Slicing with Negative Numbers in Python
Last Updated :
30 Jan, 2024
Slicing is an essential concept in Python, it allows programmers to access parts of sequences such as strings, lists, and tuples. In this article, we will learn how to perform slicing with negative indexing in Python.
Indexing in Python
In the world of programming, indexing starts at 0, and Python also follows 0-indexing what makes Python different is that Python also follows negative indexing which starts from -1. -1 denotes the last index, -2, denotes the second last index, -3 denotes the third last index, and so on.
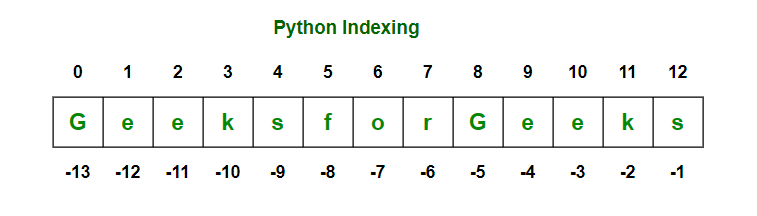
Slicing with Negative Numbers in Python
In Python, we can perform slicing on iterable objects in two ways first is using list slicing and the second is using the slice() function. Python also allows programmers to slice the iterable objects using negative indexing. Let’s first see the syntax of both methods.
- Using Slicing ( : )
- Using slice() Function
Slicing with Negative Numbers in Python Using Colon ‘:’ Operator
We can perform slicing in Python using the colon ‘:’ operator. It accepts three parameters which are start, end, and step. Start and end can be any valid index whether it is negative or positive. When we write step as -1 it will reverse the slice.
Syntax:
sequence[Start : End : Step]
Parameters:
- Start: It is the starting point of the slice or substring.
- End: It is the ending point of the slice or substring but it does not include the last index.
- Step: It is number of steps it takes.
In this example, we use the variable “text” to demonstrate string slicing. Slicing from the left starts from the beginning, and for the middle part, we specify indices. The right part is sliced similarly. The slices are stored in variables “left,” “middle,” and “right” respectively.
Python3
text = "GeeksforGeeks"
left = text[: - 8 ]
middle = text[ - 8 : - 5 ]
right = text[ - 5 :]
print (left)
print (middle)
print (right)
|
Slicing with Negative Numbers in Python Using slice() Function
This slice() function is used same as colon ‘:’ operator. In slice() function we passed the start, stop, and step arguments without using colon.
Syntax:Â slice(start, stop, step)
Parameters:Â
- start:Â Starting index where the slicing of object starts.
- stop:Â Ending index where the slicing of object stops.
- step:Â It is an optional argument that determines the increment between each index for slicing.
Return Type:Â Returns a sliced object containing elements in the given range only.Â
Note:Â If only one parameter is passed, then the start and step are considered to be None.
In this example, a string is stored in the variable “text,” and the `slice()` function is employed for string slicing. For the left part, an empty start indicates slicing from the beginning up to the index “-8,” stored in the variable “left.” The middle part is sliced by specifying indices -8 and -5. Similarly, the right part is obtained using the `slice()` function.
Python3
text = "GeeksforGeeks"
left = text[ slice ( - 8 )]
middle = text[ slice ( - 8 , - 5 )]
right = text[ slice ( - 5 , None )]
print (left)
print (middle)
print (right)
|
More Examples
Slicing List with Negative Numbers in Python
In this example, the code creates a list named “items” and utilizes slicing to extract specific items. By recognizing that the last four items represent vegetables, they are sliced and stored in the variable “vegies.” The same approach is applied to extract fruits, but this time the `slice()` function is used for the slicing operation.
Python3
items = [ "pen" , "pencil" , "eraser" ,
"apple" , "guava" , "ginger" ,
"Potato" , "carret" , "Chilli" ]
vegies = items[ - 4 :]
print (vegies)
fruits = items[ slice ( - 6 , - 4 )]
print (fruits)
|
Output
['ginger', 'Potato', 'carret', 'Chilli']
['apple', 'guava']
Reverse List with the Negative Step
In this example, we have reversed the list using normal slicing and slice() function. Both of methods are very similar we have to just pass the -1 in the third argument which is “Step” and passing nothing in normal slicing and “None” in the slice() function.
Python3
nums = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 ]
reverse_nums = nums[:: - 1 ]
print (reverse_nums)
reverse_nums2 = nums[ slice ( None , None , - 1 )]
print (reverse_nums2)
|
Output
[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
Slicing Alternates in the List with Negative Step
In this example, we sliced the alternate items from list by passing “-2” in the step argument. We can see in the output that the printed slice is alternate numbers are printed by skipping one number.
Python3
nums = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 ]
alt_nums = nums[:: - 2 ]
print (alt_nums)
alt_nums2 = nums[ slice ( None , None , - 2 )]
print (alt_nums2)
|
Output
[10, 8, 6, 4, 2]
[10, 8, 6, 4, 2]
Slicing Tuples with Negative Numbers in Python
In this example, below code shows different ways to cut a tuple. In the first two lines, it takes the last three items and items from the fifth to third from the end of the tuple. The last two lines reverse the tuple and pick every second item from the end using a specific method, creating new slices.
Python3
my_tuple = ( 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 )
slice = my_tuple[ - 3 :]
print ( slice )
slice = my_tuple[ - 5 : - 2 ]
print ( slice )
reversed_tuple = my_tuple[:: - 1 ]
print (reversed_tuple)
slice = my_tuple[:: - 2 ]
print ( slice )
|
Output
(7, 8, 9)
(5, 6, 7)
(9, 8, 7, 6, 5, 4, 3, 2, 1)
(9, 7, 5, 3, 1)
Conclusion
Slicing with negative numbers in Python streamlines sequence manipulation, offering an efficient and concise method for accessing elements from the end. This enhances code readability and is especially beneficial for sequences of varying lengths. By leveraging negative indexing, developers can efficiently extract data portions without explicitly determining sequence length, ultimately boosting flexibility and expressiveness in Python code.
Share your thoughts in the comments
Please Login to comment...