How to Handle Anchor Hash Linking in AngularJS ?
Last Updated :
25 Aug, 2023
AngularJS Anchor Hash Linking consists of developing a proper and efficient navigation experience in the one-page application. This feature of AngularJS enables users and developers to properly and smoothly scroll to specific sections of a webpage just by clicking on the navigation links, rather than performing page reload every time. In this article, we will see different approaches that will allow us to handle anchor hash linking in AngularJS. So by using this approach, we can build our own one-page application with a smooth scrolling effect.
Steps to handle anchor hash linking in AngularJS
The below steps will be followed for handling anchor hash linking in AngularJS Applications.
Step 1: Create a new folder for the project. As we are using the VSCode IDE, so we can execute the command in the integrated terminal of VSCode.
mkdir handle-anchor-hash
cd handle-anchor-hash
Step 2: Create the following files in the newly created folder, in this files we will be having our all logic and styling code:
- index.html
- app.js
- styles.css
We will understand the above concept with the help of suitable approaches & will understand its implementation through the illustration.
Approach 1: Using AngularJS Controllers (using ‘$location‘ and ‘$anchorScroll‘ services)
In this approach, we have created a smooth-scrolling one-page application. Here, the AngularJS controller, which is “MainController” is actually responsible for completely managing the scrolling behaviors in the application. We have used 2 different services which are ‘$location‘ and ‘$anchorScroll‘, using this we can properly handle anchor hash linking and provide an efficient navigation experience in the application. When we click on the navigation link, the controller’s function updates its active section, and adjusts the URL hash, then we can scroll the page to the desired data.
Example: Below is an example that showcases the handling of anchor hash linking in AngularJS.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
AngularJS Hash Linking Example (Approach 1)
</ title >
< link rel = "stylesheet"
type = "text/css"
href = "styles.css" >
</ head >
< body ng-controller = "MainController" >
< div class = "container" >
< div class = "header" >
< h2 class = "geeks-title" >
GeeksforGeeks
</ h2 >
< h3 >AngularJS Hash Linking</ h3 >
</ div >
< div class = "navbar" >
< a class = "nav-link"
ng-repeat = "item in items"
ng-href = "#{{item.id}}"
ng-click = "scrollToItem(item.id)" >
