Mobile Numeric Keypad Problem
Last Updated :
19 Sep, 2023
Given the mobile numeric keypad. You can only press buttons that are up, left, right or down to the current button. You are not allowed to press bottom row corner buttons (i.e. * and # ).
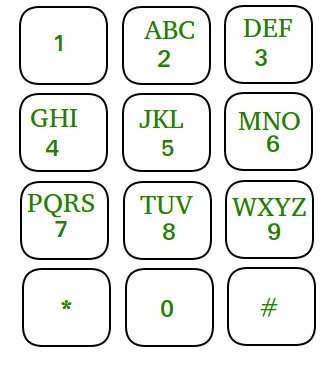
Given a number N, find out the number of possible numbers of the given length.
Examples:
For N=1, number of possible numbers would be 10 (0, 1, 2, 3, …., 9)
For N=2, number of possible numbers would be 36
Possible numbers: 00,08 11,12,14 22,21,23,25 and so on.
If we start with 0, valid numbers will be 00, 08 (count: 2)
If we start with 1, valid numbers will be 11, 12, 14 (count: 3)
If we start with 2, valid numbers will be 22, 21, 23,25 (count: 4)
If we start with 3, valid numbers will be 33, 32, 36 (count: 3)
If we start with 4, valid numbers will be 44,41,45,47 (count: 4)
If we start with 5, valid numbers will be 55,54,52,56,58 (count: 5)
....................................
....................................
We need to print the count of possible numbers.
N = 1 is trivial case, number of possible numbers would be 10 (0, 1, 2, 3, …., 9)
For N > 1, we need to start from some button, then move to any of the four direction (up, left, right or down) which takes to a valid button (should not go to *, #). Keep doing this until N length number is obtained (depth first traversal).
Recursive Solution:
Mobile Keypad is a rectangular grid of 4X3 (4 rows and 3 columns)
Lets say Count(i, j, N) represents the count of N length numbers starting from position (i, j)
If N = 1
Count(i, j, N) = 10
Else
Count(i, j, N) = Sum of all Count(r, c, N-1) where (r, c) is new
position after valid move of length 1 from current
position (i, j)
Following is the implementation of above recursive formula.
C++
#include <stdio.h>
int row[] = {0, 0, -1, 0, 1};
int col[] = {0, -1, 0, 1, 0};
int getCountUtil( char keypad[][3], int i, int j, int n)
{
if (keypad == NULL || n <= 0)
return 0;
if (n == 1)
return 1;
int k=0, move=0, ro=0, co=0, totalCount = 0;
for (move=0; move<5; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >=0 && co <= 2 &&
keypad[ro][co] != '*' && keypad[ro][co] != '#' )
{
totalCount += getCountUtil(keypad, ro, co, n-1);
}
}
return totalCount;
}
int getCount( char keypad[][3], int n)
{
if (keypad == NULL || n <= 0)
return 0;
if (n == 1)
return 10;
int i=0, j=0, totalCount = 0;
for (i=0; i<4; i++)
{
for (j=0; j<3; j++)
{
if (keypad[i][j] != '*' && keypad[i][j] != '#' )
{
totalCount += getCountUtil(keypad, i, j, n);
}
}
}
return totalCount;
}
int main( int argc, char *argv[])
{
char keypad[4][3] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
printf ( "Count for numbers of length %d: %dn \n" , 1, getCount(keypad, 1));
printf ( "Count for numbers of length %d: %dn \n" , 2, getCount(keypad, 2));
printf ( "Count for numbers of length %d: %dn \n" , 3, getCount(keypad, 3));
printf ( "Count for numbers of length %d: %dn \n" , 4, getCount(keypad, 4));
printf ( "Count for numbers of length %d: %dn" , 5, getCount(keypad, 5));
return 0;
}
|
Java
import java.util.*;
import java.io.*;
class GfG
{
static int row[] = { 0 , 0 , - 1 , 0 , 1 };
static int col[] = { 0 , - 1 , 0 , 1 , 0 };
static int getCountUtil( char keypad[][],
int i, int j, int n)
{
if (keypad == null || n <= 0 )
return 0 ;
if (n == 1 )
return 1 ;
int k = 0 , move = 0 , ro = 0 , co = 0 , totalCount = 0 ;
for (move= 0 ; move< 5 ; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >= 0 && co <= 2 &&
keypad[ro][co] != '*' && keypad[ro][co] != '#' )
{
totalCount += getCountUtil(keypad, ro, co, n - 1 );
}
}
return totalCount;
}
static int getCount( char keypad[][], int n)
{
if (keypad == null || n <= 0 )
return 0 ;
if (n == 1 )
return 10 ;
int i = 0 , j = 0 , totalCount = 0 ;
for (i = 0 ; i < 4 ; i++)
{
for (j= 0 ; j< 3 ; j++)
{
if (keypad[i][j] != '*' && keypad[i][j] != '#' )
{
totalCount += getCountUtil(keypad, i, j, n);
}
}
}
return totalCount;
}
public static void main(String[] args)
{
char keypad[][] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
System.out.printf( "Count for numbers of" +
" length %d: %d" , 1 , getCount(keypad, 1 ));
System.out.printf( "\nCount for numbers of" +
" length %d: %d" , 2 , getCount(keypad, 2 ));
System.out.printf( "\nCount for numbers of" +
" length %d: %d" , 3 , getCount(keypad, 3 ));
System.out.printf( "\nCount for numbers of" +
" length %d: %d" , 4 , getCount(keypad, 4 ));
System.out.printf( "\nCount for numbers of" +
" length %d: %d" , 5 , getCount(keypad, 5 ));
}
}
|
Python3
row = [ 0 , 0 , - 1 , 0 , 1 ]
col = [ 0 , - 1 , 0 , 1 , 0 ]
def getCountUtil(keypad, i, j, n):
if (keypad = = None or n < = 0 ):
return 0
if (n = = 1 ):
return 1
k = 0
move = 0
ro = 0
co = 0
