Shortest Path Algorithm Tutorial with Problems
Last Updated :
23 Nov, 2023
In this article, we are going to cover all the commonly used shortest path algorithm while studying Data Structures and Algorithm. These algorithms have various pros and cons over each other depending on the use case of the problem. Also we will analyze these algorithms based on the time and space complexities which are frequently asked in coding interviews.
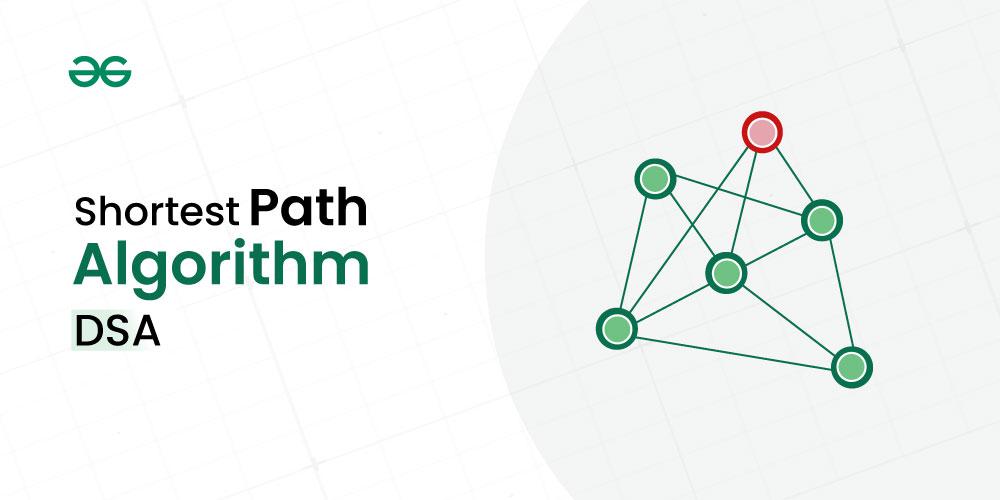
What are the Shortest Path Algorithms?
The shortest path algorithms are the ones that focuses on calculating the minimum travelling cost from source node to destination node of a graph in optimal time and space complexities.
Types of Shortest Path Algorithms:
As we know there are various types of graphs (weighted, unweighted, negative, cyclic, etc.) therefore having a single algorithm that handles all of them efficiently is not possible. In order to tackle different problems, we have different shortest-path algorithms, which can be categorised into two categories:
Single Source Shortest Path Algorithms:
In this algorithm we determine the shortest path of all the nodes in the graph with respect to a single node i.e. we have only one source node in this algorithms.
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Multi-Source BFS
- Dijkstra’s algorithm
- Bellman-Ford algorithm
- Topological Sort
- A* search algorithm
All Pair Shortest Path Algorithms:
Contrary to the single source shortest path algorithm, in this algorithm we determine the shortest path between every possible pair of nodes.
- Floyd-Warshall algorithm
- Johnson’s algorithm
Let us discuss these algorithms one by one.
1. Shortest Path Algorithm using Depth-First Search(DFS):
DFS algorithm recursively explores the adjacent nodes untill it reaches to the depth where no more valid recursive calls are possible.
For DFS to be used as a shortest path algorithm, the graph needs to be acyclic i.e. a TREE, the reason it won’t work for cyclic graphs is because due to cycles, the destination node can have multiple paths from the source node and dfs will not be able to choose the best path.
If there does not exist a path between source node and destination node then we can store -1 as the shortest path between those nodes.
Algorithm:
- Distance of source node to source node is initialized to 0.
- Start the DFS from the source node.
- As we go to a neighbouring nodes we set it’s distance from source node = edge weight + distance of parent node.
- DFS ends when all the leaf nodes are visited.
Complexity Analysis:
- Time Complexity: O(N) where N is the number of nodes in the tree.
- Auxiliary Space: O(N) call stacks in case of skewed tree.
