MongoDB was released in February 2009. It is an open-source document-oriented database and is classified as a NoSQL database. It follows the CAP theorem (Consistency Availability and Partition tolerance).
MongoDB stores the records in a document in BSON format. It is an unstructured language and provides horizontal scalability and high-performance, data persistence. It follows the BASE ( Basically Available, Soft State, and Eventual Consistency )properties.
In this article, We will learn about the View in MongoDB in detail along with its syntax, examples, and so on.
Views in MongoDB
In SQL, views are referred to as virtual tables, similarly in MongoDB views are referred to as virtual collections. Views in MongoDB are implemented using an aggregation pipeline. We can create a view using a field from one or more collections in the database.
A view can have all the documents or a specific document based on conditions. They don’t store the data but are used to represent the data.
MongoDB provides standard views and on-demand materialized views. Both types are implemented using an aggregation pipeline. View can contain fields from one or more collection.
Why are Views Useful?
View provides the specific data according to the aggregation pipeline. They are used to hide the important details of the documents and provide the necessary fields from the document. It is used to restrict access to certain fields from the document.
How do Views Relate to Data Aggregation?
Views in MongoDB are the virtual collection. Data aggregation is an important factor in creating a view. View represents the aggregated data from the collection.
The aggregation pipeline is used to aggregate the data.$match,$ group,$lookup, and many more operations are used to aggregate data.
Creating our First View
Views in MongoDB can be created using db.createCollection() or db.createView( ). To create a view, a collection with documents is required.
For creating a View in MongoDB. Let have some data into our collection on which we will perform operations.
Query:
use GeeksforGeeks; // Database in use
db.createCollection('Teacher'); .//create Collection
// Insert document in a collection
db.Teacher.insertMany([
{ name: "Anil", Age: 28, Salary: 25000, year: 2 },
{ name: "Sunil", Age: 35, Salary: 35000, year: 5 },
{ name: "Ajay", Age: 35, Salary: 45000, year: 10 },
{ name: "Amit", Age: 45, Salary: 60000, year: 12 },
]);
Now we will use createView() method contains view name,source collection name and aggregation pipeline. The find() method is used to query the view in MongoDB.
Syntax:
db.createView( "view_name" ,"source_collection_name" ,pipeline )
Query:
Create views representing a teacher with more than 7 years of experience.
db.createView("ExperiencedTeacher", "Teacher", [
{ $match: { year: { $gt: 7 } } },
]);
// To query the view.
db.ExperiencedTeacher.find();
Output:
-in--MongoDB.jpeg)
createView() in MongoDB
Explanation: Teacher collection contains some documents with name, Age, Salary and year fields. View is created using createView() method. View contains documents with year field with value more than 7 year experience. Here find() method is used to query the view.
Create a View Using createCollection() in MongDB
View are created using createCollection() method. The method contains view name, source collection and the aggregation pipeline and find() method.
Syntax:
db.createCollection(" view_name", {
viewOn: "source_collection_name",
pipeline
});
Query:
Example: Create views to represent a teacher with more than 7 years of experience.
db.createCollection("ExperiencedTeacher", {
viewOn: "Teacher",
pipeline: [{ $match: { year: { $gt: 7 } } }],
});
// To query the view.
db.ExperiencedTeacher.find();
Output:
-in-MongDB.jpeg)
Create a View using createCollection() in MongDB
Explanation: Teacher collection contains some documents with name,Age,Salary and year fields.View is created using createCollection() method .View contains documents with year field with value more than 7 year of experiences.
How to Open a View?
To “Open a view” implies to display the data within the view. The find() method is used to display the data within the view.
Syntax:
use database_name;
db.view_name.find();
How to Drop a View in MongoDB?
View are read only, standard view are not stored in the database. As the task is complete view are dropped. drop() method is used to drop the view.
Syntax:
db.view_name.drop();
Example: db.ExperiencedTeacher.drop();
How to Duplicate a View?
Duplicate view contains the same documents as the original view. Duplicate view is created using createView() method.
