Create a Simple Interest Calculator using HTML CSS and JavaScript
Last Updated :
21 Feb, 2024
Simple Interest is the term used to describe the rate at which money is borrowed or lent. Simple Interest Calculator serves as a practical tool for computing interest on loans or savings without compounding. It allows you to determine the simple interest on the principal amount, offering flexibility in calculating daily, monthly, or yearly interest. In this article, we will create a simple yet effective Simple Interest calculator using HTML, CSS, and JavaScript.
Preview
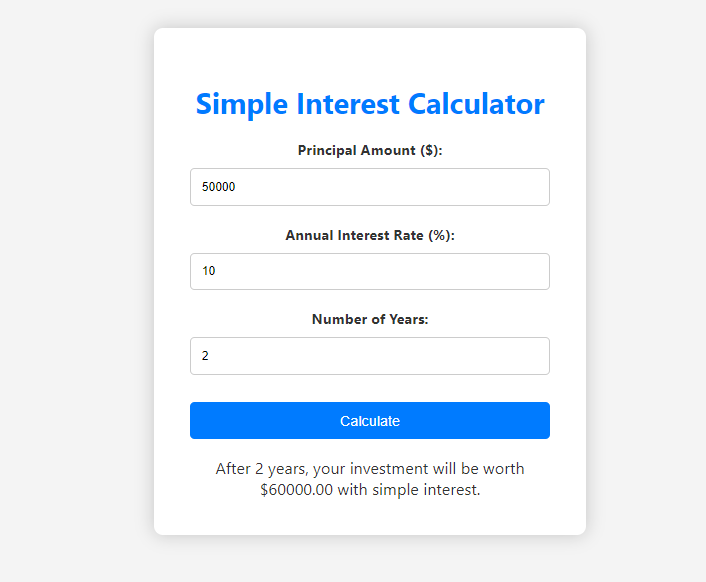
Approach
- Create the HTML structure for the calculator with labeled input fields for initial Principal Amount, interest rate, investment years, and a calculate button.
- Apply CSS styles to enhance the visual appeal of the calculator, focusing on a clean layout, readable fonts, etc.
- Initialize the JavaScript by adding an event listener to ensure the DOM is fully loaded before interacting with elements.
- Use JavaScript to retrieve user input values from the input fields for initial investment, interest rate, and investment years.
- Implement the Simple Interset logic in JavaScript to compute the future value of the investment based on the provided inputs.
- Update the HTML content dynamically with the calculated result, informing the user about the future value of their investment.
Example: In this example, we will design a Simple Interest calculator using HTML CSS, and JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >The Investment Calculator</ title >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< div class = "container" >
< h1 >Investment Calculator</ h1 >
< div class = "input-container" >
< label for = "initial-investment" >
Initial Investment ($):
</ label >
< input type = "number"
id = "initial-investment"
placeholder = "Enter initial investment" >
</ div >
< div class = "input-container" >
< label for = "interest-rate" >
Annual Interest Rate (%):
</ label >
< input type = "number"
id = "interest-rate"
placeholder = "Enter annual interest rate" >
</ div >
< div class = "input-container" >
< label for = "investment-years" >
Number of Years:
</ label >
< input type = "number"
id = "investment-years"
placeholder = "Enter number of years" >
</ div >
< button id = "calculate-button" >
Calculate
</ button >
< div id = "result"
class = "result-container" >
</ div >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : 'Segoe UI' , Tahoma , Geneva, Verdana , sans-serif ;
background-color : #f4f4f4 ;
margin : 0 ;
padding : 0 ;
display : flex;
align-items: center ;
justify- content : center ;
height : 100 vh;
}
.container {
background-color : #fff ;
border-radius: 10px ;
box-shadow: 0 0 20px rgba( 0 , 0 , 0 , 0.2 );
padding : 40px ;
width : 400px ;
display : flex;
flex- direction : column;
text-align : center ;
}
h 1 {
color : #007bff ;
margin-bottom : 20px ;
}
.input-container {
margin-bottom : 20px ;
}
label {
display : block ;
margin-bottom : 5px ;
color : #333 ;
font-weight : bold ;
}
input[type= "number" ] {
width : 100% ;
padding : 12px ;
border : 1px solid #ccc ;
border-radius: 5px ;
box-sizing: border-box;
margin-top : 5px ;
}
button {
background-color : #007bff ;
color : #fff ;
border : none ;
padding : 12px 20px ;
cursor : pointer ;
border-radius: 5px ;
font-size : 16px ;
margin-top : 10px ;
}
button:hover {
background-color : #0056b3 ;
}
.result-container {
text-align : center ;
margin-top : 20px ;
font-size : 18px ;
color : #333 ;
}
|
Javascript
document.addEventListener( "DOMContentLoaded" , function () {
const button =
document.getElementById( "calculate-button" );
const container =
document.getElementById( "result" );
button.addEventListener( "click" , function () {
const initialInvestment =
parseFloat(document.getElementById( "initial-investment" ).value);
const interestRate =
parseFloat(document.getElementById( "interest-rate" ).value) / 100;
const investmentYears =
parseInt(document.getElementById( "investment-years" ).value);
let totalAmount = initialInvestment;
for (let i = 1; i <= investmentYears; i++) {
totalAmount *= (1 + interestRate);
}
container.innerHTML =
`After ${investmentYears} years, your investment`+
` will be worth $${totalAmount.toFixed(2)}.`;
});
});
|
Output:
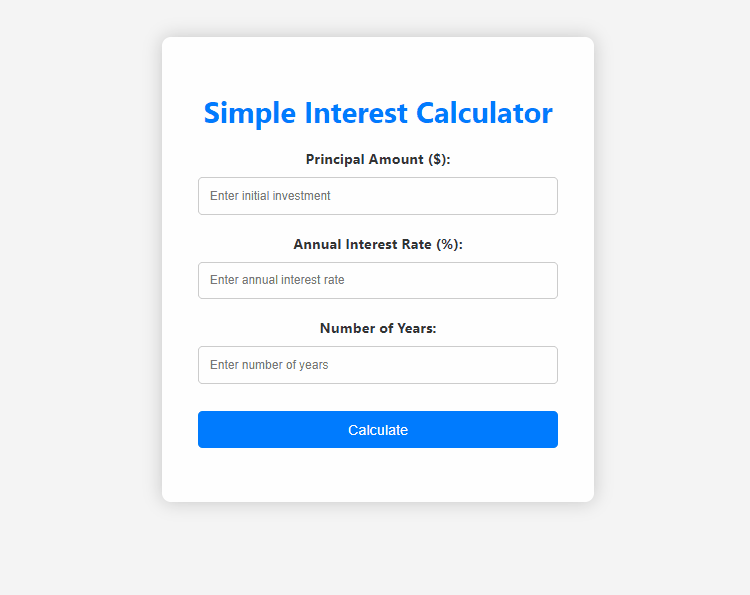
Share your thoughts in the comments
Please Login to comment...