Using ngx-webstorage with Angular 17
Last Updated :
01 May, 2024
Using ngx-webstorage with Angular 17 offers an easy approach to using the power of the browser’s Web Storage API within your Angular applications. This article will guide you through the process of integrating ngx-webstorage, a dedicated library for Angular that simplifies client-side data persistence, enabling you to store and retrieve data effortlessly using localStorage and sessionStorage.
What is ngx-webstorage?
NGX Storage is an Angular library that provides a simple and efficient way to store data in the browser’s local storage or session storage. It allows you to easily save and retrieve data, such as user preferences, application state, or any other relevant information, across page reloads or browser sessions. NGX Storage offers a simple API for storing and retrieving data, making it easy to integrate into Angular applications and manage client-side data storage requirements.
Benefits of ngx-webstorage
- Provides an easy-to-use API for interacting with localStorage and sessionStorage in Angular applications.
- Offers type-safe storage with support for storing complex data structures like objects and arrays.
- Automatically handles serialization and deserialization of data, reducing boilerplate code.
- Supports observables for reactive programming and real-time updates when storage changes.
- Provides decorators for seamless integration with Angular services and components.
- Offers synchronization mechanisms to keep data consistent across multiple browser tabs or windows.
- Maintains a lightweight footprint, ensuring efficient performance in web applications.
Below are the approaches to use ngx-webstorage with Angular 17
Steps to implement ngx-webstorage
To get started with ngx-webstorage in an Angular, follow these steps:
Step 1: Create a application and Install Required Modules
ng new my-app
cd my-app
npm install ngx-webstorage
Folder Structure:
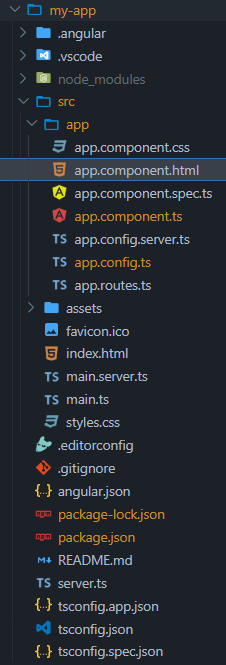
Project Structure
The updated dependencies in package.json file:
"dependencies": {
"@angular/animations": "^17.2.0",
"@angular/common": "^17.2.0",
"@angular/compiler": "^17.2.0",
"@angular/core": "^17.2.0",
"@angular/forms": "^17.2.0",
"@angular/platform-browser": "^17.2.0",
"@angular/platform-browser-dynamic": "^17.2.0",
"@angular/platform-server": "^17.2.0",
"@angular/router": "^17.2.0",
"@angular/ssr": "^17.2.3",
"express": "^4.18.2",
"ngx-webstorage": "^13.0.1",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Using Injectable Service
This approach involves creating an injectable service that wraps the Web Storage API. The service provides convenient methods to store, retrieve, and remove data from localStorage or sessionStorage.
Example:
JavaScript
//app.modules.ts
import { NgxWebstorageModule } from 'ngx-webstorage';
@NgModule({
imports: [
// ...
NgxWebstorageModule.forRoot()
]
})
export class AppModule { }
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { LocalStorageService } from 'ngx-webstorage';
@Component({
selector: 'app-root',
template: `
<input type="text" [(ngModel)]="value" />
<button (click)="storeValue()">Store Value</button>
<button (click)="retrieveValue()">Retrieve Value</button>
`
})
export class AppComponent {
value: string = '';
constructor(private localStorage: LocalStorageService) { }
storeValue() {
this.localStorage.store('key', this.value);
}
retrieveValue() {
this.value = this.localStorage.retrieve('key');
}
}
Output:
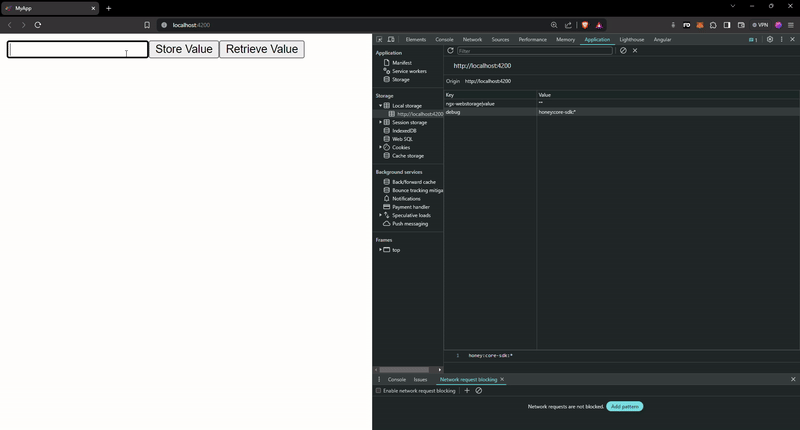
approach 1
Using Custom Decorators
ngx-webstorage provides decorators that can be used to automatically persist and retrieve data from Web Storage. These decorators can be applied to class properties, allowing developers to manage state without writing additional code.
Example:
JavaScript
//app.modules.ts
import { NgxWebstorageModule } from 'ngx-webstorage';
@NgModule({
imports: [
// ...
NgxWebstorageModule.forRoot()
]
})
export class AppModule { }
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { LocalStorage } from 'ngx-webstorage';
@Component({
selector: 'app-root',
template: `
<input type="text" [(ngModel)]="value" />
`
})
export class AppComponent {
@LocalStorage()
value: string = '';
}
Output:
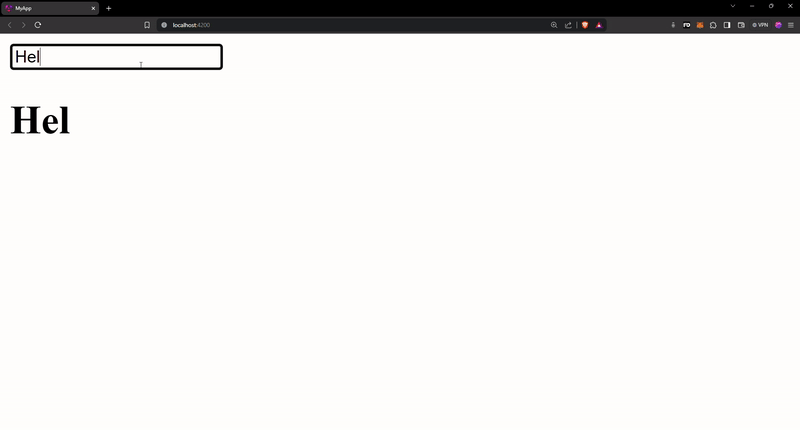
appraoch 2
Conclusion
These examples demonstrate two different approaches provided by ngx-webstorage for working with the Web Storage API in Angular 17 applications. Choose the approach that best suits your application’s requirements and coding style.
So to conclude, Ngx-webstorage streamlines working with web storage in Angular applications by offering a single interface for both local and session storage, improving user experience with features like type safety and automatic data synchronization. It also provides more flexibility by allowing easy switching between storage types and even potential creation of custom storage solutions.
Share your thoughts in the comments
Please Login to comment...