Observer Method – JavaScript Design Pattern
Last Updated :
08 Nov, 2023
Observer design pattern, a staple of behavioral design patterns in JavaScript, provides a means to establish a one-to-many relationship between objects. This design pattern is especially valuable for decoupling components and facilitating extensibility in software applications. By employing this pattern, you can have one object (the subject) notify multiple observers (subscribers) about changes or events without requiring them to have direct knowledge of each other. This decoupling enhances maintainability and extensibility in your software projects.
Important Topics for Observer Method in JavaScript
Participants of Observer Pattern in JavaScript:
The Observer pattern typically consists of three key participants:
- Subject (e.g., WeatherStation): The subject is the entity responsible for tracking a particular set of data or conditions and notifying observers when significant changes occur. In our example, we’ll use a WeatherStation as the subject.
- Observers (e.g., DisplayDevices) : Observers are entities that register interest with the subject to receive updates when specific events or changes occur. In our case, we’ll represent these as DisplayDevice objects.
- Notify Method : The subject provides a notify method that allows it to inform registered observers when changes take place. Observers, in turn, implement an update method that is called by the subject when changes occur.
Implementation of Observer Method:
Implementing the Observer Pattern with a Weather Monitoring System Example:
Javascript
class WeatherStation {
constructor() {
this .observers = [];
this .temperature = 0;
}
addObserver(observer) {
this .observers.push(observer);
}
removeObserver(observer) {
this .observers = this .observers.filter(obs => obs !== observer);
}
setTemperature(temperature) {
this .temperature = temperature;
this .notifyObservers();
}
notifyObservers() {
this .observers.forEach(observer => {
observer.update( this .temperature);
});
}
}
class DisplayDevice {
constructor(name) {
this .name = name;
}
update(temperature) {
console.log(`${ this .name} Display: Temperature is ${temperature}°C`);
}
}
const weatherStation = new WeatherStation();
const displayDevice1 = new DisplayDevice( "Display 1" );
const displayDevice2 = new DisplayDevice( "Display 2" );
weatherStation.addObserver(displayDevice1);
weatherStation.addObserver(displayDevice2);
weatherStation.setTemperature(25);
weatherStation.setTemperature(30);
|
Output
Display 1 Display: Temperature is 25°C
Display 2 Display: Temperature is 25°C
Display 1 Display: Temperature is 30°C
Display 2 Display: Temperature is 30°C
In the output, you can see that the two display devices, “Display 1” and “Display 2,” receive and display the temperature updates when the temperature changes. The first update sets the temperature to 25°C, and the second update changes it to 30°C. Both display devices log the updated temperature values to the console.
In the above Code,
- WeatherStation Class: This class represents a weather station and is responsible for managing temperature data and notifying observers when the temperature changes.
- Constructor: The WeatherStation class begins with a constructor function. It initializes two important properties:
- Observers: An empty array to hold all the observers (in this case, the display devices).
- Temperature: An initial temperature value set to 0°C.
- AddObserver Method: The addObserver method allows observers to subscribe to the weather station. It takes an observer parameter (in this context, a display device) and adds it to the list of observers.
- RemoveObserver Method: The removeObserver method enables the removal of observers from the list. It takes an observer as a parameter and filters out the matching observer from the list of observers.
- SetTemperature Method: The setTemperature method is used to update the temperature data. It takes a temperature parameter and sets the temperature property to the new value. After updating the temperature, it calls notifyObservers().
- NotifyObservers Method: The notifyObservers method iterates through the list of observers (display devices) and informs them of the temperature change. For each observer, it calls the update method and passes the current temperature as an argument.
- DisplayDevice Class: This class represents a display device, such as a digital thermometer, and is designed to respond to temperature updates.
- Constructor: The DisplayDevice class begins with its constructor function, which takes a name parameter. This parameter represents the name or identifier of the display device.
- update Method: The update method is called when the display device receives a temperature update from the weather station. It logs a message to the console, indicating the device’s name and the current temperature in degrees Celsius.
- Creating Instances: Instances of the WeatherStation and DisplayDevice classes are created. Additionally, two display devices are instantiated with names, ‘Display 1’ and ‘Display 2’.
