Template Method | JavaScript Design Patterns
Last Updated :
17 Jan, 2024
Template Method is a behavioral design pattern that defines the skeleton of an algorithm in a base class while allowing subclasses to implement specific steps of the algorithm without changing its structure. It promotes code reusability and provides a way to enforce a consistent algorithm structure across different subclasses.
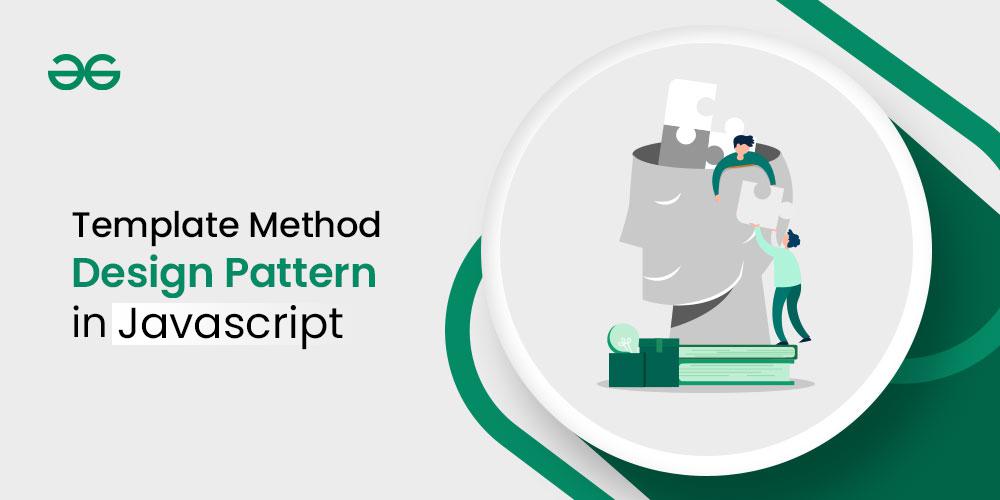
Important Topics for the Template Method in JavaScript Design Patterns
Use Cases of the Template Method in JavaScript Design Patterns
The Template Method design pattern in JavaScript can be useful in various scenarios where you want to create a common algorithm structure while allowing flexibility for specific implementations. Here are some common uses of the Template Method pattern in JavaScript:
- UI Frameworks:
- When building user interface frameworks or libraries, you can use the Template Method pattern to define a common structure for components (e.g., buttons, dialogs) while allowing developers to customize the rendering, event handling, or other behavior of those components in subclasses.
- Data Processing:
- In data processing or data transformation tasks, you can define a template method that outlines the overall process (e.g., loading data, transforming it, and saving it) while letting subclasses implement the specific data transformation logic.
- Game Development:
- In game development, you can use the Template Method pattern to define common game loops, character behaviors, or level designs while allowing different games or characters to implement their unique features within the established framework.
- HTTP Request Handling:
- When dealing with HTTP request handling, you can create a template method for request processing that includes common steps like authentication, request parsing, and response generation, while letting specific routes or endpoints define their custom logic.
- Algorithmic Processing:
- For algorithmic tasks, you can use the Template Method pattern to define the overall algorithm structure and let subclasses provide their implementations for specific algorithmic steps.
- Testing Frameworks:
- In the development of testing frameworks, you can use the Template Method pattern to define the overall testing process (e.g., setup, execution, teardown) while allowing custom test cases or test suites to specify their unique test logic.
- Document Generation:
- When generating documents or reports, you can define a template method that outlines the structure and content of the document, such as headers, footers, and sections, while allowing subclasses to provide the specific content for each section.
- Middleware and Filters:
- In web applications, you can use the Template Method pattern to define middleware or filter chains, where each middleware can perform specific tasks before or after the main request handling process, such as authentication, logging, or security checks.
- State Machines:
- When implementing state machines, you can use the Template Method pattern to define a common structure for state transitions, error handling, and actions to perform on state changes, with subclasses specifying the details for each state.
- Code Generators:
- In code generation tools, you can use the Template Method pattern to define code generation processes, such as parsing input, generating code, and handling dependencies, while allowing customization for different programming languages or target platforms.
Example for Template Method in JavaScript Design Patterns:
We can take the example of a sandwich to demonstrate the Template method in Javascript Design Patterns. Imagine you have a recipe for a sandwich, but some parts of it can vary. The Template Method is like the overall structure of making the sandwich, and the specific ingredients and steps can be customized.
