When to use a Class Component over a Function Component in ReactJS ?
Last Updated :
31 May, 2023
Introduction: Components are the building blocks of creating user interfaces in React. They allow us to develop re-usable and independent bits of inter-linked components which together form the whole web interface. React has 2 different types of components:
Syntax:
Class Component – The components created using the ES2015 class template are known as class components.
class FirstComponent extends React.Component {
render() {
return <p>This is a class component!</p>;
}
}
Function Component – The components created using the JavaScript function syntax are functional components.
function anotherComponent(props) {
return <p>This is a function component!</p>;
}
Now that we know what components are, let’s try to understand when should we use class or function components. We need to understand the concept of the state of the component first. You can refer to this article for a better understanding of React state.
In the case of class components, when the state of the component is changed then the render method is called. Whereas, function components render the interface whenever the props are changed.
Although we should prefer using function components most times as React also allows using state with function components in React version 16.8 and later by using the useState hook, which is also recommended by Meta (Facebook App) itself. But for the older react versions, we can use class components where we have a dependency on the state of the components.
Class Components vs Function Components:
Class Components
|
Function Components
|
Class components need to extend the component from “React.Component” and create a render function that returns the required element. |
Functional components are like normal functions which take “props” as the argument and return the required element. |
They are also known as stateful components. |
They are also known as stateless components. |
They implement logic and the state of the component. |
They accept some kind of data and display it in the UI. |
Lifecycle methods can be used inside them. |
Lifecycle methods cannot be used inside them. |
Format of Hooks inside class components:
constructor(props) {
super(props);
this.state = { color: ‘ ‘ }
}
|
Format of Hooks inside function components:
const [color,SetColor]= React.useState('')
|
It needs to store state therefore constructors are used. |
Constructors are not used in it. |
It has to have a “render()” method inside that. |
It does not require a render method. |
Example 1: This is a basic example that’ll illustrate Class Component and functional component:
Class Component:
Javascript
import React from "react" ;
class ClassComponent extends React.Component {
render() {
return <h1>It's a class component!</h1>;
}
}
export default ClassComponent;
|
Output:
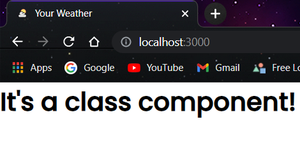
Example of Class Component
Function Component:
Javascript
import React from "react" ;
const FunctionComponent = () => {
return <h1>It's a function component!</h1>;
};
export default FunctionComponent;
|
Output:
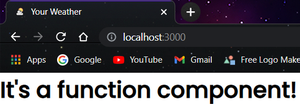
Example of Function Component
Example 2: This is another example of Class Component and functional component:
Class Component:
Javascript
import React from "react" ;
class Something extends React.Component {
render() {
return <h1>A Computer Science
Portal For Geeks</h1>;
}
}
class ClassComponent extends React.Component {
render() {
return <Something />;
}
}
export default ClassComponent;
|
Output:
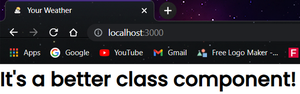
Example of Class Component
Function Component:
Javascript
import React from "react" ;
const Something = () => {
return <h1>It's a better function
component!</h1>;
};
const FunctionComponent = () => {
return <Something />;
};
export default FunctionComponent;
|
Output:
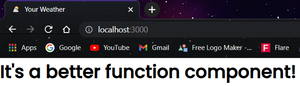
Example of Function Component
Share your thoughts in the comments
Please Login to comment...