- Adding Observers: Both display devices are added as observers to the weather station using the addObserver method. This means they will be notified when the temperature changes.
- Simulating Temperature Changes: To simulate temperature changes, the setTemperature method of the weather station is called twice. First, it sets the temperature to 25°C, and then it updates it to 30°C. Each time the temperature changes, the observers are notified, and they log the updated temperature to the console.
Diagram Explanation of Observer Method in JavaScript
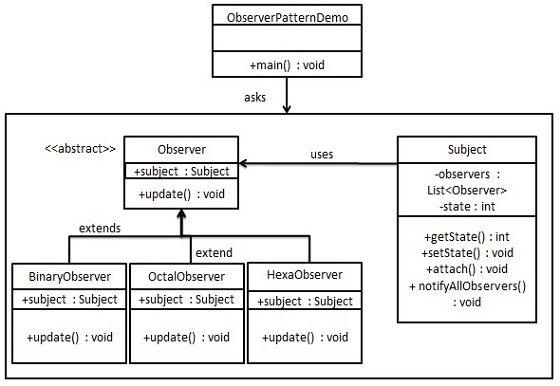
In this diagram:
- WeatherStation (Subject): This represents our central hub for monitoring the weather. It’s like a control center for checking the temperature.
- Temperature (Data): This is the actual temperature data that can change over time. It’s what we’re interested in monitoring.
- DisplayDevice (Observer): These are like digital display screens or devices. They want to show the current temperature to users.
How it Works:
- Monitoring Temperature: The WeatherStation constantly keeps an eye on the Temperature data. It’s like a sensor that detects changes in temperature.
- Notifying Observers: When the WeatherStation senses a change in temperature, it immediately notifies the DisplayDevices. This is similar to sending a message to each screen.
- Updating Display: Each DisplayDevice, upon receiving the notification, updates its own display with the new temperature data. It’s like each screen showing the updated temperature to the user.
Publish/Subscribe Pattern:
The Observer Method is often associated with the Publish/Subscribe pattern. In this pattern, there is a message broker (or event bus) that acts as an intermediary between publishers (objects producing events) and subscribers (objects interested in specific events). Publishers send events to the broker, and subscribers subscribe to specific event types.
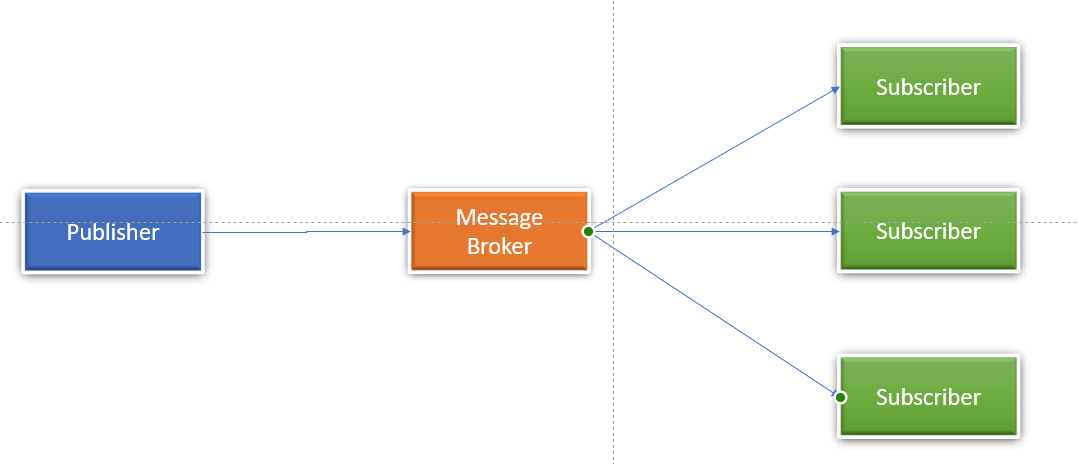
In this diagram:
- Publisher: This is the source of data or events. It publishes data to specific topics.
- Subscriber: These are entities interested in receiving data or events from specific topics.
- Topic: It’s like a channel or category that acts as an intermediary between publishers and subscribers. It stores the list of subscribers for a specific type of data or event.
How it Works:
- Publishing: The Publisher publishes data to a particular Topic. It’s like a radio station broadcasting on a specific channel.
