Simple Steps to Learn Any Programming Language in 2024
Last Updated :
06 Feb, 2024
Learning a Programming Language in 2024 could open endless possibilities. In today’s world where technology is used everywhere the ability to speak to computers is not just a skill but a power that sets you apart from the normal folks. It is not the case that only tech elites can learn a programming language rather anyone with a curious mind can learn and excel in it.
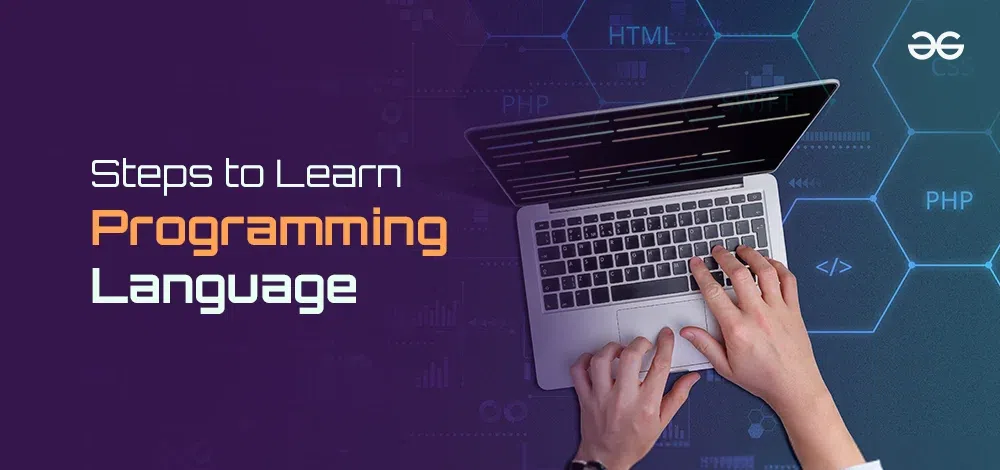
Learning a language is not only about memorizing the syntax, it is more about innovation and problem-solving skills. As our world is getting more digitized learning a programming language opens doors to lucrative fields like web development, android development, machine learning, etc.
What is a Programming Language?
Programming Language is a set of instructions following a specific syntax that a computer can understand and execute. It acts as a bridge between humans and computers allowing humans to communicate with the machine. Just as people use languages to convey their ideas to each other, programmers use programming language to convey to computers which empowers them to create software.
Steps To Learn Programming Language in 2024
Whether you’re interested in web development, data science, or simply want to boost your problem-solving skills, learning a programming language is crucial. This guide will walk you through the key steps to master a programming language, from choosing the right one to diving into advanced concepts. Whether you’re just starting out or looking to enhance your skills, this guide is your go-to resource.
1. Choose a Programming Language
Before starting your journey of learning a programming language it is essential to choose the right programming language. Consider the purpose of your learning whether it is DSA, web development, mobile app creation, data science, or another field. If you are a beginner and want to go with an easy syntax language then go for Python, if excelling in solving DSA problems is your goal then C++, Java might be the right choice for you because the DSA community support in these languages is large. If you want to try on web development then Javascript can help you but initially it is recommended to choose something from C++, Java or Python to get your basics clear and then proceed further.
2. Building your Base
Before starting to learn a programming language it is better to have a basic understanding of computer architecture such as CPU, RAM and input, and output devices. The basic knowledge of system software that works under the hood to ensure things keep running smoothly such as operating system, compiler and interpreter will give you foundational knowledge that will make your journey smooth.
3. First Steps with Your Code
- Learn to output (print) information in your desired programming language in various formats such as :
- Printing Basics: Hello World and Beyond
- Formatting and Creativity: Multi-line Printing and More
C++
#include <iostream>
using namespace std;
int main()
{
cout << "GFG!" ;
return 0;
}
|
C
#include <stdio.h>
int main() {
printf ( "Hello World!\n" );
return 0;
}
|
Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println( "Hello World!" );
}
}
|
Python
Javascript
console.log( "Hello World!" );
|
- Learn to listen : Input statements
- Reading Numbers, Decimals, and Words from the User
- Working with User Input: Printing and Manipulation
Python
number = int ( input ( "Enter a number: " ))
decimal = float ( input ( "Enter a decimal: " ))
word = input ( "Enter a word: " )
print ( "Number:" , number)
print ( "Decimal:" , decimal)
print ( "Word:" , word)
|
- Learn about operators and variables , Operators are the symbols which perform mathematical and logical operations . The combination of variable , constants and operators create an expression. There are various types of operators in a programming language such as arithmetic operators , assignment operators , bitwise operators etc.
- Variables are named containers that store data in a program. It has a unique name, data type, scope and lifetime. They can change their value during the execution of the program.
- Try writing simple programs that find the sum of two numbers, compute simple interest, compound interest, roots of quadratic equations, etc.
Want to learn more about operators check out Types of Operators
4. Conditional Statements (If… Else)
- These are statements in a programming language that allow a program to execute different blocks of code based on certain criteria.
- They are used for decision making that is they perform actions depending on whether the condition is true or false.
- These statements are useful when we want the program to react when some change occurs. e.g. write programs to find whether an input number is even or odd, if the input gender shortcut is male, female or others.
