Adapter Method | JavaScript Design Patterns
Last Updated :
31 Oct, 2023
Adapter Pattern in JavaScript is a structural design pattern that allows you to make one interface or object work with another that has a different interface. It acts as a bridge, enabling the compatibility of two systems that would not naturally work together.
Important Topics for the Adapter Method in JavaScript Design Patterns
Use Cases of the Adapter Method in JavaScript Design Patterns
The Adapter Method in JavaScript is a useful design pattern that can be applied in various scenarios such as:
- Integrating Legacy Code: When working with older systems or libraries that have incompatible interfaces, adapters can be used to make them compatible with modern systems or libraries, allowing for the integration of legacy code into new projects.
- Working with Third-Party APIs: Many times, you need to interact with external APIs that have different data formats or methods. Adapters can be used to translate the responses from these APIs into a format that your application can work with.
- Database Connectivity: Adapters can be used to standardize database connections. For example, you can create an adapter to work with different database management systems (e.g., MySQL, PostgreSQL, MongoDB) using a common interface.
- File Format Conversion: Adapters can be used to convert data or file formats. For example, you can create adapters to read and write data in different formats like XML, JSON, or CSV.
- Data Transformation: Adapters are helpful for data transformation tasks. You can adapt data from one format to another, such as converting between units of measurement, temperature scales, or date formats.
- Version Upgrades: When upgrading libraries or components that have changed their interfaces, adapters can be used to maintain compatibility with existing code until it can be updated.
Adapter Method example in JavaScript Design Patterns
Problem Statement:
Think about your electronic devices, like charging cables. You have an old charger (let’s call it “Old Plug”) with a big, round shape, and your new device (let’s call it “New Jack”) has a small, rectangular port.Now, you buy an adapter (Adapter in this case is a physical object, but it helps explain the concept). This adapter has a big round end that fits your old charger and a small rectangular end that fits your new device.
In this example:
- Your old charger represents one system with its own way of doing things.
- Your new device represents another system with its own way of doing things.
- The adapter (the physical object) acts as the bridge between them, making the old charger work with the new device.
In JavaScript, the Adapter Pattern works similarly. It allows one piece of code to work with another piece of code, even if they have different interfaces, just like your adapter makes your old charger work with your new device.
Below is the implementation of the above example:
Javascript
class OldSystem {
specificMethod() {
console.log( 'Old system specific method' );
}
}
class TargetInterface {
requiredMethod() {}
}
class Adapter extends TargetInterface {
constructor(oldSystem) {
super ();
this .oldSystem = oldSystem;
}
requiredMethod() {
this .oldSystem.specificMethod();
}
}
const oldSystem = new OldSystem();
const adapter = new Adapter(oldSystem);
adapter.requiredMethod();
|
Output
Old system specific method
Below is the explanation of the above code:
Adapter class allows you to use the OldSystem with the interface expected by the client code TargetInterface, and calling adapter.requiredMethod() correctly invokes the specificMethod of the OldSystem. This demonstrates how the Adapter pattern bridges the gap between two incompatible interfaces.
Diagrammatic Explanation of the Adapter Method in Javascript
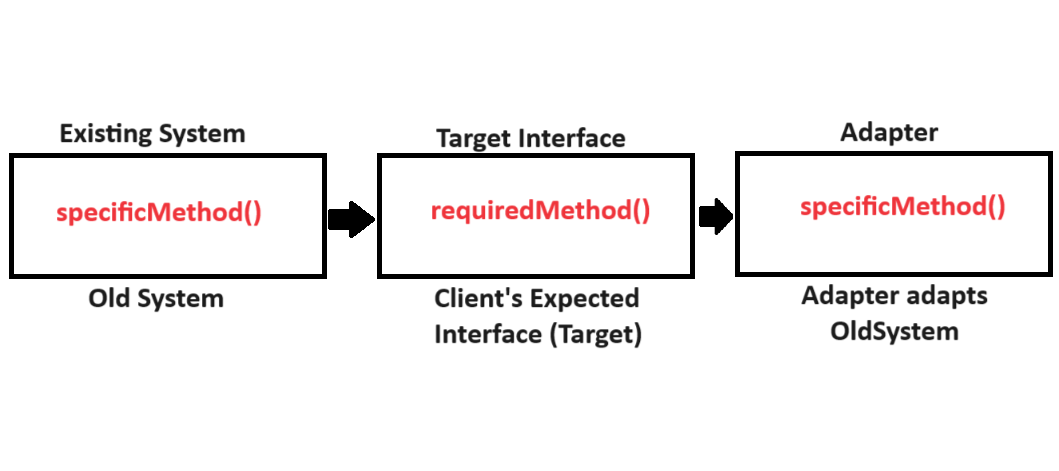
Diagram of the Adapter Method in JavaScript
In this diagram:
- “Existing System” represents the OldSystem class with its specificMethod().
- “Target Interface” represents the interface that the client code expects with its requiredMethod().
- “Adapter” is the class that adapts the OldSystem to the Target Interface and implements requiredMethod() by invoking specificMethod() from OldSystem.
The flow of the method call is from the client code through the Adapter to the OldSystem, allowing the OldSystem to work seamlessly with the client’s expected interface.