- Subscribing: Subscribers express their interest by subscribing to specific Topics. They say, “I want to listen to data from this channel.”
- Forwarding: When the Publisher sends data to a Topic, the Topic forwards that data to all Subscribers interested in that Topic. It’s like broadcasting a message to everyone tuned to a particular radio channel.
Differences Between Observer and Publish/Subscribe Patterns:
Subject directly notifies observers when an event occurs.
|
Message broker (or event bus) acts as an intermediary between publishers and subscribers.
|
Typically focuses on a single event (e.g., temperature change).
|
Supports multiple event types; subscribers can choose to subscribe to specific event types.
|
Loose coupling between subjects and observers.
|
Offers flexibility for decoupling among different publishers and subscribers.
|
Observers can be reused across different subjects.
|
Can be used to decouple various components in a system.
|
Memory management can be simpler due to a straightforward relationship between subjects and observers.
|
Requires proper management of event subscriptions and unsubscriptions to prevent memory leaks.
|
Generally lower performance overhead since it directly invokes observer methods.
|
Slightly higher performance overhead due to the involvement of a message broker.
|
Advantages of the Observer Method:
- Enhanced Decoupling : The Observer pattern fosters a desirable level of separation between components in a software system. It allows the subject to communicate changes to multiple observers without these entities being tightly bound to one another. As a result, changes made to one component do not necessitate extensive modifications in others, contributing to a more modular and maintainable codebase.
- Reusability Across Subjects : Observers are designed to be reusable. This reusability is particularly advantageous when various subjects within an application require similar notification mechanisms. It promotes code reuse, reducing redundancy in your code.
- Facilitation of Event-Driven Programming : The natural event-driven nature of the Observer pattern is a major asset. It aligns well with scenarios where you need to respond to events or changes as they occur, especially in environments that involve user interactions, such as web applications. This event-driven approach simplifies handling asynchronous operations.
- Dynamic Adaptability : The Observer pattern allows for dynamic addition and removal of observers from a subject. This dynamic adaptability is invaluable when your application’s requirements evolve over time. You can accommodate these changes without having to rewrite substantial portions of your codebase.
- Ease of Testing : Observers can be tested independently, thanks to their decoupled nature. This lends itself well to unit testing practices, as you can create mock observers to simulate different scenarios and validate individual components.
- Scalability Support : The Observer pattern is well-suited for scenarios that involve scaling the number of observers on the fly. It can efficiently manage a substantial volume of observers without compromising performance.
Disadvantages of the Observer Method:
- Complexity Concerns : Although the Observer pattern promotes decoupling, it’s crucial to exercise caution regarding its use. Excessive application of the pattern can introduce complexity and intricacy into the codebase. Managing numerous observers and their interactions can become challenging, leading to less maintainable code.
- Memory Management Responsibility : Proper memory management is paramount in the Observer pattern. Neglecting to remove observers when they are no longer necessary can result in memory leaks, a subtle but significant issue. Developers must be meticulous in managing observer subscriptions and unsubscriptions.
- Performance Overhead : In situations where there is a substantial number of observers, the notification process can introduce performance overhead. Iterating through all observers and invoking their update methods can impact the application’s real-time performance. Thus, developers need to be mindful of performance considerations, especially in time-sensitive systems.
- Potential for Event Cascades : The Observer pattern’s cascade effect is a potential challenge. When one observer’s update method triggers changes in other observers, it can lead to a cascade of events, making the system harder to predict and debug.
- Asynchronous Event Coordination : Coordinating the timing of updates between observers in asynchronous event scenarios can become intricate. Ensuring that observers handle events in a synchronized manner requires careful design and consideration.
- Order of Notification Complexity : In some scenarios, the order in which observers are notified may be significant. Managing the order of notification can add another layer of complexity to the implementation, as it becomes necessary to specify and maintain a specific notification sequence.
Conclusion :
The Observer design pattern offers a range of advantages, including enhanced decoupling, reusability, and support for event-driven programming. Nevertheless, it does present potential challenges, such as complexity, memory management, and performance overhead, which developers should navigate with care, balancing the benefits against the trade-offs in their specific applications.
Share your thoughts in the comments
Please Login to comment...