Spring Cloud – Change Default Port of Eureka Server
Last Updated :
16 Jul, 2023
Service Discovery is one of the major things of a microservice-based architecture. Eureka is the Netflix Service Discovery Server and Client. The server can be configured and deployed to be highly functional, with each server copying the state of the registered services to the others. In this article, we are going to see how to change the default port of the Eureka Server with an example project. In brief, if you want to change your default port of Eureka Server which is 8761 to any other port (for example 5000 in this case) then you have to make the following changes in your client application.
eureka.client.service-url.defaultZone=http://localhost:5000/eureka/
Now let’s deep dive into this by implementing an example project. In this example, we are going to develop our Service Discovery (which is our Eureka Server) and one Microservice (which is our Eureka Client).
Example Project
Developing Service Discovery or Eureka Server
Step 1: Create a New Spring Boot Project in Spring Initializr
To create a new Spring Boot project, please refer to How to Create a Spring Boot Project in Spring Initializr and Run it in IntelliJ IDEA. For this project choose the following things
- Project: Maven
- Language: Java
- Packaging: Jar
- Java: 17
Please choose the following dependencies while creating the project.
Eureka Server: Generate the project and run it in IntelliJ IDEA by referring to the above article. Below is the complete pom.xml file. Please cross-verify if you have missed some dependencies.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< parent >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-parent</ artifactId >
< version >3.0.6</ version >
< relativePath />
</ parent >
< groupId >com.gfg.discovery-service</ groupId >
< artifactId >discovery-service</ artifactId >
< version >0.0.1-SNAPSHOT</ version >
< name >Discovery Service</ name >
< description >Demo project for Discovery Service</ description >
< properties >
< java.version >17</ java.version >
< spring-cloud.version >2022.0.2</ spring-cloud.version >
</ properties >
< dependencies >
< dependency >
< groupId >org.springframework.cloud</ groupId >
< artifactId >spring-cloud-starter-netflix-eureka-server</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-test</ artifactId >
< scope >test</ scope >
</ dependency >
</ dependencies >
< dependencyManagement >
< dependencies >
< dependency >
< groupId >org.springframework.cloud</ groupId >
< artifactId >spring-cloud-dependencies</ artifactId >
< version >${spring-cloud.version}</ version >
< type >pom</ type >
< scope >import</ scope >
</ dependency >
</ dependencies >
</ dependencyManagement >
< build >
< plugins >
< plugin >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-maven-plugin</ artifactId >
</ plugin >
</ plugins >
</ build >
< repositories >
< repository >
< id >netflix-candidates</ id >
< name >Netflix Candidates</ name >
< snapshots >
< enabled >false</ enabled >
</ snapshots >
</ repository >
</ repositories >
</ project >
|
Step 2: Modify DiscoveryServiceApplication Class
Go to the discovery-service > src > main > java > DiscoveryServiceApplication and annotate it with @EnableEurekaServer annotation. Below is the complete code for DiscoveryServiceApplication Class.
Java
package com.gfg.discoveryservice;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class DiscoveryServiceApplication {
public static void main(String[] args) {
SpringApplication.run(DiscoveryServiceApplication. class , args);
}
}
|
Step 3: Make Changes in Your application.properties File
Now make the following changes in your application.properties file.
# Recommend Port Number to Use for Eureka Server
# But you can use any, here we are using 5000 instead of 8761
server.port=5000
spring.application.name=discovery-service
eureka.client.fetchRegistry=false
eureka.client.register-with-eureka=false
Now run your discovery-service. If everything goes well then you may see the following screen in your console. Please refer to the below image.
Now hit the following URL in your browser
http://localhost:5000/
And you can see the Spring Eureka dashboard like below.
