Public vs Package Access Modifiers in Java
Last Updated :
18 Apr, 2022
Whenever we are writing our classes, we have to provide some information about our classes to the JVM like whether this class can be accessed from anywhere or not, whether child class creation is possible or not, whether object creation is possible or not, etc. we can specify this information by using an appropriate keyword in java called access modifiers. So access modifiers are used to set accessibility of classes, methods, and other members.
Lets us discuss both of the modifiers as follows:
- Public Access Modifiers
- Package(Default) Access Modifier
Modifier 1: Public Access Modifiers
If a class is declared as public then we can access that class from anywhere.
We will be creating a package pack1 inside that package we declare a class A which is public and inside that class, we declare a method m1 which is also public. Now we create another package pack2 and inside that pack2 we import pack1 and declare a class B and in class B’s main method we create an object of type class A and trying to access the data of method m1.
Example 1:
Java
package pack1;
import java.io.*;
import java.util.*;
public class A {
public void m1() { System.out.println( "GFG" ); }
}
|
Output: Compiling and saving the above code by using the below command line:
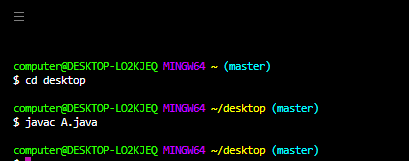
Here we will be importing the above class of the created package to the newly created package.
Example 2:
Java
package pack2;
import java.io.*;
import java.util.*;
import pack1.A;
class B {
public static void main(String[] args)
{
A a = new A();
a.m1();
}
}
|
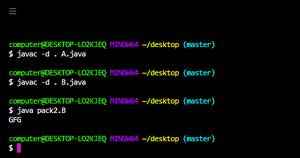
Output Explanation:
If class A is not public while compiling B class we will get a compile-time error saying pack1. A is not public in pack1 and can’t be accessed from the outside package. Similarly, a member or method, or interface is declared as public as we can access that member from anywhere.
Modifier 2: Package(Default) Access Modifier
A class or method or variable declare without any access modifier then is considered that it has a package(default)access modifier The default modifier act as public within the same package and acts as private outside the package. If a class is declared as default then we can access that class only within the current package i.e from the outside package we can’t access it. Hence, the default access modifier is also known as the package–level access modifier. A similar rule also applies for variables and methods in java.
Example:
Java
import java.io.*;
import java.util.*;
class GFG {
String s = "Geeksfor" ;
String s1 = "Geeks" ;
String fullName()
{
return s + s1;
}
public static void main(String[] args)
{
GFG g = new GFG();
System.out.println(g.fullName());
}
}
|
Output:
GeeksforGeeks
Finally, after understanding and going through both of them let us conclude the evident differences between them which are depicted in the table below as follows:
Public Access Modifier |
Package Access Modifier |
Public members can be accessed from a non-child class of outside package. |
This modifier can’t be accessed from a non-child class of the outside package. |
Public members can be accessed from child class of outside package. |
We cannot access this modifier from the child class of the outside package. |
The public modifier is more accessible than the package access modifier. |
This modifier is more restricted than the public access modifier. |
Share your thoughts in the comments
Please Login to comment...