Python MongoDB – find_one_and_update Query
Last Updated :
04 Jul, 2022
The function find_one_and_update() actually finds and updates a MongoDB document. Though default-wise this function returns the document in its original form and to return the updated document return_document has to be implemented in the code.
Syntax:
coll.find_one_and_update(filter, update, options)
Parameters:
- col- collection in MongoDB
- filter- criteria to find the document which needs to be updated
- update- The operations which need to be implemented for updating the document
- options- projection or upsert can be used here
- projection- a mapping which informs about which fields are included and excluded, it is 1/TRUE for including a field and 0/FALSE for excluding
- upsert- for inserting a new document if no file is found with the mentioned criteria upsert is TRUE
- return_document: If ReturnDocument.BEFORE (the default), returns the original document before it was replaced, or None if no document matches. If ReturnDocument.AFTER, returns the replaced or inserted document.
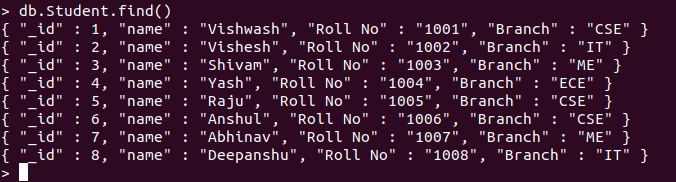
Example 1: Sample Database:
Python3
from pymongo import MongoClient
from pymongo import ReturnDocument
client = MongoClient( 'localhost' , 27017 )
db = client[ 'GFG' ]
doc = db[ 'Student' ]
print (doc.find_one_and_update({ 'name' :"Raju"},
{ '$set' : { "Branch" : 'ECE' } },
return_document = ReturnDocument.AFTER))
|
Output:
{'_id': 5, 'name': 'Raju', 'Roll No': '1005', 'Branch': 'ECE'}
Example 2:
Python3
from pymongo import MongoClient
from pymongo import ReturnDocument
client = MongoClient( 'localhost' , 27017 )
db = client[ 'GFG' ]
doc = db[ 'Student' ]
print (
doc.find_one_and_update({ 'name' : "Raju"},
{ '$set' : { "Branch" : 'CSE' } },
projection = { "name" : 1 , "Branch" : 1 },
return_document = ReturnDocument.AFTER))
|
Output:
{'_id': 5, 'name': 'Raju', 'Branch': 'CSE'}
Share your thoughts in the comments
Please Login to comment...