Median of two sorted arrays of same size
Last Updated :
09 May, 2023
There are 2 sorted arrays A and B of size n each. Write an algorithm to find the median of the array obtained after merging the above 2 arrays(i.e. array of length 2n). The complexity should be O(log(n))
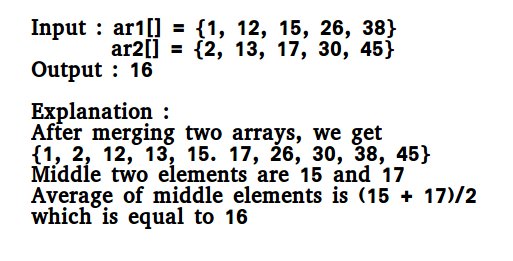
Note: Since the size of the set for which we are looking for the median is even (2n), we need to take the average of the middle two numbers and return the floor of the average.
Method 1 (Simply count while Merging)
Use the merge procedure of merge sort. Keep track of count while comparing elements of two arrays. If count becomes n(For 2n elements), we have reached the median. Take the average of the elements at indexes n-1 and n in the merged array. See the below implementation.
C
#include <stdio.h>
int getMedian( int ar1[], int ar2[], int n)
{
int i = 0;
int j = 0;
int count;
int m1 = -1, m2 = -1;
for (count = 0; count <= n; count++)
{
if (i == n)
{
m1 = m2;
m2 = ar2[0];
break ;
}
else if (j == n)
{
m1 = m2;
m2 = ar1[0];
break ;
}
if (ar1[i] <= ar2[j])
{
m1 = m2;
m2 = ar1[i];
i++;
}
else
{
m1 = m2;
m2 = ar2[j];
j++;
}
}
return (m1 + m2)/2;
}
int main()
{
int ar1[] = {1, 12, 15, 26, 38};
int ar2[] = {2, 13, 17, 30, 45};
int n1 = sizeof (ar1)/ sizeof (ar1[0]);
int n2 = sizeof (ar2)/ sizeof (ar2[0]);
if (n1 == n2)
printf ( "Median is %d" , getMedian(ar1, ar2, n1));
else
printf ( "Doesn't work for arrays of unequal size" );
getchar ();
return 0;
}
|
C++
#include <bits/stdc++.h>
using namespace std;
double getMedian( int ar1[], int ar2[], int n)
{
int i = 0;
int j = 0;
int count;
int m1 = -1, m2 = -1;
for (count = 0; count <= n; count++) {
if (i == n) {
m1 = m2;
m2 = ar2[0];
break ;
}
else if (j == n) {
m1 = m2;
m2 = ar1[0];
break ;
}
if (ar1[i] <= ar2[j]) {
m1 = m2;
m2 = ar1[i];
i++;
}
else {
m1 = m2;
m2 = ar2[j];
j++;
}
}
return (1.0 * (m1 + m2)) / 2;
}
int main()
{
int ar1[] = {1, 12, 15, 26, 38};
int ar2[] = {2, 13, 17, 30, 45};
int n1 = sizeof (ar1) / sizeof (ar1[0]);
int n2 = sizeof (ar2) / sizeof (ar2[0]);
if (n1 == n2)
cout << "Median is " << getMedian(ar1, ar2, n1);
else
cout << "Doesn't work for arrays"
<< " of unequal size" ;
getchar ();
return 0;
}
|
Java
class Main
{
static int getMedian( int ar1[], int ar2[], int n)
{
int i = 0 ;
int j = 0 ;
int count;
int m1 = - 1 , m2 = - 1 ;
for (count = 0 ; count <= n; count++)
{
if (i == n)
{
m1 = m2;
m2 = ar2[ 0 ];
break ;
}
else if (j == n)
{
m1 = m2;
m2 = ar1[ 0 ];
break ;
}
if (ar1[i] <= ar2[j])
{
m1 = m2;
m2 = ar1[i];
i++;
}
else
{
m1 = m2;
m2 = ar2[j];
j++;
}
}
return (m1 + m2)/ 2 ;
}
public static void main (String[] args)
{
int ar1[] = { 1 , 12 , 15 , 26 , 38 };
int ar2[] = { 2 , 13 , 17 , 30 , 45 };
int n1 = ar1.length;
int n2 = ar2.length;
