POTD Solutions | 3 Nov’ 23 | Pythagorean Triplet
Last Updated :
12 Feb, 2024
Javascript
<script>
class Solution {
checkTriplet(arr, n) {
for (let i = 0; i < n; i++)
arr[i] = arr[i] * arr[i];
arr.sort((a, b) => a - b);
for (let i = n - 1; i >= 2; i--) {
let l = 0;
let r = i - 1;
while (l < r) {
if (arr[l] + arr[r] === arr[i])
return true ;
(arr[l] + arr[r] < arr[i]) ? l++ : r--;
}
}
return false ;
}
}
const solution = new Solution();
const array = [3, 1, 4, 6, 5];
const result = solution.checkTriplet(array, array.length);
console.log(result);
</script>
|
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Hashing but will also help you build up problem-solving skills.
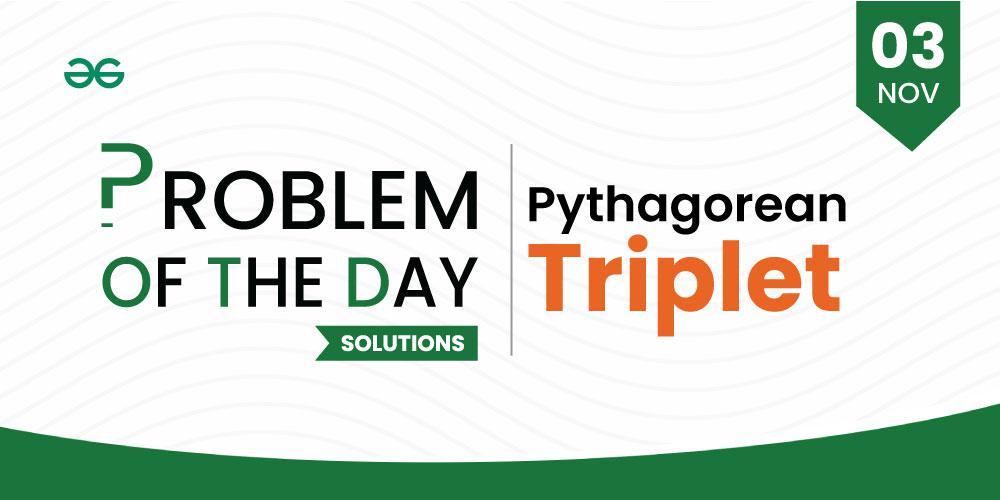
POTD Solutions | 3 November 2023
POTD 3 November: Pythagorean Triplet
Given an array arr of n integers, write a function that returns true if there is a triplet (a, b, c) from the array (where a, b, and c are on different indexes) that satisfies a2 + b2 = c2, otherwise return false.
Input: arr[] = {3, 1, 4, 6, 5}Â
Output: TrueÂ
There is a Pythagorean triplet (3, 4, 5).
Input: arr[] = {10, 4, 6, 12, 5}Â
Output: FalseÂ
There is no Pythagorean triplet.Â
A Pythagorean triplet is a set of three integers (a, b, c) such that a^2 + b^2 = c^2.
The idea is to squares the elements of the input array, sorts them, and then iterates through the array to find if there are three elements (i, l, r) such that i^2 = l^2 + r^2, where ‘i’ is the largest element and ‘l’ and ‘r’ are two other elements in the array. If such a triplet is found, the function returns true; otherwise, it returns false.
Step-by-step approach:
- Square each element of the input array.
- Sort the squared array in non-decreasing order.
- Iterate through the sorted array from the largest element to the smallest (starting from the end).
- For each current largest element ‘i‘, set two pointers, ‘l’ to the beginning of the array and ‘r’ to the end.
- While ‘l’ is less than ‘r’, check if the sum of the squared elements at ‘l’ and ‘r’ equals the squared element at ‘i’. If it does, return true because a Pythagorean triplet is found.
- If the sum is less than the squared element at ‘i’, increment ‘l’; otherwise, decrement ‘r’.
- If the loop completes without finding a Pythagorean triplet, return false because none was found in the array.