- Bread (Fixed Step): You always start with two slices of bread, which is a fixed step in making any sandwich.These steps are fixed and remain unchanged
- Custom Filling (Customizable Step): Next, you have the freedom to choose your filling. You can make a ham sandwich, a turkey sandwich, or a vegetarian sandwich. This part is like a customizable step because it varies depending on your choice.These customizable parts can vary based on specific needs.
- More Fixed Steps (Fixed Steps): After putting in your filling, you might add some lettuce, tomatoes, and mayonnaise. These are fixed steps that are the same for all sandwiches, no matter the filling.
- Top Bread (Fixed Step): Finally, you finish with another slice of bread on top, another fixed step to complete the sandwich.
So, in JavaScript, the Template Method is like a blueprint for making sandwiches. It defines the fixed steps (like using bread at the beginning and end) and leaves some parts (like the filling) open for customization. Different sandwiches (subclasses) can follow the same overall structure while allowing for variations in the filling.
Diagrammatic explanation of the Above Example
Here’s a simple diagram to illustrate the key components and interactions:
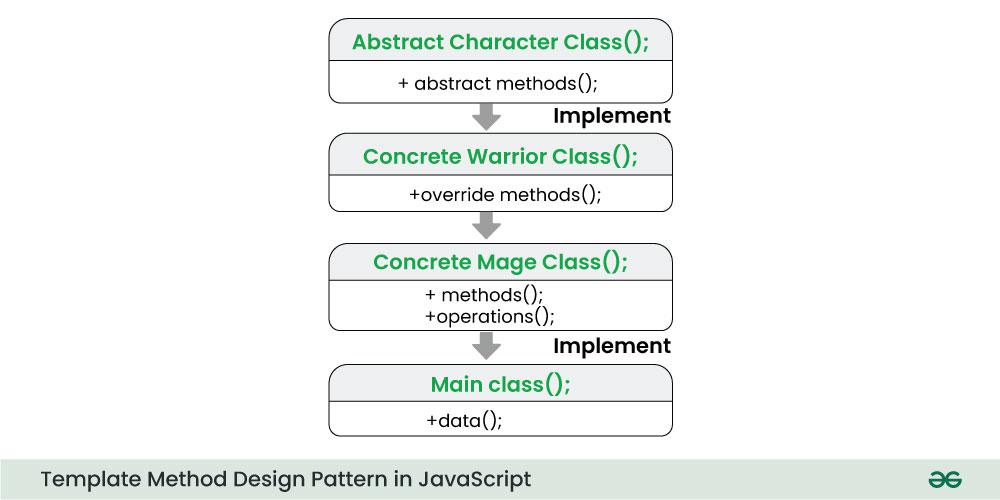
Explanation:
- AbstractClass: This is the abstract base class that defines the template method (templateMethod) and the individual steps cutBread(), addFilling(),addCondiments(),wrap() . The template method is responsible for orchestrating the algorithm’s flow.
- ConcreteClass: This is a concrete subclass that extends AbstractClass. It provides specific implementations for some or all of the steps VeggieSandwich,TurkeySandwich while inheriting the structure of the template method from the abstract class.
- Instantiate and use ConcreteClass: In your JavaScript code, you create an instance of ConcreteClass and call the templateMethod. The template method follows the predefined algorithm structure and calls the concrete implementations provided by ConcreteClass for the steps. This allows you to reuse the algorithm structure while customizing specific behaviors as needed in derived classes.
Code Implementation of the above Problem:
The Template Method pattern promotes code reuse and separation of concerns by defining a common algorithm structure in the abstract class while allowing flexibility for specific implementations in concrete subclasses.
Javascript
class Sandwich {
make() {
this .cutBread();
this .addFilling();
this .addCondiments();
this .wrap();
}
cutBread() {
console.log( 'Cutting the bread.' );
}
addCondiments() {
console.log( 'Adding condiments (mayonnaise, mustard, etc.).' );
}
wrap() {
console.log( 'Wrapping the sandwich.' );
}
addFilling() {
throw new Error( 'Subclasses must implement the addFilling method.' );
}
}
class VeggieSandwich extends Sandwich {
addFilling() {
console.log( 'Adding veggies (lettuce, tomato, cucumber, etc.).' );
}
}
class TurkeySandwich extends Sandwich {
addFilling() {
console.log( 'Adding turkey slices.' );
}
}
const veggieSandwich = new VeggieSandwich();
const turkeySandwich = new TurkeySandwich();
console.log( 'Making a Veggie Sandwich:' );
veggieSandwich.make();
console.log( '\nMaking a Turkey Sandwich:' );
turkeySandwich.make();
|
Output
Making a Veggie Sandwich:
Cutting the bread.
