In Spring Boot, Spring Security is the most powerful and customizable authentication and access control framework for Java applications, and it provides strong security features to protect web applications from various security threats such as authentication, authorization, session management, and web vulnerabilities. It is highly flexible and easy to implement with Spring applications.
Key Terminologies
- Authentication: It is the process of verifying the identity of a user. It can involve collecting the credentials of the user and validating them.
- Authorization: Authorization is the process of determining what action the authentication user is allowed to access based on their roles and permissions.
- Principal: It can be used to represent the identity of the current user and it can typically contain information such as username and any other identifying information.
- Granted Authority: It can be used to represent the permission or role granted to the principal and it can be used to define the actions the principal is allowed to access.
- Authentication Provider: The authentication provider can be responsible for authenticating mechanisms and user stores to verify user identities.
Types of Spring Security Filters
- Authentication Filter: This filter can handle the authentication process and it can typically process login requests and validate user credentials
- Authorization Filter: This filter can be used to enforce the access policies by checking whether the authenticated user has the necessary permissions to access the resource or an action.
- Session Management Filter: It can manage the user sessions and it can include session creation, expiration and tracking.
- CSRF Protection Filter: It can be used to protect against cross-site request forgery attacks by generating and validating unique tokens for each request.
- Logout Filters: It can handle the user logout request and it can perform clean-up tasks such as invalidating sessions and removing the authentication tokens.
- Cors Filters: They can be used to implement cross-origin resource-sharing policies to control access to resources from different origins.
- Header Filters: These can be used to manipulate HTTP headers for security purposes such as adding security-related headers to responses.
- Custom Filters: These can be used by developers to implement custom security filters to their applications with specific requirements.
Prerequisites
- InteljIdea Ultimate install your computer
- JDK tool kit also
- MongoDB compass required to be installed in your local machine
- Required knowledge of advanced Java
- Basic of Knowledge spring Security
- Basic Knowledge of JWT tokens
Example Project
In this project, We can develop the User Service authentication system consists of the USER and ADMIN roles and it can contains the JWT token authentication System.
Create the spring project using InteljIdea ultimate and duration of the project creation adding the below dependencies:
- Spring Web
- Spring Dev Tools
- Spring Security
- Lombok
- Spring data Mongodb
External dependencies:
implementation 'io.jsonwebtoken:jjwt-api:0.12.3'
implementation 'io.jsonwebtoken:jjwt-impl:0.12.3'
implementation 'io.jsonwebtoken:jjwt-jackson:0.12.3'
runtimeOnly 'io.jsonwebtoken:jjwt-jackson:0.12.3'
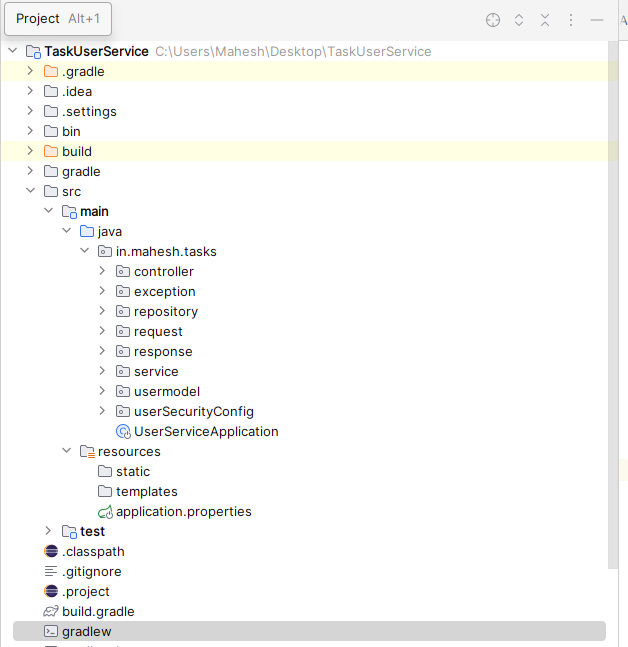
Application.properities:
server.port=8081
spring.data.mongodb.uri=mongodb://localhost:27017/userData
spring.application.name=USER-SERVICE
Step 1: Once completed the configuration of the database, eureka server, Spring security and Zipkin server. Create the one package in in.mahesh.tasks and named as usermodel and create the class named User into that package.