if (n1 == n2)
System.out.println( "Median is " +
getMedian(ar1, ar2, n1));
else
System.out.println( "arrays are of unequal size" );
}
}
|
Python3
def getMedian( ar1, ar2 , n):
i = 0
j = 0
m1 = - 1
m2 = - 1
count = 0
while count < n + 1 :
count + = 1
if i = = n:
m1 = m2
m2 = ar2[ 0 ]
break
elif j = = n:
m1 = m2
m2 = ar1[ 0 ]
break
if ar1[i] < = ar2[j]:
m1 = m2
m2 = ar1[i]
i + = 1
else :
m1 = m2
m2 = ar2[j]
j + = 1
return (m1 + m2) / 2
ar1 = [ 1 , 12 , 15 , 26 , 38 ]
ar2 = [ 2 , 13 , 17 , 30 , 45 ]
n1 = len (ar1)
n2 = len (ar2)
if n1 = = n2:
print ( "Median is " , getMedian(ar1, ar2, n1))
else :
print ( "Doesn't work for arrays of unequal size" )
|
C#
using System;
class GFG
{
static int getMedian( int []ar1,
int []ar2,
int n)
{
int i = 0;
int j = 0;
int count;
int m1 = -1, m2 = -1;
for (count = 0; count <= n; count++)
{
if (i == n)
{
m1 = m2;
m2 = ar2[0];
break ;
}
else if (j == n)
{
m1 = m2;
m2 = ar1[0];
break ;
}
if (ar1[i] <= ar2[j])
{
m1 = m2;
m2 = ar1[i];
i++;
}
else
{
m1 = m2;
m2 = ar2[j];
j++;
}
}
return (m1 + m2)/2;
}
public static void Main ()
{
int []ar1 = {1, 12, 15, 26, 38};
int []ar2 = {2, 13, 17, 30, 45};
int n1 = ar1.Length;
int n2 = ar2.Length;
if (n1 == n2)
Console.Write( "Median is " +
getMedian(ar1, ar2, n1));
else
Console.Write( "arrays are of unequal size" );
}
}
|
PHP
<?php
function getMedian( $ar1 , $ar2 , $n )
{
$i = 0;
$j = 0;
$count ;
$m1 = -1; $m2 = -1;
for ( $count = 0; $count <= $n ; $count ++)
{
if ( $i == $n )
{
$m1 = $m2 ;
$m2 = $ar2 [0];
break ;
}
else if ( $j == $n )
{
$m1 = $m2 ;
$m2 = $ar1 [0];
break ;
}
if ( $ar1 [ $i ] <= $ar2 [ $j ])
{
$m1 = $m2 ;
$m2 = $ar1 [ $i ];
$i ++;
}
else
{
$m1 = $m2 ;
$m2 = $ar2 [ $j ];
$j ++;
}
}
return ( $m1 + $m2 ) / 2;
}
$ar1 = array (1, 12, 15, 26, 38);
$ar2 = array (2, 13, 17, 30, 45);
$n1 = sizeof( $ar1 );
$n2 = sizeof( $ar2 );
if ( $n1 == $n2 )
echo ( "Median is " .
getMedian( $ar1 , $ar2 , $n1 ));
else
echo ( "Doesn't work for arrays" .
"of unequal size" );
?>
|
Javascript
<script>
function getMedian(ar1, ar2, n)
{
var i = 0;
var j = 0;
var count;
var m1 = -1, m2 = -1;
for (count = 0; count <= n; count++)
{
if (i == n)
{
m1 = m2;
m2 = ar2[0];
break ;
}
else if (j == n)
{
m1 = m2;
m2 = ar1[0];
break ;
}
if (ar1[i] <= ar2[j])
{
m1 = m2;
m2 = ar1[i];
i++;
}
else
{
m1 = m2;
m2 = ar2[j];
j++;
}
}
return (m1 + m2)/2;
}
var ar1 = [1, 12, 15, 26, 38];
var ar2 = [2, 13, 17, 30, 45];
var n1 = ar1.length;
var n2 = ar2.length;
if (n1 == n2)
document.write( "Median is " + getMedian(ar1, ar2, n1));
else
document.write( "Doesn't work for arrays of unequal size" );
</script>
|
Time Complexity: O(n)
Auxiliary Space: O(1)\
Method 2 (By Merging two arrays w/o extra space)
This method works by merging two arrays without extra space and then sorting them.
