Python MongoDB – Limit Query
Last Updated :
13 Jul, 2022
MongoDB is one of the most used databases with its document stored as collections. These documents can be compared to JSON objects. PyMongo is the Python driver for mongoDB.
Limit() Method: The function limit() does what its name suggests- limiting the number of documents that will be returned. There is only one argument in the parameter which is a number signifying the number of documents that need to be returned.
Syntax:
coll.find().limit(n)
where,
- coll- name of the collection
- n- number that needs to be returned
Sample Database is as follows:
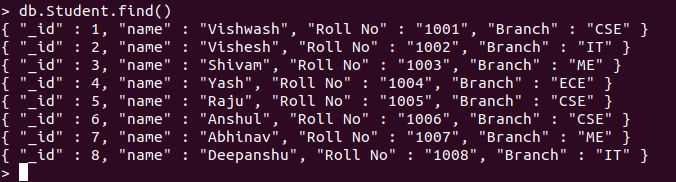
Example 1:
Python3
from pymongo import MongoClient
client = MongoClient( 'localhost' , 27017 )
db = client[ 'GFG' ]
doc = db[ 'Student' ]
print (& quot
First 3 docs in the collection are: & quot
)
for doc1 in doc.find().limit( 3 ):
print (doc1)
|
Output:
First 3 docs in the collection are: {‘_id’: 1, ‘name’: ‘Vishwash’, ‘Roll No’: ‘1001’, ‘Branch’: ‘CSE’} {‘_id’: 2, ‘name’: ‘Vishesh’, ‘Roll No’: ‘1002’, ‘Branch’: ‘IT’} {‘_id’: 3, ‘name’: ‘Shivam’, ‘Roll No’: ‘1003’, ‘Branch’: ‘ME’}
While limit() puts a limitation on the number of documents fetched, find() can be used to find documents according to some specified criteria.
Example 2:
Python3
from pymongo import MongoClient
client = MongoClient( 'localhost' , 27017 )
db = client[ 'GFG' ]
doc = db[ 'Student' ]
for doc1 in doc.find({ 'Branch' : 'CSE' }).limit( 1 ):
print (doc1)
|
Output:
{‘_id’: 1, ‘name’: ‘Vishwash’, ‘Roll No’: ‘1001’, ‘Branch’: ‘CSE’}
For skipping some files before fetching the said amount of documents skip() can be used with limit()
Example 3:
Python3
from pymongo import MongoClient
client = MongoClient( 'localhost' , 27017 )
db = client[ 'GFG' ]
doc = db[ 'Student' ]
print (& quot
3 docs in the collection are: & quot
)
for doc1 in doc.find().limit( 3 ).skip( 2 ):
print (doc1)
|
Output:
3 docs in the collection are: {‘_id’: 3, ‘name’: ‘Shivam’, ‘Roll No’: ‘1003’, ‘Branch’: ‘ME’} {‘_id’: 4, ‘name’: ‘Yash’, ‘Roll No’: ‘1004’, ‘Branch’: ‘ECE’} {‘_id’: 5, ‘name’: ‘Raju’, ‘Roll No’: ‘1005’, ‘Branch’: ‘CSE’}
Share your thoughts in the comments
Please Login to comment...