Illustration:
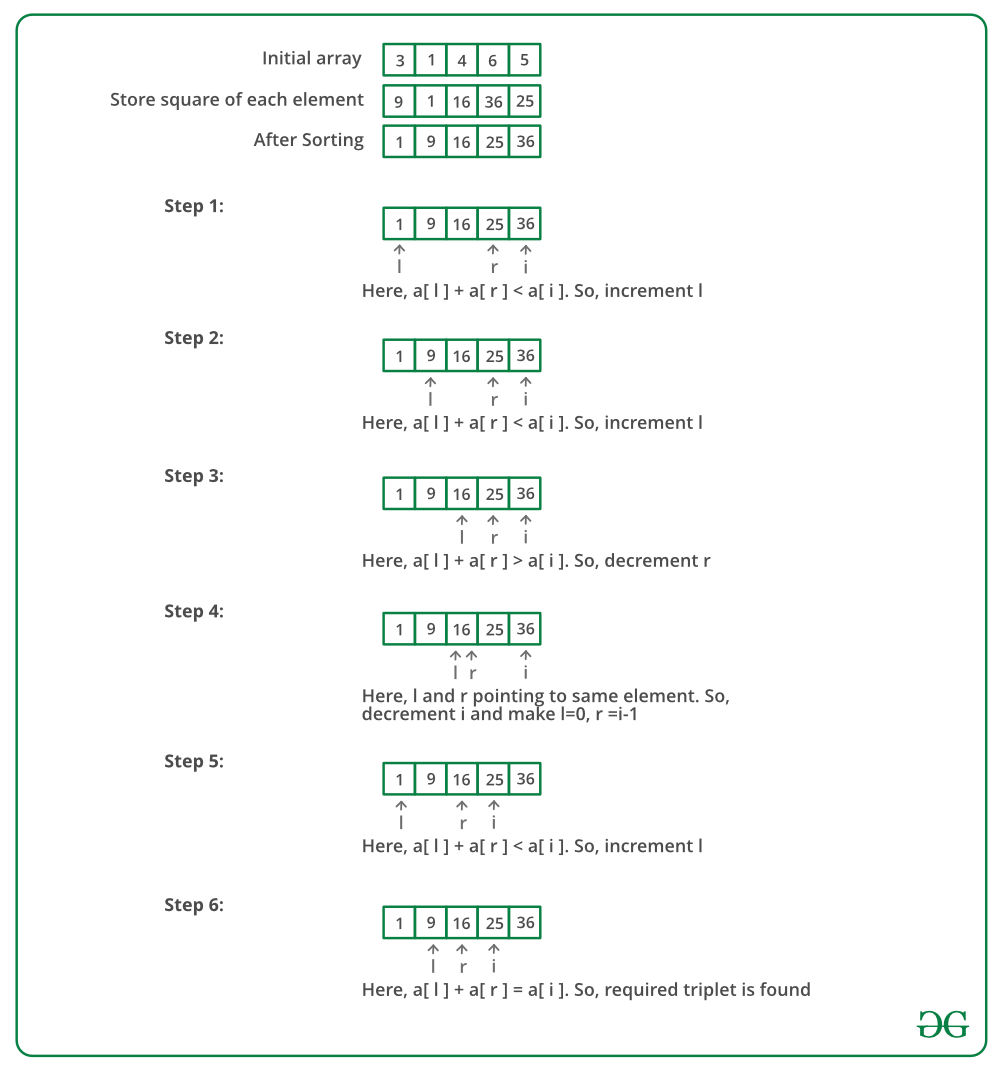
Below is the implementation of the above approach:Â
C++
class Solution{
public:
// Function to check if the
// Pythagorean triplet exists or not
bool checkTriplet(int arr[], int n) {
// Square array elements
for (int i = 0; i < n; i++)
arr[i] = arr[i] * arr[i];
// Sort array elements
sort(arr, arr + n);
// Now fix one element one by one and find the other two
// elements
for (int i = n - 1; i >= 2; i--) {
// To find the other two elements, start two index
// variables from two corners of the array and move
// them toward each other
int l = 0; // index of the first element in arr[0..i-1]
int r = i - 1; // index of the last element in arr[0..i-1]
while (l < r) {
// A triplet found
if (arr[l] + arr[r] == arr[i])
return true;
// Else either move 'l' or 'r'
(arr[l] + arr[r] < arr[i]) ? l++ : r--;
}
}
// If we reach here, then no triplet found
return false;
}
};
Java
class Solution {
boolean checkTriplet(int[] arr, int n) {
// Square array elements
for (int i = 0; i < n; i++)
arr[i] = arr[i] * arr[i];
// Sort array elements
Arrays.sort(arr);
// Now fix one element one by one and find the other two
// elements
for (int i = n - 1; i >= 2; i--) {
// To find the other two elements, start two index
// variables from two corners of the array and move
// them toward each other
int l = 0; // index of the first element in arr[0..i-1]
int r = i - 1; // index of the last element in arr[0..i-1]
while (l < r) {
// A triplet found
if (arr[l] + arr[r] == arr[i])
return true;
// Else either move 'l' or 'r'
if (arr[l] + arr[r] < arr[i])
l++;
else
r--;
}
}
// If we reach here, then no triplet found
return false;
}
}
Python3
class Solution:
def checkTriplet(self,arr, n):