totalCount = 0
for move in range ( 5 ):
ro = i + row[move]
co = j + col[move]
if (ro > = 0 and ro < = 3 and co > = 0 and co < = 2 and
keypad[ro][co] ! = '*' and keypad[ro][co] ! = '#' ):
totalCount + = getCountUtil(keypad, ro, co, n - 1 )
return totalCount
def getCount(keypad, n):
if (keypad = = None or n < = 0 ):
return 0
if (n = = 1 ):
return 10
i = 0
j = 0
totalCount = 0
for i in range ( 4 ):
for j in range ( 3 ):
if (keypad[i][j] ! = '*' and keypad[i][j] ! = '#' ):
totalCount + = getCountUtil(keypad, i, j, n)
return totalCount
keypad = [[ '1' , '2' , '3' ],
[ '4' , '5' , '6' ],
[ '7' , '8' , '9' ],
[ '*' , '0' , '#' ]]
print ( "Count for numbers of length 1:" , getCount(keypad, 1 ))
print ( "Count for numbers of length 2:" , getCount(keypad, 2 ))
print ( "Count for numbers of length 3:" , getCount(keypad, 3 ))
print ( "Count for numbers of length 4:" , getCount(keypad, 4 ))
print ( "Count for numbers of length 5:" , getCount(keypad, 5 ))
|
C#
using System;
class GfG
{
static int []row = {0, 0, -1, 0, 1};
static int []col = {0, -1, 0, 1, 0};
static int getCountUtil( char [,]keypad,
int i, int j, int n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 1;
int k = 0, move = 0, ro = 0, co = 0, totalCount = 0;
for (move=0; move<5; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >=0 && co <= 2 &&
keypad[ro,co] != '*' && keypad[ro,co] != '#' )
{
totalCount += getCountUtil(keypad, ro, co, n - 1);
}
}
return totalCount;
}
static int getCount( char [,]keypad, int n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 10;
int i = 0, j = 0, totalCount = 0;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 3; j++)
{
if (keypad[i, j] != '*' && keypad[i, j] != '#' )
{
totalCount += getCountUtil(keypad, i, j, n);
}
}
}
return totalCount;
}
public static void Main()
{
char [,]keypad = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
Console.Write( "Count for numbers of" +
" length {0}: {1}" , 1, getCount(keypad, 1));
Console.Write( "\nCount for numbers of" +
"length {0}: {1}" , 2, getCount(keypad, 2));
Console.Write( "\nCount for numbers of" +
"length {0}: {1}" , 3, getCount(keypad, 3));
Console.Write( "\nCount for numbers of" +
"length {0}: {1}" , 4, getCount(keypad, 4));
Console.Write( "\nCount for numbers of" +
"length {0}: {1}" , 5, getCount(keypad, 5));
}
}
|
Javascript
<script>
let row=[0, 0, -1, 0, 1];
let col=[0, -1, 0, 1, 0];
function getCountUtil(keypad,i,j,n)
{
if (keypad == null || n <= 0)
{ return 0;}
if (n == 1)
return 1;
let k = 0, move = 0, ro = 0, co = 0, totalCount = 0;
for (move=0; move<5; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >=0 && co <= 2 &&
keypad[ro][co] != '*' && keypad[ro][co] != '#' )
{
totalCount += getCountUtil(keypad, ro, co, n - 1);
}
}
return totalCount;
}
function getCount(keypad,n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 10;
let i = 0, j = 0, totalCount = 0;
for (i = 0; i < 4; i++)
{
for (j=0; j<3; j++)
{
if (keypad[i][j] != '*' && keypad[i][j] != '#' )
{
totalCount += getCountUtil(keypad, i, j, n);
}
}
}
return totalCount;
}
let keypad=[[ '1' , '2' , '3' ],[ '4' , '5' , '6' ],[ '7' , '8' , '9' ],[ '*' , '0' , '#' ]];
document.write( "Count for numbers of" +
" length " , 1, ": " , getCount(keypad, 1));
document.write( "<br>Count for numbers of" +
"length " , 2, ": " , getCount(keypad, 2));
document.write( "<br>Count for numbers of" +
"length " , 3, ": " , getCount(keypad, 3));
document.write( "<br>Count for numbers of" +
"length " , 4, ": " , getCount(keypad, 4));
document.write( "<br>Count for numbers of" +
"length " , 5, ": " , getCount(keypad, 5));
</script>
|
PHP
<?php
function getCountUtil( $keypad ,
$i , $j , $n )
{
static $row = array (0,0,-1,0,1);
static $col = array (0,-1,0,1,0);
if ( $keypad == null || $n <= 0)
return 0;
if ( $n == 1)
return 1;
$k = 0; $move = 0; $ro = 0; $co = 0; $totalCount = 0;
for ( $move = 0; $move < 5; $move ++)
{
$ro = $i + $row [ $move ];
$co = $j + $col [ $move ];
if ( $ro >= 0 && $ro <= 3 && $co >=0 && $co <= 2 &&
$keypad [ $ro ][ $co ] != '*' && $keypad [ $ro ][ $co ] != '#' )
{
$totalCount += getCountUtil( $keypad , $ro , $co , $n - 1);
}
}
return $totalCount ;
}
function getCount( $keypad , $n )
{
if ( $keypad == null || $n <= 0)
return 0;
if ( $n == 1)
return 10;
$i = 0; $j = 0; $totalCount = 0;
for ( $i = 0; $i < 4; $i ++)
{
for ( $j = 0; $j < 3; $j ++)
{
if ( $keypad [ $i ][ $j ] != '*' && $keypad [ $i ][ $j ] != '#' )
{
$totalCount += getCountUtil( $keypad , $i , $j , $n );
}
}
}
return $totalCount ;
}
{
$keypad = array ( array ( '1' , '2' , '3' ),
array ( '4' , '5' , '6' ),
array ( '7' , '8' , '9' ),
array ( '*' , '0' , '#' ));
echo ( "Count for numbers of" . " length" . getCount( $keypad , 1));
echo ( "\nCount for numbers of" .
