Web Components: Building Custom Elements with JavaScript
Last Updated :
27 Sep, 2023
In web development, creating reusable and encapsulated components is crucial for building scalable and maintainable applications and Web Components offer a powerful solution to this challenge by providing developers with the ability to build custom HTML elements with their functionality and styling. This article introduces Web Components and explores how they can enhance the development process and make it easier to create modular and reusable code.
Web Components are a set of web platform APIs that allow you to create custom, reusable, and encapsulated components for building web applications components can be used on any web page regardless of the framework or library being used and promote a modular and standardized approach to web development.
They consist of two main specifications:
Approach 1: Using Custom Elements with Shadow DOM
Creating custom web components using the Shadow DOM is a modern approach to building reusable and encapsulated UI elements for your web applications. Custom elements with the Shadow DOM offer a way to define your own HTML elements.
Syntax:
class MyCustomElement extends HTMLElement {
}
customElements.define('my-custom-element', MyCustomElement);
Example: This example shows the use of the above-explained approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title > Custom Element </ title >
< style >
h1 {
color: #336699;
}
</ style >
</ head >
< body style = "background-color: #C0C0C0;" >
< my-custom-element ></ my-custom-element >
< script > class MyCustomElement extends HTMLElement {
constructor() {
super();
const shadowRoot =
this.attachShadow({ mode: 'open' });
const paragraph =
document.createElement('p');
paragraph.textContent =
'**This is custom element with Shadow DOM**';
shadowRoot.appendChild(paragraph);
const style = document.createElement('style');
style.textContent = `
p {
color: red;
}
`;
shadowRoot.appendChild(style);
}
}
customElements.define('my-custom-element', MyCustomElement);
</ script >
</ body >
</ html >
|
Output:
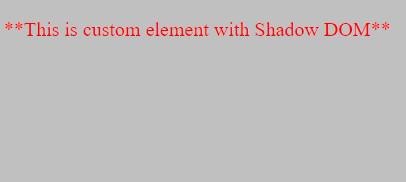
Approach 2: Using HTML Templates
The HTML templates provide an innovative way to wield logical subsetting techniques in R, offering a dynamic and interactive approach to data manipulation and integrating HTML templates you can create visually engaging reports that allow users to interact with the data selecting subsets based on their criteria.
Syntax:
class MyCustomElement extends HTMLElement {
constructor() {
super();
const template = document.getElementById('custom-template').content;
const shadowRoot = this.attachShadow({ mode: 'open' });
const clonedTemplate = document.importNode(template, true);
shadowRoot.appendChild(clonedTemplate);
}
}
Example: This example shows the use of the above-explained approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >Templates</ title >
< style >
h1 {
color: #336699;
}
</ style >
</ head >
< body style = "background-color: #FFFF00;" >
< template id = "custom-template" >
< p >
**This is custom element with HTML Templates**
</ p >
</ template >
< my-custom-element ></ my-custom-element >
< script >
class MyCustomElement extends HTMLElement {
constructor() {
super();
const template =
document.getElementById('custom-template').content;
const shadowRoot =
this.attachShadow({ mode: 'open' });
const clonedTemplate =
document.importNode(template, true);
shadowRoot.appendChild(clonedTemplate);
}
}
customElements.define('my-custom-element', MyCustomElement);
</ script >
</ body >
</ html >
|
Output :
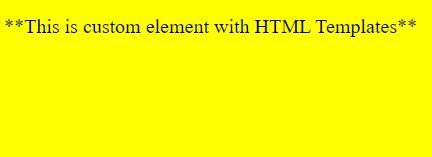
Share your thoughts in the comments
Please Login to comment...