POTD Solutions | 1 Nov’ 23 | Frequencies of Limited Range Array Elements
Last Updated :
22 Nov, 2023
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Frequency Counting algorithm but will also help you build up problem-solving skills.
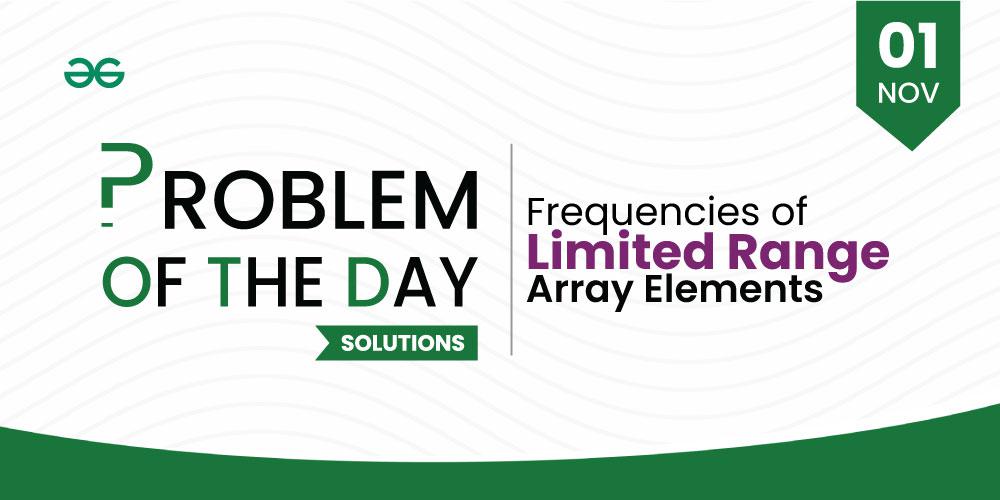
POTD 1st November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 1 November: Frequencies of Limited Range Array Elements:
Given an array arr[] of N positive integers which can contain integers from 1 to P where elements can be repeated or can be absent from the array. Your task is to count the frequency of all numbers from 1 to N. Make in-place changes in arr[], such that arr[i] = frequency(i). Assume 1-based indexing.
Note: The elements greater than N in the array can be ignored for counting and modify the array in-place.
Example:
Input:N = 5, arr[] = {2, 3, 2, 3, 5}, P = 5
Output: 0 2 2 0 1
Explanation: Counting frequencies of each array element. We have: 1 occurring 0 times, 2 occurring 2 times, 3 occurring 2 times, 4 occurring 0 times, 5 occurring 1 time.
Input:N = 4, arr[] = {3,3,3,3}, P = 3
Output: 0 0 4 0
Explanation: Counting frequencies of each array element. We have: 1 occurring 0 times, 2 occurring 0 times, 3 occurring 4 times, 4 occurring 0 times.
The idea is to traverse the given array, use elements as an index and store their counts at the index. Since the elements are positive so we will store the frequency as negative values. For a element at ith index, the frequency should be updated at index idx, where idx=arr[i]-1. If arr[idx] is less than 0 then it will decrement arr[idx] else, we will replace the element arr[i] with arr[idx] and then set arr[idx] to -1 so that element at arr[idx] is not lost.
Follow the steps to solve the above problem:
- Traverse the array from start to end.
- For each element check if the element is less than or equal to zero or not. If negative skip the element as it is the frequency. If the element is greater than N then also we will skip the element because no need to calculate its frequency.
- Let idx=arr[i]-1. Then we have 2 cases:
- If arr[idx] is negative, then it is not the first occurrence, we simply update arr[idx] to arr[idx]-1.
- If arr[idx] is positive, we will replace the element arr[i] with arr[idx] and then set arr[idx] to -1 so that element at arr[idx] is not lost.
- Traverse then array again. If the element at ith index is negative it means it represents a frequency so set negative elements to their absolute values else if the element is positive then it means the element i has not occurred, so set arr[i] to 0.
Below is the implementation of above approach:
C++
class Solution {
public :
void frequencyCount(vector< int >& arr, int N, int P)
{
int i = 0;
while (i < N) {
int idx = arr[i] - 1;
if (idx >= N || idx < 0) {
i++;
continue ;
}
if (arr[idx] <= 0) {
arr[idx]--;
i++;
}
else {
int st = arr[idx];
arr[idx] = -1;
if (idx > i) {
arr[i] = st;
}
else {
i++;
}
}
}
for ( int i = 0; i < N; i++) {
if (arr[i] < 0) {
arr[i] = -arr[i];
}
else {
arr[i] = 0;
}
}
}
};
|
Java
class Solution {
public static void frequencyCount( int arr[], int N,
int P)
{
int i = 0 ;
while (i < N) {
int idx = arr[i] - 1 ;
if (idx >= N || idx < 0 ) {
i++;
continue ;
}
if (arr[idx] <= 0 ) {
arr[idx]--;
i++;
}
else {
int st = arr[idx];
arr[idx] = - 1 ;
if (idx > i) {
arr[i] = st;
}
else {
i++;
}
}
}
for (i = 0 ; i < N; i++) {
if (arr[i] < 0 ) {
arr[i] = -arr[i];
}
else {
arr[i] = 0 ;
}
}
}
}
|
Python3
class Solution:
def frequencyCount( self , arr, N, P):
i = 0
while i < N:
idx = arr[i] - 1
if idx > = N or idx < 0 :
i + = 1
continue
if arr[idx] < = 0 :
arr[idx] - = 1
i + = 1
else :
st = arr[idx]
arr[idx] = - 1
if idx > i:
arr[i] = st
else :
i + = 1
for i in range (N):
if arr[i] < 0 :
arr[i] = - arr[i]
else :
arr[i] = 0
|
Time Complexity: O(N)
Auxilary Space: O(1)
Share your thoughts in the comments
Please Login to comment...