Node Interview Questions and Answers (2024) – Advanced Level
Last Updated :
08 Feb, 2024
In this article, you will learn NodeJS interview questions and answers – Advanced level that are most frequently asked in interviews. Before proceeding to learn NodeJS interview questions and answers – advanced level, first learn the complete NodeJS Tutorial.
NodeJS is an open-source and cross-platform runtime environment built on Chrome’s V8 JavaScript engine for executing JavaScript code outside of a browser. You need to recollect that NodeJS isn’t a framework, and it’s not a programing language. It provides an event-driven, non-blocking (asynchronous) I/O and cross-platform runtime environment for building highly scalable server-side applications using JavaScript.
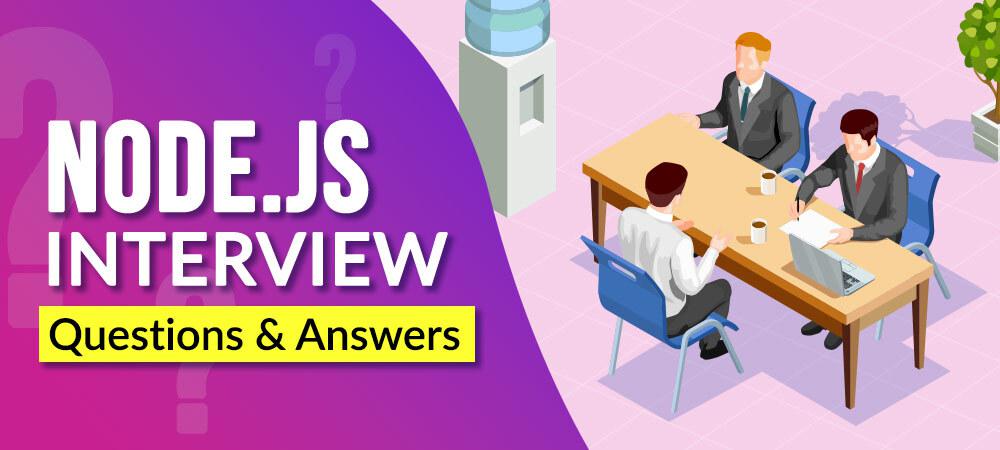
NodeJS Interview Questions & Answers
This set contains the advanced-level questions asked in the interview.
Pre-requisites:
Due to a single thread in node.js, it handles memory more efficiently because there are no multiple threads due to which no thread management is needed. Now, to handle workload efficiently and to take advantage of computer multi-core systems, cluster modules are created that provide us the way to make child processes that run simultaneously with a single parent process.
- Fork(): It creates a new child process from the master. The isMaster returns true if the current process is master or else false.
- isWorker: It returns true if the current process is a worker or else false.
- process: It returns the child process which is global.
- send(): It sends a message from worker to master or vice versa.
- kill(): It is used to kill the current worker.
Session management can be done in node.js by using the express-session module. It helps in saving the data in the key-value form. In this module, the session data is not saved in the cookie itself, just the session ID.
Types of Stream:
- Readable stream: It is the stream from where you can receive and read the data in an ordered fashion. However, you are not allowed to send anything. For example, fs.createReadStream() lets us read the contents of a file.
- Writable stream: It is the stream where you can send data in an ordered fashion but you are not allowed to receive it back. For example, fs.createWriteStream() lets us write data to a file.
- Duplex stream: It is the stream that is both readable and writable. Thus you can send in and receive data together. For example, net.Socket is a TCP socket.
- Transform stream: It is the stream that is used to modify the data or transform it as it is read. The transform stream is basically a duplex in nature. For example, zlib.createGzip stream is used to compress the data using gzip.
5. How can we implement authentication and authorization in Node.js?
Authentication is the process of verifying a user’s identity while authorization is determining what actions can be performed. We use packages like Passport and JWT to implement authentication and authorization.
The package used for file uploading in Node.js is Multer. The file can be uploaded to the server using this module. There are other modules in the market but Multer is very popular when it comes to file uploading. Multer is a node.js middleware that is used for handling multipart/form-data, which is a mostly used library for uploading files.
