Higher Order Component (HOC) named withAuth
. This HOC, encapsulating the authentication logic, verifies the user’s authentication status and redirects them to the login page if necessary. By employing this withAuth
HOC, the need for duplicating authentication logic in individual components or routes is eliminated, ensuring consistent and streamlined authentication behavior throughout the application.
Logging:
In scenarios where consistent functionality, such as authentication checks or logging, is needed across specific components, creating Higher Order Components (HOCs) like withAuth
or withLogger
centralizes and simplifies the implementation, reducing redundancy and ensuring uniform behavior.
Styling and Theming:
For efficient styling and theming in a design system, consider using the Higher Order Component (HOC) withTheme
. This HOC provides theme-related props to components, ensuring easy access and application of consistent styles based on the specified theme.
Steps to Create Higher-Order Components in React:
Step 1: Setting up the React Project:
npx create-react-app hoc
Step 2: After creating your project folder, move to it using the following command.
cd hoc
Example: Below is an Example of creating a High-Order Componet.
Javascript
import React from 'react' ;
const withLoading = (WrappedComponent) => {
return function WithLoadingComponent(props) {
const [loading, setLoading] = React.useState( false );
React.useEffect(() => {
setLoading( true );
const timer = setTimeout(() => {
setLoading( false );
}, 2000);
return () => clearTimeout(timer);
}, []);
return <WrappedComponent
{...props} loading={loading} />;
};
};
const MyComponent = ({ loading }) => (
<div>
{loading ? <p>Loading...</p> :
<p>Data Loaded Successfully!</p>}
</div>
);
const EnhancedComponent = withLoading(MyComponent);
function App() {
return (
<>
{ }
<EnhancedComponent />
</>
);
}
export default App;
|
Output:
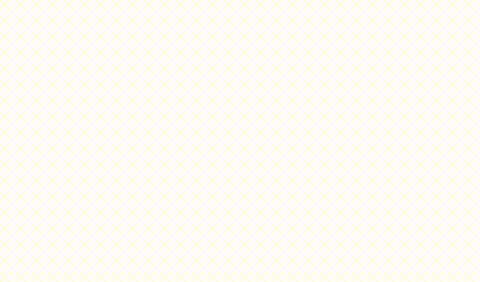
Hoc Change
Conclusion:
In Conclusion, Higher Order Components offers a various range of powerful ways of reusing the functionality of the React Components. Showed various different approaches and followed the steps , which everyone can follow to take Higher Order Components in their React Applications, leading to more scalable, maintainable, and efficient codebases.
Share your thoughts in the comments
Please Login to comment...