Bridge Method | JavaScript Design Pattern
Last Updated :
30 Oct, 2023
In software design, as systems grow and evolve, the complexity of their components can increase exponentially. This complexity often arises from the intertwining of different functionalities and features, making the system rigid, less maintainable, and harder to scale. The Bridge design pattern emerges as a solution to this problem, offering a way to separate a system’s abstractions from its implementations.
At its core, the Bridge pattern is about creating a bridge between two potentially complex systems, ensuring that changes in one will not affect the other. Imagine a bustling city with two sides separated by a river. Without a bridge, crossing from one side to the other would be a challenge, especially as the city grows and traffic increases. But with a bridge, the two sides can operate independently, yet remain connected in a controlled manner.
In software terms, the “city” represents our system’s functionalities, the “river” represents the system’s complexities, and the “bridge” represents the design pattern that connects and manages these complexities.
The Bridge pattern is especially useful in situations where multiple dimensions of a system need to evolve independently. For instance, if you have a set of operations that need to work on multiple platforms, instead of intertwining platform-specific code with operation-specific code, the Bridge pattern allows you to keep them separate.
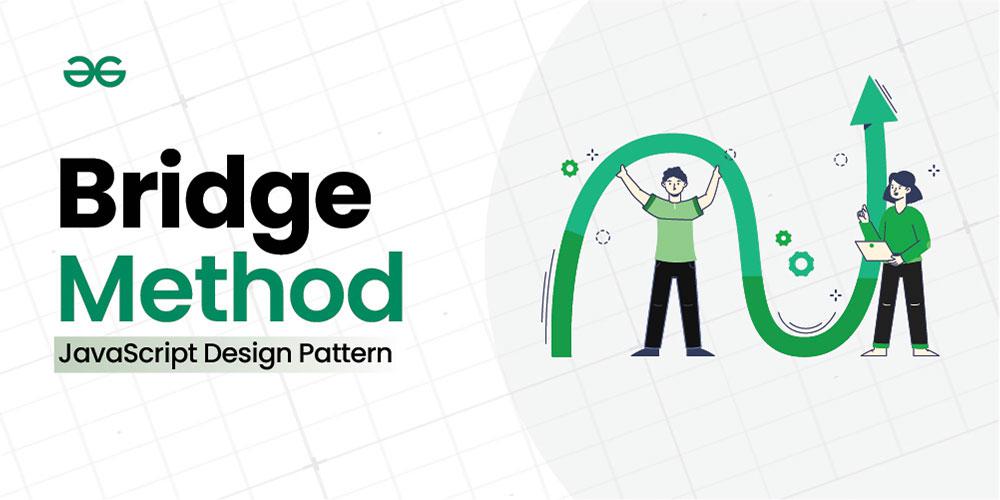
Important Topics for Bridge Method in JavaScript Design Pattern
Components of the Bridge Pattern in Javascript Design Patterns
The Bridge pattern is composed of several key components that work together to decouple an abstraction from its implementation. Here’s a detailed breakdown:
Abstraction
- Role: Represents the high-level interface or control layer of the pattern. It maintains a reference to the Implementor but only communicates through a generalized interface.
- Responsibility: Defines and manages the interface for the “control” side of the two class hierarchies. It’s the entry point for the client and delegates the work to the Implementor.
- Example: In the context of a UI library, the Abstraction might represent a generic UI element, like a Button.
RefinedAbstraction
- Role: Extends or expands upon the Abstraction by providing more specific or varied implementations.
- Responsibility: Offers variants of the abstraction. While the Abstraction provides a generalized interface, the RefinedAbstraction might offer more specialized or diverse functionalities.
- Example: In the UI library context, RefinedAbstraction could represent specialized buttons like IconButton or ToggleButton.
Implementor
- Role: This is the interface for the implementation classes. It defines how the Abstraction communicates with the various implementations.
- Responsibility: Provides the contract for the “implementation” side of the two class hierarchies. It doesn’t need to align directly with the Abstraction’s interface, allowing for flexibility in how the implementation is carried out.
- Example: In the UI library scenario, the Implementor might define methods like render or handleEvent, but it won’t specify how these methods work for a specific platform.
ConcreteImplementor
- Role: Provides concrete implementations of the Implementor interface. This is where the actual work gets done.
- Responsibility: Implements the specifics. While the Implementor provides a general contract, the ConcreteImplementor fulfills this contract in a specific way.
- Example: For our UI library, a ConcreteImplementor might be WebButtonRenderer or MobileButtonRenderer, detailing how a button is rendered on a web platform versus a mobile platform.
How The Components Interact With Each Other?
The client interacts with the Abstraction, which in turn delegates the work to its Implementor reference. This delegation is often where the “bridge” happens. The client isn’t aware of the specific ConcreteImplementor doing the work, ensuring a clean separation of concerns.
For instance, when a client wants to render a button on a web platform, it interacts with the Button abstraction (which might be a RefinedAbstraction like IconButton). The Button then delegates the rendering task to its Implementor, which, in this case, would be the WebButtonRenderer (a ConcreteImplementor).
This separation allows developers to introduce new types of buttons or support new platforms without altering existing code, demonstrating the power and flexibility of the Bridge pattern.
Example of the Bridge Pattern in Javascript Design Patterns: Remote Control and Devices
Imagine you’re designing a universal remote control system. You have different types of remote controls (basic, advanced) and various devices (TV, radio). Without the Bridge pattern, you’d end up with a combinatorial explosion of classes like BasicTVRemote, AdvancedTVRemote, BasicRadioRemote, AdvancedRadioRemote, and so on.