Algorithm :
1) Merge the two input arrays ar1[] and ar2[].
2) Sort ar1[] and ar2[] respectively.
3) The median will be the last element of ar1[] + the first
element of ar2[] divided by 2. [(ar1[n-1] + ar2[0])/2].
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int getMedian( int ar1[], int ar2[], int n)
{
int j = 0;
int i = n - 1;
while (ar1[i] > ar2[j] && j < n && i > -1)
swap(ar1[i--], ar2[j++]);
sort(ar1, ar1 + n);
sort(ar2, ar2 + n);
return (ar1[n - 1] + ar2[0]) / 2;
}
int main()
{
int ar1[] = { 1, 12, 15, 26, 38 };
int ar2[] = { 2, 13, 17, 30, 45 };
int n1 = sizeof (ar1) / sizeof (ar1[0]);
int n2 = sizeof (ar2) / sizeof (ar2[0]);
if (n1 == n2)
cout << "Median is " << getMedian(ar1, ar2, n1);
else
cout << "Doesn't work for arrays"
<< " of unequal size" ;
getchar ();
return 0;
}
|
C
#include <stdio.h>
#include <stdlib.h>
int compare( const void * num1, const void * num2)
{
if (*( int *)num1 > *( int *)num2)
return 1;
else
return -1;
}
int getMedian( int ar1[], int ar2[], int n)
{
int j = 0;
int i = n - 1;
while (ar1[i] > ar2[j] && j < n && i > -1) {
int temp = ar1[i];
ar1[i] = ar2[j];
ar2[j] = temp;
i--;
j++;
}
qsort (ar1, n, sizeof ( int ), compare);
qsort (ar2, n, sizeof ( int ), compare);
return (ar1[n - 1] + ar2[0]) / 2;
}
int main()
{
int ar1[] = { 1, 12, 15, 26, 38 };
int ar2[] = { 2, 13, 17, 30, 45 };
int n1 = sizeof (ar1) / sizeof (ar1[0]);
int n2 = sizeof (ar2) / sizeof (ar2[0]);
if (n1 == n2)
printf ( "Median is %d " , getMedian(ar1, ar2, n1));
else
printf ( "Doesn't work for arrays of unequal size" );
return 0;
}
|
Java
import java.io.*;
import java.util.*;
class GFG
{
public static int getMedian( int ar1[],
int ar2[], int n)
{
int j = 0 ;
int i = n - 1 ;
while (ar1[i] > ar2[j] && j < n && i > - 1 )
{
int temp = ar1[i];
ar1[i] = ar2[j];
ar2[j] = temp;
i--; j++;
}
Arrays.sort(ar1);
Arrays.sort(ar2);
return (ar1[n - 1 ] + ar2[ 0 ]) / 2 ;
}
public static void main (String[] args)
{
int ar1[] = { 1 , 12 , 15 , 26 , 38 };
int ar2[] = { 2 , 13 , 17 , 30 , 45 };
int n1 = 5 ;
int n2 = 5 ;
if (n1 == n2)
System.out.println( "Median is " + getMedian(ar1, ar2, n1));
else
System.out.println( "Doesn't work for arrays of unequal size" );
}
}
|
Python3
def getMedian(ar1, ar2, n):
i, j = n - 1 , 0
while (ar1[i] > ar2[j] and i > - 1 and j < n):
ar1[i], ar2[j] = ar2[j], ar1[i]
i - = 1
j + = 1
ar1.sort()
ar2.sort()
return (ar1[ - 1 ] + ar2[ 0 ]) >> 1
if __name__ = = '__main__' :
ar1 = [ 1 , 12 , 15 , 26 , 38 ]
ar2 = [ 2 , 13 , 17 , 30 , 45 ]
n1, n2 = len (ar1), len (ar2)
if (n1 = = n2):
print ( 'Median is' , getMedian(ar1, ar2, n1))
else :
print ( "Doesn't work for arrays of unequal size" )
|
C#
using System;
public class GFG
{
public static int getMedian( int []ar1,
int []ar2, int n)
{
int j = 0;
int i = n - 1;
while (ar1[i] > ar2[j] && j < n && i > -1)
{
int temp = ar1[i];
ar1[i] = ar2[j];
ar2[j] = temp;
i--; j++;
}
Array.Sort(ar1);
Array.Sort(ar2);
return (ar1[n - 1] + ar2[0]) / 2;
}
public static void Main(String[] args)
{
int []ar1 = { 1, 12, 15, 26, 38 };
