What is the use of middleware Redux Saga ?
Last Updated :
06 Oct, 2023
What is Redux Saga?
Redux Saga is a middleware library for Redux that provides an elegant way to handle side effects in your React applications. Side effects can include asynchronous actions like making API calls, managing WebSocket connections, and more.
Before we dive into the implementation details, let’s gain a basic understanding of Redux Saga.
Redux Saga is built on the concept of generators, a feature introduced in ES6. Generators are special functions that can be paused and resumed, making them perfect for handling asynchronous operations in a non-blocking manner. Redux Saga uses these generators to manage side effects in a declarative and testable way.
Redux workflow with a middleware:
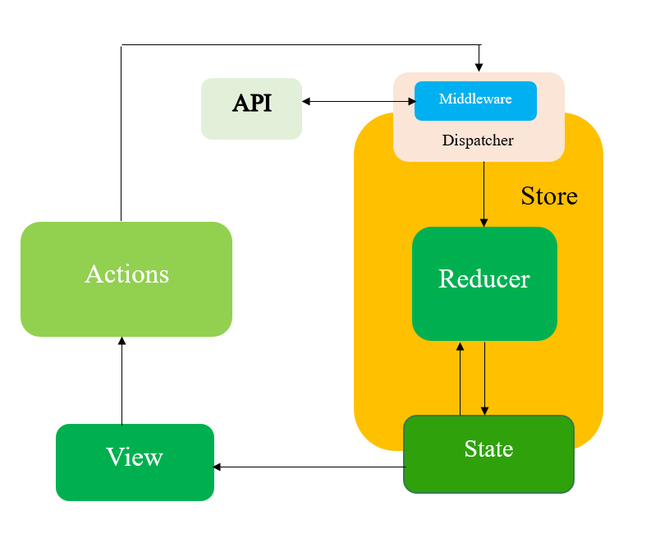
Redux Workflow
Redux-Saga is a library that aims to make application side effects (i.e., asynchronous things like data fetching and impure things like accessing the browser cache) easier to manage, more efficient to execute, easy to test, and better at handling failures.
Setting Up Redux Saga
Step 1: Create a React Application
npx create-react-app saga-app
Step 2: Install Required Packages
cd saga-app
npm install @reduxjs/toolkit react-redux redux-saga
Project Structure
Now create a index.js file in a directory actions inside the src directory.
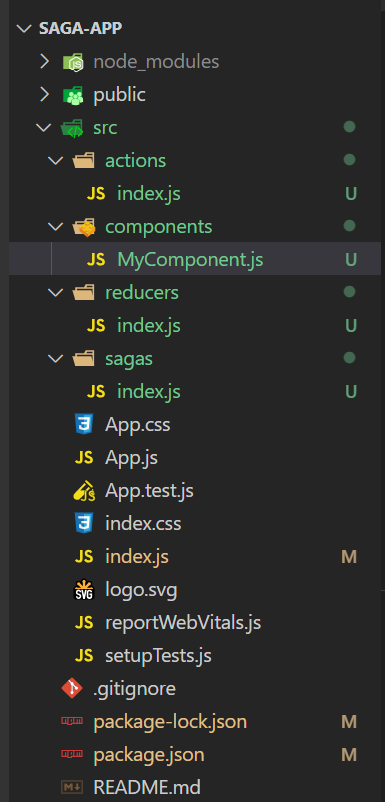
Project Directory
Step 3: Implementing Redux Saga
The saga-app is a React-based web application showcasing Redux Saga, a middleware for handling asynchronous tasks. It fetches data from JSONPlaceholderAPI, a mock RESTful API for testing, and displays it on the web page. Users can delete the displayed data. JSONPlaceholderAPI provides simulated data, making it ideal for testing and development. The app demonstrates how to manage asynchronous actions effectively with Redux Saga in a React application.
Actions
Create action creators in the actions/index.js file. These actions will be used to trigger sagas.
Javascript
export const fetchDataRequest = () => ({
type: "FETCH_DATA_REQUEST" ,
});
export const fetchDataSuccess = (data) => ({
type: "FETCH_DATA_SUCCESS" ,
payload: data,
});
export const fetchDataFailure = (error) => ({
type: "FETCH_DATA_FAILURE" ,
payload: error,
});
export const deleteDataRequest = () => ({
type: "DELETE_DATA_REQUEST" ,
});
|
Reducers
Create reducers in the reducers/index.js file to manage the state based on the actions.