Syntax:
db.createView( "Duplicate_view_name" ," Original_view_name" ,[] );
Query:
Example: Create a duplicate view on ExperiencedTeacher view.
db.createView("Experience","ExperiencedTeacher", [] ) ;
Output:
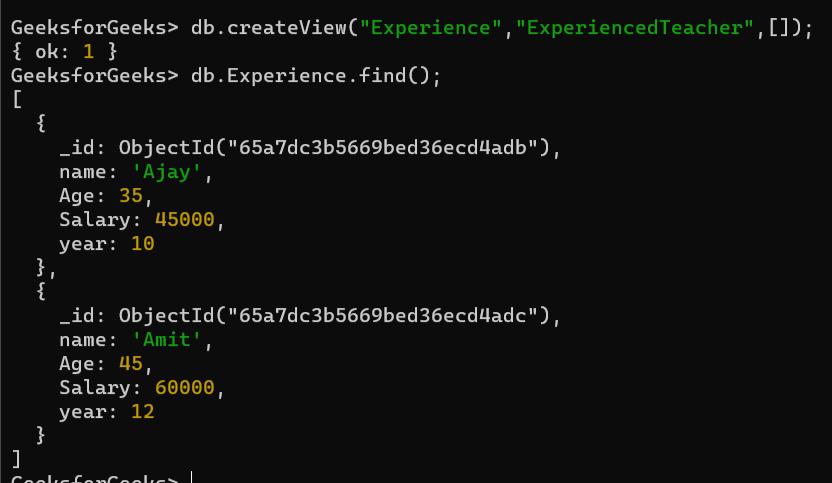
How to Duplicate View
Explanation: Duplicate View is created using createView() method. The createView method contains duplicate view name,original view name and the aggregation pipeline is empty.
Modify View in MongoDB- Method 1
Drop and Recreate the View
View cannot be modified directly using update() method. To modify the view it is dropped using drop() method and view is created again with new conditions.
Syntax:
db.view_name.drop();
db.createView( "view_name" ,"source_collection_name" ,pipeline_with_updated pipeline ,collation )
Query:
Example: Modify views to represent a teacher with less than 7 years of experience.
// Initial view
db.createView("ExperiencedTeacher", "Teacher", [
{ $match: { year: { $gt: 7 } } },
]);
// Drop and Recreate the View
db.ExperiencedTeacher.drop();
db.createView("ExperiencedTeacher", "Teacher", [
{ $match: { year: { $lt: 7 } } },
]);
Output:
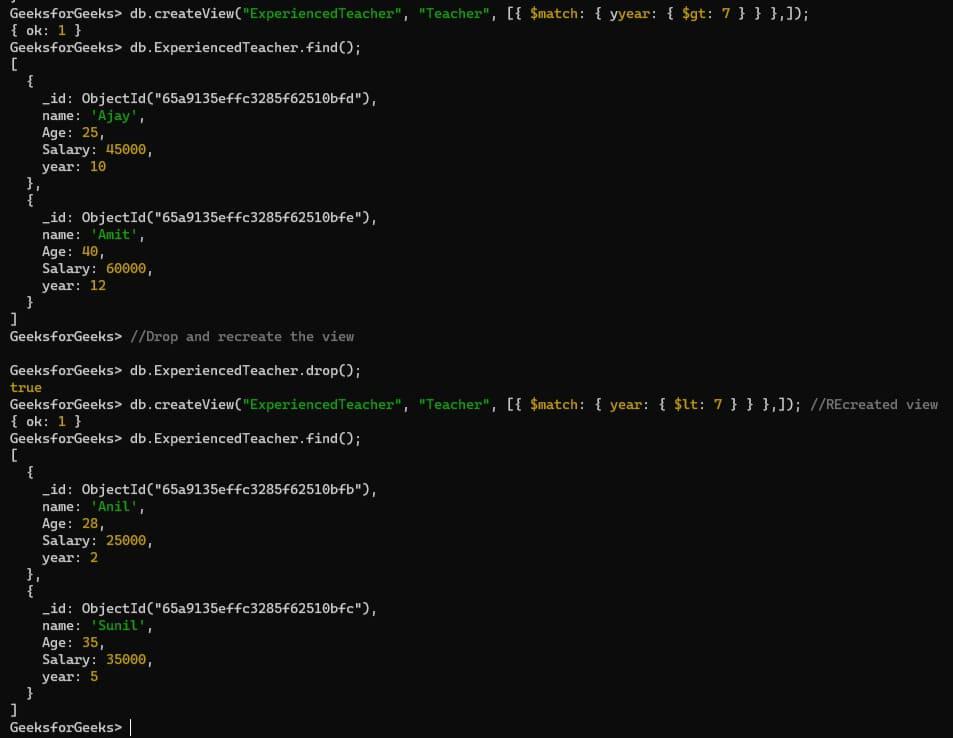
Modify View in MongoDB- Method 1
Explanation: Initially view is created which contains year field with value greater than 7. This view is dropped and new view is created using createView() method. This view contains year field with value less than 7.