{{item.name}}
</ a >
</ div >
< div class = "content" >
< div class = "item"
ng-repeat = "item in items"
id = "{{item.id}}"
ng-class = "{ 'active': activeItemId === item.id }" >
< h4 >{{item.name}}</ h4 >
< div class = "description" >
{{item.description}}
</ div >
</ div >
</ div >
</ div >
< script src =
</ script >
< script src = "app.js" ></ script >
</ body >
</ html >
|
CSS
body, h 2 , h 3 , ul, li {
margin : 0 ;
padding : 0 ;
}
.container {
font-family : Arial , sans-serif ;
margin : 0 auto ;
max-width : 800px ;
padding : 20px ;
box-sizing: border-box;
}
.header {
text-align : center ;
margin-bottom : 20px ;
}
.navbar {
background-color : #f5f5f5 ;
padding : 10px ;
text-align : center ;
display : flex;
justify- content : center ;
}
.nav-link {
margin : 0 10px ;
cursor : pointer ;
}
.item {
margin-bottom : 50px ;
border : 1px solid #ccc ;
padding : 10px ;
transition: background-color 0.3 s ease-in-out;
}
.item.active {
background-color : #ffeeba ;
}
.description {
margin-top : 10px ;
font-size : 14px ;
}
.geeks-title {
color : green ;
}
|
Javascript
var app = angular.module( 'myApp' , []);
app.controller( 'MainController' , [ '$scope' ,
'$location' ,
'$anchorScroll' ,
function ($scope, $location, $anchorScroll) {
$scope.items = [
{ id: 'courses' ,
name: 'Courses' ,
description:
'Discover a wide range of online courses offered by GeeksforGeeks,
covering various programming topics and technologies.' },
{ id: 'articles' ,
name: 'Articles' ,
description:
'Access a vast collection of articles on programming, algorithms,
data structures, and more at GeeksforGeeks.' },
{ id: 'practice' ,
name: 'Practice' ,
description:
'Improve your coding skills by practicing coding challenges, quizzes,
and exercises available on the GeeksforGeeks platform.' }
];
$scope.activeItemId = '' ;
$scope.scrollToItem = function (itemId) {
$scope.activeItemId = itemId;
$location.hash(itemId);
var scrollElement = document.getElementById(itemId);
scrollElement.scrollIntoView({ behavior: 'smooth' });
};
}]);
|
Output:
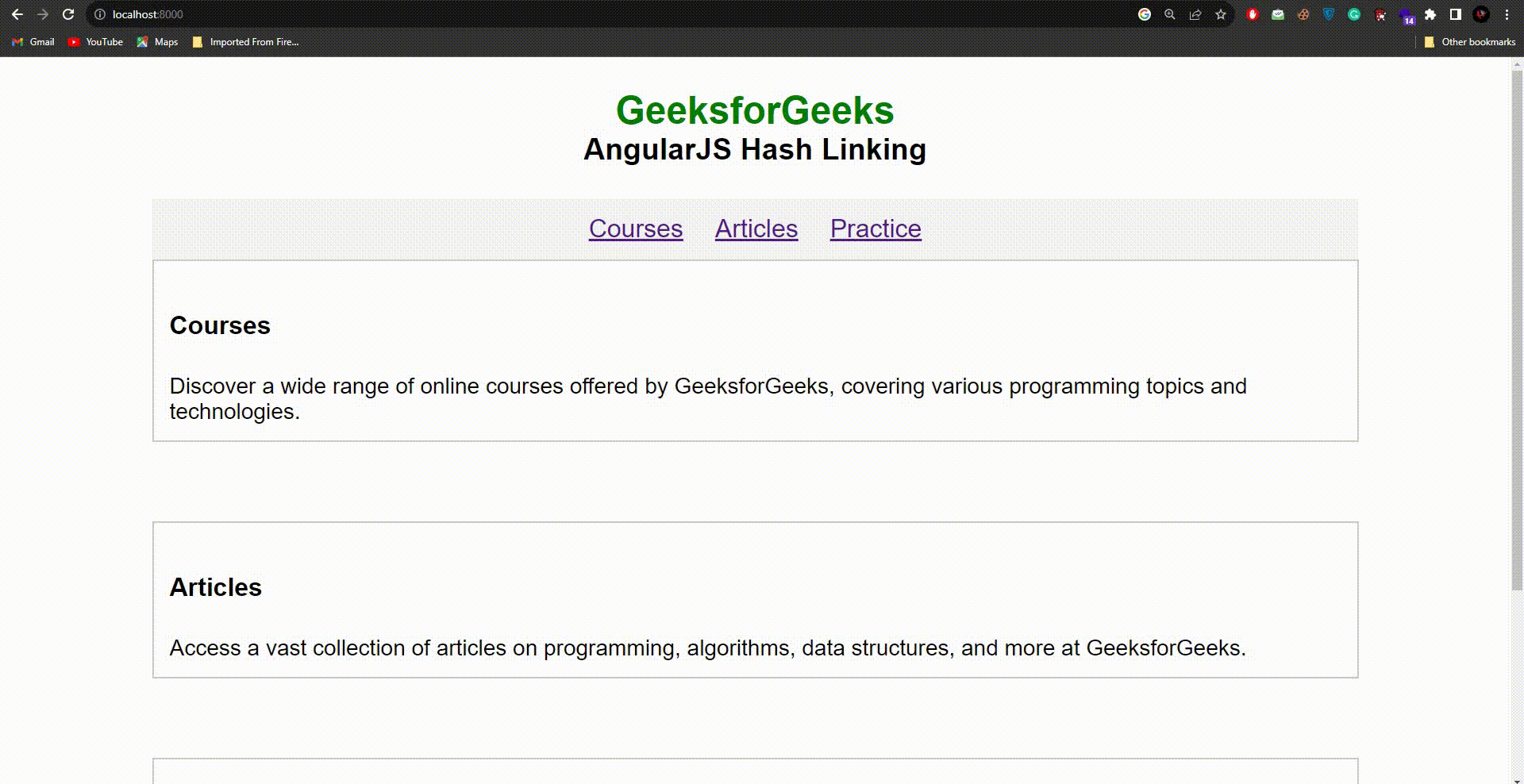
Approach 2:
In this approach, we are using the Directives for handling the hash linking in AngularJS. There is the customer directive as ‘hash-link‘, which basically generates the navigation links in the navigation bar. If the user clicks the link, then the directive uses the ‘scrollIntoView‘ function which scrolls to the particular item’s description. The directives used here use AngularJS capabilities to actually develop dynamic navigation and scrolling.