Go to src > java > in.mahesh.tasks > usermodel > User and put the code below:
Java
package in.mahesh.tasks.usermodel;
import java.util.List;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Document(collection = "user")
@AllArgsConstructor
@NoArgsConstructor
@Data
public class User {
@Id
private String id;
private String fullName;
private String email;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private String password;
private String role = "ROLE_CUSTOMER";
private String mobile;
private List<Long> completedTasks;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public List<Long> getCompletedTasks() {
return completedTasks;
}
public void setCompletedTasks(List<Long> completedTasks) {
this.completedTasks = completedTasks;
}
public String get_id() {
return id;
}
public void set_id(String id) {
this.id = id;
}
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
public String getMobile() {
return mobile;
}
public void setMobile(String mobile) {
this.mobile = mobile;
}
}
Step 2: Create the one package in in.mahesh.tasks and named as repository and create the interface named UserRepository into that package.
Go to src > java > in.mahesh.tasks > repository > UserRepository and put the code below:
Java
package in.mahesh.tasks.repository;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.data.mongodb.repository.Query;
import org.springframework.stereotype.Repository;
import in.mahesh.tasks.usermodel.User;
@Repository
public interface UserRepository extends MongoRepository<User,String> {
@Query("{email :?0}")
User findByEmail(String email);
}
Step 3: Create the one package in in.mahesh.tasks and named as service and create the class named UserService into that package.
Go to src > java > in.mahesh.tasks > service > UserService and put the code below:
Java
package in.mahesh.tasks.service;
import in.mahesh.tasks.exception.UserException;
import in.mahesh.tasks.usermodel.User;
import java.util.List;
public interface UserService {
public List<User> getAllUser() throws UserException;
public User findUserProfileByJwt(String jwt) throws UserException;
public User findUserByEmail(String email) throws UserException;
public User findUserById(String userId) throws UserException;
public List<User> findAllUsers();
}
Step 4 : create the one more class in same package and it named as UserServiceImplementation into that package.
Go to src > java > in.mahesh.tasks > service > UserServiceImplementation and put the code below:
Java
package in.mahesh.tasks.service;
import in.mahesh.tasks.exception.UserException;
import in.mahesh.tasks.repository.UserRepository;
import in.mahesh.tasks.userSecurityConfig.JwtProvider;
import in.mahesh.tasks.usermodel.User;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserServiceImplementation implements UserService {
@Autowired
private UserRepository userRepository;
@Override
public User findUserProfileByJwt(String jwt) throws UserException {
String email= JwtProvider.getEmailFromJwtToken(jwt);
User user = userRepository.findByEmail(email);
if(user==null) {
throw new UserException("user not exist with email "+email);
}
return user;
}
public List<User> getAllUser() throws UserException{
return userRepository.findAll();
}
@Override
public User findUserByEmail(String email) throws UserException {
User user=userRepository.findByEmail(email);
return user;
}
@Override
public User findUserById(String userId) throws UserException {
// TODO Auto-generated method stub
java.util.Optional<User> opt = userRepository.findById(userId);
if(opt.isEmpty()) {
throw new UserException("user not found with id "+userId);
}
return opt.get();
}
@Override
public List<User> findAllUsers() {
// TODO Auto-generated method stub
return userRepository.findAll();
}
}
Step 5: Create the one more class in same package and it named as CustomerServiceImplementation into that package.
Go to src > java > in.mahesh.tasks > service > CustomerServiceImplementation and put the code below:
Java
package in.mahesh.tasks.service;
import in.mahesh.tasks.repository.UserRepository;
import in.mahesh.tasks.usermodel.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
public class CustomerServiceImplementation implements UserDetailsService {
@Autowired
private UserRepository userRepository;
public CustomerServiceImplementation(UserRepository userRepository) {
this.userRepository=userRepository;
}
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByEmail(username);
System.out.println(user);
if(user==null) {
throw new UsernameNotFoundException("User not found with this email"+username);
}
System.out.println("Loaded user: " + user.getEmail() + ", Role: " + user.getRole());
List<GrantedAuthority> authorities = new ArrayList<>();
return new org.springframework.security.core.userdetails.User(
user.getEmail(),
user.getPassword(),
authorities);
}
}
Step 6: Create the another package and it named as taskSecurityConfig and create the one class and it named as ApplicationConfig into that package.
