What is the difference between ng-app and data-ng-app in AngularJS ?
Last Updated :
15 Nov, 2023
In web application development, AngularJS is one of the most favorite dynamic JavaScript frameworks that has HTML through script tags, enabling us to augment the HTML attributes using the directives and facilitating the data binding through the expressions.
In this article, we will see the concepts of ng-app and data-ng-app in AngularJS. As these attributes are fundamental concepts while defining the AngularJS applications with the HTML documents. In this article, we will see both of these directives, their basic implementation, and the core differences between these concepts. Although they are used for the same purpose, but there are some key differences between them.
ng-app
ng-app in AngularJS is the directive that defines the main root element of the Angular application. This ng-app is mainly used to tell AngularJS which part of the HTML document should be treated as the Angular application. We mainly place the ng-app directive as an attribute in the HTML element and that element becomes the main root of the AngularJS application. When the HTML document of the application is been loaded, the AngularJS automatically utilizes the application defined by the ng-app. This mainly processes the DOM within the element that contains the ng-app and binds the data, applied directive to enhance the user interactions.
Syntax
<div ng-app="myApp">
<!-- AngularJS application content -->
</div>
Example: The below example demonstrates the usage of the ng-app directive in AngularJS. Here, as per the user input, we will be changing the text and also the style (color) of the container by giving it a random color at every click.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
AngularJS ng-app Directive
</ title >
< script src =
</ script >
< style >
body {
text-align: center;
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
h1 {
color: green;
}
h3 {
font-style: italic;
color: #333;
}
.container {
border: 2px solid #008CBA;
padding: 20px;
border-radius: 10px;
background-color: white;
margin: 20px auto;
width: 400px;
}
p {
font-size: 18px;
color: #333;
}
button {
background-color: #008CBA;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
}
</ style >
</ head >
< body >
< div ng-app = "myApp" >
< div ng-controller = "MyController"
class = "container" >
< h1 >GeeksforGeeks</ h1 >
< h3 >ng-app directive</ h3 >
< p >{{ name }} is the portal for geeks.</ p >
< label for = "nameInput" >
Change the text:
</ label >
< input type = "text"
id = "nameInput"
ng-model = "name" >
< button ng-click = "updateText()" >
Update Text
</ button >
< button ng-click = "changeStyle()" >
Change Style
</ button >
</ div >
</ div >
< script >
var app = angular.module('myApp', []);
app.controller('MyController', function ($scope) {
$scope.name = 'GeeksforGeeks';
$scope.updateText = function () {
if ($scope.name) {
$scope.name += ' is amazing!';
}
};
$scope.changeStyle = function () {
document.querySelector('.container').style.backgroundColor
= getRandomColor();
document.querySelector('p').style.fontSize = '24px';
};
function getRandomColor() {
var letters = '0123456789ABCDEF';
var color = '#';
for (var i = 0; i < 6 ; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
});
</script>
</ body >
</ html >
|
Output:
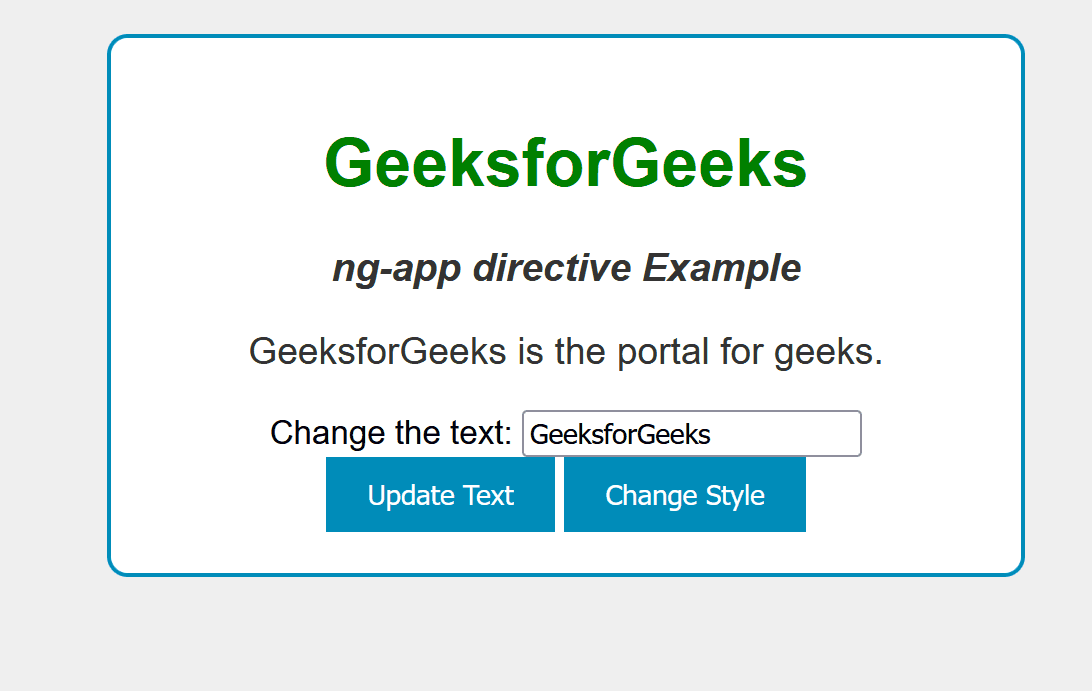
data-ng-app
data-ng-app directive is one of the alternative methods to define the main root element of an AngularJS app in HTML. This directive is also used for bootstrapping the Angular App like the ng-app directive. But this is more used with the HTML validation tools and the parsers. By using the data-ng-app, we can ensure compatibility with the HTML validation tools and parsers that might not be recognized with the custom AngularJS attributes. We can use this data-ng-app directive where there is a need to work with other libraries or tools that rely on strict HTML validation.