With the Bridge pattern, you can separate the remote control type (the abstraction) from the device it controls (the implementation), allowing you to add new remote types or devices without affecting the other hierarchy.
Javascript
class Device {
turnOn() {}
turnOff() {}
}
class TV extends Device {
turnOn() {
console.log("TV is now ON");
}
turnOff() {
console.log("TV is now OFF");
}
}
class Radio extends Device {
turnOn() {
console.log("Radio is now ON");
}
turnOff() {
console.log("Radio is now OFF");
}
}
class RemoteControl {
constructor(device) {
this .device = device;
}
togglePower() {
console.log("Default implementation of togglePower");
}
}
class BasicRemote extends RemoteControl {
togglePower() {
console.log("Using Basic Remote to toggle power.");
this .device.turnOn();
}
}
class AdvancedRemote extends RemoteControl {
togglePower() {
console.log("Using Advanced Remote to toggle power with additional features.");
this .device.turnOff();
}
}
const tv = new TV();
const radio = new Radio();
const basicTVRemote = new BasicRemote(tv);
basicTVRemote.togglePower();
const advancedRadioRemote = new AdvancedRemote(radio);
advancedRadioRemote.togglePower();
|
Output
Using Basic Remote to toggle power.
TV is now ON
Using Advanced Remote to toggle power with additional features.
Radio is now OFF
Explanation of the above code:
In this example:
- Device (like TV and Radio) acts as the Implementor. It defines the interface for the “implementation” side of the two class hierarchies.
- RemoteControl (and its variants BasicRemote and AdvancedRemote) acts as the Abstraction. It represents the interface for the “control” side of the hierarchies.
The RemoteControl doesn’t directly control the device. Instead, it delegates the control operations to the associated Device object. This separation allows us to add new types of remote controls or devices without affecting the other side of the hierarchy, demonstrating the power and flexibility of the Bridge pattern.
Advantages of the Bridge Method in Javascript Design Patterns
- Decoupling of Abstraction and Implementation: The primary benefit of the Bridge pattern is the separation of the abstraction from its implementation. This decoupling ensures that changes in the abstraction hierarchy don’t affect the implementation hierarchy and vice versa.
- Improved Extensibility: Both the abstraction and its implementation can be extended independently. This means you can add new operations to the abstraction or introduce new implementations without altering existing code.
- Single Responsibility Principle: The pattern adheres to the Single Responsibility Principle, ensuring that a class has only one reason to change. This makes the system more maintainable.
- Composition Over Inheritance: The Bridge pattern prefers composition over inheritance, which provides more flexibility in the structuring of code. This means you can change the behavior of an object at runtime by changing its composition rather than its class.
- Reduces Class Explosion: In systems where classes can be combined in multiple ways, the Bridge pattern can drastically reduce the number of resulting classes.
Disadvantages of the Bridge Method in Javascript Design Patterns
- Increased Complexity: While the pattern promotes flexibility, it can introduce complexity in the codebase, especially when there are multiple levels of abstraction and implementation. This can make the system harder to understand for newcomers.
- Initial Setup Overhead: Setting up the Bridge pattern can seem like an overhead, especially for simpler scenarios where such a level of decoupling might not be necessary.
- Requires Deep Understanding: For the pattern to be effective, developers need to understand its intent thoroughly. Misuse or overuse can lead to an overly complicated design.
- Potential Performance Overhead: The indirection introduced by the bridge can, in some scenarios, introduce a slight performance overhead. However, in most cases, this overhead is negligible.
Real-world Application of the Bridge Pattern in Javascript Design Patterns
Graphical User Interfaces (GUIs)
- Scenario: Consider a cross-platform GUI library. The library needs to provide consistent components like buttons, sliders, and text boxes across multiple platforms (Windows, macOS, Linux).
- Application of Bridge: The component’s general behavior (like a button being clicked) is the abstraction. The actual rendering and handling of the component on each platform is the implementation. The Bridge pattern allows the GUI library to add new components or support new platforms without intertwining the two hierarchies.
Web Service Integrations
- Scenario: An application needs to integrate with various payment gateways like PayPal, Stripe, and Square.
- Application of Bridge: The general process of making a payment is the abstraction (e.g., authenticate, transfer funds, confirm payment). The specific API calls and data handling for each payment gateway is the implementation. Using the Bridge pattern, the application can easily switch between payment gateways or add new ones without altering the core payment processing logic.
Device Drivers
- Scenario: A computer needs to communicate with a range of printers, each having its own communication protocol.
- Application of Bridge: The general command to print is the abstraction. The specific steps and protocols to communicate with each printer model form the implementation. The Bridge pattern ensures that adding support for a new printer or updating an existing one doesn’t require changes to the main printing logic of the computer.
Media Players and Codecs
- Scenario: A media player application that needs to support various file formats like MP3, MP4, AVI, etc.
- Application of Bridge: The user interface and controls (play, pause, stop) of the media player are the abstraction. The decoding and playing of each file format using different codecs is the implementation. With the Bridge pattern, the media player can easily support new file formats without changing the user interface or control logic.
Networking Tools and Protocols
- Scenario: A network monitoring tool that needs to support various communication protocols like TCP, UDP, and ICMP.
- Application of Bridge: The monitoring and reporting features are the abstraction. The specific methods to capture and analyze traffic for each protocol are the implementation. By employing the Bridge pattern, the tool can seamlessly add support for new protocols without affecting its core monitoring features.
Conclusion
In each of these real-world scenarios, the Bridge pattern provides a structured way to keep the high-level logic (abstraction) separate from the low-level details (implementation). This separation ensures flexibility, scalability, and maintainability, especially in systems that need to evolve along multiple axes.
Share your thoughts in the comments
Please Login to comment...