Go to src > java > in.mahesh.tasks > taskSecurityConfig > ApplicationConfig and put the code below:
Java
package in.mahesh.tasks.userSecurityConfig;
import jakarta.servlet.http.HttpServletRequest;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.authentication.www.BasicAuthenticationFilter;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.CorsConfigurationSource;
import java.util.Arrays;
import java.util.Collections;
@Configuration
public class ApplicatonConfig {
@SuppressWarnings("deprecation")
@Bean
SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http.sessionManagement(management -> management.sessionCreationPolicy(SessionCreationPolicy.STATELESS))
.authorizeRequests(
authorize -> authorize.requestMatchers("/api/**")
.authenticated().anyRequest().permitAll())
.addFilterBefore(new JwtTokenValidator(), BasicAuthenticationFilter.class)
.csrf(csrf -> csrf.disable())
.cors(cors -> cors.configurationSource(corsConfigurationSource()));
//.httpBasic(Customizer.withDefaults())
//.formLogin(Customizer.withDefaults());
return http.build();
}
private CorsConfigurationSource corsConfigurationSource() {
return new CorsConfigurationSource() {
@Override
public CorsConfiguration getCorsConfiguration(HttpServletRequest request) {
CorsConfiguration ccfg = new CorsConfiguration();
ccfg.setAllowedOrigins(Arrays.asList("http://localhost:3000"));
ccfg.setAllowedMethods(Collections.singletonList("*"));
ccfg.setAllowCredentials(true);
ccfg.setAllowedHeaders(Collections.singletonList("*"));
ccfg.setExposedHeaders(Arrays.asList("Authorization"));
ccfg.setMaxAge(3600L);
return ccfg;
}
};
}
@Bean
PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
Step 7: Create the one more class in that same package and it named as JwtProvider into that package.
Go to src > java > in.mahesh.tasks > taskSecurityConfig > JwtProvider and put the code below:
Java
package in.mahesh.tasks.userSecurityConfig;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.security.Keys;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.GrantedAuthority;
import javax.crypto.SecretKey;
import java.util.Collection;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
public class JwtProvider {
static SecretKey key = Keys.hmacShaKeyFor(JwtConstant.SECRET_KEY.getBytes());
public static String generateToken(Authentication auth) {
Collection<? extends GrantedAuthority> authorities = auth.getAuthorities();
String roles = populateAuthorities(authorities);
@SuppressWarnings("deprecation")
String jwt = Jwts.builder()
.setIssuedAt(new Date())
.setExpiration(new Date(new Date().getTime()+86400000))
.claim("email", auth.getName())
.claim( "authorities",roles)
.signWith(key)
.compact();
System.out.println("Token for parsing in JwtProvider: " + jwt);
return jwt;
}
private static String populateAuthorities(Collection<? extends GrantedAuthority> authorities) {
Set<String> auths = new HashSet<>();
for(GrantedAuthority authority: authorities) {
auths.add(authority.getAuthority());
}
return String.join(",",auths);
}
@SuppressWarnings("deprecation")
public static String getEmailFromJwtToken(String jwt) {
jwt = jwt.substring(7); // Assuming "Bearer " is removed from the token
try {
//Claims claims=Jwts.parserBuilder().setSigningKey(key).build().parseClaimsJws(jwt).getBody();
Claims claims = Jwts.parser().setSigningKey(key).build().parseClaimsJws(jwt).getBody();
String email = String.valueOf(claims.get("email"));
System.out.println("Email extracted from JWT: " + claims);
return email;
} catch (Exception e) {
System.err.println("Error extracting email from JWT: " + e.getMessage());
e.printStackTrace();
return null;
}
}
}
Step 8: Create the one more class in that same package and it named as JwtTokenValidator into that package.
