Visitor Pattern | JavaScript Design Patterns
Last Updated :
01 Nov, 2023
The visitor pattern is a behavioral design pattern that allows you to add new behaviors or operations to a set of objects without modifying their structure. It achieves this by separating the algorithm from the objects on which it operates.
Important Topics for the Visitor Pattern in JavaScript Design Patterns
Characteristics of the Visitor Pattern in JavaScript Design Patterns
Here are the main features and characteristics of the Visitor pattern:
- Separation of Concerns: The Visitor pattern promotes the separation of concerns by isolating the operations that can be performed on a data structure or object hierarchy from the structure itself. This separation makes it easier to add new operations or behaviors without modifying existing classes.
- Open-Closed Principle: The pattern follows the Open-Closed Principle, which states that a class should be open for extension but closed for modification. With the Visitor pattern, you can introduce new behaviors (visitors) without changing the existing elements being visited.
- Double Dispatch: The Visitor pattern uses a technique called double dispatch. In dynamic languages like JavaScript, this means that the specific method to be executed depends on both the type of the element being visited and the type of the visitor. This enables the selection of the appropriate behavior at runtime.
- Traversal of Complex Structures: It’s particularly useful when working with complex data structures, object hierarchies, or composite structures (like trees or graphs). Visitors can traverse these structures and perform operations on their elements without exposing the details of the structure.
- Extensibility: You can add new visitors to introduce new operations without modifying the existing elements. This makes the pattern suitable for scenarios where you expect the set of operations to change or grow over time.
- Maintainability: The Visitor pattern can enhance code maintainability by keeping related operations encapsulated within their own visitor classes, making it easier to understand and maintain the codebase.
Explanation of the Visitor Pattern in Javascript
In JavaScript, the Visitor pattern is a design pattern that allows you to add new behaviors or operations to objects of different types without modifying their individual classes. It separates the algorithm from the objects being operated upon. This is particularly useful when dealing with complex data structures or object hierarchies.
Here’s an explanation of the Visitor pattern in JavaScript:
Key Components:
- Visitor Interface: You define an interface that lists the methods representing the operations you want to perform on various object types. Each method typically corresponds to a different object type or class.
- Concrete Visitors: Concrete visitor classes implement the Visitor interface and provide the actual implementation of the operations for each object type. These classes encapsulate the behavior you want to add.
- Visitable Elements: Objects or elements that you want to apply the visitor pattern to should implement an accept method. This method takes a visitor object as an argument and calls the appropriate visitor method.
Example of the Visitor Pattern for Shapes in JavaScript
Let’s use an example of different geometric shapes to illustrate the Visitor pattern in JavaScript:
Javascript
class ShapeVisitor {
visitCircle(circle) {}
visitSquare(square) {}
visitTriangle(triangle) {}
}
class AreaCalculator extends ShapeVisitor {
visitCircle(circle) {
}
visitSquare(square) {
}
visitTriangle(triangle) {
}
}
class PerimeterCalculator extends ShapeVisitor {
visitCircle(circle) {
}
visitSquare(square) {
}
visitTriangle(triangle) {
}
}
class Circle {
accept(visitor) {
visitor.visitCircle( this );
}
}
class Square {
accept(visitor) {
visitor.visitSquare( this );
}
}
class Triangle {
accept(visitor) {
visitor.visitTriangle( this );
}
}
|
Now, you can use the Visitor pattern to calculate areas and perimeters of shapes without modifying their individual classes:
const circle = new Circle();
const square = new Square();
const triangle = new Triangle();
const areaCalculator = new AreaCalculator();
const perimeterCalculator = new PerimeterCalculator();
const circleArea = circle.accept(areaCalculator);
const squarePerimeter = square.accept(perimeterCalculator);
const triangleArea = triangle.accept(areaCalculator);
Note: In above example, the Visitor pattern allows you to perform different operations (calculating area and perimeter) on different types of shapes without changing the shape classes themselves. It promotes separation of concerns and extensibility in your code.
