React MUI Content Security Policy
Last Updated :
11 Jul, 2023
Content Security Policy (CSP) is a security feature that helps to protect your web applications from a variety of attacks including cross-site scripting (XSS), data exfiltration, and code injection. CSP works by restricting the types of content that can be loaded by a web page.
Material-UI is a popular React UI library that provides a wide range of components for building user interfaces. While Material-UI is secure by default, it is still a good idea to configure CSP for your application to add an extra layer of security.
When you are using MUI(Material UI) with React.js, it is important to set up Content Security Policy(CSP) with your React App to secure your web app against cross-site scripting (XSS) attacks. CSP helps to prevent XSS attacks on your web page.
Syntax:
const csp = `
default-src 'self';
script-src 'self';
style-src 'self';
`;
To configure CSP with your Material-UI React application, you will need to follow the steps mentioned below:
Basic Setup and React App Installation:
Step 1: Create a React app boilerplate using the below command
npx create-react-app <app-name>
Ex: npx create-react-app react-mui
Step 2: Go to the root directory of your app.
cd react-mui
Step 3: Install react required MUI packages.
npm install @emotion/styled @material-ui/core @emotion/react
step 4: install React helmet package.
npm install helmet react-helmet
step 5: Create a new file MaterialUi.js inside src>component>MaterialUi.js
cd src
mkdir component
touch MaterialUi.js
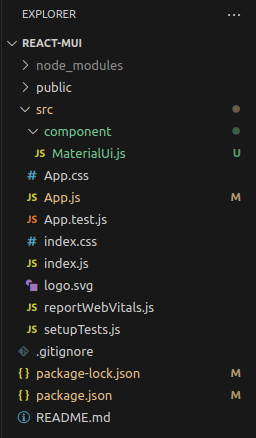
folder structure
Step 6: import the helmet library into the App.js file
import { Helmet } from "react-helmet";
Example 1: Setting up CSP with React MUI using react helmet library
App.js
Javascript
import MaterialUi from "./component/MaterialUi" ;
import { Helmet } from "react-helmet" ;
const csp = `
default -src 'self' ;
script-src 'self' 'unsafe-inline' ;
style-src 'self' 'unsafe-inline' ;
img-src 'self' data:;
font-src 'self' data:;
`;
console.log( "csp--> " , { csp });
const App = () => {
return (
<>
<Helmet>
<meta
http-equiv= "Content-Security-Policy"
content={csp} />
</Helmet>
<MaterialUi />
</>
);
};
export default App;
|
MaterialUi.js
Javascript
import React from "react" ;
function MaterialUi() {
return (
<>
<div>
<h2>Hello Geeks</h2>
Welcome to the React MUI
Content Security Policy in
<h4>GeeksforGeeks</h4>
</div>
</>
);
}
export default MaterialUi;
|
Step 7: Run your React app using the command.
npm run start
Output:
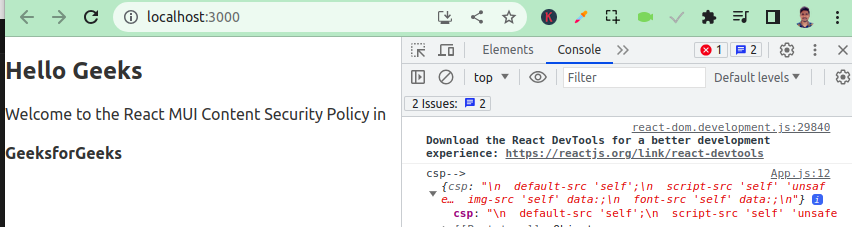
Example 2: Setting up CSP with React MUI using the react-helmet-async library.
Step 1: Install the react-helmet-async library.
npm i react-helmet-async
App.js
Javascript
import MaterialUi from "./component/MaterialUi" ;
import { HelmetProvider } from 'react-helmet-async' ;
import { CssBaseline } from '@material-ui/core' ;
const csp = `
default -src 'self' ;
script-src 'self' 'unsafe-inline' ;
style-src 'self' 'unsafe-inline' ;
img-src 'self' data:;
font-src 'self' data:;
`;
console.log( "csp--> " , { csp });
const App = () => {
return (
<>
<HelmetProvider>
<CssBaseline />
<MaterialUi />
</HelmetProvider>
</>
);
};
export default App;
|
MaterialUi.js
Javascript
import React from "react" ;
import { Helmet } from 'react-helmet-async' ;
import { Button } from '@material-ui/core' ;
function MaterialUi() {
return (
<>
<Helmet>
<meta
http-equiv= "Content-Security-Policy"
content= "
default-src 'self';
script-src 'self' 'unsafe-inline' 'unsafe-eval';
style-src 'self' 'unsafe-inline';
font-src 'self' data:;
img-src 'self' data:;
"
/>
</Helmet>
<Button variant= "contained" color= "secondary" >
Hello, Geeks!
</Button>
</>
);
}
export default MaterialUi;
|
Output:
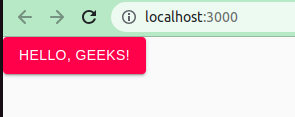
This sets the ‘Content-Security-Policy’ header to allow resources to be loaded only from the same origin (‘self’) and also allows inline scripts and styles.
Share your thoughts in the comments
Please Login to comment...