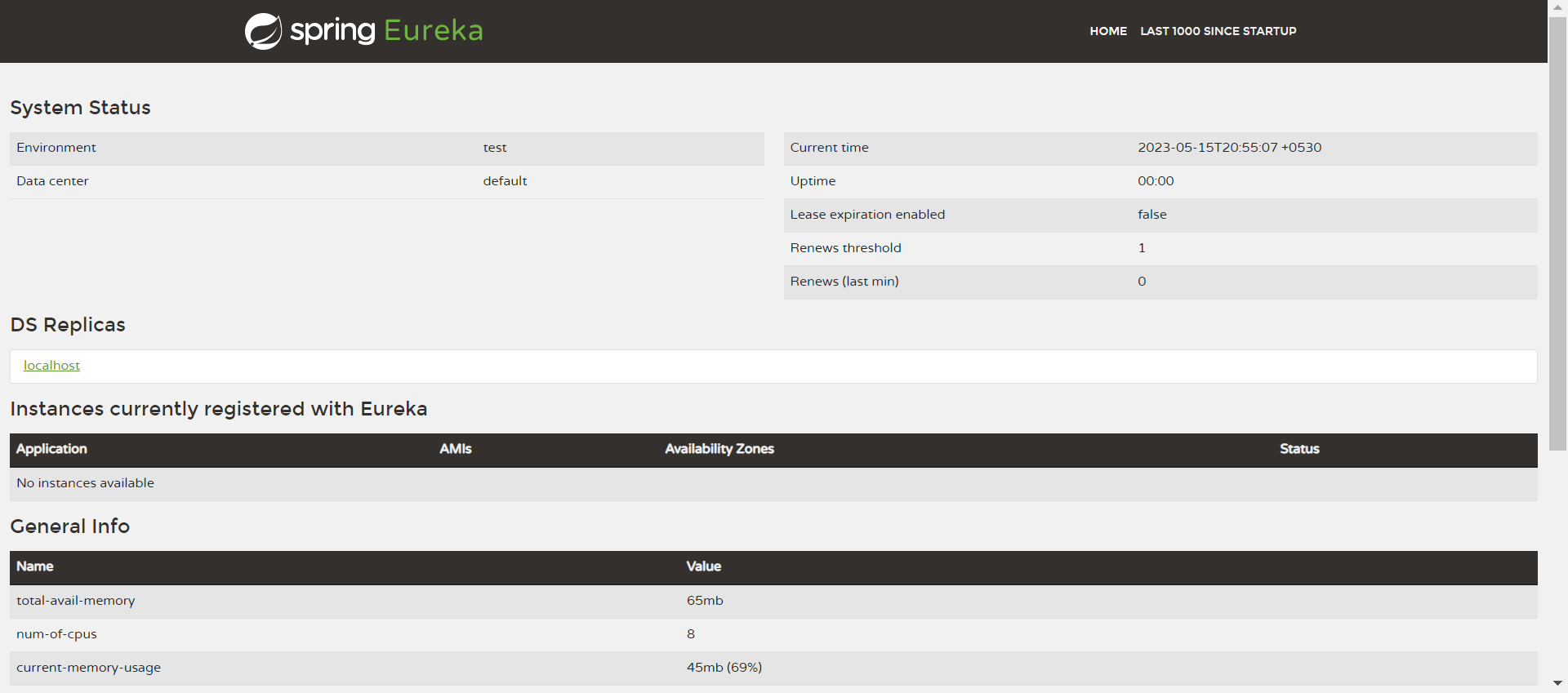
Developing Microservice or Eureka Client
Step-by-Step Implementation
Step 1: Create a New Spring Boot Project in Spring Initializr
To create a new Spring Boot project, please refer to How to Create a Spring Boot Project in Spring Initializr and Run it in IntelliJ IDEA. For this project choose the following things
- Project: Maven
- Language: Java
- Packaging: Jar
- Java: 17
Please choose the following dependencies while creating the project.
- Eureka Discovery Client
- Spring Web
Generate the project and run it in IntelliJ IDEA by referring to the above article. Please refer to the below image.
Below is the complete pom.xml file. Please cross-verify if you have missed some dependencies.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< modelVersion >4.0.0</ modelVersion >
< parent >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-parent</ artifactId >
< version >3.0.6</ version >
< relativePath />
</ parent >
< groupId >com.gfg.demomicroservice</ groupId >
< artifactId >demomicroservice</ artifactId >
< version >0.0.1-SNAPSHOT</ version >
< name >Demo Microservice</ name >
< description >Demo project for Spring Boot</ description >
< properties >
< java.version >17</ java.version >
< spring-cloud.version >2022.0.2</ spring-cloud.version >
</ properties >
< dependencies >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-web</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.cloud</ groupId >
< artifactId >spring-cloud-starter-netflix-eureka-client</ artifactId >
</ dependency >
< dependency >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-starter-test</ artifactId >
< scope >test</ scope >
</ dependency >
</ dependencies >
< dependencyManagement >
< dependencies >
< dependency >
< groupId >org.springframework.cloud</ groupId >
< artifactId >spring-cloud-dependencies</ artifactId >
< version >${spring-cloud.version}</ version >
< type >pom</ type >
< scope >import</ scope >
</ dependency >
</ dependencies >
</ dependencyManagement >
< build >
< plugins >
< plugin >
< groupId >org.springframework.boot</ groupId >
< artifactId >spring-boot-maven-plugin</ artifactId >
</ plugin >
</ plugins >
</ build >
< repositories >
< repository >
< id >netflix-candidates</ id >
< name >Netflix Candidates</ name >
< snapshots >
< enabled >false</ enabled >
</ snapshots >
</ repository >
</ repositories >
</ project >
|
Step 2: Make Changes in Your application.properties File
Now make the following changes in your application.properties file.