" length " . getCount( $keypad , 2));
echo ( "\nCount for numbers of" .
" length " .getCount( $keypad , 3));
echo ( "\nCount for numbers of" .
" length " .getCount( $keypad , 4));
echo ( "\nCount for numbers of" .
" length " .getCount( $keypad , 5));
}
|
Output
Count for numbers of length 1: 10n
Count for numbers of length 2: 36n
Count for numbers of length 3: 138n
Count for numbers of length 4: 532n
Count for numbers of length 5: 2062n
Time Complexity: O(n*m) where n and m are row and column of keypad .
Auxiliary Space: O(n*m) where n and m are row and column of keypad .
Dynamic Programming
There are many repeated traversal on smaller paths (traversal for smaller N) to find all possible longer paths (traversal for bigger N). See following two diagrams for example. In this traversal, for N = 4 from two starting positions (buttons ‘4’ and ‘8’), we can see there are few repeated traversals for N = 2 (e.g. 4 -> 1, 6 -> 3, 8 -> 9, 8 -> 7 etc).
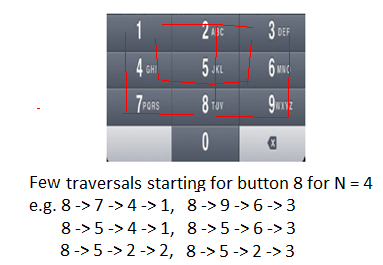
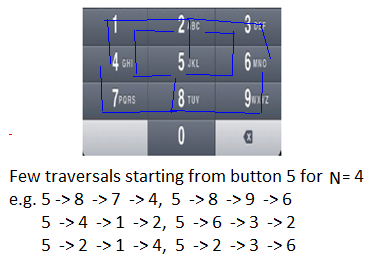
Since the problem has both properties: Optimal Substructure and Overlapping Subproblems, it can be efficiently solved using dynamic programming.
Following is the program for dynamic programming implementation.
C++
#include <stdio.h>
int getCount( char keypad[][3], int n)
{
if (keypad == NULL || n <= 0)
return 0;
if (n == 1)
return 10;
int row[] = {0, 0, -1, 0, 1};
int col[] = {0, -1, 0, 1, 0};
int count[10][n+1];
int i=0, j=0, k=0, move=0, ro=0, co=0, num = 0;
int nextNum=0, totalCount = 0;
for (i=0; i<=9; i++)
{
count[i][0] = 0;
count[i][1] = 1;
}
for (k=2; k<=n; k++)
{
for (i=0; i<4; i++)
{
for (j=0; j<3; j++)
{
if (keypad[i][j] != '*' && keypad[i][j] != '#' )
{
num = keypad[i][j] - '0' ;
count[num][k] = 0;
for (move=0; move<5; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >=0 && co <= 2 &&
keypad[ro][co] != '*' && keypad[ro][co] != '#' )
{
nextNum = keypad[ro][co] - '0' ;
count[num][k] += count[nextNum][k-1];
}
}
}
}
}
}
totalCount = 0;
for (i=0; i<=9; i++)
totalCount += count[i][n];
return totalCount;
}
int main( int argc, char *argv[])
{
char keypad[4][3] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
printf ( "Count for numbers of length %d: %dn" , 1, getCount(keypad, 1));
printf ( "\nCount for numbers of length %d: %dn" , 2, getCount(keypad, 2));
printf ( "\nCount for numbers of length %d: %dn" , 3, getCount(keypad, 3));
printf ( "\nCount for numbers of length %d: %dn" , 4, getCount(keypad, 4));
printf ( "\nCount for numbers of length %d: %dn" , 5, getCount(keypad, 5));
return 0;
}
|
Java
import java.util.*;
import java.io.*;
class GFG
{
static int getCount( char keypad[][], int n)
{
if (keypad == null || n <= 0 )
return 0 ;
if (n == 1 )
return 10 ;
int row[] = { 0 , 0 , - 1 , 0 , 1 };
int col[] = { 0 , - 1 , 0 , 1 , 0 };
int [][]count = new int [ 10 ][n + 1 ];
int i = 0 , j = 0 , k = 0 , move = 0 ,
ro = 0 , co = 0 , num = 0 ;
int nextNum = 0 , totalCount = 0 ;
for (i = 0 ; i <= 9 ; i++)
{
count[i][ 0 ] = 0 ;
count[i][ 1 ] = 1 ;
}
for (k = 2 ; k <= n; k++)
{
for (i = 0 ; i < 4 ; i++)
{
for (j = 0 ; j < 3 ; j++)
{
if (keypad[i][j] != '*' &&
keypad[i][j] != '#' )
{
num = keypad[i][j] - '0' ;
count[num][k] = 0 ;
for (move = 0 ; move < 5 ; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >= 0 &&
co <= 2 && keypad[ro][co] != '*' &&
keypad[ro][co] != '#' )
{
nextNum = keypad[ro][co] - '0' ;
count[num][k] += count[nextNum][k - 1 ];
}
}
}
}
}
}
totalCount = 0 ;
for (i = 0 ; i <= 9 ; i++)
totalCount += count[i][n];
return totalCount;
}
public static void main(String[] args)
{
char keypad[][] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
System.out.printf( "Count for numbers of length %d: %d\n" , 1 ,
getCount(keypad, 1 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 2 ,
getCount(keypad, 2 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 3 ,
getCount(keypad, 3 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 4 ,
getCount(keypad, 4 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 5 ,
getCount(keypad, 5 ));
}
}
|
Python3
def getCount(keypad, n):
if (keypad = = None or n < = 0 ):
return 0
if (n = = 1 ):
return 10
row = [ 0 , 0 , - 1 , 0 , 1 ]
col = [ 0 , - 1 , 0 , 1 , 0 ]
count = [[ 0 ] * (n + 1 )] * 10
i = 0
j = 0
k = 0
move = 0
ro = 0
co = 0
num = 0
nextNum = 0
totalCount = 0
for i in range ( 10 ):
count[i][ 0 ] = 0
count[i][ 1 ] = 1
for k in range ( 2 , n + 1 ):
for i in range ( 4 ):
for j in range ( 3 ):
if (keypad[i][j] ! = '*' and keypad[i][j] ! = '#' ):
num = ord (keypad[i][j]) - 48
