POTD Solutions | 09 Nov’ 23 | Predict the Column
Last Updated :
09 Jan, 2024
View all POTD Solutions
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Matrix but will also help you build up problem-solving skills.
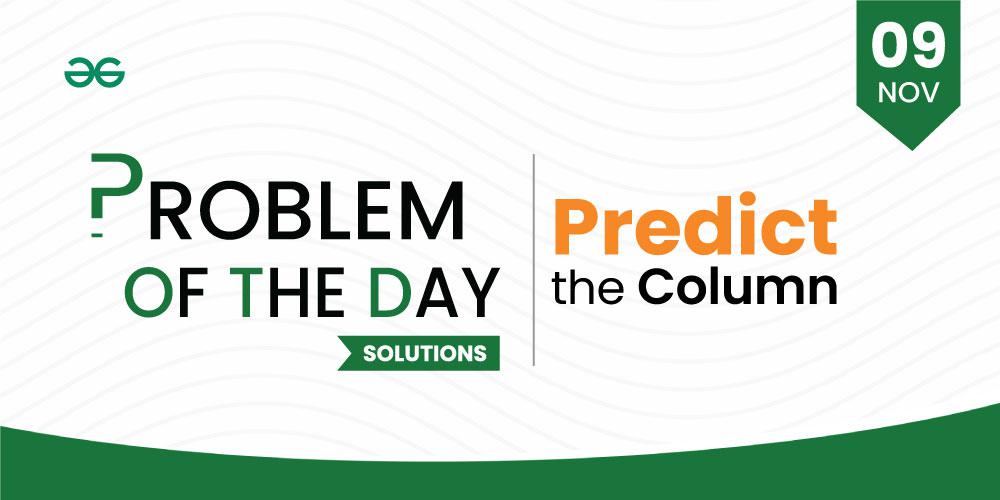
POTD Solutions 9 November 2023
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
C++
class Solution {
columnWithMaxZeros(arr, N) {
let max_count = 0;
let ans = -1;
for (let i = 0; i < N; i++) {
let count = 0;
for (let j = 0; j < N; j++) {
if (arr[j][i] === 0) {
count++;
}
}
if (count > max_count) {
max_count = count;
ans = i;
}
}
return ans;
}
}
|
Java
class Solution {
int columnWithMaxZeros( int arr[][], int N)
{
int idx = - 1 , val = 0 ;
for ( int j = 0 ; j < N; j++) {
int sum = 0 ;
for ( int i = 0 ; i < N; i++) {
sum += 1 - arr[i][j];
}
if (val < sum) {
val = sum;
idx = j;
}
}
return idx;
}
}
|
Python3
class Solution:
def columnWithMaxZeros( self , arr, N):
max_count = 0
ans = - 1
for i in range (N):
count = 0
for j in range (N):
if arr[j][i] = = 0 :
count + = 1
if count > max_count:
max_count = count
ans = i
return ans
|
C#
using System;
using System.Collections.Generic;
class Solution
{
public int ColumnWithMaxZeros(List<List< int >> arr, int N)
{
int maxCount = 0, columnIndexWithMaxZeros = -1;
for ( int i = 0; i < N; i++)
{
int count = 0;
for ( int j = 0; j < N; j++)
{
if (arr[j][i] == 0)
{
count++;
}
}
if (count > maxCount)
{
maxCount = count;
columnIndexWithMaxZeros = i;
}
}
return columnIndexWithMaxZeros;
}
}
|
Javascript
class Solution {
columnWithMaxZeros(arr, N) {
let maxCount = 0;
let ans = -1;
for (let i = 0; i < N; i++) {
let count = 0;
for (let j = 0; j < N; j++) {
if (arr[j][i] === 0) {
count++;
}
}
if (count > maxCount) {
maxCount = count;
ans = i;
}
}
return ans;
}
}
|
Time Complexity: O(N X N), where N is the number of rows or columns in the matrix
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...