What is HTML DOM ?
Last Updated :
09 Jan, 2024
HTML DOM stands for the Document Object Model is a tree-like structure that offers dynamic interaction and manipulation of the content, structure, and style of an HTML document. It is an interface for web documents. Based on user activity The HTML DOM offers to create interactive web pages where elements can be added, removed, or modified.
Significance of HTML DOM
The HTML DOM defines the logical structure of the document and how a document is accessed and manipulated. The DOM is automatically created by the browser when the HTML document is parsed. It is the process of creating the DOM that involves converting the HTML document into a tree-like structure, which can be further manipulated with JavaScript. Additionally, The HTML DOM is widely used for various real-world applications like updating content dynamically, Form Validation, AJAX Requests, Event Handling, and Web Scraping.
HTML DOM Structure
The image below shows the tree-like structure of the Document Object Model. The below table shows the properties of the document object with their description.
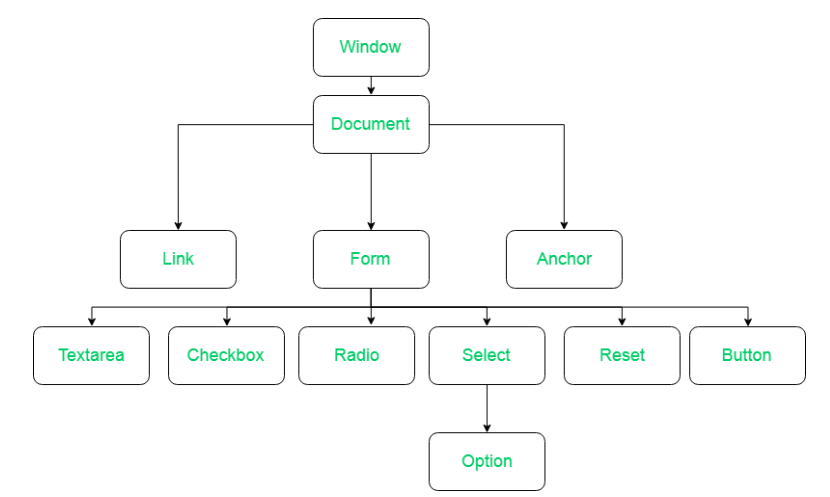
Representation of DOM
The below table illustrates the various Objects of the DOM hierarchy with their description.
Window Object |
It is an object of the browser which is at top of the hierarchy. |
Document object |
When an HTML document is loaded on the web browser into a window, it becomes a document object. |
Form Object |
The form object is defined as the form tags. |
Link Object |
The Link object is defined as the link tags. |
Anchor Object |
The anchor object is defined as the a tags with href attributes. |
Form Control Elements |
Form control elements such as text fields, buttons, radio buttons, checkboxes used when form is created which wrapped inside form tags |
Adding New Element to the DOM
We can add new element dynamically by creating a new element using createElement, and finally appending the new element to the DOM with methods like appendChild.
Example: In the example, we will see how to add new elements to the DOM.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >HTML DOM</ title >
</ head >
< body >
< h1 id = "GeeksforGeeks"
style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Adding new element to the DOM
</ h3 >
< button id = "btn" >
Click to Add Element
</ button >
< script >
const btn =
document.getElementById("btn");
btn.addEventListener("click", function () {
// Create a new paragraph element
const newPara =
document.createElement("p");
newPara.textContent =
"This paragraph element was added dynamically on click.";
// Append the new element to the body
document.body
.appendChild(newPara);
});
</ script >
</ body >
</ html >
|
Output:
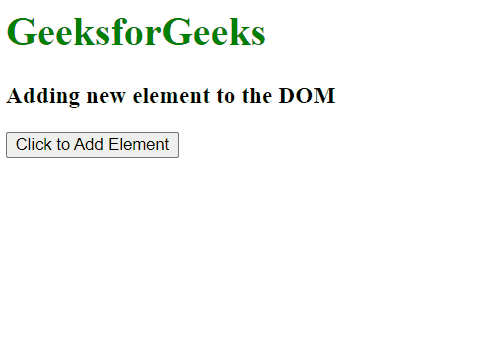
Techniques for Finding DOM Elements
The below table illustrates the various techniques for Finding DOM Elements with their description and syntax.
id |
It returns the HTML element with the specified id value. |
document.getElementById(“mybox”) |
tag name |
It returns all the HTML element with the specified name value. |
document.getElementsByTagName(“a”) |
class name |
It returns the element with the specified id value. |
document.getElementsByClassName(“box”) |
CSS selectors |
Used to find any element(s) for styling purpose. |
document.querySelectorAll(selectors) |
Example: The example illustrates how to find the element and make operations using CSS Selectors property.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >HTML DOM</ title >
</ head >
< body >
< h1 id = "mybox" >GeeksforGeeks</ h1 >
< h3 >
HTML DOM using
property querySelectorAll
</ h3 >
< script >
const element =
document.querySelectorAll("h1")[0]
element.style.color = "green";
</ script >
</ body >
</ html >
|
Output:
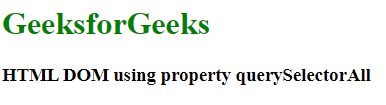
Event Listeners
Event Listeners offers the addEventListener() method which is an inbuilt function in JavaScript responsible for handling events like clicks or form submissions. There are various event defined including Click Event Listener, Mouseover Event Listener, Mouseout Event Listener, Keydown Event Listener, Keyup Event Listener etc.
Accessing the DOM
The below table illustrates the various techniques for Accessing DOM Elements with their description.
document.anchors |
The document.anchors property returns a collection of all anchor elements (<a>) in the current HTML document. |
document.forms |
The document.forms property returns a collection of all form elements (<form>) in the current HTML document. |
document.images |
The document.images property returns a collection of all image elements (<img>) in the current HTML document. |
document.links |
The document.links property returns a collection of all links elements (<a>) with a valid href attribute in the current HTML document. |
document.scripts |
The document.scripts property returns a collection of all anchor elements (<scripts>) in the current HTML document. |
Example: The example illustrates the properties for finding HTML elements by HTML Object Collection document.anchor.
HTML
<!DOCTYPE html>
< html >
< head >
< title >DOM anchors collection</ title >
< style >
.gfg {
color: green;
}
</ style >
</ head >
< body >
< h1 class = "gfg" >
GeeksforGeeks
</ h1 >
< h3 >DOM anchors Collection</ h3 >
< a >GeeksforGeeks</ a >< br >
< a >Placement</ a >< br >
< a >Courses</ a >
< br >< br >
< button onclick = "afunGeeks()" >
Submit
</ button >
< p id = "pid" ></ p >
< script >
function afunGeeks() {
let x = document.anchors.length;
document.getElementById("pid").innerHTML =
"Number of Anchors elements: " + x;
}
</ script >
</ body >
</ html >
|
Output:
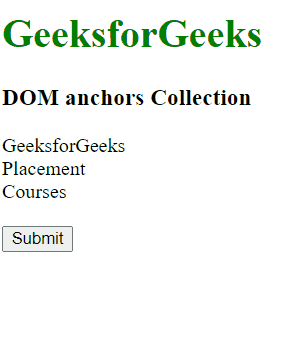
Share your thoughts in the comments
Please Login to comment...