Syntax
<div data-ng-app="myApp">
<!-- AngularJS application content -->
</div>
Example: The below example demonstrates the usage of the data-ng-app directive in AngularJS. Here, when the button is clicked, the h1 title is been changed with a different title, and also transition effect is been applied.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
AngularJS data-ng-app Directive
</ title >
< script src =
</ script >
< script src =
</ script >
< style >
body {
text-align: center;
font-family: Arial, sans-serif;
background-color: #f0f0f0;
padding: 20px;
}
h1 {
color: green;
transition: transform 0.5s;
}
h1.ng-hide-add,
h1.ng-hide-remove {
display: inline-block !important;
}
h1.ng-hide {
opacity: 0;
}
h3 {
color: #008CBA;
font-style: italic;
}
.btn {
background-color: #008CBA;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
}
</ style >
</ head >
< body >
< div data-ng-app = "myApp"
ng-controller = "MyController"
style="border: 2px solid #008CBA;
border-radius: 10px;
padding: 20px;
background-color: white;
margin: 0 auto;
width: 400px;">
< h1 ng-style = "h1Style"
class = "animate-fade" >
{{ title }}
</ h1 >
< h3 >data-ng-app Example</ h3 >
< button class = "btn"
ng-click = "changeTitle()" >
Change Title
</ button >
</ div >
< script >
var app = angular.module('myApp', ['ngAnimate']);
app.controller('MyController', function ($scope) {
var titles = ['GeeksforGeeks', 'AngularJS is Awesome'];
var currentTitleIndex = 0;
$scope.title = titles[currentTitleIndex];
$scope.h1Style = {};
$scope.changeTitle = function () {
$scope.h1Style.transform = 'rotate(360deg)';
$scope.title = titles[currentTitleIndex];
currentTitleIndex =
(currentTitleIndex + 1) % titles.length;
};
});
</ script >
</ body >
</ html >
|
Output:
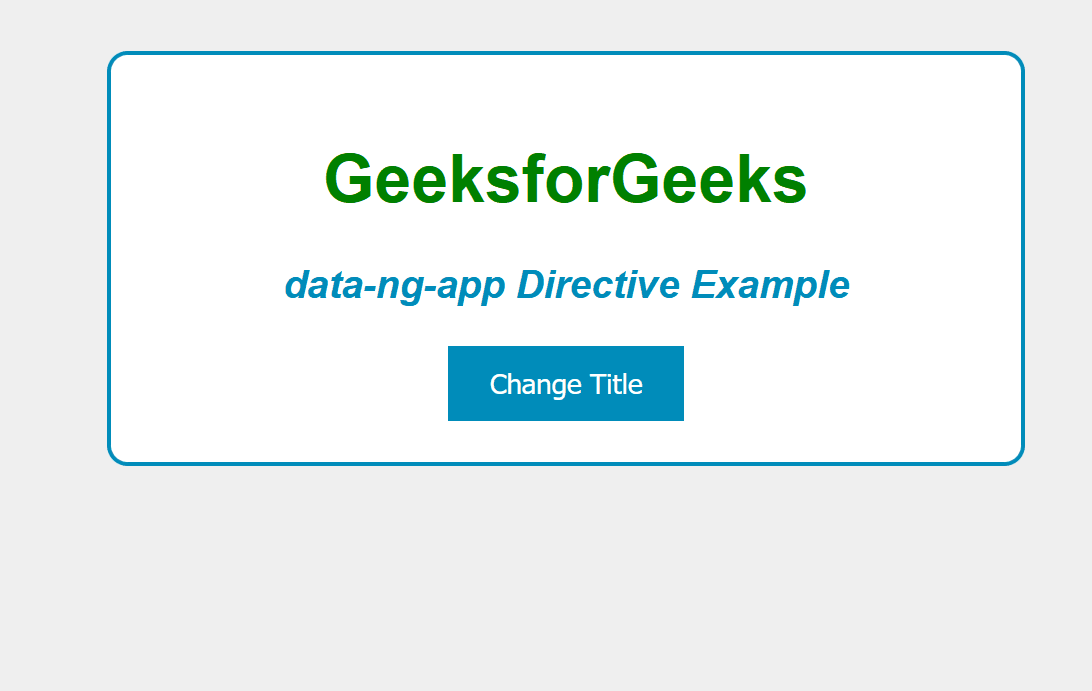
Difference Between ng-app and data-ng-app Directives
Basis
|
ng-app
|
data-ng-app
|
HTML Validation |
May not pass HTML validation tools and parsers. |
Designed to be HTML validation compliant. |
Custom Attributes |
Not recognized by all HTML validators. |
Recognized as a valid data-* attribute. |
Usage |
Commonly used in AngularJS applications. |
Preferred when strict HTML validation is needed. |
Customization |
Less flexible in custom attribute naming. |
Allows more flexibility in attribute naming. |
Data Attribute Convention |
Does not strictly adhere to HTML5’s data attribute convention. |
Does not strictly adhere to HTML5’s data attribute convention. |
Share your thoughts in the comments
Please Login to comment...