Spring Cloud Kubernetes
Last Updated :
24 Mar, 2024
Modernizing applications for cloud-native settings is critical for microservices and containerization. In this article, we will look at how to deploy a Spring Boot application to a Kubernetes cluster with Spring Cloud Kubernetes.
Prerequisites:
- Apache Maven: It is necessary to install Maven to manage project dependencies and build artifacts.
- Docker: Docker enables the packaging of applications into lightweight containers.
- Minikube: For local development, Minikube provides a simple way to run a single-node Kubernetes cluster on the machine.
- kubectl (Kubernetes CLI): Install kubectl to interact with the Kubernetes cluster from the command line.
Deployment of Spring Boot application to a Kubernetes cluster with Spring Cloud Kubernetes
Below are the steps to deploy a simple Spring Boot application to a Kubernetes cluster with Spring Cloud Kubernetes.
Step 1: Develop a Spring Boot Application
Begin by creating a basic Spring Boot application. Develop a RESTful endpoint using a controller, such as the following HelloController:
Java
package com.example.springcloudk8sdemo;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
// Endpoint to return a hello message
@GetMapping("/hello")
public String hello() {
return "Hello from Spring Cloud Kubernetes";
}
}
Step 2: Containerize the Application
To deploy the Spring Boot application in a Kubernetes cluster, containerize it using Docker. Create a Dockerfile in the project directory:
# Use an official OpenJDK runtime as a base image
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /app
# Copy the JAR file into the container (add your own jar file name here)
COPY target/spring-cloud-k8s-demo-0.0.1-SNAPSHOT.jar app.jar
# Expose the port your application runs on
EXPOSE 8080
# Command to run your application
CMD ["java", "-jar", "app.jar"]
Build and push the Docker image to the container registry:
docker build -t your-docker-image:tag .
docker push your-docker-image:tag
After building successful, the console output is below:

Push Docker in container registry:

Docker Image Created Successfully:

Step 3: Set Up a Kubernetes Cluster
Start Minikube to create a local Kubernetes cluster for development by using the below command:
minikube start
Step 4: Deploy the Application to Kubernetes
Create Kubernetes deployment and service YAML files (deployment.yaml and service.yaml) if they are not auto generated by your IDE plugin:
deployment.yaml:
apiVersion: apps/v1
kind: Deployment
metadata:
name: spring-cloud-k8s-demo
spec:
replicas: 1
selector:
matchLabels:
app: spring-cloud-k8s-demo
template:
metadata:
labels:
app: spring-cloud-k8s-demo
spec:
containers:
- name: spring-cloud-k8s-demo
image: my-spring-app
ports:
- containerPort: 8080
service.yaml:
apiVersion: v1
kind: Service
metadata:
name: spring-cloud-k8s-demo
spec:
selector:
app: spring-cloud-k8s-demo
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: LoadBalancer
Apply the above files to cluster:
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
- deployment.yaml: This YAML file defines a Kubernetes Deployment for the Spring Cloud application. The apiVersion and kind specify that it’s a Deployment resource within the apps/v1 API version. The metadata section provides a name for the Deployment, here named “spring-cloud-k8s-demo“.
- service.yaml: This YAML file outlines a Kubernetes Service responsible for exposing the Spring Cloud application externally. It uses the v1 API version for the Service resource. The metadata section assigns a name, “spring-cloud-k8s-demo“ to the Service.

Step 5: Access the Application
Retrieve the external IP of the service and access the application using the /hello endpoint:
kubectl get services
curl http://<external-ip>/hello
Or simply open http://<external-ip>/hello in a new browser tab.
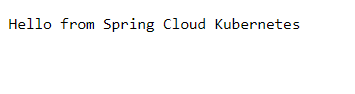
Step 6: Access the Application locally (optional)
Now if we are not using any cloud service provider, we will not be allocated with an external ip. In that case, we can also interact with the application locally using the internal IP of minikube. This is useful for testing and development purposes.
1. Retrieve NodePort
To access the application locally, we need to obtain the PORT number assigned to the service within the Kubernetes cluster. Run the following command to retrieve the details of the services:
kubectl get services
Look for the Spring Boot application service and note the value under the PORT(S) column. It will look like 80:xxxxx/TCP, where xxxxx is the NodePort.

2. Retrieve Minikube IP
Obtain the Minikube IP by running the following command:
minikube ip
3. Access the Application
With the Minikube IP and NodePort, we can access the Spring Boot application from our local machine using the following command:
curl http://<minikube-ip>:<node-port>/hello
Replace <minikube-ip> with the Minikube IP obtained in point 2 and <node-port> with the NodePort obtained in point 1.
This approach allows us to make HTTP requests to our service from our local machine, facilitating testing and development.
Share your thoughts in the comments
Please Login to comment...