How to access the Scope from outside JS Function in AngularJS ?
Last Updated :
28 Oct, 2023
In the AngularJS application, we generally need to access the scope from the outside JS function. This can be done using the global scope object like $rootScope or the AngularJS services. Accessing the scope from the outside of JS functions is to enable the manipulation of data in the scope of the application in response to user actions. In this article, we will see how we can access the scope from outside the JavaScript function in AngularJS.
Steps for Configuring AngularJS Application
The below steps will be followed to configure the AngularJS Application:
- Create a new folder for the project. We are using the VSCode IDE to execute the command in the integrated terminal of VSCode.
mkdir access-scope
cd access-scope
- Create the index.html file in the newly created folder, we will have all our logic and styling code in this file. We can also create separate files for HTML, CSS, and JS:
We will explore the above approaches & will understand their implementation through the illustration.
Accessing Using $rootScope Object
In this approach, we are using the $rootScope object. This object refers to an object which is accessible from everywhere in the application. This object is mainly a global storage for the data which is to be shared throughout the controllers in the application. Using this object we can update the data within the scope from the functions that are not connected to the specific controller in the application. In the below example, there is the message that is set in the $rootScope, making this globally accessible throughout the application. Here, mainly we are performing the dependy injection where we are simply injecting the objects in our controller function, which includes the scope itself.
Example: Below is an example that demonstrates accessing the scope from outside the js function in AngularJS using $rootScope Object.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
AngularJS Scope Access
</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.container {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.2);
}
h1 {
color: green;
}
h3 {
color: rgb(0, 0, 0);
}
p {
font-size: 18px;
margin: 20px 0;
}
button {
background-color: #007BFF;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
</ style >
</ head >
< body ng-controller = "myController" >
< div class = "container" >
< h1 >
GeeksforGeeks
</ h1 >
< h3 >
Approach 1: Using $rootScope
</ h3 >
< p >
Message: {{ message }}
</ p >
< button ng-click = "updateMessage()" >
Update Message
</ button >
< button ng-click = "resetMessage()" >
Reset Message
</ button >
</ div >
< script >
var app = angular.module('myApp', []);
app.controller('myController', function ($scope, $rootScope) {
// Set an initial message in the root scope
$rootScope.message =
"Hello from $rootScope!";
// Function to update the message in the root scope
$scope.updateMessage = function () {
$rootScope.message =
"Message updated using $rootScope!";
};
// Function to reset the message to its initial value
$scope.resetMessage = function () {
$rootScope.message =
"Hello from $rootScope!";
};
});
</ script >
</ body >
</ html >
|
Output
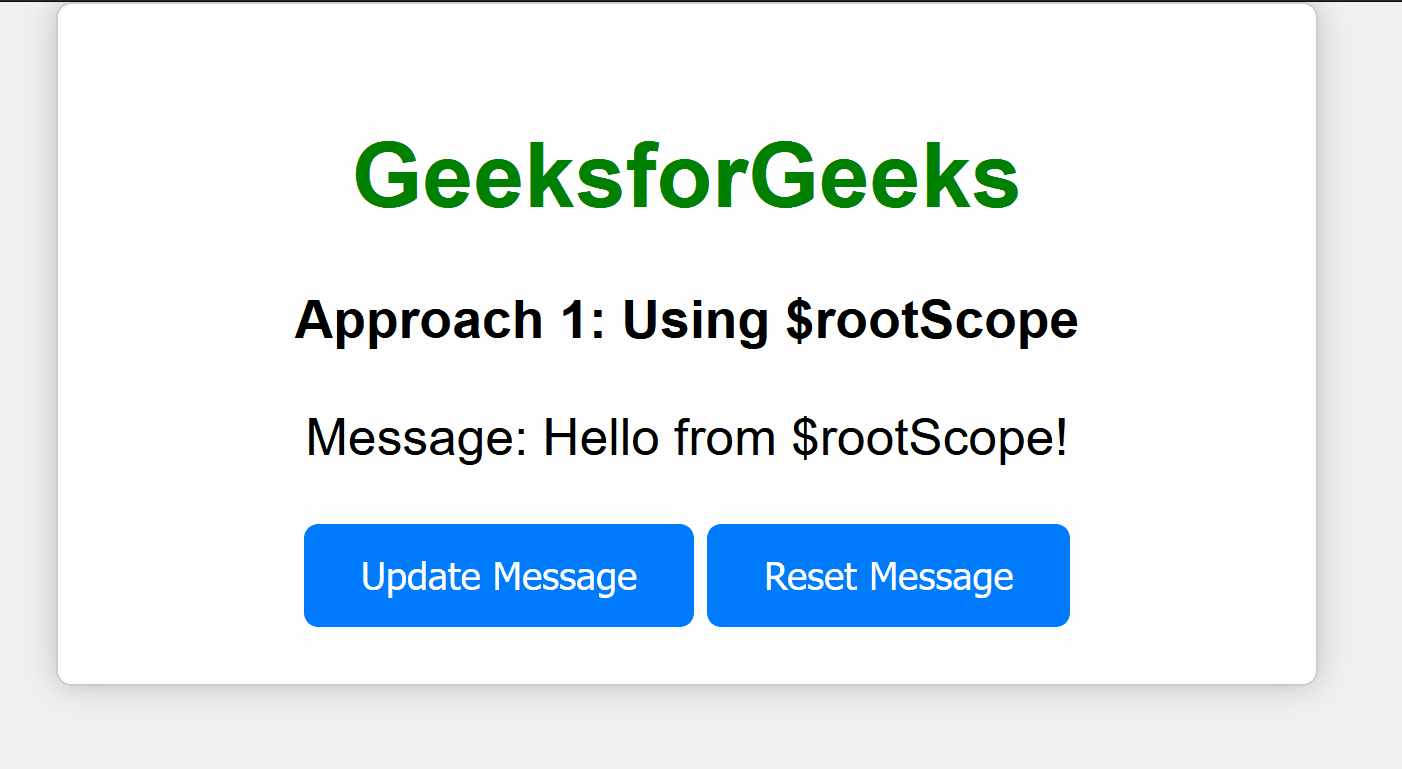
Using AngularJS Services
In this approach, we are accessing the scope from outside the JS function by using the AngularJS services. Here, we have used the messageService which mainly wraps the message variable and allows it to be accessed from within the controller. When the user performs the edit of the message in the input field, the updateServiceMessage() function is been actioned and the message is updated using the service.
