Menu-Driven program using Switch-case in C
Last Updated :
21 Jul, 2023
A Menu Driven program is a program that represents a menu of options to the user and different actions are performed based on different options. In this article, we will learn to write a Menu Driven program using Switch-case in C.
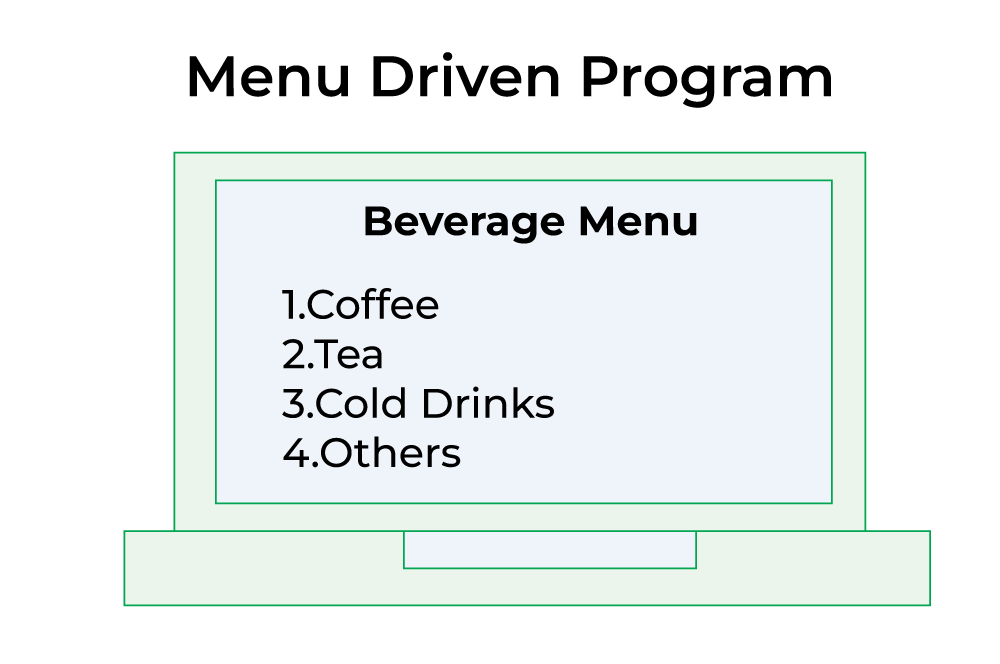
Menu Driven Program in C
The below program demonstrates an example of a Menu-Driven program using a Switch case to calculate:
- Area of a circle
- Area of square
- Area of sphere
C
#include <stdio.h>
int input();
void output( float );
int main()
{
float result;
int choice, num;
printf ( "Press 1 to calculate area of circle\n" );
printf ( "Press 2 to calculate area of square\n" );
printf ( "Press 3 to calculate area of sphere\n" );
printf ( "Enter your choice:\n" );
choice = input();
switch (choice) {
case 1: {
printf ( "Enter radius:\n" );
num = input();
result = 3.14 * num * num;
printf ( "Area of sphere=" );
output(result);
break ;
}
case 2: {
printf ( "Enter side of square:\n" );
num = input();
result = num * num;
printf ( "Area of square=" );
output(result);
break ;
}
case 3: {
printf ( "Enter radius:\n" );
num = input();
result = 4 * (3.14 * num * num);
printf ( "Area of sphere=" );
output(result);
break ;
}
default :
printf ( "wrong Input\n" );
}
return 0;
}
int input()
{
int number;
scanf ( "%d" , &number);
return (number);
}
void output( float number) { printf ( "%f" , number); }
|
Output
Press 1 to calculate area of circle
Press 2 to calculate area of square
Press 3 to calculate area of sphere
Enter your choice:
1
Enter radius:
5
Area of circle=78.5
Complexity Analysis
Time Complexity: O(1)
Auxiliary Space: O(1)
Related Articles
Share your thoughts in the comments
Please Login to comment...