server.port=9090
spring.application.name=demo-service
eureka.client.service-url.defaultZone=http://localhost:5000/eureka/
Note: So you have to add the above line if you are changing your default port of the Eureka server. If we don’t do it it will by default try to look for Eureka in the 8761 port number and that will result in an exception and registration failure. For more explanation refer to this article How Eureka Server and Client Communicate with Each Other
Now run your microservice that we have developed above. And you can see your DEMO-SERVICE has been registered with your Eureka Server. Please refer to the below image.
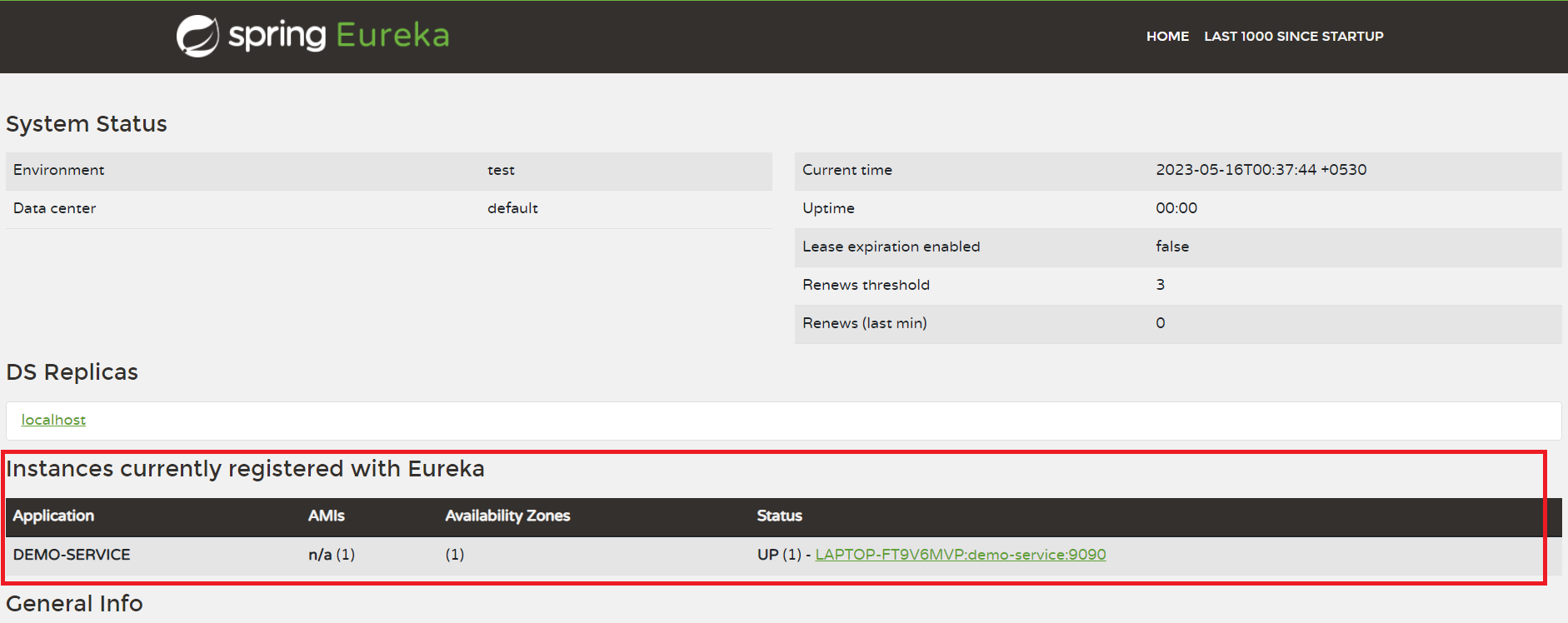
Share your thoughts in the comments
Please Login to comment...