Javascript
const initialState = {
data: null ,
error: null ,
};
const dataReducer = (state = initialState, action) => {
switch (action.type) {
case "FETCH_DATA_SUCCESS" :
return {
...state,
data: action.payload,
error: null ,
};
case "FETCH_DATA_FAILURE" :
return {
...state,
data: null ,
error: action.payload,
};
case "DELETE_DATA_REQUEST" :
return {
...state,
data: null ,
error: null ,
};
default :
return state;
}
};
export default dataReducer;
|
Sagas
In the sagas/index.js file, you can define your sagas. Sagas are generator functions that watch for specific actions and perform asynchronous operations.
Javascript
import { takeEvery, put, call } from "redux-saga/effects" ;
import * as actions from "../actions" ;
const fetchDataFromAPI = async () => {
try {
const response = await fetch(
);
const data = await response.json();
return data;
} catch (error) {
throw error;
}
};
function * fetchData() {
try {
const data = yield call(fetchDataFromAPI);
yield put(actions.fetchDataSuccess(data));
} catch (error) {
yield put(actions.fetchDataFailure(error.message));
}
}
export function * watchFetchData() {
yield takeEvery( "FETCH_DATA_REQUEST" , fetchData);
}
|
Connecting Redux Saga to Your App
In your index.js file, set up the Redux store with middleware to include Redux Saga.
Javascript
import React from "react" ;
import ReactDOM from "react-dom/client" ;
import "./index.css" ;
import App from "./App" ;
import reportWebVitals from "./reportWebVitals" ;
import { configureStore } from "@reduxjs/toolkit" ;
import { Provider } from "react-redux" ;
import createSagaMiddleware from "redux-saga" ;
import rootReducer from "./reducers" ;
import { watchFetchData } from "./sagas" ;
const sagaMiddleware = createSagaMiddleware();
const store = configureStore({
reducer: rootReducer,
middleware: (getDefaultMiddleware) =>
getDefaultMiddleware().concat(sagaMiddleware),
});
sagaMiddleware.run(watchFetchData);
const root = ReactDOM.createRoot(
document.getElementById( "root" )
);
root.render(
<Provider store={store}>
<React.StrictMode>
<App />
</React.StrictMode>
</Provider>
);
|
Using Redux Saga in Components
Now, you can use Redux Saga in your components to trigger asynchronous actions. In your components/MyComponent.js file:
