What is the Difference Between required and ng-required in AngularJS ?
Last Updated :
11 Dec, 2023
In web application development, AngularJS is one of the most favorite dynamic JavaScript frameworks that has HTML through script tags, enabling us to augment the HTML attributes using the directives and facilitating the data binding through the expressions.
In AngularJS, we can use certain Directives or Attributes for form validations, that require some form inputs to be filled, conditionally or unconditionally, before submitting the form. For this, we can use the required attribute and ng-required Directive, and both of them have different use cases.Â
In this article, we will learn the difference between the required and ng-required, and understand them with the help of basic examples.
required Attribute
The required Attribute is a standard HTML5 Attribute, which can also be used in angularJS, which makes an input field required before submitting the form. This directive is especially used when we want to make an input field required in all cases, and not conditionally, i.e., depending on some other input’s value.
Syntax
<input required>
Example: In the example below, we will use the required attribute to make the first name and last name input values, as always required in the form.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< form >
< label for = "first_name" >First name:</ label >
< input type = "text"
id = "first_name"
name = "first_name" required>
< label for = "last_name" >Last name:</ label >
< input type = "text"
id = "last_name"
name = "last_name" required>
< button type = "submit" >Submit</ button >
</ form >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent { }
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule } from '@angular/platform-browser' ;
import { HttpClientModule } from '@angular/common/http' ;
import { AppComponent } from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
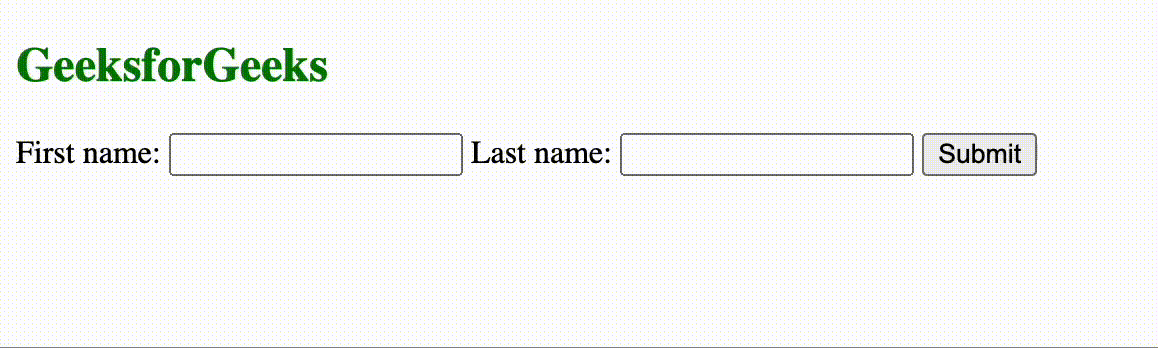
ng-required Directive
The ng-required Directive is a directive provided by AngularJS that allows us to set the validation on an input field in a more dynamic way. This directive is especially used when we want to make an input field required conditionally, i.e., depending on some other input’s value, or a boolean that when returns true, should make the field required, else make the field not required.
Syntax
<input ng-required="booleanExpression">
Here, the “booleanExpression” is basically a javascript expression, which specifies when evaluates to true, it makes the input a required field.
Example: In the below example, we will only make the last name not required field, when the first name input has a non-empty value in it, i.e., the last name field will become not required if there’s some value in the first name field.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< form >
< label for = "first_name" >First name:</ label >
< input type = "text"
id = "first_name"
name = "first_name"
ng-model = "user.firstName" required>
< label for = "last_name" >Last name:</ label >
< input type = "text"
id = "last_name"
name = "last_name"
ng-required = "!user.firstName" >
< button type = "submit" >Submit</ button >
</ form >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent { }
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule } from '@angular/platform-browser' ;
import { HttpClientModule } from '@angular/common/http' ;
import { AppComponent } from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
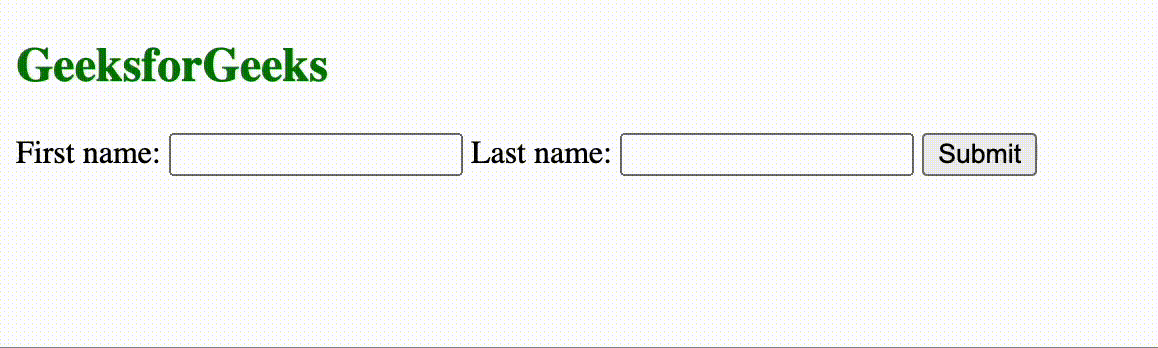
Difference Between required and ng-required
required
|
ng-required
|
Makes an input field required in all cases. It cannot be used to make an input field required conditionally. |
It can be used to make an input field required conditionally. |
It is a standard HTML5 attribute and can be used in any javascript framework |
It is an AngularJS directive, and can only be used in the AngularJS framework. |
It doesn’t require any value to be passed to the attribute |
It required a boolean value to be passed to the attribute |
Share your thoughts in the comments
Please Login to comment...