Java Program to Create a Package to Access the Member of External Class and Same Package
Last Updated :
18 Aug, 2021
As the name suggests a package in java that contains all the same kinds of classes, Abstract classes, interfaces, etc that have the same functionality. A Single package can contain many classes, Abstract classes e.g. java.util package etc.
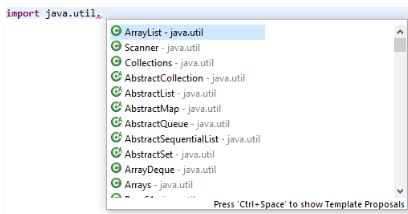
There are two types of packages:
- Built-in-package: These are the packages that are predefined in the java jar file. The most commonly used package in java. util and java.io package in java.
- User-defined package: These packages are created by the user that used to store related classes some other utility functions, interfaces and abstract classes, etc which are defined for the specific task is called a user-defined package.
Project structure:
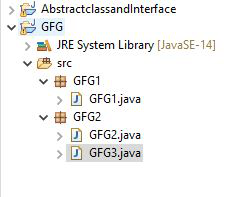
Project -- GFG
|
|
Package 1 GFG1
|
GFG1.java (class)
Package 2 GFG2
|
GFG2.java (class)
|
GFG3.java (class)
Package 1:
GFG1.java
Java
package GFG1;
interface GFG1Interface {
String name = "This is the Interface of GF1" ;
void GFG1Interface();
}
public class GFG1 {
String name;
public String getName() { return name; }
public void setName(String name) { this .name = name; }
}
|
Package 2:
GFG3.java
Java
package GFG2;
interface GFG3Interface {
String name = "GFG" ;
public void interfaceGFG();
}
abstract class GFGabstract {
String name = "GFGAbstract" ;
abstract public void print();
}
public class GFG3 {
int first;
int second;
GFG3( int a, int b)
{
this .first = a;
this .second = b;
}
public int add() { return this .first + this .second; }
}
|
Accessing the members of package 1 class in package 2 class:
GFG2.java
Java
package GFG2;
import GFG1.*;
public class GFG2 implements GFG3Interface {
@Override public void interfaceGFG()
{
System.out.println(
"This is the interface of the GFG3class" );
}
public static void main(String args[])
{
GFG1 ob = new GFG1();
ob.setName( "GFGsetter" );
System.out.println(ob.getName());
GFG2 ob2 = new GFG2();
ob2.interfaceGFG();
}
}
|
Output:

Share your thoughts in the comments
Please Login to comment...