POTD Solutions | 4 Nov’ 23 | Find Transition Point
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Binary Search algorithm but will also help you build up problem-solving skills.
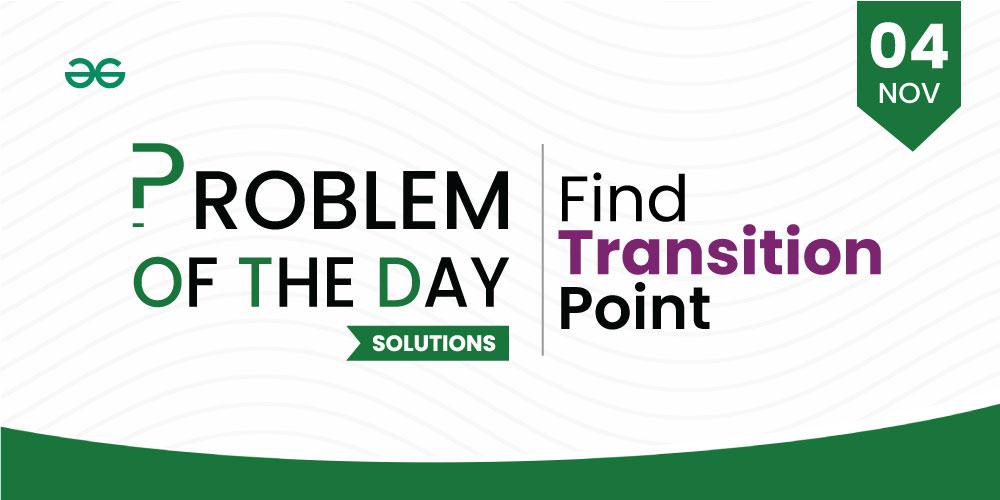
POTD Solution 4th November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 4 November: Find Transition Point:
Given a sorted array containing only 0s and 1s, find the transition point, i.e., the first index where 1 was observed, and before that, only 0 was observed.
Examples:
Input: N = 5
arr[] = {0,0,0,1,1}
Output: 3
Explanation: Index 3 is the transition point where 1 begins
Input: N = 4
arr[] = {0,0,0,0}
Output: -1
Explanation: Since, there is no “1”,
the answer is -1.
The idea is to use Binary Search since the array is sorted. Iteratively check the middle element of the array, if the element encountered is 1 store its index and then repeat the same procedure for left part of the array else if the element encountered is 1 repeat the same procedure for right part of the array.
Follow the steps to solve the problem:
- Create two variables, l and r, initialize l = 0 and r = n-1 and a variable index= -1 to store the answer.
- Iterate the steps below till l <= r, the lower-bound is less than the upper-bound.
- Set mid is equal to (l+r)/2 and check the element at position mid,
- If the element is 1, set index to mid and h (upper bound) to mid-1.
- If the element is 0, l (lower bound) to mid+1.
- Return the index
Below is the implementation of above approach:
C++
class Solution {
public :
int transitionPoint( int arr[], int n) {
int l = 0;
int h = n - 1;
int index = -1;
while (l <= h) {
int mid = (l + h) / 2;
if (arr[mid] == 1) {
index = mid;
h = mid - 1;
} else {
l = mid + 1;
}
}
return index;
}
};
|
Java
class Solution {
int transitionPoint( int arr[], int n) {
int l = 0 ;
int h = n - 1 ;
int index = - 1 ;
while (l <= h) {
int mid = (l + h) / 2 ;
if (arr[mid] == 1 ) {
index = mid;
h = mid - 1 ;
} else {
l = mid + 1 ;
}
}
return index;
}
}
|
Python3
class Solution:
def transitionPoint( self , arr, n):
l = 0
h = n - 1
index = - 1
while l < = h:
mid = (l + h) / / 2
if arr[mid] = = 1 :
index = mid
h = mid - 1
else :
l = mid + 1
return index
|
Time Complexity: O(log n). The time complexity for binary search is O(log n).
Auxiliary Space: O(1). No extra space is required.
Share your thoughts in the comments
Please Login to comment...