count[num][k] = 0
for move in range ( 5 ):
ro = i + row[move]
co = j + col[move]
if (ro > = 0 and ro < = 3 and co > = 0 and co < = 2 and
keypad[ro][co] ! = '*' and keypad[ro][co] ! = '#' ):
nextNum = ord (keypad[ro][co]) - 48
count[num][k] + = count[nextNum][k - 1 ]
totalCount = 0
for i in range ( 10 ):
totalCount + = count[i][n]
return totalCount
if __name__ = = "__main__" :
keypad = [[ '1' , '2' , '3' ],
[ '4' , '5' , '6' ],
[ '7' , '8' , '9' ],
[ '*' , '0' , '#' ]]
print ( "Count for numbers of length" , 1 , ":" , getCount(keypad, 1 ))
print ( "Count for numbers of length" , 2 , ":" , getCount(keypad, 2 ))
print ( "Count for numbers of length" , 3 , ":" , getCount(keypad, 3 ))
print ( "Count for numbers of length" , 4 , ":" , getCount(keypad, 4 ))
print ( "Count for numbers of length" , 5 , ":" , getCount(keypad, 5 ))
|
C#
using System;
class GFG
{
static int getCount( char [,]keypad, int n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 10;
int []row = {0, 0, -1, 0, 1};
int []col = {0, -1, 0, 1, 0};
int [,]count = new int [10,n + 1];
int i = 0, j = 0, k = 0, move = 0,
ro = 0, co = 0, num = 0;
int nextNum = 0, totalCount = 0;
for (i = 0; i <= 9; i++)
{
count[i, 0] = 0;
count[i, 1] = 1;
}
for (k = 2; k <= n; k++)
{
for (i = 0; i < 4; i++)
{
for (j = 0; j < 3; j++)
{
if (keypad[i, j] != '*' &&
keypad[i, j] != '#' )
{
num = keypad[i, j] - '0' ;
count[num, k] = 0;
for (move = 0; move < 5; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >= 0 &&
co <= 2 && keypad[ro, co] != '*' &&
keypad[ro, co] != '#' )
{
nextNum = keypad[ro, co] - '0' ;
count[num, k] += count[nextNum, k - 1];
}
}
}
}
}
}
totalCount = 0;
for (i = 0; i <= 9; i++)
totalCount += count[i, n];
return totalCount;
}
public static void Main(String[] args)
{
char [,]keypad = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
Console.Write( "Count for numbers of.Length {0}: {1}\n" , 1,
getCount(keypad, 1));
Console.Write( "Count for numbers of.Length {0}: {1}\n" , 2,
getCount(keypad, 2));
Console.Write( "Count for numbers of.Length {0}: {1}\n" , 3,
getCount(keypad, 3));
Console.Write( "Count for numbers of.Length {0}: {1}\n" , 4,
getCount(keypad, 4));
Console.Write( "Count for numbers of.Length {0}: {1}\n" , 5,
getCount(keypad, 5));
}
}
|
Javascript
<script>
function getCount(keypad, n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 10;
let row = [ 0, 0, -1, 0, 1 ];
let col = [ 0, -1, 0, 1, 0 ];
let count = new Array(10);
for (let i = 0; i < 10; i++)
{
count[i] = new Array(n + 1);
for (let j = 0; j < n + 1; j++)
{
count[i][j] = 0;
}
}
let i = 0, j = 0, k = 0, move = 0,
ro = 0, co = 0, num = 0;
let nextNum = 0, totalCount = 0;
for (i = 0; i <= 9; i++)
{
count[i][0] = 0;
count[i][1] = 1;
}
for (k = 2; k <= n; k++)
{
for (i = 0; i < 4; i++)
{
for (j = 0; j < 3; j++)
{
if (keypad[i][j] != '*' &&
keypad[i][j] != '#' )
{
num = keypad[i][j].charCodeAt(0) -
'0' .charCodeAt(0);
count[num][k] = 0;
for (move = 0; move < 5; move++)
{
ro = i + row[move];
co = j + col[move];
if (ro >= 0 && ro <= 3 && co >= 0 &&
co <= 2 && keypad[ro][co] != '*' &&
keypad[ro][co] != '#' )
{
nextNum = keypad[ro][co].charCodeAt(0) -
'0' .charCodeAt(0);
count[num][k] += count[nextNum][k - 1];
}
}
}
}
}
}
totalCount = 0;
for (i = 0; i <= 9; i++)
totalCount += count[i][n];
return totalCount;
}
let keypad = [ [ '1' , '2' , '3' ],
[ '4' , '5' , '6' ],
[ '7' , '8' , '9' ],
[ '*' , '0' , '#' ] ];
document.write( "Count for numbers of length " +
1 + " : " + getCount(keypad, 1) + "<br>" )
document.write( "Count for numbers of length " +
2 + " : " + getCount(keypad, 2) + "<br>" )
document.write( "Count for numbers of length " +
3 + " : " + getCount(keypad, 3) + "<br>" )
document.write( "Count for numbers of length " +
4 + " : " + getCount(keypad, 4) + "<br>" )
document.write( "Count for numbers of length " +
5 + " : " + getCount(keypad, 5) + "<br>" )
</script>
|
Output
Count for numbers of length 1: 10n
Count for numbers of length 2: 36n
Count for numbers of length 3: 138n
Count for numbers of length 4: 532n
Count for numbers of length 5: 2062n
A Space Optimized Solution:
The above dynamic programming approach also runs in O(n) time and requires O(n) auxiliary space, as only one for loop runs n times, other for loops runs for constant time. We can see that nth iteration needs data from (n-1)th iteration only, so we need not keep the data from older iterations. We can have a space efficient dynamic programming approach with just two arrays of size 10. Thanks to Nik for suggesting this solution.
