Running a Scala Application using Docker
Last Updated :
01 Feb, 2024
Docker has revolutionized the way applications are developed, deployed, and scaled. In this article, we will delve into Scala, a powerful programming language, and explore how to run a Scala application using Docker. Whether you are a beginner or an experienced developer, this step-by-step guide will help you understand the process easily.
Scala is widely used in industry, particularly in environments where the benefits of both object-oriented and functional programming are appreciated. It’s commonly used in big data processing frameworks like Apache Spark and has a strong presence in the web development community.
Prerequisites
Before we begin, make sure you have the following prerequisites installed on the local system:
- Docker: Visit Docker’s official website to download and install Docker on your local machine.
- Scala: If you don’t have Scala installed, you can get it from the official Scala website OR you can refer GeeksForGeeks Article here.
Check your setup with the command, which should output:
scala --version
docker --version
Output


Create a Scala Application
Start by creating a simple Scala application. Open your favourite code editor I prefer VS Code and create a new file, for example, Hello.scala. Enter the following code:
Scala
object Geeks {
def main(args : Array[String]) : Unit = {
println( "Hello Geeks!" )
}
}
|
After completing the installation process, any IDE or text editor can be used to write Scala Codes and Run them on the IDE or the Command prompt with the use of command:
scalac file_name.Scala
scala class_name
Output

Scala application ouput
Create a Dockerfile
Create a new file named Dockerfile in the same directory as your Scala file. Add the following content to it:
FROM openjdk:8-jre-slim
This line sets the base image for the Docker image. We are using the official OpenJDK 8 runtime image.
WORKDIR /app
Sets the working directory within the container to /app.
COPY . /app:
Copies the contents of the current directory (where the Dockerfile is located) into the container at /app.
RUN apt-get update && \
apt-get install -y scala
The RUN instruction in a Dockerfile is used to execute commands during the image-building process
RUN scalac Geeks.scala
This command compiles the Scala code (Geeks.scala) using the scalac compiler. The compiled bytecode will be generated with the same name as the Scala file but with a .class extension.
CMD ["scala", "Geeks"]:
Specifies the command to run when the container starts. In this case, it runs the compiled Scala program using the scala command with the entry point being the Geeks object.
Overall Dockerfile :
# Use an official Scala runtime as a parent image
FROM openjdk:8-jre-slim
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install Scala
RUN apt-get update && \
apt-get install -y scala
# Compile the Scala code
RUN scalac Hello.scala
# Run the Scala program
CMD ["scala", "Geeks"]
If you want to know more about Dockerfile creation then please visit GeeksForGeeks Article Here.
Building a Docker Image
Assuming you have the Dockerfile and your Scala code (Geeks.scala) in the same directory, you can build and run the Docker image
# Build the Docker image
docker build -t <image_name> <tag> <path_to_dockerfile_directory>
docker build -t docker-scala-img .
Output
-(1).png)
If you are getting error during creation of docker image then create .dockerignore file and add .metals/ to created file.
The Overall Root Directory Should Looks Like
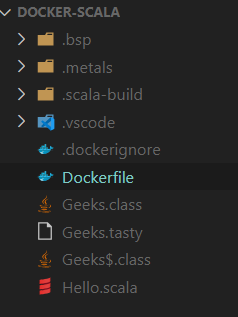
To see the created Docker images use following command :
docker images
Output

Also you can see images using Docker Desktop application,
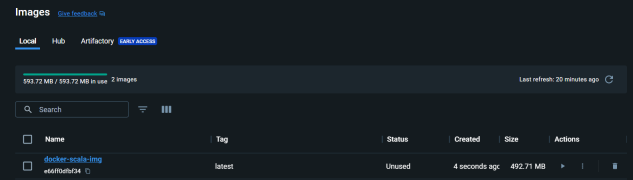
Run the Docker Container:
After building the image, run the Docker container with the following command:
docker run <image-name>
Output

Also you can see Running Container using Docker Desktop application,
.png)
You should see the output “Hello, Geeks!” indicating that your Scala application is running inside a Docker container.
Running a Scala Application using Docker – FAQs
What is Docker, and why use it for Scala applications?
Docker is a containerization platform that simplifies application deployment. Using Docker for Scala applications `ensures consistent environments across different stages, from development to production.
How do I create a Docker image for my Scala application?
Create a Dockerfile specifying the Scala base image, copy your application code, and define necessary dependencies. Then, use docker build to create the image.
Can I run a Scala application as a Docker container locally for testing?
Yes, use docker run to start a container locally, exposing necessary ports. This allows you to test your Scala application in a controlled Docker environment.
How can I manage dependencies in my Scala project within a Docker container?
Use build tools like SBT or Maven. Copy the build.sbt or pom.xml file into the Docker image, and run dependency resolution during the Docker build process.
What considerations are important for optimizing Scala Docker images?
Minimize image layers, use multi-stage builds to reduce image size, and avoid unnecessary dependencies. This enhances performance and speeds up image deployment.
How can I configure environment variables for my Scala application in Docker?
Use the -e option with docker run or specify environment variables in your Docker Compose file. This allows dynamic configuration of your Scala application.
Share your thoughts in the comments
Please Login to comment...