Go to src > java > in.mahesh.tasks > taskSecurityConfig > JwtTokenValidator and put the code below:
Java
package in.mahesh.tasks.userSecurityConfig;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.security.Keys;
import jakarta.servlet.FilterChain;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import org.springframework.security.authentication.BadCredentialsException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.AuthorityUtils;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.web.filter.OncePerRequestFilter;
import javax.crypto.SecretKey;
import java.io.IOException;
import java.util.List;
public class JwtTokenValidator extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
String jwt = request.getHeader(JwtConstant.JWT_HEADER);
System.out.println("JWT Token in JwtTokenValidator: " + jwt);
if (jwt != null && jwt.startsWith("Bearer ")) {
jwt = jwt.substring(7);
System.out.println("JWT Token in JwtTokenValidator: " + jwt);
try {
SecretKey key = Keys.hmacShaKeyFor(JwtConstant.SECRET_KEY.getBytes());
@SuppressWarnings("deprecation")
Claims claims = Jwts.parser().setSigningKey(key).build().parseClaimsJws(jwt).getBody();
System.out.print(claims);
String email = String.valueOf(claims.get("email"));
System.out.print(email);
String authorities = String.valueOf(claims.get("authorities"));
List<GrantedAuthority> auth = AuthorityUtils.commaSeparatedStringToAuthorityList(authorities);
Authentication authentication = new UsernamePasswordAuthenticationToken(email, null, auth);
SecurityContextHolder.getContext().setAuthentication(authentication);
} catch (Exception e) {
throw new BadCredentialsException("Invalid token", e);
}
}
filterChain.doFilter(request, response);
}
}
Step 9: Create the one more class in that same package and it named as JwtConstant into that package.
Go to src > java > in.mahesh.tasks > taskSecurityConfig > JwtConstant and put the code below:
Java
package in.mahesh.tasks.userSecurityConfig;
public class JwtConstant {
public static final String SECRET_KEY = "wpembytrwcvnryxksdbqwjebruyGHyudqgwveytrtrCSnwifoesarjbwe";
public static final String JWT_HEADER = "Authorization";
}
Step 10: Create the new package named as the controller and create the one class in that same package and it named as AuthController into that package.
Go to src > java > in.mahesh.tasks > controller > AuthController and put the code below:
Java
package in.mahesh.tasks.controller;
import in.mahesh.tasks.exception.UserException;
import in.mahesh.tasks.repository.UserRepository;
import in.mahesh.tasks.request.LoginRequest;
import in.mahesh.tasks.response.AuthResponse;
import in.mahesh.tasks.service.CustomerServiceImplementation;
import in.mahesh.tasks.service.UserService;
import in.mahesh.tasks.userSecurityConfig.JwtProvider;
import in.mahesh.tasks.usermodel.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.security.authentication.BadCredentialsException;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/auth")
public class AuthController {
@Autowired
private UserRepository userRepository;
@Autowired
private PasswordEncoder passwordEncoder;
@Autowired
private CustomerServiceImplementation customUserDetails;
@Autowired
private UserService userService;
@PostMapping("/signup")
public ResponseEntity<AuthResponse> createUserHandler(@RequestBody User user) throws UserException {
String email = user.getEmail();
String password = user.getPassword();
String fullName = user.getFullName();
String mobile = user.getMobile();
String role = user.getRole();
User isEmailExist = userRepository.findByEmail(email);
if (isEmailExist != null) {
throw new UserException("Email Is Already Used With Another Account");
}
User createdUser = new User();
createdUser.setEmail(email);
createdUser.setFullName(fullName);
createdUser.setMobile(mobile);
createdUser.setRole(role);
createdUser.setPassword(passwordEncoder.encode(password));
User savedUser = userRepository.save(createdUser);
userRepository.save(savedUser);
Authentication authentication = new UsernamePasswordAuthenticationToken(email,password);
SecurityContextHolder.getContext().setAuthentication(authentication);
String token = JwtProvider.generateToken(authentication);
AuthResponse authResponse = new AuthResponse();
authResponse.setJwt(token);
authResponse.setMessage("Register Success");
authResponse.setStatus(true);
return new ResponseEntity<AuthResponse>(authResponse, HttpStatus.OK);
}
@PostMapping("/signin")
public ResponseEntity<AuthResponse> signin(@RequestBody LoginRequest loginRequest) {
String username = loginRequest.getemail();
String password = loginRequest.getPassword();
System.out.println(username+"-------"+password);
Authentication authentication = authenticate(username,password);
SecurityContextHolder.getContext().setAuthentication(authentication);
String token = JwtProvider.generateToken(authentication);
AuthResponse authResponse = new AuthResponse();
authResponse.setMessage("Login success");
authResponse.setJwt(token);
// authResponse.setStatus(true);
return new ResponseEntity<>(authResponse,HttpStatus.OK);
}
private Authentication authenticate(String username, String password) {
System.out.println(username+"---++----"+password);
UserDetails userDetails = customUserDetails.loadUserByUsername(username);
System.out.println("Sig in in user details"+ userDetails);
if(userDetails == null) {
System.out.println("Sign in details - null" + userDetails);
throw new BadCredentialsException("Invalid username and password");
}
if(!passwordEncoder.matches(password,userDetails.getPassword())) {
System.out.println("Sign in userDetails - password mismatch"+userDetails);
throw new BadCredentialsException("Invalid password");
}
return new UsernamePasswordAuthenticationToken(userDetails,null,userDetails.getAuthorities());
}
}
Step 11: Create the one class in that same package and it named as UserController into that package.
