How to Conditionally Disable the Input in VueJS ?
Last Updated :
21 Dec, 2023
In VueJS, we can conditionally disable the input-taking field. In this article, we will learn to conditionally disable the input-taking field by using two different approaches listed below:
Using :disabled Property
In this approach, we are using the :disabled property to conditionally disable the input field which is based on the boolean variable disableCheck. When the button is clicked, the event occurs and the input field is been disabled.
Syntax:
<input type="text" v-model="inputVal" :disabled="disableCheck">
Example: The below code example illustrates the use of the :disabled property to conditionally disable the input taking in VueJS.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 1</ title >
</ head >
< body >
< div id = "app" >
< input type = "text"
v-model = "inputVal"
:disabled = "disableCheck" >
< button @ click = "changeDisable" >
{{ disableCheck ? 'Enable Input' :
'Disable Input' }}
</ button >
</ div >
< script src =
</ script >
< script >
new Vue({
el: '#app',
data: {
inputVal: '',
disableCheck: false
},
methods: {
changeDisable() {
this.disableCheck =
!this.disableCheck;
}
}
});
</ script >
</ body >
</ html >
|
Output:
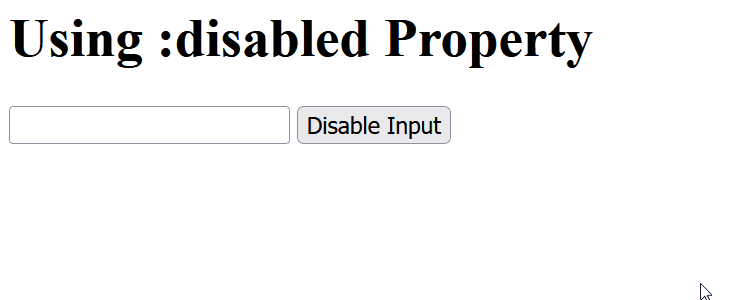
Using v-if and v-else
In this approach, we are using the v-if and v-else directives to conditionally render input fields based on the value of the condition variable. When a user clicks on the button the input field is been switched and a disabled input field is been shown.
Syntax:
<input v-if="!condition" v-model="textInput">
<input v-else :readonly="true" :style="{ 'background-color': '#eee' }" v-model="textInput">
Example: The below code example illustrates the use of a v-if and v-else to conditionally disable the input taking in VueJS.
HTML
<!DOCTYPE html>
< head >
< title >Example 2</ title >
</ head >
< body >
< div id = "app" >
< h1 > Using v-if and v-else</ h1 >
< button @ click = "disableCondition" >
Toggle Input
</ button >
< input v-if = "!condition" v-model = "textInput" >
< input v-else :readonly = "true"
:style = "{ 'background-color': '#eee' }"
v-model = "textInput" >
</ div >
< script >
new Vue({
el: '#app',
data: {
textInput: '',
condition: true
},
methods: {
disableCondition: function () {
this.condition = !this.condition;
if (this.condition) {
this.textInput = '';
}
}
}
});
</ script >
</ body >
</ html >
|
Output:
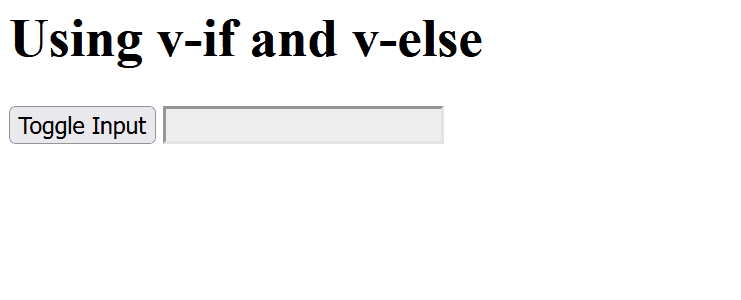
Share your thoughts in the comments
Please Login to comment...