int []ar2 = { 2, 13, 17, 30, 45 };
int n1 = 5;
int n2 = 5;
if (n1 == n2)
Console.WriteLine( "Median is " + getMedian(ar1, ar2, n1));
else
Console.WriteLine( "Doesn't work for arrays of unequal size" );
}
}
|
Javascript
<script>
function getMedian(ar1, ar2, n)
{
let j = 0;
let i = n - 1;
while (ar1[i] > ar2[j] && j < n && i > -1)
{
let temp = ar1[i];
ar1[i] = ar2[j];
ar2[j] = temp;
i--; j++;
}
ar1.sort( function (a, b){ return a - b});
ar2.sort( function (a, b){ return a - b});
return parseInt((ar1[n - 1] + ar2[0]) / 2, 10);
}
let ar1 = [ 1, 12, 15, 26, 38 ];
let ar2 = [ 2, 13, 17, 30, 45 ];
let n1 = 5;
let n2 = 5;
if (n1 == n2)
document.write( "Median is " + getMedian(ar1, ar2, n1));
else
document.write( "Doesn't work for arrays of unequal size" );
</script>
|
Time Complexity: O(nlogn)
Auxiliary Space: O(1)
Method 3 (Using binary search)
This method can also be used for arrays of different sizes.
Algorithm:
We can find the kth element by using binary search on whole range of constraints of elements.
- Initialize ans = 0.0
- Initialize low = -10^9, high = 10^9 and pos = n
- Run a loop while(low <= high):
- Calculate mid = (low + (high – low)>>1)
- Find total elements less or equal to mid in the given arrays
- If the count is less or equal to pos
- Update low = mid + 1
- Else high = mid – 1
- Store low in ans, i.e., ans = low.
- Again follow step3 with pos as n – 1
- Return (sum + low * 1.0)/2
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
double getMedian( int arr1[], int arr2[], int n)
{
int low = ( int )-1e9, high = ( int )1e9;
int pos = n;
double ans = 0.0;
while (low <= high) {
int mid = low + ((high - low) >> 1);
int ub = upper_bound(arr1, arr1 + n, mid) - arr1
+ upper_bound(arr2, arr2 + n, mid) - arr2;
if (ub <= pos)
low = mid + 1;
else
high = mid - 1;
}
ans = low;
pos--;
low = ( int )-1e9;
high = ( int )1e9;
while (low <= high) {
int mid = low + ((high - low) >> 1);
int ub = upper_bound(arr1, arr1 + n, mid) - arr1
+ upper_bound(arr2, arr2 + n, mid) - arr2;
if (ub <= pos)
low = mid + 1;
else
high = mid - 1;
}
ans = (ans + low) / 2;
return ans;
}
int main()
{
int arr1[] = { 1, 4, 5, 6, 10 };
int arr2[] = { 2, 3, 4, 5, 7 };
int n = sizeof (arr1) / sizeof (arr1[0]);
double median = getMedian(arr1, arr2, n);
cout << "Median is " << median << endl;
return 0;
}
|
Java
import java.io.*;
class GFG {
public static double getMedian( int [] nums1, int [] nums2,
int n)
{
int low = ( int )-1e9, high = ( int )1e9;
int pos = n;
double ans = 0.0 ;
while (low <= high) {
int mid = low + ((high - low) >> 1 );
int ub = upperBound(nums1, mid)
+ upperBound(nums2, mid);
if (ub <= pos)
low = mid + 1 ;
else
high = mid - 1 ;
}
ans = low;
pos--;
low = ( int )-1e9;
high = ( int )1e9;
while (low <= high) {
int mid = low + ((high - low) >> 1 );
int ub = upperBound(nums1, mid)
+ upperBound(nums2, mid);
if (ub <= pos)
low = mid + 1 ;
else
high = mid - 1 ;
}
ans = (ans + low * 1.0 ) / 2 ;
return ans;
}
public static int upperBound( int [] arr, int key)
{
int low = 0 , high = arr.length;
while (low < high) {