BFS is a great shortest path algorithm for all graphs, the path found by breadth first search to any node is the shortest path to that node, i.e the path that contains the smallest number of edges in unweighted graphs.
Reason: This works due to the fact that unlike DFS, BFS visits all the neighbouring nodes before exploring a single node to its neighbour. As a result, all nodes with distance ‘d‘ from the source are visited after all the nodes with distance smaller than d. In simple terms all the nodes with distance 1 from the node will be visited first, then distance 2 nodes, then 3 and so on.
Algorithm:
- Use a queue to store a pair of values {node, distance}
- Distance of source node to source node is initialized to 0 i.e. push {src, 0} to the queue
- While queue is not empty do the following:
- Get the {node, distance} value of top of the queue
- Pop the top element of the queue
- Store the distance for the node as the shortest distance for that node from the source
- For all non visited neighbours of the current node, push {neighbour, distance+1} into the queue and mark it visited
Complexity Analysis:
- Time Complexity: O(N) where N is the number of nodes in the graph
- Auxiliary Space: O(N) for storing distance for each node.
Like BFS, it is also applicable to unweighted graph. To perform a Multi-source search, we basically start BFS from multiple nodes at the same time. When all the BFS meet, we’ve found the shortest path.
Algorithm:
- Push all source nodes in a Queue with distance 0.
- While the queue is not empty:
- Get the {node, distance} value of top of the queue
- Pop the top element of the queue
- Store the distance for the node as the shortest distance for that node from the source
- For all non visited neighbours of the current node, push {neighbour, distance+1} into the queue and mark it visited
Complexity Analysis:
- Time Complexity: O(N) where N is the number of nodes in the tree.
- Auxiliary Space: O(N) call stacks in case of skewed tree.
Dijkstra’s algorithm finds the shortest path from a source node to all other nodes in a weighted graph by iteratively selecting the node with the smallest tentative distance and updating the distances to its neighbours. It ensures the shortest path is progressively discovered and is based on the principle of greedy optimization.
Algorithm:
- Create a Set sptSet (shortest path tree set) that keeps track of vertices included in the shortest path tree, i.e., whose minimum distance from the source is calculated and finalized. Initially, this set is empty.
- Assign a distance value to all vertices in the input graph. Initialize all distance values as INFINITE. Assign the distance value as 0 for the source vertex so that it is picked first.
- While Set doesn’t include all vertices
- Pick a vertex ‘u‘ that is not there in Set and has a minimum distance value.
- Include ‘u‘ to sptSet.
- Then update the distance value of all adjacent vertices of u.
- To update the distance values, iterate through all adjacent vertices.
- For every adjacent vertex v, if the sum of the distance value of u (from source) and weight of edge u-v, is less than the distance value of v, then update the distance value of v.
Complexity Analysis:
- Time Complexity: O(E*log(V)), where E is the number of edges and V is the number of vertices in the graph:
- Auxiliary Space: O(V)
Bellman-Ford is a single source shortest path algorithm that determines the shortest path between a given source vertex and every other vertex in a graph. This algorithm can be used on both weighted and unweighted graphs.
A Bellman-Ford algorithm is also guaranteed to find the shortest path in a graph, similar to Dijkstra’s algorithm. Although Bellman-Ford is slower than Dijkstra’s algorithm, it is capable of handling graphs with negative edge weights, which makes it more versatile. The shortest path cannot be found if there exists a negative cycle in the graph. If we continue to go around the negative cycle an infinite number of times, then the cost of the path will continue to decrease (even though the length of the path is increasing). As a result, Bellman-Ford is also capable of detecting negative cycles, which is an important feature.
Algorithm:
- Initialize distance array dist[] for each vertex ‘v‘ as dist[v] = INFINITY.
- Assume any vertex (let’s say ‘0’) as source and assign dist = 0.
- Relax all the edges(u,v,weight) N-1 times as per the below condition:
- dist[v] = minimum(dist[v], distance[u] + weight)
Complexity Analysis:
- Time Complexity: O(V*E), where V is the number of vertices and E is the number of edges.
