Python MongoDB – find_one_and_delete query
Last Updated :
15 Jun, 2022
MongoDB is a cross-platform document-oriented and a non relational (i.e NoSQL) database program. It is an open-source document database, that stores the data in the form of key-value pairs.
find_one_and_delete()
This function is used to delete a single document from the collection based on the filter that we pass and returns the deleted document from the collection. It finds the first matching document that matches the filter and deletes it from the collection i.e finds a single document and deletes it, returning the document.
Syntax:
Collection.find_one_and_delete(filter, projection=None, sort=None, session=None, **kwargs)
Parameters:
- ‘filter’ : A query that matches the document to delete.
- ‘projection’ (optional): A list of field names that should be returned in the
result document or a mapping specifying the fields to include or exclude. If
‘projection’ is a list “_id” will always be returned. Use a mapping to exclude
fields from the result (e.g. projection={‘_id’: False}).
‘
- sort’ (optional): A list of (key, direction) pairs specifying the sort order for
the query. If multiple documents match the query, they are sorted and the first is deleted.
- ‘session’ (optional): A class: “~pymongo.client_session.ClientSession”.
- ‘**kwargs’ (optional): Additional command arguments can be passed as keyword arguments
(for example maxTimeMS can be used with recent server versions).
The sample database is as follows:
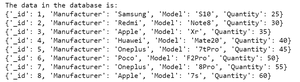
The database on which we operate.
Example 1:
Python3
from pymongo import MongoClient
db = myclient[ "mydatabase" ]
Collection = db[ "GeeksForGeeks" ]
Filter = { 'Manufacturer' : 'Apple' }
print ( "The returned document is:" )
print (Collection.find_one_and_delete( Filter ))
print ( "\nThe data after find_one_and_delete() operation is:" )
for data in Collection.find():
print (data)
|
Output :
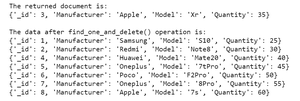
Example 2:
In this example we delete the Redmi data from the database using the find_one_and_delete() method:
Python3
from pymongo import MongoClient
db = myclient[ "mydatabase" ]
Collection = db[ "GeeksForGeeks" ]
Filter = { 'Manufacturer' : 'Redmi' }
print ( "The returned document is:" )
print (Collection.find_one_and_delete( Filter )
print ( "\nThe data after find_one_and_delete() operation is:" )
for data in Collection.find():
print (data)
|
Output :
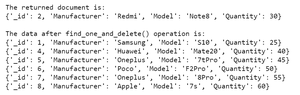
Share your thoughts in the comments
Please Login to comment...