React JS Component Composition and Nested Components
Last Updated :
18 Mar, 2024
React JS Component Composition allows you to build complex UIs by combining smaller, reusable components. Nested Components refer to components that are used within other components, enabling a modular and structured approach to building React applications.
Steps to setup React Application
Step 1: Create a new react app and enter it by running the following commands
npx create-react-app composition-vs-nesting
Step 2: Change the directory
cd composition-vs-nesting
Project Structure:
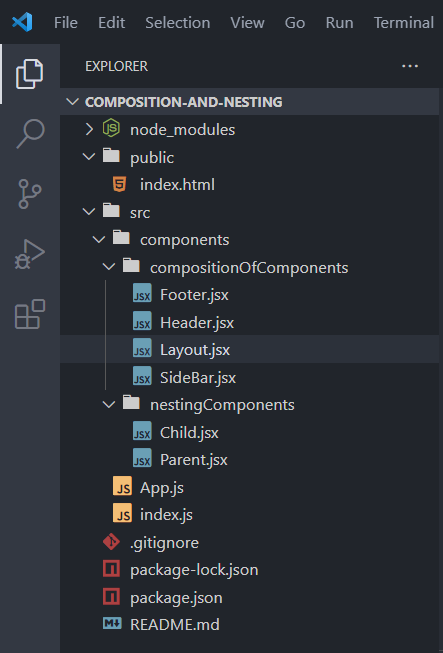
PROJECT STRUCTURE FOR COMPOSITION AND NESTING OF COMPONENTS
Updated Dependencies: The updated dependencies in package.json file should look like this.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Component Composition
Component Composition means combining various other smaller React components in a bigger component so as to create modular architecture in the web application. It breaks the application into smaller reusable components and hence makes the user interface look much more simple.
Example: Illustration to showcase the use of composition component.
Javascript
// Layout.js
import React from 'react'
import Header from './Header'
import SideBar from './SideBar'
import Footer from './Footer'
const Layout = () => {
return (
<div>
<b>Layout ---</b>
<Header />
<SideBar />
<Footer />
</div>
)
}
export default Layout
Javascript
// Header.js
import React from 'react'
const Header = () => {
return (
<div>Header</div>
)
}
export default Header
Javascript
// SideBar.js
import React from 'react'
const SideBar = () => {
return (
<div>SideBar</div>
)
}
export default SideBar
Javascript
// Footer.js
import React from 'react'
const Footer = () => {
return (
<div>Footer</div>
)
}
export default Footer
JavaScript
// App.js
import Layout from "./components/compositionOfComponents/Layout";
import Parent from "./components/nestingComponents/Parent";
function App() {
return (
<div>
<h3>Composition of Components</h3>
<Layout />
</div>
);
}
export default App;
Output :
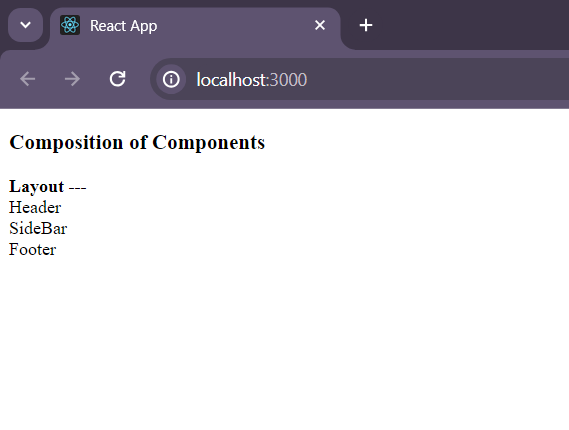
OUTPUT IMAGE FOR COMPOSITION OF COMPONENTS
Nested Component
Nesting of Components creates the parent-child relationship between the nested components. In this approach, children components are nested by including them in the JSX markup of parent component. It helps in organizing the components in proper structure.
Example: Illustration to showcase the use of nested component.
Javascript
// Parent.js
import React from 'react'
import Child from './Child'
const Parent = () => {
return (
<div>
<b>This is Parent</b>
<Child />
</div>
)
}
export default Parent
Javascript
// Child.js
import React from 'react'
const Child = () => {
return (
<div>Child nested within parent</div>
)
}
export default Child
JavaScript
// App.js
import Layout from "./components/compositionOfComponents/Layout";
import Parent from "./components/nestingComponents/Parent";
function App() {
return (
<div>
<h3>Nesting of Components</h3>
<Parent />
</div>
);
}
export default App;
Output:
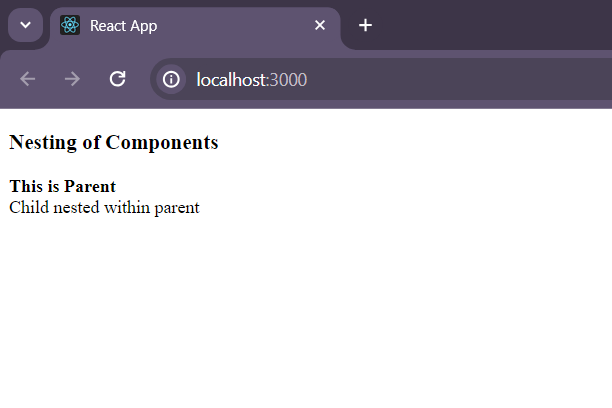
OUTPUT IMAGE FOR NESTING OF COMPONENTS
Similarity between component composition and nesting component
- Increases the reusability of components
- Incorporates modularity in web application
- Increases readability of the code
- It eases the code maintainability
- It leads to easy scalability of the application
- It eases the flow of data across the application
Difference between component composition and nesting component
Aspect | Component Composition | Nesting Components |
---|
Relationship | Combines smaller parts into a larger component | Creates a parent-child relationship |
Flexibility | Offers more flexibility | Can be less flexible due to tight coupling |
Complexity Management | Converts complex problems into simpler ones | Can become complex, especially in large apps |
Reusability | Promotes reusability of smaller components | Utilizes hierarchical structure for reusability |
Code Structure | Provides a modular architecture | Creates a structured hierarchy |
Maintenance | Simplifies maintenance with modular components | Requires careful management of parent-child relationship |
Share your thoughts in the comments
Please Login to comment...