Modify View in MongoDB Method- 2
View are modified using the collMod command. The runCommand() method is used to carry out the modification in the view.
Syntax:
db.runCommand( collMod : "View_name " , viewOn: "source_collection ",pipeline )
Explanation:
- runCommand() is used to carry out operations that are not included with CRUD operations.
- collMod is used to modify validation rules.
- viewOn is used to define the aggregation pipeline that define the view.
Query:
Example: Modify views to represent a teacher with less than 7 years of experience.
db.runCommand({
collMod: "ExperiencedTeacher",
viewOn: "Teacher",
pipeline: [{ $match: { year: { $gt: 7 } } }],
});
Output:
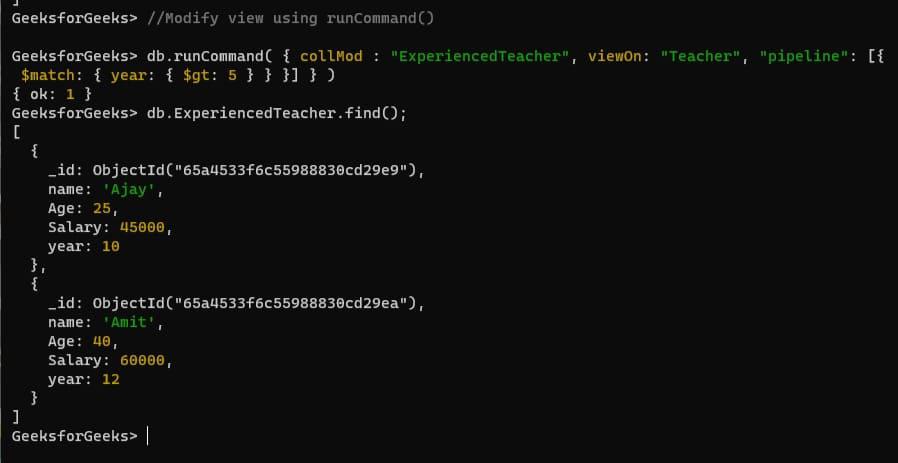
Modify View in MongoDB Method 2
Explanation: Initially view is created which contains year field with value greater than 7. This view is modified using collMod command. This view contains year field with value less than 7.
Use a View to Join Two Collections
View are used to join two collections .createView() is used to join the two collections.
Syntax:
db.createView(
"joined_view_name", // View name
"collection1_name", // Source collection 1
[
{
// Pipeline for collection 1
$lookup: {
from: "collection2_name", // Source collection 2
localField: "field_in_collection1",
foreignField: "field_in_collection2",
as: "joined_data",
},
},
// Additional pipeline
]
);
Query:
Example: Join two collections using Views.
//First Collection
db.createCollection("users");
// Insert in first collection
db.users.insertMany([
{ name: "Anil", age: 25 },
{ name: "Jay", age: 30 },
{ name: "Om", age: 22 },
]);
//Second Collection
db.createCollection("orders");
// Insert in second Collection
db.orders.insertMany([
{ user_id: 1, product: "Pen", quantity: 2 },
{ user_id: 2, product: "Pencil", quantity: 1 },
{ user_id: 3, product: "Sneaker", quantity: 3 },
]);
//Create View to join two collections.