Go to src > java > in.mahesh.tasks > controller > UserController and put the code below:
Java
package in.mahesh.tasks.controller;
import in.mahesh.tasks.exception.UserException;
import in.mahesh.tasks.service.UserService;
import in.mahesh.tasks.usermodel.User;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/profile")
public ResponseEntity<User> getUserProfile(@RequestHeader("Authorization") String jwt) throws UserException {
User user = userService.findUserProfileByJwt(jwt);
user.setPassword(null);
System.out.print(user);
return new ResponseEntity<>(user, HttpStatus.OK);
}
@GetMapping("/api/users/{userId}")
public ResponseEntity<User> findUserById(
@PathVariable String userId,
@RequestHeader("Authorization") String jwt) throws UserException {
User user = userService.findUserById(userId);
user.setPassword(null);
return new ResponseEntity<>(user, HttpStatus.ACCEPTED);
}
@GetMapping("/api/users")
public ResponseEntity<List<User>> findAllUsers(
@RequestHeader("Authorization") String jwt) {
List<User> users = userService.findAllUsers();
return new ResponseEntity<>(users, HttpStatus.ACCEPTED);
}
@GetMapping()
public ResponseEntity<?> getUsers(@RequestHeader("Authorization") String jwt) {
try {
List<User> users = userService.getAllUser();
System.out.print(users);
return new ResponseEntity<>(users, HttpStatus.OK);
} catch (Exception e) {
// Log the exception or handle it based on your application's requirements
return new ResponseEntity<>("Error retrieving users", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
Step 12: Create the one class in that same package and it named as HomeController into that package.
Go to src > java > in.mahesh.tasks > controller > HomeController and put the code below:
Java
package in.mahesh.tasks.controller;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import in.mahesh.tasks.response.ApiResponse;
@RestController
public class HomeController {
@GetMapping
public ResponseEntity<ApiResponse> homeController(){
ApiResponse res=new ApiResponse("Welcome To User Service Management",true);
return new ResponseEntity<ApiResponse>(res,HttpStatus.ACCEPTED);
}
@GetMapping("/users")
public ResponseEntity<ApiResponse> userHomeController(){
ApiResponse res=new ApiResponse("Welcome To User Service",true);
return new ResponseEntity<ApiResponse>(res,HttpStatus.ACCEPTED);
}
}
Step 13: Create the new package named as exception and create the one class in that same package and it named as UserException into that package.
Go to src > java > in.mahesh.tasks > exception > UserException and put the code below:
Java
package in.mahesh.tasks.exception;
public class UserException extends Exception {
public UserException(String message) {
super(message);
}
}
Java
package in.mahesh.tasks.request;
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
import org.springframework.data.mongodb.core.mapping.Document;
@AllArgsConstructor
@NoArgsConstructor
@Document
public class LoginRequest {
private String email;
private String password;
public String getemail() {
return email;
}
public void setUsername(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public void setemail(String username) {
// TODO Auto-generated method stub
this.setUsername(username);
}
}
Step 14: Create the new package named as response and create the one class in that same package and it named as ApiReponse into that package.