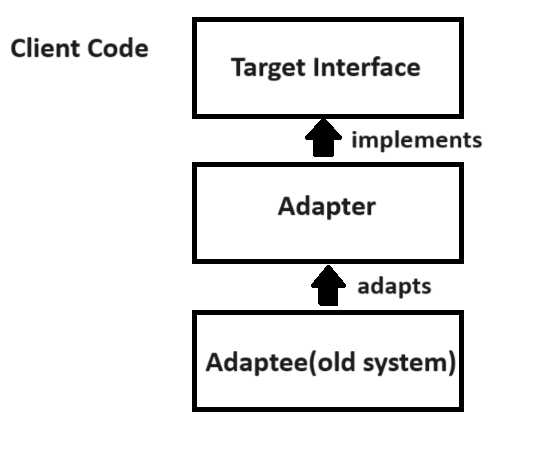
Diagram explaining Adapter Method in Javascript
In this diagram:
- “Client Code” is the code that expects to use the Target Interface.
- “Target Interface” is the interface that the client code expects to interact with.
- “Adapter” is the class that adapts the Adaptee (Old System) to the Target Interface.
- “Adaptee (Old System)” is the existing class with an incompatible interface.
The relationships and interactions:
- The “Target Interface” is implemented by the “Adapter.”
- The “Adapter” adapts the “Adaptee” by encapsulating its methods and providing a compatible interface to the client code.
- The client code interacts with the “Adapter,” which, in turn, interacts with the “Adaptee” to make the two interfaces compatible.
Advantages of the Adapter Method in JavaScript Design Patterns
Here are the advantages of using the Adapter Method:
- Compatibility: The Adapter Method allows you to make two incompatible interfaces work together, promoting interoperability between different parts of your application or external libraries.
- Code Reusability: It enables the integration of existing components or systems into new contexts without modifying their core code, enhancing code reusability and reducing redundancy.
- Maintainability: By encapsulating the adaptation logic within an Adapter class, it simplifies maintenance. You can make changes to the Adapter without affecting the client code or the Adaptee, making the codebase more maintainable.
- Flexibility: The Adapter Method provides flexibility in software design by allowing you to extend or evolve systems without breaking existing functionality. New systems can be integrated with older ones, and vice versa, with ease.
- Testing Simplification: The client code interacts with a consistent and expected interface, making testing straightforward. You can test the client code without needing to deal with the complexities of the Adaptee’s interface.
- Encapsulation: It promotes encapsulation by isolating the adaptation logic within the Adapter class. This separation of concerns leads to cleaner, more modular, and maintainable code.
- Enhanced Security: Adapters can provide security and access control by controlling how the Adaptee’s methods are accessed and ensuring that only authorized actions are performed.
- Promotion of Good Design Principles: The Adapter Method aligns with good design principles, such as the Single Responsibility Principle (SRP) and the Open/Closed Principle (OCP), which encourage clean, extensible, and maintainable code.
- Smooth Integration: It allows you to integrate external or third-party libraries with your codebase, even if they have different interfaces, enabling you to leverage the functionality of these libraries without extensive modifications.
- Consistent Interfaces: Clients interacting with an Adapter only need to know the expected interface, which simplifies the design and usage of complex systems.
- Cost-Effective: The Adapter Method can save development time and cost by reducing the need for extensive refactoring or rewriting of existing code to make it compatible with new requirements.
Disadvantages of the Adapter Method in JavaScript Design Patterns
Here are the disadvantages of using the Adapter Method:
- Complexity: Using adapters can introduce additional complexity to your codebase, especially when dealing with multiple adapters and complex adaptions. This added complexity can make the code harder to understand and maintain.
- Performance Overhead: Adapters may introduce some performance overhead because they involve additional method calls and translation between interfaces. In performance-critical applications, this overhead could be a concern.
- Overhead for Simple Cases: In simple scenarios where the interfaces are almost identical, using an adapter might seem like an unnecessary abstraction and add unnecessary code.
- Potential for Bugs: The introduction of adapters can lead to potential bugs or errors if the adaptation logic is not correctly implemented or updated when changes occur in the Adaptee.
- Maintenance Challenges: Adapters need to be maintained as the Adaptee or the expected interface changes. If not properly maintained, they can become a source of issues.
- Abstraction Leaks: In some cases, the details of the Adaptee may leak through the adapter, making it less effective in isolating the client code from the complexities of the underlying system.
- Overhead in Memory Usage: Adapters can consume additional memory, particularly in scenarios with a large number of objects, which may not be efficient in memory-constrained environments.
- Potential for Unintended Consequences: Adapting the behavior of one system to match another may have unintended consequences, especially if the behavior of the two systems differs significantly.
- Design Overhead: Implementing and maintaining adapters requires additional design work, which can add overhead to the development process.
- Learning Curve: Developers new to the codebase may need to understand the purpose and implementation of adapters, adding to the learning curve.
Conclusion
These are just a few examples of how the Adapter Method can be employed in JavaScript to address compatibility and integration challenges in a wide range of scenarios. It’s a versatile design pattern that promotes flexibility, reusability, and maintainability in your codebase.
Share your thoughts in the comments
Please Login to comment...