What is Angular Expression ?
Last Updated :
06 Nov, 2023
Angular is a great, reusable UI (User Interface) library for developers that help in building attractive, and steady web pages and web application. In this article, we will learn about Angular expression.
Angular Expression
An Angular Expression is a code snippet that can be simple or complex JavaScript-like code, like, the variable references, function calls, operators, and filters, etc., written within double curly braces {{ }} in order to evaluate & display dynamic values or perform calculations in the template.
Different Use Cases of Angular Expressions
Angular expressions are commonly used for various purposes, which are described below:
- Displaying Data: Angular expressions are extremely useful for displaying data obtained from variables or properties within templates.
- Performing Calculations: Expressions allow us to perform calculations and display the results dynamically.
- Conditional Rendering: Angular expressions can be used with directives like ng-if to conditionally render elements based on data conditions.
- Filtering Data: We can use Angular filters in expressions to format and filter data before displaying it.
- Event Handling: It can be used to handle user interactions and trigger actions in response to events.
Syntax
In the below syntax, the msg property is bound to the <p> element and its value will be displayed.
// app.component.html
<p> {{ mymsg }} </p>
// app.component.ts
export class AppComponent {
mymsg = 'Hello, Geek!';
}
Approach
There are different approaches to implementing the Angular Expression, among which the most commonly used approach are:
- By Interpolation
- By creating the Custom Directives
We’ll explore both the approaches & understand their basic implementation through the examples.
By Interpolation
This is the most common and used method to use angular expression. In this, we use the {{ }} brackets to enclose the expressions within it. Interpolation is a way to transfer the data from a TypeScript code to an HTML template (view), i.e. it is a method by which we can put an expression in between some text and get the value of that expression.
Example: The below example demonstrates the basic usage of Expressions in the Angular application.
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
template: `
<h1>Welcome to {{ appName }}</h1>`,
})
export class AppComponent {
appName = 'GeeksforGeeks' ;
}
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { AppComponent } from './app.component' ;
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
bootstrap: [AppComponent],
})
export class AppModule { }
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >GFG App</ title >
</ head >
< body >
< app-root ></ app-root >
</ body >
</ html >
|
Output:
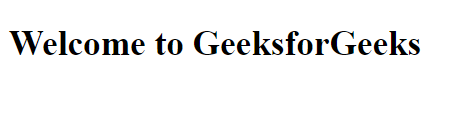
By creating the Custom Directives
Another approach for using expressions in Angular is to use directives, like ngFor or ngModel, etc. to create custom directives, in order to bind expressions to specific element properties or events.
Example: The below example demonstrates the usage of expressions with the custom directive, in order to bind in the Angular application. Here, we are using the directive binding with [textContent] for displaying the geekName, geekCourse, etc along with the standard property binding using our first approach interpolation to display the geekRank property.
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-gfg-profile' ,
template: `
<h2>GeeksforGeeks Profile</h2>
<div>
<p>Geek name:
<span [textContent]= "geekName" ></span></p>
<p>Course:
<span [textContent]= "geekCourse" ></span></p>
<p>Rank: <span>{{ geekRank }}</span></p>
</div>`,
})
export class GfgProfileComponent {
geekName = 'Tarun Singh' ;
geekCourse = 'DSA Self Paced' ;
geekRank = 5;
}
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { GfgProfileComponent }
from './gfg-profile/gfg-profile.component' ;
@NgModule({
declarations: [GfgProfileComponent],
imports: [BrowserModule],
bootstrap: [GfgProfileComponent],
})
export class AppModule { }
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >gfg</ title >
</ head >
< body >
< app-gfg-profile ></ app-gfg-profile >
</ body >
</ html >
|
Output:
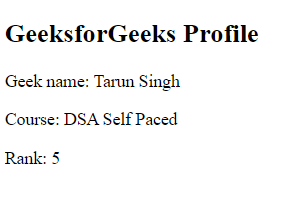
Share your thoughts in the comments
Please Login to comment...