C++
#include <bits/stdc++.h>
using namespace std;
int getCount( char keypad[][3], int n)
{
if (keypad == NULL || n <= 0)
return 0;
if (n == 1)
return 10;
int odd[10], even[10];
int i = 0, j = 0, useOdd = 0, totalCount = 0;
for (i = 0; i <= 9; i++)
odd[i] = 1;
for (j = 2; j <= n; j++)
{
useOdd = 1 - useOdd;
if (useOdd == 1)
{
even[0] = odd[0] + odd[8];
even[1] = odd[1] + odd[2] + odd[4];
even[2] = odd[2] + odd[1] + odd[3] + odd[5];
even[3] = odd[3] + odd[2] + odd[6];
even[4] = odd[4] + odd[1] + odd[5] + odd[7];
even[5] = odd[5] + odd[2] + odd[4] + odd[8] + odd[6];
even[6] = odd[6] + odd[3] + odd[5] + odd[9];
even[7] = odd[7] + odd[4] + odd[8];
even[8] = odd[8] + odd[0] + odd[5] + odd[7] + odd[9];
even[9] = odd[9] + odd[6] + odd[8];
}
else
{
odd[0] = even[0] + even[8];
odd[1] = even[1] + even[2] + even[4];
odd[2] = even[2] + even[1] + even[3] + even[5];
odd[3] = even[3] + even[2] + even[6];
odd[4] = even[4] + even[1] + even[5] + even[7];
odd[5] = even[5] + even[2] + even[4] + even[8] + even[6];
odd[6] = even[6] + even[3] + even[5] + even[9];
odd[7] = even[7] + even[4] + even[8];
odd[8] = even[8] + even[0] + even[5] + even[7] + even[9];
odd[9] = even[9] + even[6] + even[8];
}
}
totalCount = 0;
if (useOdd == 1)
{
for (i = 0; i <= 9; i++)
totalCount += even[i];
}
else
{
for (i = 0; i <= 9; i++)
totalCount += odd[i];
}
return totalCount;
}
int main()
{
char keypad[4][3] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
cout << "Count for numbers of length 1: " << getCount(keypad, 1) << endl;
cout << "Count for numbers of length 2: " << getCount(keypad, 2) << endl;
cout << "Count for numbers of length 3: " << getCount(keypad, 3) << endl;
cout << "Count for numbers of length 4: " << getCount(keypad, 4) << endl;
cout << "Count for numbers of length 5: " << getCount(keypad, 5) << endl;
return 0;
}
|
C
#include <stdio.h>
int getCount( char keypad[][3], int n)
{
if (keypad == NULL || n <= 0)
return 0;
if (n == 1)
return 10;
int odd[10], even[10];
int i = 0, j = 0, useOdd = 0, totalCount = 0;
for (i=0; i<=9; i++)
odd[i] = 1;
for (j=2; j<=n; j++)
{
useOdd = 1 - useOdd;
if (useOdd == 1)
{
even[0] = odd[0] + odd[8];
even[1] = odd[1] + odd[2] + odd[4];
even[2] = odd[2] + odd[1] + odd[3] + odd[5];
even[3] = odd[3] + odd[2] + odd[6];
even[4] = odd[4] + odd[1] + odd[5] + odd[7];
even[5] = odd[5] + odd[2] + odd[4] + odd[8] + odd[6];
even[6] = odd[6] + odd[3] + odd[5] + odd[9];
even[7] = odd[7] + odd[4] + odd[8];
even[8] = odd[8] + odd[0] + odd[5] + odd[7] + odd[9];
even[9] = odd[9] + odd[6] + odd[8];
}
else
{
odd[0] = even[0] + even[8];
odd[1] = even[1] + even[2] + even[4];
odd[2] = even[2] + even[1] + even[3] + even[5];
odd[3] = even[3] + even[2] + even[6];
odd[4] = even[4] + even[1] + even[5] + even[7];
odd[5] = even[5] + even[2] + even[4] + even[8] + even[6];
odd[6] = even[6] + even[3] + even[5] + even[9];
odd[7] = even[7] + even[4] + even[8];
odd[8] = even[8] + even[0] + even[5] + even[7] + even[9];
odd[9] = even[9] + even[6] + even[8];
}
}
totalCount = 0;
if (useOdd == 1)
{
for (i=0; i<=9; i++)
totalCount += even[i];
}
else
{
for (i=0; i<=9; i++)
totalCount += odd[i];
}
return totalCount;
}
int main()
{
char keypad[4][3] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }
};
printf ( "Count for numbers of length %d: %dn" , 1, getCount(keypad, 1));
printf ( "Count for numbers of length %d: %dn" , 2, getCount(keypad, 2));
printf ( "Count for numbers of length %d: %dn" , 3, getCount(keypad, 3));
printf ( "Count for numbers of length %d: %dn" , 4, getCount(keypad, 4));
printf ( "Count for numbers of length %d: %dn" , 5, getCount(keypad, 5));
return 0;
}
|
Java
import java.util.*;
import java.io.*;
class GFG
{
static int getCount( char keypad[][], int n)
{
if (keypad == null || n <= 0 )
return 0 ;
if (n == 1 )
return 10 ;
int []odd = new int [ 10 ];
int []even = new int [ 10 ];
int i = 0 , j = 0 , useOdd = 0 , totalCount = 0 ;
for (i = 0 ; i <= 9 ; i++)
odd[i] = 1 ;
for (j = 2 ; j <= n; j++)
{
useOdd = 1 - useOdd;
if (useOdd == 1 )
{
even[ 0 ] = odd[ 0 ] + odd[ 8 ];
even[ 1 ] = odd[ 1 ] + odd[ 2 ] + odd[ 4 ];