Code implementing Visitor Pattern in Javascript
Here’s a JavaScript code implementation of the Visitor pattern for shapes along with sample output:
Javascript
class ShapeVisitor {
visitCircle(circle) {}
visitSquare(square) {}
visitTriangle(triangle) {}
}
class AreaCalculator extends ShapeVisitor {
visitCircle(circle) {
return Math.PI * Math.pow(circle.radius, 2);
}
visitSquare(square) {
return Math.pow(square.side, 2);
}
visitTriangle(triangle) {
const s = (triangle.sideA + triangle.sideB + triangle.sideC) / 2;
return Math.sqrt(s * (s - triangle.sideA) * (s - triangle.sideB) * (s - triangle.sideC));
}
}
class PerimeterCalculator extends ShapeVisitor {
visitCircle(circle) {
return 2 * Math.PI * circle.radius;
}
visitSquare(square) {
return 4 * square.side;
}
visitTriangle(triangle) {
return triangle.sideA + triangle.sideB + triangle.sideC;
}
}
class Circle {
constructor(radius) {
this .radius = radius;
}
accept(visitor) {
return visitor.visitCircle( this );
}
}
class Square {
constructor(side) {
this .side = side;
}
accept(visitor) {
return visitor.visitSquare( this );
}
}
class Triangle {
constructor(sideA, sideB, sideC) {
this .sideA = sideA;
this .sideB = sideB;
this .sideC = sideC;
}
accept(visitor) {
return visitor.visitTriangle( this );
}
}
const circle = new Circle(5);
const square = new Square(4);
const triangle = new Triangle(3, 4, 5);
const areaCalculator = new AreaCalculator();
const perimeterCalculator = new PerimeterCalculator();
const circleArea = circle.accept(areaCalculator);
const squarePerimeter = square.accept(perimeterCalculator);
const triangleArea = triangle.accept(areaCalculator);
console.log(`Circle Area: ${circleArea}`);
console.log(`Square Perimeter: ${squarePerimeter}`);
console.log(`Triangle Area: ${triangleArea}`);
|
Output
Circle Area: 78.53981633974483
Square Perimeter: 16
Triangle Area: 6
In this code, we calculate the area of a circle, the perimeter of a square, and the area of a triangle using the Visitor pattern. The results are printed to the console.
Diagram of Visitor Pattern in Javascript Design Patterns
In this simplified diagram:
- Visitor is the visitor interface defining methods like visitElementA and visitElementB that correspond to the elements to be visited.
- ConcreteVisitorA is a concrete visitor class that implements the Visitor interface and provides implementations for visiting ElementA and ElementB.
- Element is an interface or abstract class defining the accept method, which takes a visitor as an argument. Both ConcreteElementA and ConcreteElementB implement this interface.
- ConcreteElementA and ConcreteElementB are concrete elements that implement the accept method to allow visitors to visit them.
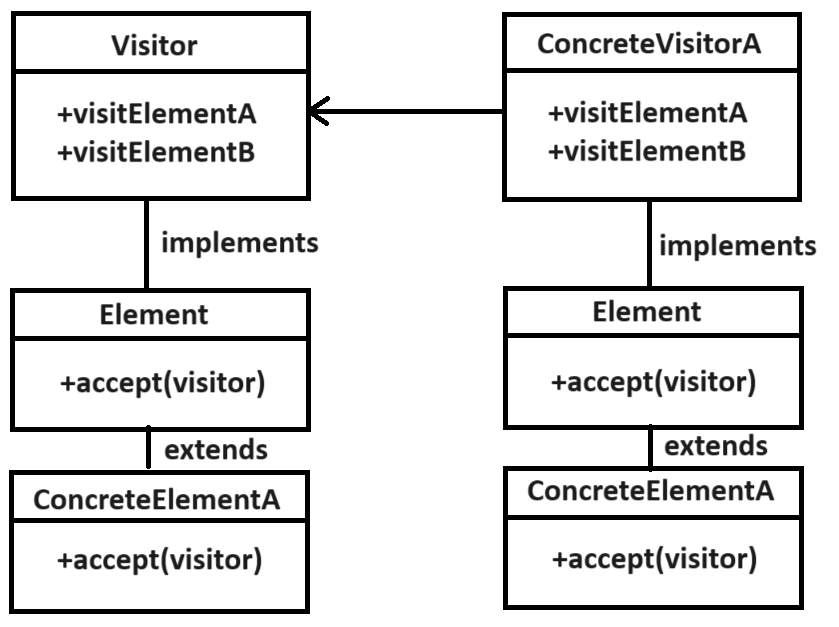
Flow diagram of Visitor Method
In the diagram, arrows indicate relationships, such as inheritance (extends) and interface implementation (implements). This simplified UML class diagram illustrates the core components and relationships in the Visitor pattern in JavaScript.
Advantages of the Visitor Pattern in JavaScript Design Patterns
- Separation of Concerns: The Visitor pattern helps separate the data structure (elements) from the operations (visitors) that can be performed on that structure. This separation of concerns promotes cleaner and more maintainable code because you can modify or extend the behavior without changing the element classes.
- Open-Closed Principle: It adheres to the Open-Closed Principle, one of the SOLID principles of object-oriented design. This principle states that classes should be open for extension but closed for modification. With the Visitor pattern, you can add new operations (new visitors) without altering the existing element classes, promoting code stability.
- Extensibility: It’s highly extensible. You can add new visitor implementations to perform new operations on elements without modifying those elements. This makes it suitable for scenarios where the set of operations may change frequently.
- Maintainability: By encapsulating related behaviors within visitor classes, the code becomes more organized and easier to understand and maintain. Each visitor focuses on a single operation, making it easier to modify or debug a specific behavior.
- Cleaner Code: The Visitor pattern can lead to cleaner code since it avoids the proliferation of conditional statements (e.g., if-else or switch) that are often used for selecting behavior based on object types. Instead, the behavior is encapsulated within the visitor classes.
- Reusability: Visitors can be reused across different element hierarchies or data structures. Once you create a visitor for a specific set of elements, you can reuse it with other sets of elements as long as they implement the visitor-accepting mechanism.