Javascript
import React, { useEffect } from "react" ;
import { useDispatch, useSelector } from "react-redux" ;
import {
fetchDataRequest,
deleteDataRequest,
} from "../actions" ;
const MyComponent = () => {
const dispatch = useDispatch();
const data = useSelector((state) => state.data);
const error = useSelector((state) => state.error);
useEffect(() => {
dispatch(fetchDataRequest());
}, [dispatch]);
const handleDeleteData = () => {
dispatch(deleteDataRequest());
};
return (
<div className= "app-container" >
<h1>Redux Saga App</h1>
<div className= "data-container" >
{data ? (
<div className= "data" >
{JSON.stringify(data)}
</div>
) : (
<div className= "loading" >
{error
? `Error: ${error}`
: "Loading data..." }
</div>
)}
</div>
<button
className= "fetch-button"
onClick={() => dispatch(fetchDataRequest())}
>
Fetch Data
</button>
<button
className= "delete-button"
onClick={handleDeleteData}
>
Delete Data
</button>
</div>
);
};
export default MyComponent;
|
App.js
Javascript
import "./App.css" ;
import MyComponent from "./components/myComponent" ;
function App() {
return <MyComponent />;
}
export default App;
|
App.css
CSS
.app-container {
text-align : center ;
margin : 20px ;
padding : 20px ;
border : 1px solid #ccc ;
border-radius: 5px ;
background-color : #f8f8f8 ;
box-shadow: 0 4px 6px rgba( 0 , 0 , 0 , 0.1 );
}
h 1 {
font-size : 24px ;
margin-bottom : 20px ;
}
.data-container {
margin : 20px 0 ;
}
.data {
font-size : 16px ;
background-color : #e0e0e0 ;
padding : 10px ;
border-radius: 5px ;
}
.loading {
font-size : 16px ;
color : #777 ;
padding : 10px ;
}
.fetch-button {
background-color : #007bff ;
color : #fff ;
padding : 10px 20px ;
font-size : 16px ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
}
.fetch-button:hover {
background-color : #0056b3 ;
}
.delete-button {
background-color : #dc3545 ;
color : #fff ;
padding : 10px 20px ;
font-size : 16px ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
margin-top : 10px ;
}
.delete-button:hover {
background-color : #c82333 ;
}
|
Step 4: use npm command to run the application and output will be on http://localhost:3000/
npm start
Output:
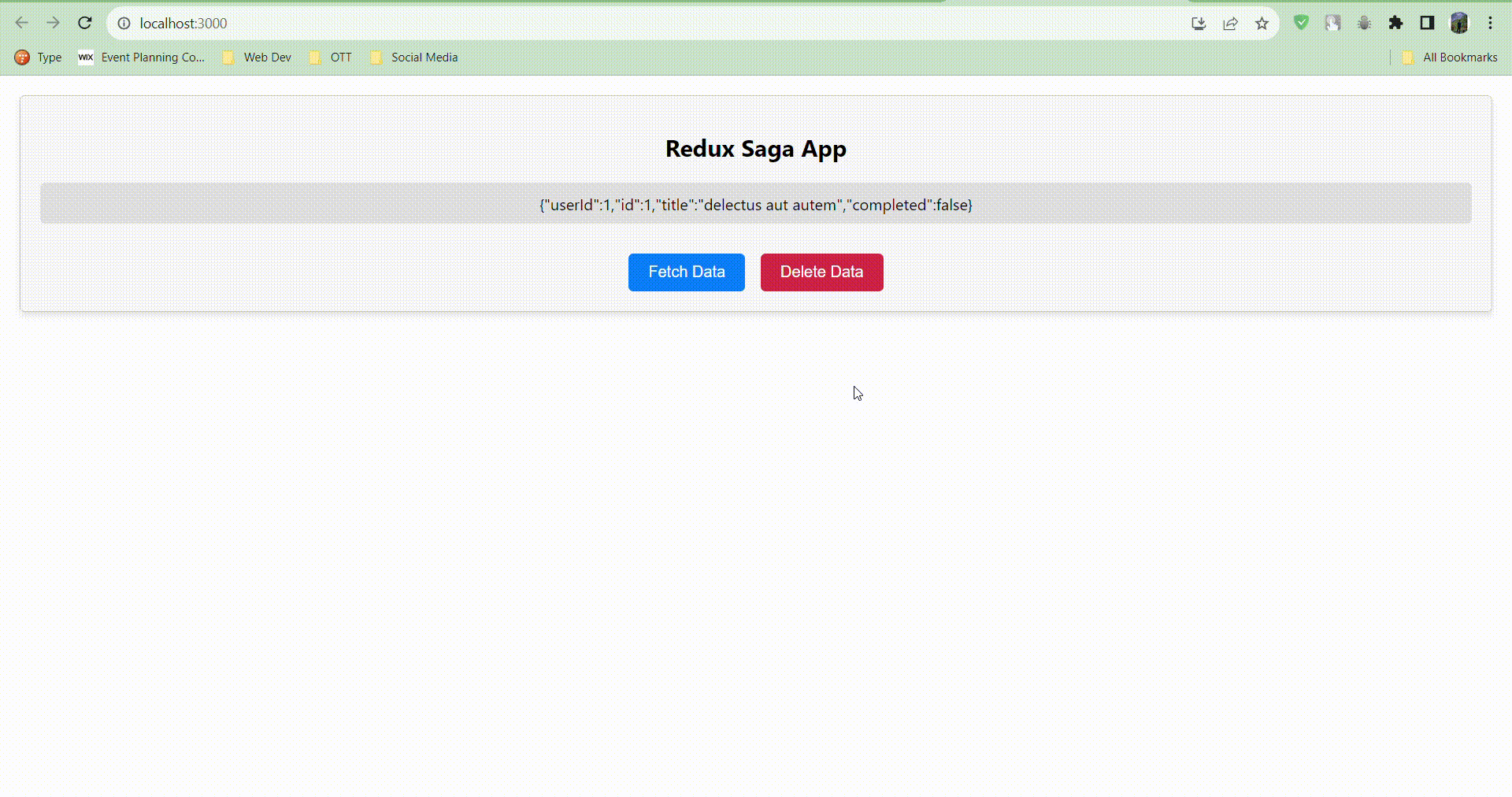
Sample Video
Share your thoughts in the comments
Please Login to comment...