What are the Types of Linking Function in Angular ?
Last Updated :
26 Jul, 2023
When working with Angular directives, understanding the concept and types of linking functions is essential. Linking functions are a crucial part of the directive’s lifecycle, allowing developers to interact with the directive’s DOM element and scope. In this article, we will explore the different types of linking functions in Angular and how to use them effectively.
Linking Function
The Linking function in Angular provides a bridge between the directive and the template’s DOM elements. They play a significant role during the linking phase of the directive’s lifecycle. The linking function executes once the directive has been compiled and linked to the template, giving developers the ability to manipulate DOM elements, handle events, and update scope data.
Types of Linking Functions
In Angular, there are two types of linking functions:
Pre-linking Function
The pre-linking function executes before the child elements are linked to the parent directive. It allows developers to perform tasks before Angular compiles and links the child elements.
Syntax:
function preLinkingFunction(scope: ng.IScope, element: JQLite, attrs: ng.IAttributes):
void {
// Your code here
}
Post-linking Function
The post-linking function executes after the child elements are linked to the parent directive. It enables developers to interact with the DOM after Angular has finished linking the child elements.
Syntax:
function postLinkingFunction(scope: ng.IScope, element: JQLite, attrs: ng.IAttributes):
void {
// Your code here
}
Parameter Values:
It is basically a callback function that will retrieve the following arguments:
- scope: This parameter represents the directive’s scope. You can use it to interact with the parent scope and manipulate data.
- element: This parameter represents the jqLite-wrapped element of the directive’s DOM. You can use it to modify the element’s properties and add event listeners.
- attrs: This parameter contains the attributes associated with the directive as key-value pairs.
- controller: It is an optional parameter that permits to retrieve the controller instances that are associated with the directive.
Approach 1: Using Linking Function to Manipulate Scope Data
In this approach, we will demonstrate how to use the linking function to interact with the directive’s scope and update data. Here, the basic HTML template declared that contains an <button> element with ng-mouseover and ng-mouseleave attributes, & calls the onHover() and onHoverOut() functions when the mouse hovers over and leaves the <button>. We then define two event handler functions, onHover() and onHoverOut().
- onHover(): This function is called when the mouse hovers over the <button>. It sets this.message to “Hovered!”, changing the displayed message.
- onHoverOut(): This function is called when the mouse leaves the <button>. It sets this.message back to “Not hovered.”, resetting the displayed message.
Example: This example illustrates the Linking function in Angular.
HTML
< div >
< button (mouseover)="onHover()"
(mouseleave)="onHoverOut()">
Hover over me!
</ button >
< div >{{ message }}</ div >
</ div >
|
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
message: string = "Not hovered." ;
onHover() {
this .message = "Hovered!" ;
}
onHoverOut() {
this .message = "Not hovered." ;
}
}
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { AppComponent } from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
Approach 2: Combining Pre-linking and Post-linking Functions
In this approach, we will combine the pre-linking and post-linking functions into a single function. Here, when the mouse Hovers over the element, the this.messageStyle.color will change the background color from light blue to yellow.
Example: This is another example that demonstrates the Linking Function by combining Pre-linking and Post-linking Functions.
HTML
< div customDirective>
< button >Hover over me!</ button >
< div >{{ message }}</ div >
</ div >
|
Javascript
import { Component, Directive, Input }
from '@angular/core' ;
@Directive({
selector: '[customDirective]'
})
export class CustomDirective {
@Input() message: string;
messageStyle: any = {};
constructor() {
this .messageStyle = {
color: 'black'
};
}
toggleColor() {
this .messageStyle.color =
this .messageStyle.color === 'yellow' ? 'lightblue' : 'yellow' ;
}
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent { }
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { AppComponent, CustomDirective }
from './app.component' ;
@NgModule({
declarations: [
AppComponent,
CustomDirective
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
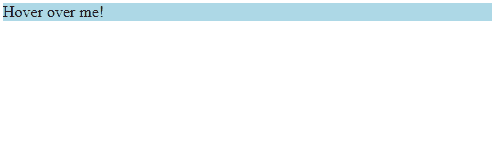
output
Share your thoughts in the comments
Please Login to comment...