Example: Below is an example that showcases the handling of anchor hash linking in AngularJS using Directives.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >
AngularJS Hash Linking Example (Directives Approach)
</ title >
< link rel = "stylesheet"
type = "text/css"
href = "styles.css" >
</ head >
< body ng-controller = "MainController" >
< div class = "container" >
< div class = "header" >
< h2 class = "geeks-title" >
GeeksforGeeks
</ h2 >
< h3 >
AngularJS Hash Linking (Directives Approach)
</ h3 >
</ div >
< div class = "navbar" >
< hash-link ng-repeat = "section in sections"
target-id = "{{section.id}}"
content = "{{section.name}}" >
</ hash-link >
</ div >
< div class = "content" >
< div class = "item"
ng-repeat = "section in sections"
id = "{{section.id}}" >
< h4 >{{section.name}}</ h4 >
< div class = "description" >
{{section.description}}
</ div >
</ div >
</ div >
</ div >
< script src =
</ script >
< script src = "app.js" ></ script >
</ body >
</ html >
|
CSS
body, h 2 , h 3 , ul, li {
margin : 0 ;
padding : 0 ;
}
.container {
font-family : Arial , sans-serif ;
margin : 0 auto ;
max-width : 800px ;
padding : 20px ;
box-sizing: border-box;
}
.header {
text-align : center ;
margin-bottom : 20px ;
}
.navbar {
background-color : #f5f5f5 ;
padding : 10px ;
text-align : center ;
display : flex;
justify- content : center ;
}
.nav-link {
margin : 0 10px ;
cursor : pointer ;
text-decoration : none ;
color : #333 ;
font-weight : bold ;
transition: color 0.3 s;
}
.nav-link:hover {
color : #007bff ;
}
.item {
margin-bottom : 50px ;
border : 1px solid #ccc ;
padding : 10px ;
transition: background-color 0.3 s ease-in-out;
position : relative ;
}
.item.active {
background-color : #ffeeba ;
}
.item.active::before {
content : '' ;