Go to src > java > in.mahesh.tasks > response > ApiReponse and put the code below:
Java
package in.mahesh.tasks.response;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
public class ApiResponse {
private String message;
private boolean status;
public ApiResponse(String string, boolean b) {
// TODO Auto-generated constructor stub
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public boolean isStatus() {
return status;
}
public void setStatus(boolean status) {
this.status = status;
}
}
Step 15: Create the new package named as response and create the one class in that same package and it named as AuthReponse into that package.
Go to src > java > in.mahesh.tasks > response > AuthReponse and put the code below:
Java
package in.mahesh.tasks.response;
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
@AllArgsConstructor
@NoArgsConstructor
public class AuthResponse {
private String jwt;
private String message;
private Boolean status;
public String getJwt() {
return jwt;
}
public void setJwt(String jwt) {
this.jwt = jwt;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Boolean getStatus() {
return status;
}
public void setStatus(Boolean status) {
this.status = status;
}
}
Step 16: Open the main class and in that main class enables the Hystrix using @EnableHystrix annotation.
Java
package in.mahesh.tasks;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
build.gradle
XML
plugins {
id 'java'
id 'org.springframework.boot' version '3.2.2'
id 'io.spring.dependency-management' version '1.1.4'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
java {
sourceCompatibility = '17'
}
configurations {
compileOnly {
extendsFrom annotationProcessor
}
}
repositories {
mavenCentral()
}
ext {
set('springCloudVersion', "2023.0.0")
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-data-mongodb'
compileOnly 'org.projectlombok:lombok'
developmentOnly 'org.springframework.boot:spring-boot-devtools'
annotationProcessor 'org.projectlombok:lombok'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
testImplementation 'org.springframework.security:spring-security-test'
implementation 'io.jsonwebtoken:jjwt-api:0.12.3'
implementation 'io.jsonwebtoken:jjwt-impl:0.12.3'
