State Hooks in React
Last Updated :
20 Feb, 2024
State Hooks, introduced in React 16.8, revolutionized how developers manage state in functional components. Before State Hooks, state management was primarily confined to class components using the setState
method. State Hooks, such as useState
, enable functional components to manage local state effortlessly, aligning with React’s philosophy of simplicity and composability.
- useState hook is used to declare state variable in functional components
- useReducer hook is used when state management becomes difficult with useState Hook
There are different state hooks we will learn about them:
useState Hook:
useState() hook allows one to declare a state variable inside a function. It should be noted that one use of useState() can only be used to declare one state variable. It was introduced in version 16.8.
Syntax:
const [var, setVar] = useState(0);
Internal working of useState hook:
useState() maintains state by updating a stack within the functional component’s object in memory. Each render creates a new stack cell for the state variable. The stack pointer tracks the latest value for subsequent renders. Upon user-initiated refresh, the stack is cleared, triggering a fresh allocation for the component’s rendering.
Example: Below is the code example of useState Hook:
Javascript
import React, { useState } from 'react' ;
function App() {
const [click, setClick] = useState(0);
return (
<div>
<p>You clicked {click} times</p>
<button onClick={() => setClick(click + 1)}>
Click me
</button>
</div>
);
}
export default App;
|
Steps to run the App:
npm start
Output:
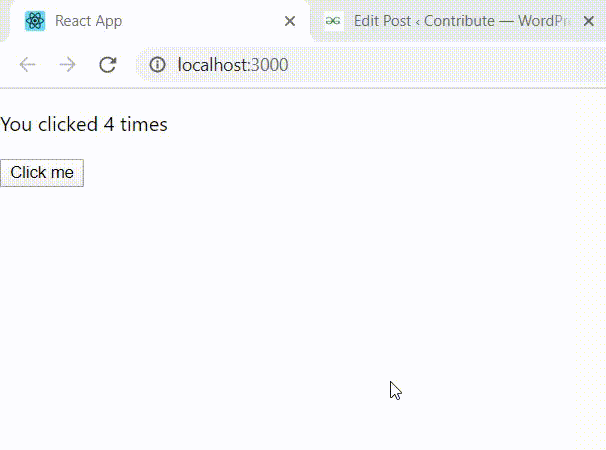
useReducer
Hook:
The useReducer Hook is the better alternative to the useState hook and is generally more preferred over the useState hook when you have complex state-building logic or when the next state value depends upon its previous value or when the components are needed to be optimized.
The useReducer hook takes three arguments including reducer, initial state, and the function to load the initial state lazily.
Syntax:
const [state, dispatch] = useReducer(reducer, initialArgs, init);
Example:Â Here reducer is the user-defined function that pairs the current state with the dispatch method to handle the state, initialArgs refers to the initial arguments and init is the function to initialize the state lazily.
Javascript
import React,
{
useReducer
} from "react" ;
const initialState = 0;
const reducer = (state, action) => {
switch (action) {
case "add" :
return state + 1;
case "subtract" :
return state - 1;
case "reset" :
return 0;
default :
throw new Error( "Unexpected action" );
}
};
const App = () => {
const [count, dispatch] =
useReducer(reducer, initialState);
return (
<div>
<h2>{count}</h2>
<button onClick={() => dispatch( "add" )}>
add
</button>
<button onClick={() => dispatch( "subtract" )}>
subtract
</button>
<button onClick={() => dispatch( "reset" )}>
reset
</button>
</div>
);
};
export default App;
|
Steps to run the App:
npm start
Output:
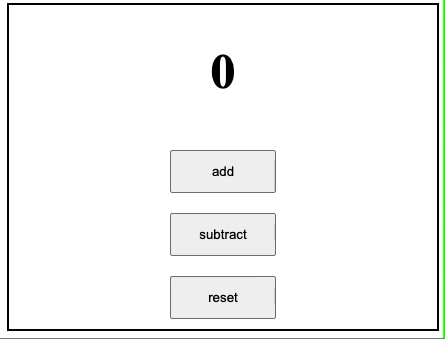
Share your thoughts in the comments
Please Login to comment...