Example: Below is an example that demonstrates accessing the scope from outside the js function in AngularJS using Services.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
AngularJS Scope Access
</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.container {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.2);
}
h1 {
color: green;
}
h3 {
color: #333;
}
p {
font-size: 18px;
margin: 20px 0;
}
.input-field {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
</ style >
</ head >
< body ng-controller = "myController" >
< div class = "container" >
< h1 >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using AngularJS Services
</ h3 >
< p >Edit the message:</ p >
< input class = "input-field"
type = "text"
ng-model = "message"
ng-change = "updateServiceMessage()" />
< p >
The message is from the service:
{{ serviceMessage }}
</ p >
</ div >
< script >
var app = angular.module('myApp', []);
app.service('messageService', function () {
var message = "Hello, World!";
this.getMessage = function () {
return message;
};
this.setMessage = function (newMessage) {
message = newMessage;
};
});
app.controller('myController',
function ($scope, messageService) {
$scope.serviceMessage =
messageService.getMessage();
$scope.updateServiceMessage = function () {
messageService.setMessage($scope.message);
$scope.serviceMessage =
messageService.getMessage();
};
});
</ script >
</ body >
</ html >
|
Output
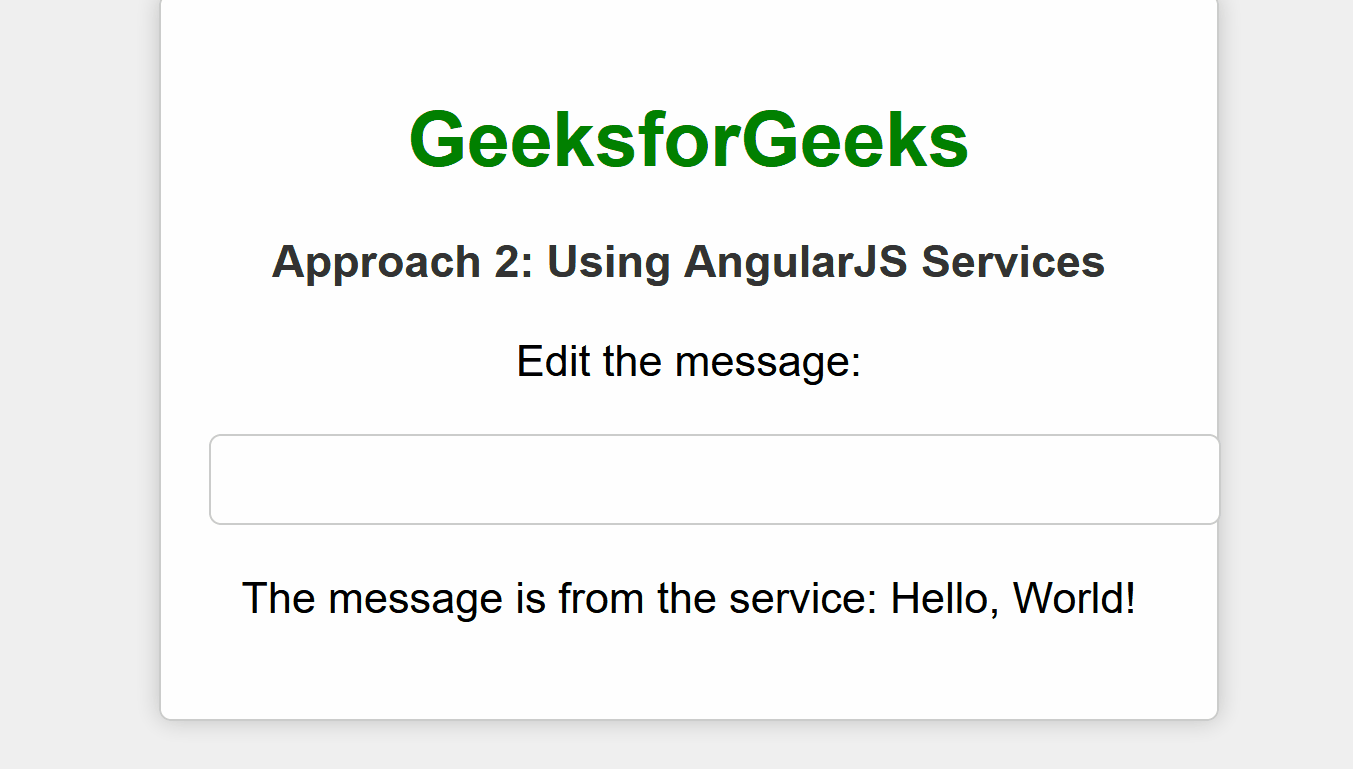
Share your thoughts in the comments
Please Login to comment...