position : absolute ;
width : 4px ;
height : 100% ;
left : -4px ;
top : 0 ;
background-color : #007bff ;
opacity: 1 ;
transition: opacity 0.3 s;
}
.description {
margin-top : 10px ;
font-size : 14px ;
opacity: 0.7 ;
transition: opacity 0.3 s, color 0.3 s;
color : #333 ;
}
.item.active .description {
opacity: 1 ;
color : #007bff ;
font-weight : bold ;
}
.geeks-title {
color : green ;
}
|
Javascript
var app = angular.module( 'myApp' , []);
app.directive( 'hashLink' , function () {
return {
restrict: 'E' ,
template:
'<span class="nav-link" ng-click="scrollToTarget(targetId)">{{content}}</span>' ,
scope: {
targetId: '@' ,
content: '@'
},
link: function (scope, element, attrs) {
scope.scrollToTarget = function (targetId) {
var scrollElement = document.getElementById(targetId);
scrollElement.scrollIntoView({ behavior: 'smooth' });
scope.highlightSection(targetId);
};
scope.highlightSection = function (sectionId) {
var descriptions =
document.querySelectorAll( '.description' );
descriptions.forEach( function (description) {
description.style.color = '#333' ;
});
var clickedDescription =
document.querySelector(` #${sectionId} .description`);
if (clickedDescription) {
clickedDescription.style.color = '#007bff' ;
}
};
}
};
});
app.controller( 'MainController' , [ '$scope' , function ($scope) {
$scope.sections = [
{ id: 'introduction' ,
name: 'Introduction' ,
description: 'Article on How to handle anchor hash
linking in AngularJS' },
{ id: 'implementation' ,
name: 'Implementation' ,
description: 'Implementing hash linking using AngularJS directives.' },
{ id: 'example' ,
name: 'Example' ,
description: 'This is example of hash linking.' }
];
}]);
|
Output:
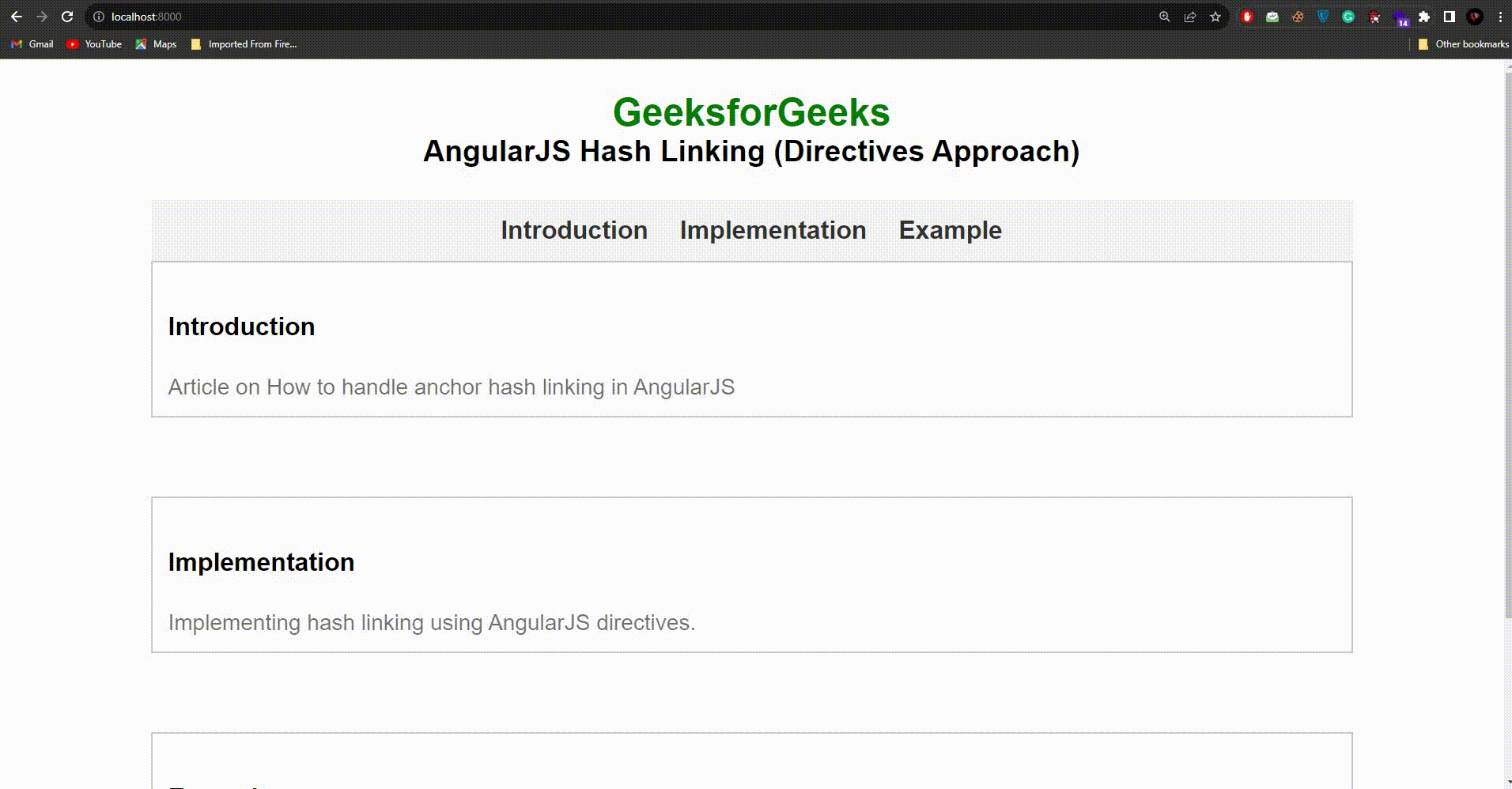
Share your thoughts in the comments
Please Login to comment...