C
#include <stdio.h>
int main()
{
int number;
printf ( "Enter a number: " );
scanf ( "%d" , &number);
if (number % 2 == 0) {
printf ( "%d is even.\n" , number);
}
else {
printf ( "%d is odd.\n" , number);
}
return 0;
}
|
5. Repeating Yourself Efficiently: Loops
- In programming, a loop is a concept that is executed once but repeats a set of instructions until a condition is met.
- It is used to automate repetitive tasks and simplify writing code.
- A loop can be inside another loop which is known as a nested loop.
You can try examples such as programs like a table of a given number, the factorial of a number, GCD of two numbers, divisors of a number, prime factors of a number, and check if a number is a prime.
Python
number = 5
for i in range ( 1 , 11 ):
result = number * i
print (f "{number} x {i} = {result}" )
|
6. Learn About Functions
Functions are blocks of code that perform a specific task. They take the data as input process it and return the result. Once a function is written it can be used repeatedly. Functions can be called within another function. For practicing what you can do is that the problems you solved in step 4 try solving them by writing functions like printable(n), factorial(n), etc.
Python
def print_table(number):
for i in range ( 1 , 11 ):
result = number * i
print (f "{number} x {i} = {result}" )
print_table( 5 )
|
7. Array and Strings
- Array are collection of elements of the same data type. It is of fixed size and it has mutable contents. They can contain values of any data-type .
- Strings are sequence of characters, they can be of variable size. In many programming languages, strings are treated as arrays of characters. Each character in the string has a separate position or index like the elements in the array.
To get more hands on try solving the common problems like string rotation, reverse a string, check if a string is palindrome or not. Check out the below example to reverse a string
C++
#include <bits/stdc++.h>
using namespace std;
void reverseStr(string& str)
{
int n = str.length();
for ( int i = 0, j = n - 1; i < j; i++, j--)
swap(str[i], str[j]);
}
int main()
{
string str = "geeksforgeeks" ;
reverseStr(str);
cout << str;
return 0;
}
|
Java
import java.io.*;
class GFG {
static void reverseStr(String str)
{
int n = str.length();
char []ch = str.toCharArray();
char temp;
for ( int i= 0 , j=n- 1 ; i<j; i++,j--)
{
temp = ch[i];
ch[i] = ch[j];
ch[j] = temp;
}
System.out.println(ch);
}
public static void main(String[] args) {
String str = "geeksforgeeks" ;
reverseStr(str);
}
}
|
Python3
def reverseStr( str ):
n = len ( str )
i, j = 0 , n - 1
while i < j:
str [i], str [j] = str [j], str [i]
i + = 1
j - = 1
if __name__ = = "__main__" :
str = "geeksforgeeks"
str = list ( str )
reverseStr( str )
str = ''.join( str )
print ( str )
|
8. Object Oriented Programming
- Learn about the fundamentals of a object oriented programming like classes, objects, OOP principles, etc.
-  It is a programming paradigm that uses the concept of “objects” to represent something with properties and methods. Objects can contain data and code to manipulate that data.Â
- The aim of this paradigm is to implement real world entities like inheritance, hiding , polymorphism in the programming. It is the best way to create complex programs.
Python3
class Person:
def __init__( self , name, age):
self .name = name
self .age = age
person1 = Person(name = "Rahul" , age = 25 )
print (f "{person1.name} is {person1.age} years old." )
|
9. Diving Deeper into Data Structure and Algorithms(DSA)
Now you have learned the basics of the programming language. If you wish to go deeper and want to be a more proficient programmer, you need to learn about data structures and algorithms. First implement all standard data structures and algorithms on your own, then learn about libraries of your learned language so that you can efficiently solve problems. Data structures and algorithms are fundamental to programming.  A data structure is a collection of data types used to store and modify data. An algorithm is a set of instructions that solve a problem.Â
Want to learn in depth about Data Structures and Algorithms, Check Out – Data Structures and Algorithms – Self Paced
Conclusion
This article has explained to you the basic steps to learn a programming language now what’s next remember that writing a program is not only about memorizing the syntax but is about developing problem problem-solving mindset. The journey ahead lies in continuous exploration, you can take help from online courses, books, and tutorials each will offer you a unique insight. Try building projects on your chosen domain with your specific tech stack. You are going to learn the most while building projects. Join thriving communities, share your code and collaborate on projects. Learn from others’ experiences and contribute your own knowledge to help new programmers in the community.
FAQs
Why is learning a programming language important in today’s world?
In a tech-driven world, it sets individuals apart by enabling them to communicate with computers, opening doors to lucrative fields such as web development, android development, and machine learning
Do I need to be a tech elite to learn a programming language?
No, anyone with a curious mind can learn programming. The focus is on innovation and problem-solving skills making it accessible to individuals from various backgrounds.
What are the essential steps to learn a programming language?
The steps include building a foundational understanding of computer architecture, learning to write basic code (printing, input/output), understanding operators and variables, exploring conditional statements and loops, delving into functions, arrays, and strings, learning about object-oriented programming (OOP), and finally, diving deeper into data structures and algorithms(DSA).
How can I deepen my programming skills after learning the basics?
To become a more proficient programmer, dive into data structures and algorithms. Implement standard data structures and algorithms on your own and then explore libraries in your chosen language. Understanding data structures and algorithms is fundamental to programming as it helps in you writing optimize code.
Share your thoughts in the comments
Please Login to comment...