- Auxiliary Space: O(V)
Topological sorting can be used as a single source shortest path algorithm for Directed Acyclic Graphs (DAGs). This works because DAGs guarantees the non-existence of negative cycle.
Working: In DAGs the nodes with indegree=0 will act as source node because of their unreachability from all other nodes. These source nodes are then put into a queue and are allowed to run a DFS traversal to the adjacent nodes to calcualte the shortest path for nodes one by one.
Complexity Analysis:
- Time Complexity: O(V+E).
- Auxiliary space: O(V).
The Floyd Warshall Algorithm is for solving all pairs of shortest-path problems. The problem is to find the shortest distances between every pair of vertices in a given edge-weighted directed Graph.
It is an algorithm for finding the shortest path between all the pairs of vertices in a weighted graph. This algorithm follows the dynamic programming approach to find the shortest path.
Algorithm:
- Initialize the solution matrix same as the input graph matrix as a first step.
- Then update the solution matrix by considering all vertices as an intermediate vertex.
- The idea is to one by one pick all vertices and updates all shortest paths which include the picked vertex as an intermediate vertex in the shortest path.
- When we pick vertex number k as an intermediate vertex, we already have considered vertices {0, 1, 2, .. k-1} as intermediate vertices.
- For every pair (i, j) of the source and destination vertices respectively, there are two possible cases.
- k is not an intermediate vertex in shortest path from i to j. We keep the value of dist[i][j] as it is.
- k is an intermediate vertex in shortest path from i to j. We update the value of dist[i][j] as dist[i][k] + dist[k][j] if dist[i][j] > dist[i][k] + dist[k][j]
Complexity Analysis:
- Time Complexity: O(V^3), where V is the number of vertices.
- Auxiliary Space: O(V^2)
A* Search algorithm is one of the best and popular technique used in path-finding and graph traversals.
Informally speaking, A* Search algorithms, unlike other traversal techniques, it has “brains”. What it means is that it is really a smart algorithm which separates it from the other conventional algorithms. This fact is cleared in detail in below sections.
It is also worth mentioning that many games and web-based maps use this algorithm to find the shortest path very efficiently (approximation).
Complexity Analysis:
- Time Complexity: O(E*logV), where E is the number of edges and V is the number of Vertices in the graph.
- Auxiliary Space: O(V)
Using Johnson’s algorithm, we can find all pair shortest paths in O(V2log V + VE) time. Johnson’s algorithm uses both Dijkstra and Bellman-Ford as subroutines. If we apply Dijkstra’s Single Source shortest path algorithm for every vertex, considering every vertex as the source, we can find all pair shortest paths in O(V*VLogV) time.
So using Dijkstra’s single-source shortest path seems to be a better option than Floyd Warshall’s Algorithm, but the problem with Dijkstra’s algorithm is, that it doesn’t work for negative weight edge. The idea of Johnson’s algorithm is to re-weight all edges and make them all positive, then apply Dijkstra’s algorithm for every vertex.
Complexity Analysis:
- Time Complexity: O(V^2 * log V + V*E), where V is the number of vertices and E is the number of edges in the graph.
- Auxiliary Space: O(V^2)
Complexity Analysis For All Shortest Path Algorithms:
Here V and E, denote the number of vertices and the number of Edges in the graph respectively.
O(V)
|
O(V)
|
O(V+E)
|
O(V)
|
O(V+E)
|
O(V)
|
O(E*log(V))
|
O(V)
|
O(V*E)
|
O(V)
|
O(V+E)
|
O(V)
|
O(V^3)
|
O(V^2)
|
O(E*log(V))
|
O(V)
|
O(V^2 * log V + V*E)
|
O(V^2)
|
Real World Applications of Shortest Path Algorithms:
- GPS Navigation
- Network Routing
- Transportation Planning
- Airline Flight Planning
- Social Network Analysis
- Circuit Design
Share your thoughts in the comments
Please Login to comment...