db.orders.insertMany([
{ user_id: 1, product: "Pen", quantity: 2 },
{ user_id: 2, product: "Pencil", quantity: 1 },
{ user_id: 3, product: "Sneaker", quantity: 3 },
]);
db.createView("userOrders", "users", [
{
$lookup: {
from: "orders",
localField: "_id",
foreignField: "user_id",
as: "orders",
},
},
{ $unwind: { path: "$orders",preserveNullAndEmptyArrays:true } },
{
$project: {
_id: 1,
name: 1,
orderId: "$orders._id",
product: "$orders.product",
quantity: "$orders.quantity",
},
},
]);
Output:
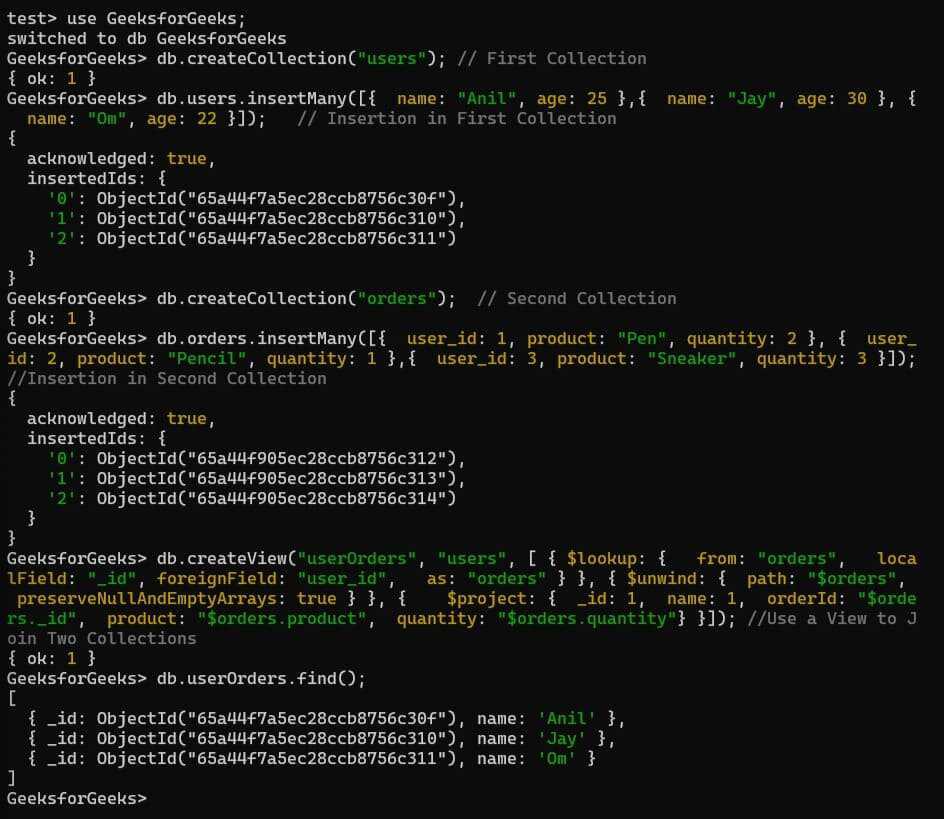
Use view to join two Collection in MongDB.
Explanation: users and orders collections are joined using the view.
Supported Operations in MongoDB View.
MongoDB supports various operations,here are the operations supported for view:
Database Commands
- collMod to modify the validation rule.
- count is used to count the document
- distinct provides the distinct documents.
- find provide document from the collection.
Mongosh Methods
- db.collection.aggregate() to specify the aggregation pipeline.
- db.collection.count() is used to provide the count of documents .This command is deprecated ,use next specified command.
- db.collection.countDocuments() is use to provide the count of documents.
- db.collection.distinct() provide the unique value.
- db.collection.find() return the document from collection.
- db.collection.findOne() returns only one document from the collection.
- db.createCollection() is used to create collection and view.
- db.createView() is used to create view.
Standard Views vs On-demand Materialized Views in MongoDB
They are read-only and are not stored on the disk
|
They are stored on a disk.
|
They use the indexes of the original collection.
|
An index can be directly created.
|
They require processing time to display views.
|
They are pre-computed hence offer fast retrieval
|
They don’t require maintenance
|
They require maintenance when there are updates in collection.
|
Conclusion
View are used to represent the data and they are not stored in database.They are used for abstraction,enforcing security and for restricting some fields from the document. The article covers detailed information about the view with suitable example. View in mongoDB are the virtual collection. They are used to represent the data.They are read only ,i.e any modification to it is not integrated with the original collection or database. Indexes of the original collections are used by View.
Share your thoughts in the comments
Please Login to comment...