int mid = low + ((high - low) >> 1 );
if (arr[mid] <= key)
low = mid + 1 ;
else
high = mid;
}
return low;
}
public static void main(String[] args)
{
int [] arr = { 1 , 4 , 5 , 6 , 10 };
int [] brr = { 2 , 3 , 4 , 5 , 7 };
double median = getMedian(arr, brr, arr.length);
System.out.println( "Median is " + median);
}
}
|
C#
using System;
public class GFG
{
public static double getMedian( int [] nums1, int [] nums2, int n)
{
var low = ( int )-1.0E9;
var high = ( int )1.0E9;
var pos = n;
var ans = 0.0;
while (low <= high)
{
var mid = low + ((high - low) >> 1);
var ub = upperBound(nums1, mid) + upperBound(nums2, mid);
if (ub <= pos)
{
low = mid + 1;
}
else
{
high = mid - 1;
}
}
ans = low;
pos--;
low = ( int )-1.0E9;
high = ( int )1.0E9;
while (low <= high)
{
var mid = low + ((high - low) >> 1);
var ub = upperBound(nums1, mid) + upperBound(nums2, mid);
if (ub <= pos)
{
low = mid + 1;
}
else
{
high = mid - 1;
}
}
ans = (ans + low * 1.0) / 2;
return ans;
}
public static int upperBound( int [] arr, int key)
{
var low = 0;
var high = arr.Length;
while (low < high)
{
var mid = low + ((high - low) >> 1);
if (arr[mid] <= key)
{
low = mid + 1;
}
else
{
high = mid;
}
}
return low;
}
public static void Main(String[] args)
{
int [] arr = {1, 4, 5, 6, 10};
int [] brr = {2, 3, 4, 5, 7};
var median = getMedian(arr, brr, arr.Length);
Console.WriteLine( "Median is " + median.ToString());
}
}
|
Python
def count_less_than_or_equal_to_mid(mid, arrays):
count = 0
for array in arrays:
count + = len ([x for x in array if x < = mid])
return count
def find_kth_element(arrays, n):
ans = 0.0
low = - 1e9
high = 1e9
pos = n
while low < = high:
mid = low + (high - low) / / 2
count = count_less_than_or_equal_to_mid(mid, arrays)
if count < = pos:
low = mid + 1
else :
high = mid - 1
ans = low
pos = n - 1
low = - 1e9
high = 1e9
while low < = high:
mid = low + (high - low) / / 2
count = count_less_than_or_equal_to_mid(mid, arrays)
if count < = pos:
low = mid + 1
else :
high = mid - 1
ans + = low
return (ans / 2.0 )
arrays = [[ 1 , 4 , 5 , 6 , 10 ], [ 2 , 3 , 4 , 5 , 7 ]]
n = 5
print ( "Median in" , find_kth_element(arrays, n))
|
Javascript
function getMedian(arr1, arr2, n) {
let low = -1e9,
high = 1e9,
pos = n,
ans = 0.0;
while (low <= high) {
let mid = low + ((high - low) >> 1);
let ub = 0;
if (ub <= pos) {
low = mid + 1;
} else {
high = mid - 1;
}
}
ans = low;
pos--;
low = -1e9;
high = 1e9;
while (low <= high) {
let mid = low + ((high - low) >> 1);
let ub = 0;
if (ub <= pos) {
low = mid + 1;
} else {
high = mid - 1;
}
}
ans = (ans + low) / 2;
return ans;
}
function main() {
let arr1 = [1, 4, 5, 6, 10];
let arr2 = [2, 3, 4, 5, 7];
let n = arr1.length;
let median = getMedian(arr1, arr2, n);
console.log( "Median is " + median);
return 0;
}
main();
|
Time Complexity: O(log n)
Auxiliary Space: O(1)
Median of two sorted arrays of different sizes
Please write comments if you find the above codes/algorithms incorrect, or find other ways to solve the same problem.
Share your thoughts in the comments
Please Login to comment...