even[ 2 ] = odd[ 2 ] + odd[ 1 ] +
odd[ 3 ] + odd[ 5 ];
even[ 3 ] = odd[ 3 ] + odd[ 2 ] + odd[ 6 ];
even[ 4 ] = odd[ 4 ] + odd[ 1 ] +
odd[ 5 ] + odd[ 7 ];
even[ 5 ] = odd[ 5 ] + odd[ 2 ] + odd[ 4 ] +
odd[ 8 ] + odd[ 6 ];
even[ 6 ] = odd[ 6 ] + odd[ 3 ] +
odd[ 5 ] + odd[ 9 ];
even[ 7 ] = odd[ 7 ] + odd[ 4 ] + odd[ 8 ];
even[ 8 ] = odd[ 8 ] + odd[ 0 ] + odd[ 5 ] +
odd[ 7 ] + odd[ 9 ];
even[ 9 ] = odd[ 9 ] + odd[ 6 ] + odd[ 8 ];
}
else
{
odd[ 0 ] = even[ 0 ] + even[ 8 ];
odd[ 1 ] = even[ 1 ] + even[ 2 ] + even[ 4 ];
odd[ 2 ] = even[ 2 ] + even[ 1 ] +
even[ 3 ] + even[ 5 ];
odd[ 3 ] = even[ 3 ] + even[ 2 ] + even[ 6 ];
odd[ 4 ] = even[ 4 ] + even[ 1 ] +
even[ 5 ] + even[ 7 ];
odd[ 5 ] = even[ 5 ] + even[ 2 ] + even[ 4 ] +
even[ 8 ] + even[ 6 ];
odd[ 6 ] = even[ 6 ] + even[ 3 ] +
even[ 5 ] + even[ 9 ];
odd[ 7 ] = even[ 7 ] + even[ 4 ] + even[ 8 ];
odd[ 8 ] = even[ 8 ] + even[ 0 ] + even[ 5 ] +
even[ 7 ] + even[ 9 ];
odd[ 9 ] = even[ 9 ] + even[ 6 ] + even[ 8 ];
}
}
totalCount = 0 ;
if (useOdd == 1 )
{
for (i = 0 ; i <= 9 ; i++)
totalCount += even[i];
}
else
{
for (i = 0 ; i <= 9 ; i++)
totalCount += odd[i];
}
return totalCount;
}
public static void main(String[] args)
{
char keypad[][] = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
System.out.printf( "Count for numbers of length %d: %d\n" , 1 ,
getCount(keypad, 1 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 2 ,
getCount(keypad, 2 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 3 ,
getCount(keypad, 3 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 4 ,
getCount(keypad, 4 ));
System.out.printf( "Count for numbers of length %d: %d\n" , 5 ,
getCount(keypad, 5 ));
}
}
|
Python3
def getCount(keypad, n):
if ( not keypad or n < = 0 ):
return 0
if (n = = 1 ):
return 10
odd = [ 0 ] * 10
even = [ 0 ] * 10
i = 0
j = 0
useOdd = 0
totalCount = 0
for i in range ( 10 ):
odd[i] = 1
for j in range ( 2 ,n + 1 ):
useOdd = 1 - useOdd
if (useOdd = = 1 ):
even[ 0 ] = odd[ 0 ] + odd[ 8 ]
even[ 1 ] = odd[ 1 ] + odd[ 2 ] + odd[ 4 ]
even[ 2 ] = odd[ 2 ] + odd[ 1 ] + odd[ 3 ] + odd[ 5 ]
even[ 3 ] = odd[ 3 ] + odd[ 2 ] + odd[ 6 ]
even[ 4 ] = odd[ 4 ] + odd[ 1 ] + odd[ 5 ] + odd[ 7 ]
even[ 5 ] = odd[ 5 ] + odd[ 2 ] + odd[ 4 ] + odd[ 8 ] + odd[ 6 ]
even[ 6 ] = odd[ 6 ] + odd[ 3 ] + odd[ 5 ] + odd[ 9 ]
even[ 7 ] = odd[ 7 ] + odd[ 4 ] + odd[ 8 ]
even[ 8 ] = odd[ 8 ] + odd[ 0 ] + odd[ 5 ] + odd[ 7 ] + odd[ 9 ]
even[ 9 ] = odd[ 9 ] + odd[ 6 ] + odd[ 8 ]
else :
odd[ 0 ] = even[ 0 ] + even[ 8 ]
odd[ 1 ] = even[ 1 ] + even[ 2 ] + even[ 4 ]
odd[ 2 ] = even[ 2 ] + even[ 1 ] + even[ 3 ] + even[ 5 ]
odd[ 3 ] = even[ 3 ] + even[ 2 ] + even[ 6 ]
odd[ 4 ] = even[ 4 ] + even[ 1 ] + even[ 5 ] + even[ 7 ]
odd[ 5 ] = even[ 5 ] + even[ 2 ] + even[ 4 ] + even[ 8 ] + even[ 6 ]
odd[ 6 ] = even[ 6 ] + even[ 3 ] + even[ 5 ] + even[ 9 ]
odd[ 7 ] = even[ 7 ] + even[ 4 ] + even[ 8 ]
odd[ 8 ] = even[ 8 ] + even[ 0 ] + even[ 5 ] + even[ 7 ] + even[ 9 ]
odd[ 9 ] = even[ 9 ] + even[ 6 ] + even[ 8 ]
totalCount = 0
if (useOdd = = 1 ):
for i in range ( 10 ):
totalCount + = even[i]
else :
for i in range ( 10 ):
totalCount + = odd[i]
return totalCount
if __name__ = = "__main__" :
keypad = [[ '1' , '2' , '3' ],
[ '4' , '5' , '6' ],
[ '7' , '8' , '9' ],
[ '*' , '0' , '#' ]]
print ( "Count for numbers of length " , 1 , ": " , getCount(keypad, 1 ))
print ( "Count for numbers of length " , 2 , ": " , getCount(keypad, 2 ))
print ( "Count for numbers of length " , 3 , ": " , getCount(keypad, 3 ))
print ( "Count for numbers of length " , 4 , ": " , getCount(keypad, 4 ))
print ( "Count for numbers of length " , 5 , ": " , getCount(keypad, 5 ))
|
C#
using System;
class GFG
{
static int getCount( char [,]keypad, int n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 10;
int []odd = new int [10];
int []even = new int [10];
int i = 0, j = 0, useOdd = 0, totalCount = 0;
for (i = 0; i <= 9; i++)
odd[i] = 1;
for (j = 2; j <= n; j++)
{
useOdd = 1 - useOdd;
if (useOdd == 1)
{
even[0] = odd[0] + odd[8];
even[1] = odd[1] + odd[2] + odd[4];
even[2] = odd[2] + odd[1] +
odd[3] + odd[5];
even[3] = odd[3] + odd[2] + odd[6];
