Understanding React State and Data Flow
Last Updated :
14 Jan, 2024
React, a very popular JavaScript library used for building user interfaces has the concept of components and they can manage the states efficiently. ReactJs is based on the concept of uni-directional data transfer. In this article, we will understand React State and Data Flow across the application.
What is React State?
A state in React is a special object that represents the current state of a component. Unlike props, which are passed from parent to child components, state is internal and can be changed by the component itself. State is used to manage dynamic data and to trigger re-rendering of components when the state changes. Each component can have its own state, making it a self-contained unit with its own data.
Setting State:
State can be initialised using the useState hook in functional components or via the this.state and this.setState methods in class components.
Example: Creating a counter button to count the number of times the button is clicked.
Javascript
import React, { useState } from "react" ;
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
|
Output:
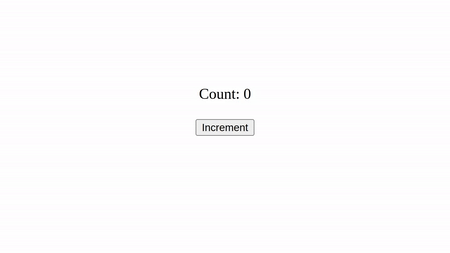
Output
State in Class Components: In class components, state is initialized in the constructor, and setState is used to update it:
Example: Creating a counter button to count the number of times the button is clicked.
Javascript
import React, { Component } from 'react' ;
class Counter extends Component {
constructor(props) {
super (props);
this .state = {
count: 0,
};
}
render() {
return (
<div>
<p>Count: { this .state.count}</p>
<button onClick={() => this .setState({ count: this .state.count + 1 })}>
Increment
</button>
</div>
);
}
}
export default Counter;
|
Output:
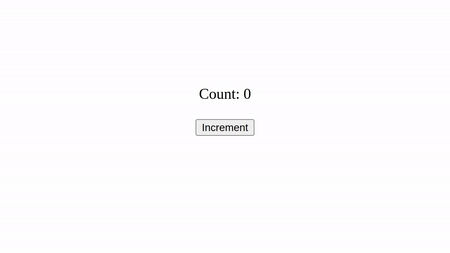
Output
Data Flow in React JS:
React follows a unidirectional data flow, meaning that data flows in a single direction through the components. This helps in maintaining a predictable state and making it easier to understand how changes affect the application. In React we can pass props from Parent component to child and with the help of callback can also pass props from child to parent.
1. Parent to Child:
Data is passed from parent components to child components through props. The parent component owns the state, and it passes down relevant data to its child components as props.
Props (short for “properties”) in React are a mechanism for passing data from a parent component to its child component as a set of read-only attributes.
For example: Sending the data from parent to child as “Hello, this is data from the parent to child”.
Javascript
import React, { useState } from 'react' ;
import ChildComponent from './ChildComponent' ;
function ParentComponent() {
const [data, setData] = useState( '"Hello, this is the data from the parent to child".!' );
return <ChildComponent data={data} />;
}
export default ParentComponent;
|
Javascript
import React from 'react' ;
function ChildComponent(props) {
return <p>{props.data}</p>;
}
export default ChildComponent;
|
Output:
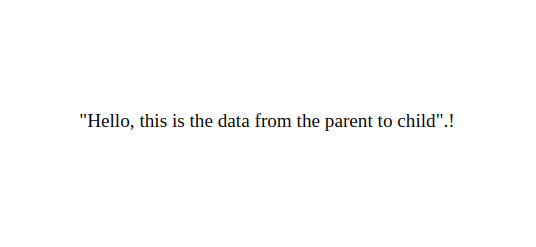
2. Child to Parent:
To communicate from child to parent, the parent can pass down a callback function as a prop to the child. The child can then invoke this function to send data back to the parent.
Example: Sending the data from child to parent as “Hello, this is data from the child to parent”.
Javascript
import React, { useState } from "react" ;
import ChildComponent from "./ChildComponent" ;
function ParentComponent() {
const [dataFromChild, setDataFromChild] = useState( "" );
const handleDataFromChild = (data) => {
setDataFromChild(data);
};
return (
<div>
<p>Data from child: {dataFromChild}</p>
<ChildComponent sendDataToParent={handleDataFromChild} />
</div>
);
}
export default ParentComponent;
|
Javascript
import React from "react" ;
function ChildComponent(props) {
const sendData = () => {
props.sendDataToParent( "Hello from child!" );
};
return <button onClick={sendData}>Send Data to Parent</button>;
}
export default ChildComponent;
|
Output:
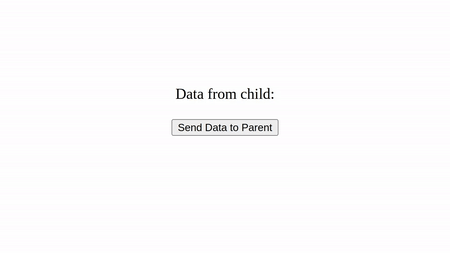
Output
Share your thoughts in the comments
Please Login to comment...