Node.js is the best choice for asynchronous programming Python is not the best choice for asynchronous programming. Node.js is best suited for small projects to enable functionality that needs less amount of scripting. Python is the best choice if you’re developing larger projects. Node.js is best suited for memory-intensive activities. Not recommended for memory-intensive activities. Node.js is a better option if your focus is exactly on web applications and website development. But, Python is an all-rounder and can perform multiple tasks like- web applications, integration with back-end applications, numerical computations, machine learning, and network programming. Node.js is an ideal and vibrant platform available right now to deal with real-time web applications. Python isn’t an ideal platform to deal with real-time web applications. The fastest speed and great performance are largely due to Node.js being based on Chrome’s V8 which is a very fast and powerful engine. Python is slower than Node.js, As Node.js is based on fast and powerful Chrome’s V8 engine, Node.js utilizes JavaScript interpreter. Python using PyPy as Interpreter. In case of error handling and debugging Python beats Node.js. Error handling in Python takes significantly very little time and debugging in Python is also very easy compared to Node.js.
8. How to handle database connection in Node.js?
To handle database connection in Node.js we use the driver for MySQL and libraries like Mongoose for connecting to the MongoDB database. These libraries provide methods to connect to the database and execute queries.
Command-line arguments (CLI) are strings of text used to pass additional information to a program when an application is running through the command line interface of an operating system. We can easily read these arguments by the global object in node i.e. process object. Below is the approach:
Step 1: Save a file as index.js and paste the below code inside the file.
Javascript
let arguments = process.argv ;
console.log(arguments) ;
|
Step 2: Run the index.js file using the below command:
node index.js
Redis is an Open Source store for storing data structures. It is used in multiple ways. It is used as a database, cache, and message broker. It can store data structures such as strings, hashes, sets, sorted sets, bitmaps, indexes, and streams. Redis is very useful for Node.js developers as it reduces the cache size which makes the application more efficient. However, it is very easy to integrate Redis with Node.js applications.
Web Socket is a protocol that provides full-duplex (multiway) communication i.e. allows communication in both directions simultaneously. It is a modern web technology in which there is a continuous connection between the user’s browser (client) and the server. In this type of communication, between the web server and the web browser, both of them can send messages to each other at any point in time. Traditionally on the web, we had a request/response format where a user sends an HTTP request and the server responds to that. This is still applicable in most cases, especially those using RESTful API. But a need was felt for the server to also communicate with the client, without getting polled(or requested) by the client. The server in itself should be able to send information to the client or the browser. This is where Web Socket comes into the picture.
The Util module in node.js provides access to various utility functions. There are various utility modules available in the node.js module library.
- OS Module: Operating System-based utility modules for node.js are provided by the OS module.
- Path Module: The path module in node.js is used for transforming and handling various file paths.
- DNS Module: DNS Module enables us to use the underlying Operating System name resolution functionalities. The actual DNS lookup is also performed by the DNS Module.
- Net Module: Net Module in node.js is used for the creation of both client and server. Similar to DNS Module this module also provides an asynchronous network wrapper.
We use process.env to handle environment variables in Node.js. We can specify environment configurations as well as keys in the .env file. To access the variable in the application, we use the “process.env.VARIABLE_NAME” syntax. To use it we have to install the dotenv package using the below command:
npm install dotenv
DNS is a node module used to do name resolution facility which is provided by the operating system as well as used to do an actual DNS lookup. Its main advantage is that there is no need for memorizing IP addresses – DNS servers provide a nifty solution for converting domain or subdomain names to IP addresses.
15. What are child processes in Node.js?
Usually, Node.js allows single-threaded, non-blocking performance but running a single thread in a CPU cannot handle increasing workload hence the child_process module can be used to spawn child processes. The child processes communicate with each other using a built-in messaging system.
Validation in node.js can be easily done by using the express-validator module. This module is popular for data validation. There are other modules available in the market like hapi/joi, etc but express-validator is widely used and popular among them.
17. What is the role of net module in Node.js?
The net module in Node.js is used to create TCP client and serve in Node.js. This module establishes connections, handles incoming requests, and share data over the network.
The Tracing Objects are used for a set of categories to enable and disable the tracing. When tracing events are created then tracing objects is disabled by calling tracing.enable() method and then categories are added to the set of enabled trace and can be accessed by calling tracing.categories.
Reactor Pattern is used to avoid the blocking of the Input/Output operations. It provides us with a handler that is associated with I/O operations. When the I/O requests are to be generated, they get submitted to a demultiplexer, which handles concurrency in avoiding the blocking of the I/O mode and collects the requests in the form of an event and queues those events.
To connect to MongoDB database write the following code after installing the Mongoose package
Javascript
const mongoose = require( "mongoose" );
mongoose.connect( "DATABASE_URL_HERE" , {
useNewUrlParser: true ,
useUnifiedTopology: true
});
|
Share your thoughts in the comments
Please Login to comment...