even[4] = odd[4] + odd[1] +
odd[5] + odd[7];
even[5] = odd[5] + odd[2] + odd[4] +
odd[8] + odd[6];
even[6] = odd[6] + odd[3] +
odd[5] + odd[9];
even[7] = odd[7] + odd[4] + odd[8];
even[8] = odd[8] + odd[0] + odd[5] +
odd[7] + odd[9];
even[9] = odd[9] + odd[6] + odd[8];
}
else
{
odd[0] = even[0] + even[8];
odd[1] = even[1] + even[2] + even[4];
odd[2] = even[2] + even[1] +
even[3] + even[5];
odd[3] = even[3] + even[2] + even[6];
odd[4] = even[4] + even[1] +
even[5] + even[7];
odd[5] = even[5] + even[2] + even[4] +
even[8] + even[6];
odd[6] = even[6] + even[3] +
even[5] + even[9];
odd[7] = even[7] + even[4] + even[8];
odd[8] = even[8] + even[0] + even[5] +
even[7] + even[9];
odd[9] = even[9] + even[6] + even[8];
}
}
totalCount = 0;
if (useOdd == 1)
{
for (i = 0; i <= 9; i++)
totalCount += even[i];
}
else
{
for (i = 0; i <= 9; i++)
totalCount += odd[i];
}
return totalCount;
}
public static void Main(String[] args)
{
char [,]keypad = {{ '1' , '2' , '3' },
{ '4' , '5' , '6' },
{ '7' , '8' , '9' },
{ '*' , '0' , '#' }};
Console.Write( "Count for numbers of length {0}: {1}\n" , 1,
getCount(keypad, 1));
Console.Write( "Count for numbers of length {0}: {1}\n" , 2,
getCount(keypad, 2));
Console.Write( "Count for numbers of length {0}: {1}\n" , 3,
getCount(keypad, 3));
Console.Write( "Count for numbers of length {0}: {1}\n" , 4,
getCount(keypad, 4));
Console.Write( "Count for numbers of length {0}: {1}\n" , 5,
getCount(keypad, 5));
}
}
|
Javascript
<script>
function getCount(keypad , n)
{
if (keypad == null || n <= 0)
return 0;
if (n == 1)
return 10;
var odd = Array.from({length: 10}, (_, i) => 0);
var even = Array.from({length: 10}, (_, i) => 0);
var i = 0, j = 0, useOdd = 0, totalCount = 0;
for (i = 0; i <= 9; i++)
odd[i] = 1;
for (j = 2; j <= n; j++)
{
useOdd = 1 - useOdd;
if (useOdd == 1)
{
even[0] = odd[0] + odd[8];
even[1] = odd[1] + odd[2] + odd[4];
even[2] = odd[2] + odd[1] +
odd[3] + odd[5];
even[3] = odd[3] + odd[2] + odd[6];
even[4] = odd[4] + odd[1] +
odd[5] + odd[7];
even[5] = odd[5] + odd[2] + odd[4] +
odd[8] + odd[6];
even[6] = odd[6] + odd[3] +
odd[5] + odd[9];
even[7] = odd[7] + odd[4] + odd[8];
even[8] = odd[8] + odd[0] + odd[5] +
odd[7] + odd[9];
even[9] = odd[9] + odd[6] + odd[8];
}
else
{
odd[0] = even[0] + even[8];
odd[1] = even[1] + even[2] + even[4];
odd[2] = even[2] + even[1] +
even[3] + even[5];
odd[3] = even[3] + even[2] + even[6];
odd[4] = even[4] + even[1] +
even[5] + even[7];
odd[5] = even[5] + even[2] + even[4] +
even[8] + even[6];
odd[6] = even[6] + even[3] +
even[5] + even[9];
odd[7] = even[7] + even[4] + even[8];
odd[8] = even[8] + even[0] + even[5] +
even[7] + even[9];
odd[9] = even[9] + even[6] + even[8];
}
}
totalCount = 0;
if (useOdd == 1)
{
for (i = 0; i <= 9; i++)
totalCount += even[i];
}
else
{
for (i = 0; i <= 9; i++)
totalCount += odd[i];
}
return totalCount;
}
var keypad = [[ '1' , '2' , '3' ],
[ '4' , '5' , '6' ],
[ '7' , '8' , '9' ],
[ '*' , '0' , '#' ]];
document.write( "Count for numbers of length " + 1+ ": " +
getCount(keypad, 1));
document.write( "<br>Count for numbers of length " + 2+ ": " +
getCount(keypad, 2));
document.write( "<br>Count for numbers of length " + 3+ ": " +
getCount(keypad, 3));
document.write( "<br>Count for numbers of length " + 4+ ": " +
getCount(keypad, 4));
document.write( "<br>Count for numbers of length " + 5+ ": " +
getCount(keypad, 5));
</script>
|
Output
Count for numbers of length 1: 10
Count for numbers of length 2: 36
Count for numbers of length 3: 138
Count for numbers of length 4: 532
Count for numbers of length 5: 2062
Time complexity :- O(N)
Auxiliary Space: O(1)
BRUTE APPROACH USING TABULATION:
Intuition:
- We declare a 2-D matrix of size arr[n+1][10]
- We put the no of times the keypad is pressed ie N in the row side and the numbers form 0-9 in the column side
- since it mentioned that we can press only left ,right down,up and the number itself after clicking
- so we store that numbers in 2-D array to keep a track of all number neighboring them
- Atlast we return the sum of the last row as that would given all the required possibilities.