Adding veggies (lettuce, tomato, cucumber, etc.).
Adding condiments (mayonnaise, mustard, etc.).
Wrapping the sandwich.
Making a Turkey Sandwich:
Cutting ...
In this example,
Sandwich
is the base class that defines the template method make()
, which includes fixed steps like cutting the bread, adding condiments, and wrapping the sandwich. The addFilling()
method is marked as an abstract method, which means it must be implemented by subclasses.
VeggieSandwich
and TurkeySandwich
are subclasses that extend the Sandwich
class. They implement the addFilling()
method to specify the filling for each type of sandwich.
- When you create instances of these sandwich subclasses and call the
make()
method, the template method ensures that the fixed steps are followed (cutting the bread, adding condiments, wrapping), and the custom filling is added as specified in the subclasses.
Note: This allows you to create a common structure for algorithms while allowing flexibility for specific implementations in derived classes.
Advantages of the Template Method in JavaScript Design Patterns
The Template Method design pattern offers several advantages in software development:
- Code Reusability: The primary benefit of the Template Method pattern is code reuse. By defining a common template for an algorithm in an abstract class, you can reuse the same algorithm structure in multiple concrete subclasses. This reduces code duplication and promotes a more maintainable codebase.
- Consistency: The pattern enforces a consistent structure for a particular algorithm across different parts of an application or within different subclasses. This consistency can lead to a more predictable and understandable codebase.
- Separation of Concerns: The pattern helps separate the overall algorithm structure from the specific implementation details. This separation allows developers to focus on individual steps or components of the algorithm independently, making it easier to maintain and update the code.
- Reduced Errors: Since the algorithm’s structure is defined in a single place (the abstract class), there is a reduced chance of introducing errors when implementing the same algorithm in different parts of the codebase.
- Easy Maintenance: When changes are required in the algorithm’s structure or behavior, you only need to make modifications in one place—the abstract class. This simplifies maintenance and ensures that the changes are propagated consistently to all subclasses.
- Improved Collaboration: The Template Method pattern can enhance collaboration among team members by providing a clear structure and guidelines for implementing specific parts of an algorithm. It makes it easier for team members to work on different aspects of the code independently.
- Enhanced Readability: Code that follows the Template Method pattern tends to be more readable and understandable.
- Testing: It facilitates testing because you can test the abstract algorithm and its individual steps independently. This makes it easier to write unit tests for the algorithm’s components.
Disadvantages of the Template Method in JavaScript Design Patterns
While the Template Method design pattern offers several advantages, it also has some potential disadvantages and considerations:
- Rigidity: The pattern can introduce rigidity into the codebase because the overall algorithm structure is defined in the abstract class. Any significant changes to this structure may require modifications in multiple concrete subclasses, which can be time-consuming and error-prone.
- Complexity: In some cases, using the Template Method pattern can lead to increased complexity, especially when dealing with a large number of concrete subclasses or when the algorithm has many variations. Understanding and maintaining the relationships between the abstract class and its subclasses can become challenging.
- Limited Flexibility: While the Template Method pattern allows for customization of specific steps, it may not handle scenarios where the algorithm’s structure needs to change significantly between subclasses. In such cases, it may be more appropriate to use other design patterns or a more flexible approach.
- Inheritance Issues: The pattern relies on class inheritance, which can lead to issues such as the diamond problem (when a class inherits from two classes that have a common ancestor). Careful consideration and management of class hierarchies are necessary to avoid inheritance-related problems.
- Tight Coupling: Concrete subclasses are tightly coupled to the abstract class, as they rely on its structure and may override its methods. This can make it difficult to change the abstract class without affecting the concrete subclasses.
- Limited Support for Runtime Changes: The Template Method pattern is primarily suited for defining the algorithm structure at compile time. If you need to change the algorithm dynamically at runtime, it may not be the most suitable pattern, and you may consider using other approaches, such as strategy patterns or dependency injection.
- Overhead: In some cases, creating a hierarchy of abstract classes and concrete subclasses to implement the Template Method pattern can introduce additional complexity and overhead. This can be especially true for small or simple algorithms where the benefits of the pattern may not outweigh the added complexity.
- Maintenance Challenges: While the pattern can simplify maintenance in some cases, it may complicate maintenance in others, especially if the codebase has evolved over time with many concrete subclasses and variations of the template method.
- Not Always the Best Fit: The Template Method pattern is not always the best fit for every situation. It’s essential to evaluate whether it aligns with the specific requirements and design goals of a given project. In some cases, alternative patterns or approaches may be more suitable.
Share your thoughts in the comments
Please Login to comment...