- Improved Testing: Separation of concerns and well-defined interfaces make it easier to write unit tests for the visitor classes and the elements separately. This enhances testability and reduces the complexity of testing individual behaviors.
- Dynamic Dispatch: The Visitor pattern often relies on dynamic dispatch, allowing the appropriate visitor method to be selected at runtime based on the actual type of the object being visited. This dynamic behavior can be powerful and flexible.
- Hierarchical Structures: The Visitor pattern is well-suited for traversing hierarchical structures, such as composite patterns (e.g., trees or graphs), where different operations need to be performed on different parts of the structure.
- Type Safety: When implemented correctly, the Visitor pattern can provide type safety by ensuring that all necessary visitor methods are implemented for each type of element, helping catch errors during compilation rather than runtime.
Disadvantages of the Visitor Pattern in JavaScript Design Patterns
- Complexity: Implementing the Visitor pattern can lead to increased code complexity. It introduces multiple classes for visitors, which can make the codebase harder to understand, especially for simple use cases.
- Coupling: The Visitor pattern can create a high degree of coupling between the elements being visited and the visitor classes. Each new element or operation often requires modifications to multiple classes (both elements and visitors), which can increase maintenance challenges.
- Violates Encapsulation: In some cases, the Visitor pattern can lead to a violation of encapsulation because it requires exposing internal details of elements to visitors. This can make it difficult to maintain a clean separation between an object’s interface and its implementation.
- Runtime Overhead: The use of dynamic dispatch (method resolution at runtime) to select the appropriate visitor method can introduce some runtime overhead, potentially affecting performance in performance-critical applications.
- Inflexibility: The Visitor pattern is not always the best choice for scenarios where new elements and new operations are frequently added. It may require modifying a significant amount of existing code when adding new elements or visitors.
- Limited Use Cases: The Visitor pattern is most useful when dealing with complex data structures or hierarchies. In simpler scenarios, it may introduce unnecessary complexity and boilerplate code.
- Code Bloat: For large hierarchies or numerous operations, the number of visitor classes can grow quickly, leading to code bloat and potentially making the codebase harder to manage.
- Maintainability: While it promotes maintainability in certain situations, the Visitor pattern can make the codebase less maintainable if not used judiciously. It may be challenging to navigate and understand the interactions between elements and visitors, especially for newcomers to the codebase.
- Limited Language Support: Some programming languages may not support the Visitor pattern as elegantly as others. Implementing dynamic dispatch and dealing with type hierarchies can be more challenging in languages with limited reflection capabilities.
- Increased Development Time: Implementing the Visitor pattern can require additional development time and effort, particularly when dealing with a large number of elements and operations.
Uses of the Visitor Pattern
The Visitor pattern is a powerful design pattern used in various scenarios in software development. Here are some common use cases for the Visitor pattern:
- Traversal of Complex Data Structures: When you have complex data structures like trees, graphs, or composite structures (e.g., directories and files), the Visitor pattern can be used to traverse these structures while performing specific operations at each node or element.
- Adding New Operations to Classes: When you need to add new behaviors or operations to a set of classes without modifying their code, the Visitor pattern allows you to encapsulate these behaviors in visitor classes, promoting the Open-Closed Principle.
- Type-Safe Operations: It can be used to ensure that operations are type-safe. By defining specific visitor methods for each type of element, you can catch type-related errors at compile-time rather than runtime.
- Distributed Behavior: In distributed systems, the Visitor pattern can be used to distribute behavior or processing logic across various components or nodes of the system while keeping the behavior encapsulated within visitors.
- Parsing and AST Processing: When building compilers, interpreters, or any system that deals with abstract syntax trees (ASTs), the Visitor pattern can be employed to traverse the AST and perform semantic analysis, code generation, or other operations.
- Database Query Generation: In database systems or ORMs (Object-Relational Mapping), the Visitor pattern can be used to generate complex SQL queries or other database operations based on an object-oriented representation of queries.
- GUI Components: In graphical user interfaces, the Visitor pattern can be used to perform various operations on UI components, such as rendering, event handling, or layout calculations.
- Document Manipulation: When working with documents, such as XML or JSON structures, the Visitor pattern can be used to traverse and manipulate document elements based on specific criteria.
- Optimizing Data Structures: In some cases, you might use the Visitor pattern to optimize data structures or perform transformations. For example, you could use it to collect statistics, validate data, or reorganize elements in a data structure.
- Logging and Debugging: The Visitor pattern can be used for logging, debugging, or profiling by attaching visitors to different parts of the code to gather information or trace execution.
- Modeling Game Entities: In game development, the Visitor pattern can be employed to model game entities and behaviors. Visitors can represent various actions that game entities can perform.
- Extensible Plugins: When building extensible systems with plugins or extensions, the Visitor pattern can be used to add new functionality through plugins without modifying the core code.
Conclusion
It’s important to note that while the Visitor pattern provides benefits in these scenarios, it also introduces some complexities, especially in terms of maintaining a clean separation of concerns and managing the relationships between elements and visitors. Therefore, its usage should be considered carefully based on the specific requirements of the application or system being developed.
Share your thoughts in the comments
Please Login to comment...