Understanding Templates Variable in Angular
Last Updated :
19 Mar, 2024
Template variables in Angular allow us to use the data from one part of the template to another part of the same template. There are various use cases where these template variables come in handy. For example, responding to the user input or responding to the form submission.
In this article, we’ll explore what are template variables and how to use them.
What are Template Variables?
As we’ve seen in the description itself, by using template variables we can use data from one part of the template to another part of the same template. Now let’s see the places in the template where template variables can be used.
- DOM Elements within a Template: using the template variables we can create a reference to a specific DOM element within the template. This allows us to access that particular DOM element or its value programmatically.
- Directives or Components: By using the template variables we can also refer to the directives or components. For example, using a template variable we can refer to a form component to collect its data.
- TemplateRefs from an ng-template: Suppose we’re using Angular’s <ng-template> then by using template variables we can refer to the template itself. It is handy when working with structure directives.
Syntax for Declaring Template Variables
In the template, we use hash symbol (#) to declare the template variable. In the below syntax we’ve declared a template variable called “templateVarName” for the input element. Then the button element is referring to this variable and passes its value to the handleSubmit() method.
<input #templateVarName />
<button type="button" (click)="handleSubmit(templateVarName.value)">Submit</button>
Scope of Template Variables
Just like any other local variable, these template variables are also locally scoped to the templates. In other words, we cannot access the value of the template variable outside the template. Therefore it is not possible to use the value of a template variables in the component code directly. To use the value of the template variable in the component code we pass it as an argument to the function from template itself.
DOM Element Template Variable
In this example, we’ll create a template variable called “ageField” for the input element. Then we’ll create a button element that will refer this template variable to display the message.
app.component.html
HTML
<h2>Are you Eligible for Voting?</h2>
<input #ageField type="number" name="age" id="age" placeholder="Enter the age"/>
<button type="button" (click)="checkAge(ageField.value)">Check</button>
<p>{{message}}</p>
JavaScript
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
message: string = 'Waiting for result...';
checkAge(arg: string) {
const age: number = Number.parseInt(arg);
if (age >= 18) {
this.message = 'Yes, you can vote.';
} else {
this.message = 'No, you can not vote.';
}
}
}
JavaScript
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule { }
Output:
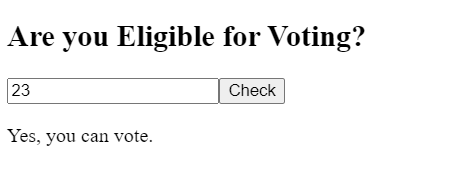
output for Example 1
Let’s consider an example where we’ll create a template variable called “userForm” referring to the Angular’s NgForm directive instance. We’ll check the validity of the form and then display a message accordingly.
HTML
<!-- app.component.html !-->
<h2>GeeksForGeeks</h2>
<form #userForm="ngForm" (ngSubmit)="onSubmit(userForm)">
<label for="name">Name</label>
<input type="text" id="name" class="form-control" name="name" ngModel required />
<label for="age"Age></label>
<input type="number" name="age" id="age" ngModel required />
<button type="submit">Submit</button>
</form>
<div [hidden]="!userForm.form.valid">
<p>My name is {{name}} and I'm {{age}} years old.</p>
</div>
JavaScript
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
name: string = '';
age: number = 0;
onSubmit(form: any) {
this.name = form.value.name;
this.age = form.value.age;
}
}
JavaScript
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
Output:
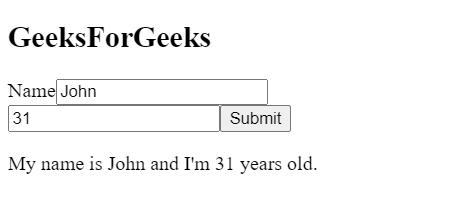
Output for Example 2
Share your thoughts in the comments
Please Login to comment...