Simple BMI calculator using Python
Last Updated :
02 Feb, 2024
In this article, we will guide you through the process of building a straightforward Body Mass Index (BMI) calculator using Python. To enhance user interaction, we will implement a simple graphical user interface (GUI) form. This form will allow users to input their weight and height, and with the help of the Django Framework, the BMI will be automatically calculated.
What is BMI Calculator ?
BMI stands for Body Mass Index, and it is a numerical value of a person’s weight in relation to their height. It is a widely used screening tool to categorize individuals into different weight status categories, such as underweight, normal weight, overweight, and obesity.
Simple BMI calculator using Python
Below are the step by step procedure by which we can create a simple BMI calculator using Python:
Installation
Before starting, install the following modules
Starting the Project Folder
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp home
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
]
File Structure
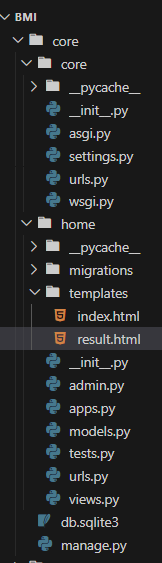
Setting Necessary Files
views.py : In this Django application calculates Body Mass Index (BMI) using user-submitted weight and height. The `calculate_bmi` function computes BMI and determines its interpretation (‘Underweight,’ ‘Healthy Weight,’ or ‘Overweight’). The result is displayed in ‘result.html.’ If not a ‘POST’ request, it renders the input form in ‘index.html.’ The `get_bmi_interpretation` function categorizes BMI values.
Python3
from django.shortcuts import render
def calculate_bmi(request):
if request.method = = 'POST' :
weight = float (request.POST[ 'weight' ])
height = float (request.POST[ 'height' ])
bmi = round (weight / (height * * 2 ))
interpretation = get_bmi_interpretation(bmi)
return render(request, 'result.html' , { 'bmi' : bmi, 'interpretation' : interpretation})
return render(request, 'index.html' )
def get_bmi_interpretation(bmi):
if bmi < 18.5 :
return 'Underweight'
elif 18.5 < = bmi < 25 :
return 'Healthy Weight'
else :
return 'Overweight'
|
project/urls.py : below, code defines Django URL patterns, including an admin path and routing any other path to the ‘home.urls’ module.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' home.urls')),
]
|
app.urls.py : below, code defines a single URL pattern that maps the root path to the ‘calculate_bmi’ view.
Python3
from django.urls import path
from .views import calculate_bmi
urlpatterns = [
path(' ', calculate_bmi, name=' calculate_bmi'),
]
|
Creating GUI
index.html : This HTML code creates a styled BMI Calculator webpage with a form for entering weight and height. It uses a card layout, specifies fonts, and includes CSRF protection for the ‘calculate_bmi’ view. The design is clean, responsive, and user-friendly.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >BMI Calculator</ title >
< style >
body {
font-family: 'Arial', sans-serif;
background-color: #f8f9fa;
}
.container {
max-width: 400px;
margin: 50px auto;
}
.card {
border: 1px solid #ced4da;
border-radius: 5px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
background-color: #fff;
}
.card-header {
background-color: #ced4da;
text-align: center;
font-family: 'Times New Roman', Times, serif;
padding: 15px;
border-top-left-radius: 10px;
border-top-right-radius: 10px;
}
.card-body {
padding: 20px;
}
label {
font-family: 'Times New Roman', Times, serif;
margin-bottom: 5px;
display: block;
font-size: 14px;
}
.form-control {
width: 100%;
padding: 8px;
margin-bottom: 15px;
border: 1px solid #ced4da;
border-radius: 5px;
box-sizing: border-box;
}
button {
width: 100%;
padding: 10px;
background-color: rgb(156, 220, 156);
font-weight: bold;
border: none;
font-size: 17px;
font-family: 'Times New Roman', Times, serif;
border-radius: 5px;
cursor: pointer;
}
</ style >
</ head >
< body >
< div class = "container" >
< div class = "card" >
< div class = "card-header" >
< h2 >BMI Calculator</ h2 >
</ div >
< div class = "card-body" >
< form method = "post" action = "{% url 'calculate_bmi' %}" >
{% csrf_token %}
< label for = "weight" >Weight (kg): </ label >
< input type = "number" class = "form-control" name = "weight" required step = "0.01" >
< label for = "height" >Height (m): </ label >
< input type = "number" class = "form-control" name = "height" required step = "0.01" >
< button type = "submit" >Calculate BMI</ button >
</ form >
</ div >
</ div >
</ div >
</ body >
</ html >
|
result.html : This HTML code creates a visually appealing BMI Result webpage with a centered card layout. It displays the calculated BMI and its interpretation with a clean design, incorporating a green color scheme. The page includes an image for visual appeal, enhancing the overall presentation of the BMI results.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >BMI Result</ title >
< style >
body {
font-family: 'Arial', sans-serif;
background-color: #f8f9fa;
text-align: center;
padding: 50px;
}
.card {
max-width: 300px;
margin: 0 auto;
background-color: #fff;
border: 1px solid #ced4da;
border-radius: 5px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
padding: 20px;
}
h2 {
color: rgb(94, 174, 48);
margin-bottom: 20px;
font-family: 'Times New Roman', Times, serif;
}
img {
max-width: 50%;
height: 50%;
border-radius: 5px;
margin-top: 20px;
}
</ style >
</ head >
< body >
< div class = "card" >
< h2 > Your BMI Result</ h2 >
< p > < strong >Your BMI is: </ strong > {{ bmi }}</ p >
< p > < strong > Interpretation: </ strong >{{ interpretation }}</ p >
</ div >
</ body >
</ html >
|
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output:
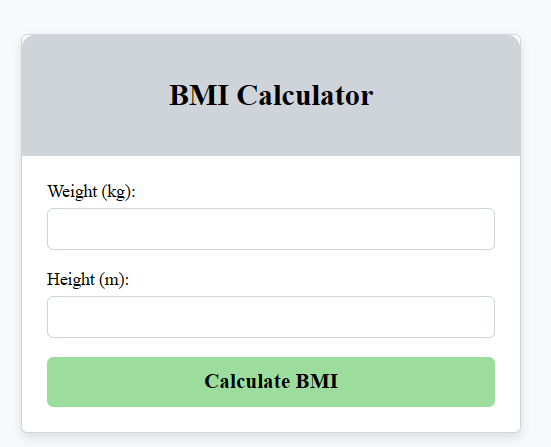
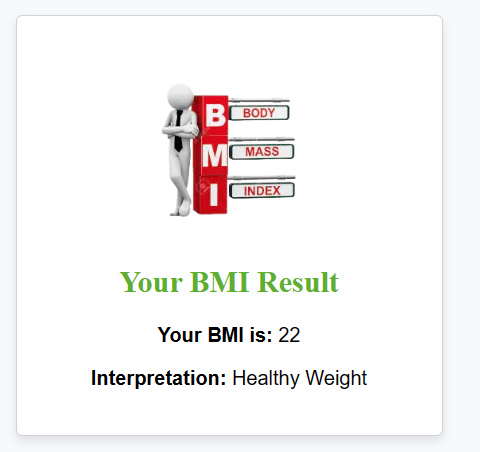
Share your thoughts in the comments
Please Login to comment...