Implementation:
C++
#include <bits/stdc++.h>
using namespace std;
long getCount( int N)
{
long dp[N + 1][10];
vector<vector< int > > data
= { { 0, 8 }, { 1, 2, 4 },
{ 1, 2, 3, 5 }, { 2, 3, 6 },
{ 1, 4, 5, 7 }, { 2, 4, 5, 6, 8 },
{ 3, 5, 6, 9 }, { 4, 7, 8 },
{ 5, 7, 8, 9, 0 }, { 6, 8, 9 } };
for ( int i = 1; i <= N; i++) {
for ( int j = 0; j <= 9; j++) {
if (i == 1)
dp[i][j] = 1;
else {
dp[i][j] = 0;
for ( int k = 0; k < data[j].size(); k++)
dp[i][j] += dp[i - 1][data[j][k]];
}
}
}
long sum = 0;
for ( int j = 0; j <= 9; j++) {
sum += dp[N][j];
}
return sum;
}
int main()
{
cout << getCount(5) << endl;
return 0;
}
|
Java
import java.util.*;
import java.io.*;
class GFG {
public static long getCount( int N)
{
long dp[][] = new long [N + 1 ][ 10 ];
int [][] data
= { { 0 , 8 }, { 1 , 2 , 4 },
{ 1 , 2 , 3 , 5 }, { 2 , 3 , 6 },
{ 1 , 4 , 5 , 7 }, { 2 , 4 , 5 , 6 , 8 },
{ 3 , 5 , 6 , 9 }, { 4 , 7 , 8 },
{ 5 , 7 , 8 , 9 , 0 }, { 6 , 8 , 9 } };
for ( int i = 1 ; i <= N; i++) {
for ( int j = 0 ; j <= 9 ; j++) {
if (i == 1 )
dp[i][j] = 1 ;
else {
for ( int prev : data[j])
dp[i][j] += dp[i - 1 ][prev];
}
}
}
long sum = 0 ;
for ( int j = 0 ; j <= 9 ; j++) {
sum += dp[N][j];
}
return sum;
}
public static void main(String[] args)
{
System.out.println(getCount( 5 ));
}
}
|
Python3
def get_count(N):
dp = [[ 0 ] * 10 for _ in range (N + 1 )]
data = [[ 0 , 8 ], [ 1 , 2 , 4 ], [ 1 , 2 , 3 , 5 ], [ 2 , 3 , 6 ],
[ 1 , 4 , 5 , 7 ], [ 2 , 4 , 5 , 6 , 8 ], [ 3 , 5 , 6 , 9 ],
[ 4 , 7 , 8 ], [ 5 , 7 , 8 , 9 , 0 ], [ 6 , 8 , 9 ]]
for i in range ( 1 , N + 1 ):
for j in range ( 10 ):
if i = = 1 :
dp[i][j] = 1
else :
for prev in data[j]:
dp[i][j] + = dp[i - 1 ][prev]
sum = 0
for j in range ( 10 ):
sum + = dp[N][j]
return sum
def main():
print (get_count( 5 ))
if __name__ = = '__main__' :
main()
|
C#
using System;
class GFG {
public static long GetCount( int N)
{
long [, ] dp = new long [N + 1, 10];
int [][] data = {
new int [] { 0, 8 },
new int [] { 1, 2,
4 },
new int [] { 1, 2, 3,
5 },
new int [] { 2, 3,
6 },
new int [] { 1, 4, 5,
7 },
new int [] { 2, 4, 5, 6,
8 },
new int [] { 3, 5, 6,
9 },
new int [] { 4, 7,
8 },
new int [] { 5, 7, 8, 9,
0 },
new int [] { 6, 8,
9 }
};
for ( int j = 0; j <= 9; j++)
dp[1, j] = 1;
for ( int i = 2; i <= N; i++) {
for ( int j = 0; j <= 9; j++) {
foreach ( int prev in data[j])
{
dp[i, j] += dp[i - 1, prev];
}
}
}
long sum = 0;
for ( int j = 0; j <= 9; j++) {
sum += dp[N, j];
}
return sum;
}
public static void Main( string [] args)
{
Console.WriteLine(GetCount(5));
}
}
|
Javascript
function getCount(N) {
let dp = new Array(N + 1);
for (let i = 0; i <= N; i++) {
dp[i] = new Array(10).fill(0);
}
let data = [
[0, 8], [1, 2, 4], [1, 2, 3, 5], [2, 3, 6],
[1, 4, 5, 7], [2, 4, 5, 6, 8], [3, 5, 6, 9],
[4, 7, 8], [5, 7, 8, 9, 0], [6, 8, 9]
];
for (let i = 1; i <= N; i++) {
for (let j = 0; j < 10; j++) {
if (i === 1) {
dp[i][j] = 1;
} else {
for (let prev of data[j]) {
dp[i][j] += dp[i - 1][prev];
}
}
}
}
let sum = 0;
for (let j = 0; j < 10; j++) {
sum += dp[N][j];
}
return sum;
}
function main() {
console.log(getCount(5));
}
main();
|
Time complexity :- O(N)
Space Complexity: O(N*10)
Share your thoughts in the comments
Please Login to comment...