POTD Solutions | 21 Nov’ 23 | Determine if Two Trees are Identical
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Binary Tree but will also help you build up problem-solving skills.
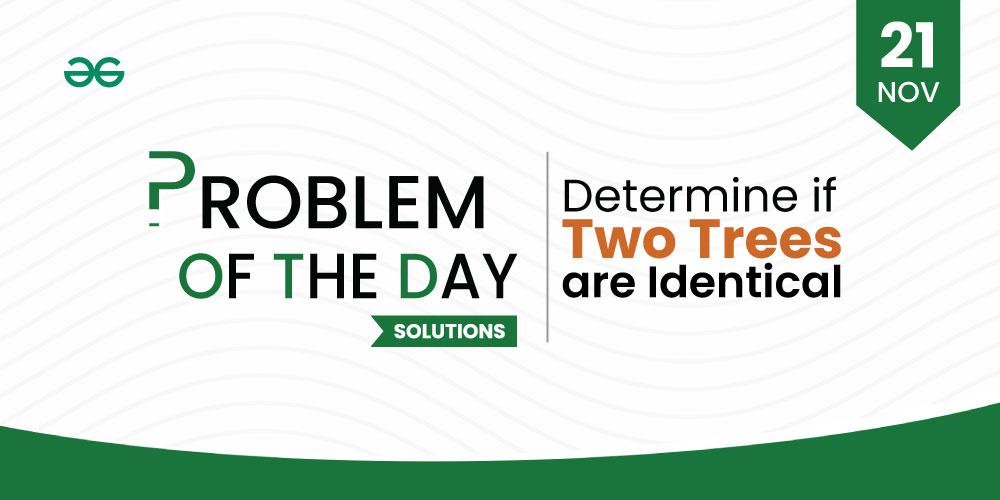
POTD Solution 21 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 21 November: Determine if Two Trees are Identical:
Given two binary trees, the task is to find if both of them are identical or not. Return true if they are identical, else return false.
Examples:
Input: 1 1
/ \ / \
2 3 2 3
/ /
4 4
Output: Yes
Explanation: Both trees are identical as the have same root, left and right child.
Input: 1 1
/ \ / \
2 3 5 3
/ /
4 4
Output: Trees are not identical
The basic idea behind the Morris traversal approach to solve the problem of checking if two binary trees are identical is to use the Morris traversal algorithm to traverse both trees in-order simultaneously, and compare the nodes visited at each step.
Follow the steps to implement the above idea:
- Check if both trees are empty. If they are, return true. If only one of them is empty, return false.
- Perform the Morris traversal for in-order traversal of both trees simultaneously. At each step, compare the nodes visited in both trees.
- If at any step, the nodes visited in both trees are not equal, return false.
- If we reach the end of both trees simultaneously (i.e., both nodes are NULL), return true.
Below is the implementation of the above idea:
C++
class Solution {
public :
bool isIdentical(Node* r1, Node* r2)
{
if (r1 == NULL && r2 == NULL)
return true ;
if (r1 == NULL || r2 == NULL)
return false ;
while (r1 != NULL && r2 != NULL) {
if (r1->data != r2->data)
return false ;
if (r1->left == NULL) {
r1 = r1->right;
}
else {
Node* pre = r1->left;
while (pre->right != NULL
&& pre->right != r1)
pre = pre->right;
if (pre->right == NULL) {
pre->right = r1;
r1 = r1->left;
}
else {
pre->right = NULL;
r1 = r1->right;
}
}
if (r2->left == NULL) {
r2 = r2->right;
}
else {
Node* pre = r2->left;
while (pre->right != NULL
&& pre->right != r2)
pre = pre->right;
if (pre->right == NULL) {
pre->right = r2;
r2 = r2->left;
}
else {
pre->right = NULL;
r2 = r2->right;
}
}
}
return (r1 == NULL && r2 == NULL);
}
};
|
Java
class Solution {
boolean isIdentical(Node root1, Node root2)
{
if (root1 == null && root2 == null )
return true ;
if (root1 == null || root2 == null )
return false ;
while (root1 != null && root2 != null ) {
if (root1.data != root2.data)
return false ;
if (root1.left == null ) {
root1 = root1.right;
}
else {
Node pre = root1.left;
while (pre.right != null
&& pre.right != root1)
pre = pre.right;
if (pre.right == null ) {
pre.right = root1;
root1 = root1.left;
}
else {
pre.right = null ;
root1 = root1.right;
}
}
if (root2.left == null ) {
root2 = root2.right;
}
else {
Node pre = root2.left;
while (pre.right != null
&& pre.right != root2)
pre = pre.right;
if (pre.right == null ) {
pre.right = root2;
root2 = root2.left;
}
else {
pre.right = null ;
root2 = root2.right;
}
}
}
return (root1 == null && root2 == null );
}
}
|
Python3
class Solution:
def isIdentical( self , root1, root2):
if not root1 and not root2:
return True
if not root1 or not root2:
return False
while root1 and root2:
if root1.data ! = root2.data:
return False
if not root1.left:
root1 = root1.right
else :
pre = root1.left
while pre.right and pre.right ! = root1:
pre = pre.right
if not pre.right:
pre.right = root1
root1 = root1.left
else :
pre.right = None
root1 = root1.right
if not root2.left:
root2 = root2.right
else :
pre = root2.left
while pre.right and pre.right ! = root2:
pre = pre.right
if not pre.right:
pre.right = root2
root2 = root2.left
else :
pre.right = None
root2 = root2.right
return not root1 and not root2
|
Time Complexity: O(N), where N is the number of nodes in the binary tree
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...