# Create a dictionary to store the square of each element as keys
# and the corresponding original element as values.
square_map = {i * i: i for i in arr}
# Iterate through the square_map to find triplets with the sum of squares.
for square1 in square_map:
for square2 in square_map:
# Calculate the sum of two squared values.
sum_of_squares = square1 + square2
# Check if the sum exists in the square_map, indicating the presence of a triplet.
if sum_of_squares in square_map:
return True
# If no triplet is found, return False.
return False
Time complexity: O(n2).
Auxiliary Space: O(1)
The problem can also be solved using hashing. We can use a hash map to mark all the values of the given array. Using two loops, we can iterate for all the possible combinations of a and b, and then check if there exists the third value c. If there exists any such value, then there is a Pythagorean triplet.Â
Below is the implementation of the above approach:Â Â
C++
class Solution{
public:
// Function to check if the
// Pythagorean triplet exists or not
bool checkTriplet(int arr[], int n) {
int maximum = 0;
// Find the maximum element
for (int i = 0; i < n; i++) {
maximum = max(maximum, arr[i]);
}
// Hashing array
int hash[maximum + 1] = { 0 };
// Increase the count of array elements
// in hash table
for (int i = 0; i < n; i++)
hash[arr[i]]++;
// Iterate for all possible a
for (int i = 1; i < maximum + 1; i++) {
// If a is not there
if (hash[i] == 0)
continue;
// Iterate for all possible b
for (int j = 1; j < maximum + 1; j++) {
// If a and b are same and there is only one a
// or if there is no b in original array
if ((i == j && hash[i] == 1) || hash[j] == 0)
continue;
// Find c
int val = sqrt(i * i + j * j);
// If c^2 is not a perfect square
if ((val * val) != (i * i + j * j))
continue;
// If c exceeds the maximum value
if (val > maximum)
continue;
// If there exists c in the original array,
// we have the triplet
if (hash[val]) {
return true;
}
}
}
return false;
}
};
Java
class Solution {
boolean checkTriplet(int[] arr, int n) {
int maximum = 0;
// Find the maximum element
for (int i = 0; i < n; i++) {
maximum = Math.max(maximum, arr[i]);
}
// Hashing array
int[] hash = new int[maximum + 1];
// Increase the count of array elements in the hash table
for (int i = 0; i < n; i++) {
hash[arr[i]]++;
}
// Iterate for all possible 'a'
for (int i = 1; i < maximum + 1; i++) {
// If 'a' is not there
if (hash[i] == 0)
continue;
// Iterate for all possible 'b'
for (int j = 1; j < maximum + 1; j++) {
// If 'a' and 'b' are the same and there is only one 'a'
// or if there is no 'b' in the original array
if ((i == j && hash[i] == 1) || hash[j] == 0)
continue;
// Find 'c'
int val = (int) Math.sqrt(i * i + j * j);
// If 'c^2' is not a perfect square
if (val * val != (i * i + j * j))
continue;
// If 'c' exceeds the maximum value
if (val > maximum)
continue;
// If there exists 'c' in the original array, we have the triplet
if (hash[val] > 0) {
return true;
}
}
}
return false;
}
}
Python
import math
class Solution:
def checkTriplet(self,ar, n):
maximum = 0
# Find the maximum element
for num in arr:
maximum = max(maximum, num)
# Hashing array
hash_table = [0] * (maximum + 1)
# Increase the count of array elements in the hash table
for num in arr:
hash_table[num] += 1
# Iterate for all possible 'a'
for i in range(1, maximum + 1):
# If 'a' is not there
if hash_table[i] == 0:
continue
# Iterate for all possible 'b'
for j in range(1, maximum + 1):
# If 'a' and 'b' are the same and there is only one 'a'
# or if there is no 'b' in the original array
if (i == j and hash_table[i] == 1) or hash_table[j] == 0:
continue
# Find 'c'
val = int(math.sqrt(i * i + j * j))
# If 'c^2' is not a perfect square
if val * val != (i * i + j * j):
continue
# If 'c' exceeds the maximum value
if val > maximum:
continue
# If there exists 'c' in the original array, we have the triplet
if hash_table[val] > 0:
return True
return False