implementation 'io.jsonwebtoken:jjwt-jackson:0.12.3'
// https://mvnrepository.com/artifact/io.jsonwebtoken/jjwt-jackson
runtimeOnly 'io.jsonwebtoken:jjwt-jackson:0.12.3'
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
tasks.named('test') {
useJUnitPlatform()
}
Step 17: Once completed the project then run the application project as the spring boot project and once run the successful it can be started at port number 8081. Refer the below for better understanding.
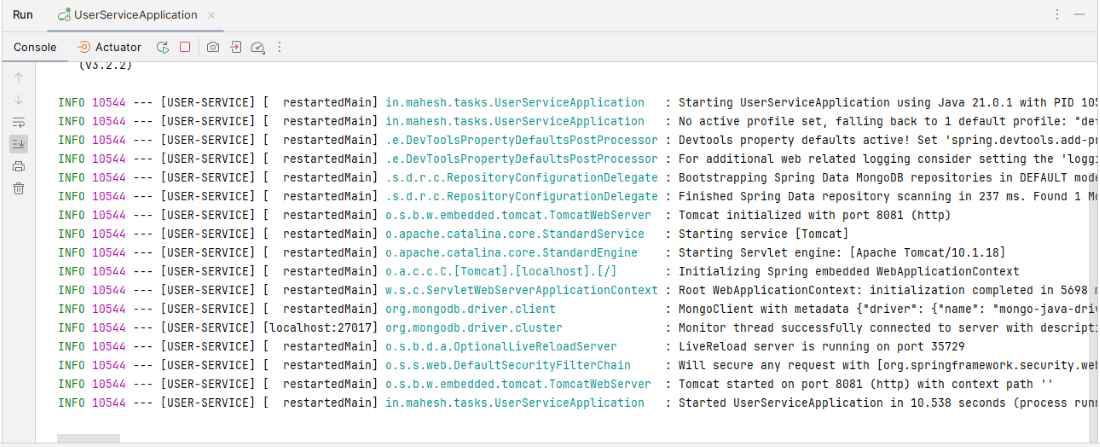
Register API Endpoint:
http://localhost:8081/auth/signup
Refer the Image:
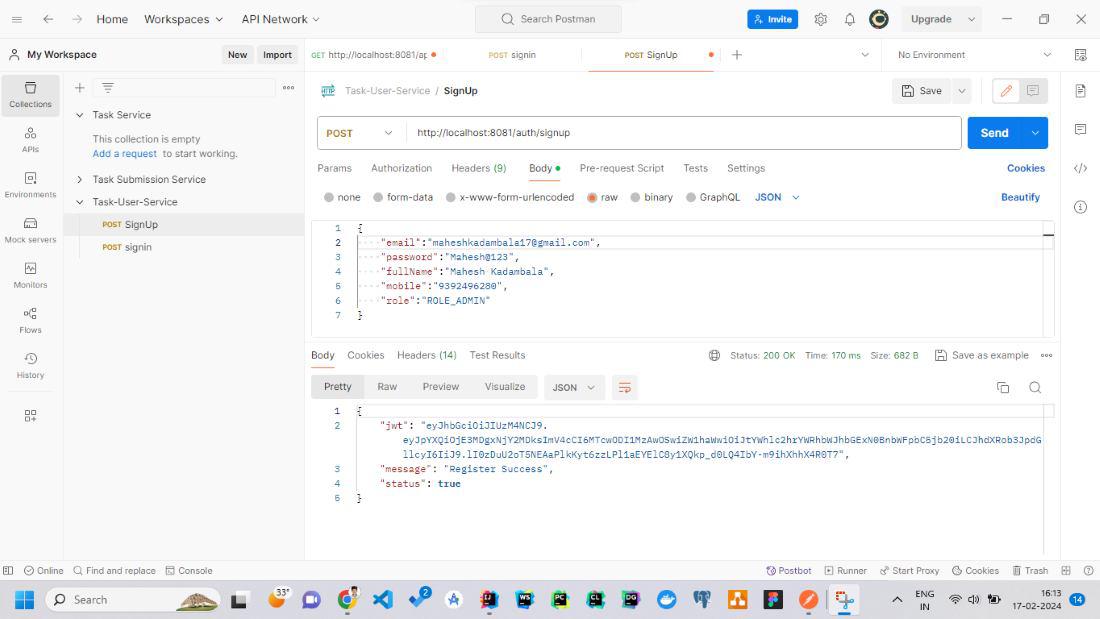
Login API Endpoint:
http:localhost:8081/auth/signin
JWT Token: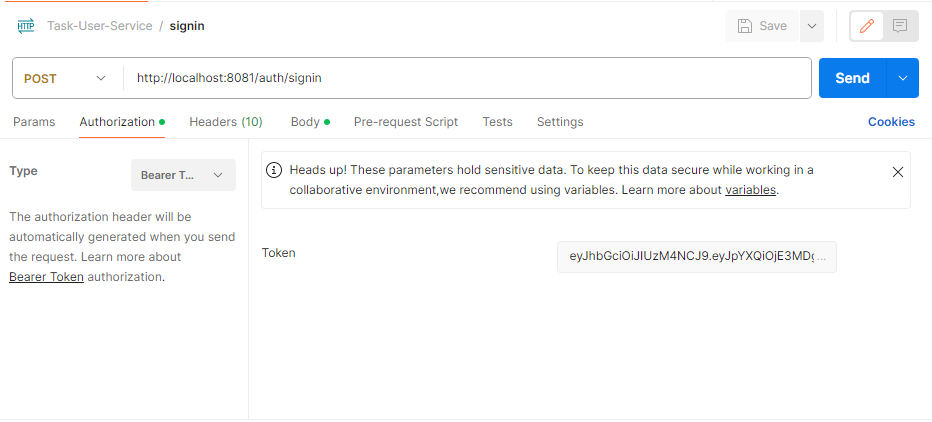
Body:
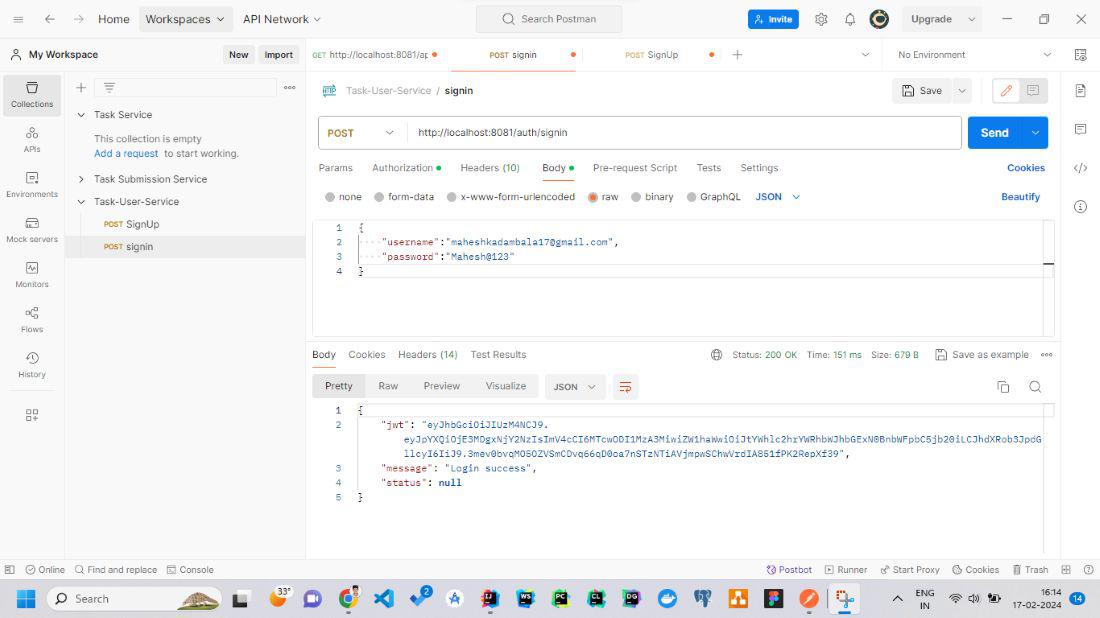
Profile API Endpoint:
http:localhost:8081/api/user/profile
Refer the image:
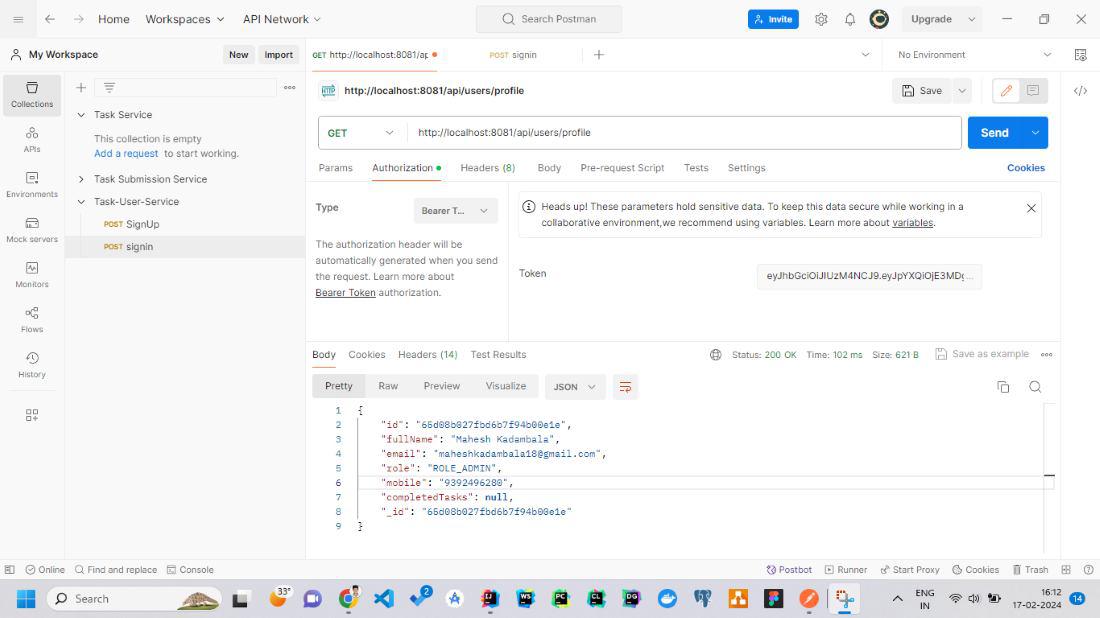
If you are following steps then successful completed the spring demonstration project of the User service Management spring project.
Share your thoughts in the comments
Please Login to comment...