What is the difference between ‘@’ and ‘=’ in directive scope in AngularJS ?
Last Updated :
30 Oct, 2023
AngularJS is a popular JavaScript framework, that provides powerful features for building dynamic web applications. When creating custom directives in AngularJS, you may come across the need to define a scope for your directive. The two most common methods to do this are by using the @ and = symbols. These 2 symbols are used to describe the different Binding types while defining the directives with isolated scopes. These symbols govern the passing of the data between the parent scope and the directive’s isolated scope. In this article, we’ll understand the ‘@’ and ‘=’ symbols in AngularJS, along with knowing the differences between them, and then will understand their basic implementation through the examples. Before we proceed into the differences between them, we should learn what are scopes in AngularJS.
Directives Scope
In AngularJS Directives have the ability to possess their scopes. These scopes can be inherited from the parent scope providing control over data flow and encapsulation within your directives. Directives Scope has different types of bindings that define the data flows in and out of the directive.
- Inherited Scope: This refers to a scope that uses the scope as its parent. Any changes made within the directive will affect the parent scope and vice versa. This is particularly useful when you want your directive to share data and functions, with the surrounding controller or template.
- Isolated Scope: This is the scope for a directive that operates independently from its parent. It allows for the creation of self-contained directives, with their data and functions. Isolated scopes are commonly utilized when building components.
AngularJS ‘@’ Symbol
The @ symbol is a type of text binding or one-way binding of strings or interpolated values from the parent scope to the directive’s isolated scope which allows you to pass values as text.
Syntax
scope: {
attributeName: '@'
}
Example: This example describes the basic implementation of the ‘@’ symbol in AngularJS.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< script src =
</ script >
</ head >
< body ng-controller = "MyController" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< div my-directive attribute-name = "{{ msg }}" ></ div >
< script >
angular.module('myApp', [])
.controller('MyController', function ($scope) {
$scope.msg = 'Hello, geek!';
})
.directive('myDirective', function () {
return {
scope: {
attributeName: '@'
},
link: function (scope) {
},
template:
'< p >Directive Message using @ scope: '+
'{{ attributeName }}</ p >'
};
});
</ script >
</ body >
</ html >
|
Output:
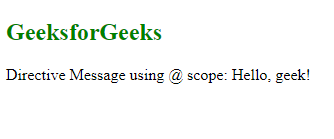
AngularJS ‘=’ Symbol
The ‘=’ symbol is a type of two-way binding between the directive’s isolated scope and the parent scope. In this scope, the changes in the directive’s scope are reflected in the parent scope.
Syntax:
scope: {
attributeName: '='
}
Example: This example describes the basic implementation of the ‘=’ symbol in AngularJS.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< script src =
</ script >
</ head >
< body ng-controller = "MyController" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< div my-directive attribute-name = "msg " ></ div >
< script >
angular.module('myApp', [])
.controller('MyController', function ($scope) {
$scope.msg = 'Hello, geek!';
})
.directive('myDirective', function () {
return {
scope: {
attributeName: '='
},
link: function (scope) {
},
template:
'< p >Directive Message using = scope:'
' {{ attributeName }}</ p >'
};
});
</ script >
</ body >
</ html >
|
Output:
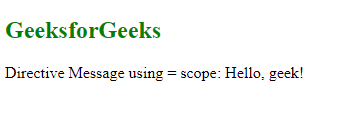
Differences between ‘@’ directive scope and ‘=’ in directive Scope
‘@’ in directive scope
|
‘=’ in directive scope
|
The scope modification is read-only. |
The scope modification is both read and write. |
This scope contains the data types of strings or interpolated values. |
This scope contains variables for two-way data binding. |
It gives one-way or we can say from parent to directive. |
It gives two-way or bidirectional. |
It is used where there is static content or simple data transfer. |
It is used when there is dynamic data binding and synchronization. |
It is commonly used with simple text values like titles, labels, or messages. |
We use this when there are interactive components with real-time updates. |
Share your thoughts in the comments
Please Login to comment...