Machine Learning Breast Cancer Prediction Project in Django
Last Updated :
21 Mar, 2024
The “Machine Learning Breast Cancer Prediction Project in Django” is a sophisticated healthcare initiative that harnesses the power of machine learning and web development through the Django framework to aid in the early detection of breast cancer. This project combines the prowess of artificial intelligence with the accessibility of a web application, allowing users to input relevant medical data, which is then processed by machine learning algorithms to provide accurate predictions regarding the likelihood of breast cancer.
Machine Learning Breast Cancer Prediction Project in Django
To install Django follow these steps.
Starting the Project Folder
To start the project use this command
django-admin startproject core
cd core
To start the app use this command
python manage.py startapp predictor
Now add this app to the ‘settings.py’
Setting up the files
views.py: The code defines two views in a Django web app: one for breast cancer prediction using machine learning and another for a homepage. User input is collected through a form, and predictions are displayed on a web page.
- Import Modules: The code imports necessary modules for a Django web app, including numpy, pandas, and machine learning tools.
- ‘breast’ View: A Django view function ‘breast’ reads training data, handles user input, trains a RandomForestClassifier model, and makes predictions for breast cancer.
- Handling User Input: The code retrieves user input attributes such as radius, texture, etc., from a web form when the user submits it.
- Machine Learning Model: A RandomForestClassifier model is created and trained with specific settings using the training data.
- Making Predictions: User input is used to make predictions with the trained model, determining if the user “has” or “doesn’t have” breast cancer.
- Rendering Templates: The ‘breast’ view renders a web page (‘breast.html’) and passes context data like the prediction result, page title, CSS styling, and a form for user input.
- ‘home’ View: An additional ‘home’ view is defined to render a homepage template (‘home.html’).
Python3
from django.shortcuts import render
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.neighbors import KNeighborsClassifier
from predictor.forms import BreastCancerForm
def breast(request):
df = pd.read_csv( 'static/Breast_train.csv' )
data = df.values
X = data[:, : - 1 ]
Y = data[:, - 1 ]
print (X.shape, Y.shape)
value = ''
if request.method = = 'POST' :
radius = float (request.POST[ 'radius' ])
texture = float (request.POST[ 'texture' ])
perimeter = float (request.POST[ 'perimeter' ])
area = float (request.POST[ 'area' ])
smoothness = float (request.POST[ 'smoothness' ])
rf = RandomForestClassifier(
n_estimators = 16 , criterion = 'entropy' , max_depth = 5 )
rf.fit(np.nan_to_num(X), Y)
user_data = np.array(
(radius, texture, perimeter, area, smoothness)
).reshape( 1 , 5 )
predictions = rf.predict(user_data)
print (predictions)
if int (predictions[ 0 ]) = = 1 :
value = 'have'
elif int (predictions[ 0 ]) = = 0 :
value = "don't have"
return render(request,
'breast.html' ,
{
'result' : value,
'title' : 'Breast Cancer Prediction' ,
'active' : 'btn btn-success peach-gradient text-white' ,
'breast' : True ,
'form' : BreastCancerForm(),
})
def home(request):
return render(request, 'home.html' )
|
settings.py: Here MEDIA_ROOT defines the directory where user-uploaded media files (e.g., images) are stored, and MEDIA_URL sets the URL prefix for serving them.
Python3
import os
STATIC_URL = '/static/'
STATIC_ROOT = os.path.join(BASE_DIR, 'static_serve' )
STATICFILES_DIRS = [
'static' ,
]
MEDIA_ROOT = os.path.join(BASE_DIR, 'media' )
MEDIA_URL = '/media/'
|
Creating GUI
home.html: This code is used to view the home page of the project.
HTML
{% load static %}
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta
name = "viewport"
content = "width=device-width, initial-scale=1, shrink-to-fit=no"
/>
< title >Disease Predictor</ title >
< style >
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
margin: 0;
padding: 0;
}
.pricing {
text-align: center;
padding: 20px;
}
.card-title {
font-size: 36px;
color: green;
margin-bottom: 20px;
}
.btn {
font-size: 18px;
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
text-decoration: none;
}
.btn:hover {
background-color: #0056b3;
}
</ style >
</ head >
< body >
< section class = "pricing py-5" >
< h1 class = "card-title" >GeeksforGeeks</ h1 >
< button onclick = "window.location.href='breast'" class = "btn" >KNOW BREAST CANCER STATUS</ button >
</ section >
</ body >
</ html >
|
breast.html: This code is used to view the view the result of the patient.
HTML
{% extends 'base.html' %}
{% block content %}
< style >
.card-title {
font-size: 40px;
color: green;
margin-bottom: 20px;
margin-left: 40%;
}
</ style >
< div class = "container" >
< h1 class = "card-title" >GeeksforGeeks</ h1 >
< div class = "display-4 my-2 text-center" >Breast Cancer Prediction</ div >
< hr />
{% if result %}
< div class = "h1 my-2 text-center" >
You {{ result }} breast cancer.
</ div >
{% endif %}
< div class = "col-md-4" >
< form class = "" method = "POST" action = "{% url 'breast' %}" >
{% csrf_token %}
{{ form }}
< div class = "mt-4" >
< button type = "submit" class = "btn-lg btn-block btn btn-outline-danger mb-5" >
Submit
</ button >
</ div >
</ form >
</ div >
</ div >
{% endblock %}
|
base.html: This is the base HTML file.
HTML
{% load static %}
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta
name = "viewport"
content = "width=device-width, initial-scale=1, shrink-to-fit=no"
/>
< link
rel = "stylesheet"
integrity = "sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T"
crossorigin = "anonymous"
/>
< title >{{title}}</ title >
</ head >
< body class = "{{background}}" >
{% block content %} {% endblock %}
</ body >
</ html >
|
forms.py
This code defines a Django form class called BreastCancerForm with fields for collecting data related to breast cancer attributes. Each field is defined as a FloatField and includes a label, minimum and maximum allowed values, and a widget with a specific CSS class (form-control) for styling. This form can be used in a Django application to collect input data from users for breast cancer-related predictions or analysis.
Python3
from django import forms
class BreastCancerForm(forms.Form):
radius = forms.FloatField(
label = 'Mean Radius' ,
min_value = 0 ,
widget = forms.NumberInput(attrs = { 'class' : 'form-control' }),
)
texture = forms.FloatField(
label = 'Mean Texture' ,
min_value = 0 ,
widget = forms.NumberInput(attrs = { 'class' : 'form-control' }),
)
perimeter = forms.FloatField(
label = 'Mean Perimeter' ,
min_value = 0 ,
widget = forms.NumberInput(attrs = { 'class' : 'form-control' }),
)
area = forms.FloatField(
label = 'Mean Area' ,
min_value = 0 ,
widget = forms.NumberInput(attrs = { 'class' : 'form-control' }),
)
smoothness = forms.FloatField(
label = 'Mean Smoothness' ,
min_value = 0 ,
widget = forms.NumberInput(attrs = { 'class' : 'form-control' }),
)
|
core/urls.py:This file is main URL file where URL of app are included.
Python3
from django.contrib import admin
from django.urls import path, include
from django.conf.urls.static import static
from django.conf import settings
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' predictor.urls')),
]
urlpatterns + = static(settings.MEDIA_URL, document_root = settings.MEDIA_ROOT)
|
predictor/urls.py: This is the URL file where we define all URL related to app.
Python3
from django.urls import path
from . import views
urlpatterns = [
path( 'breast' , views.breast, name = "breast" ),
path('', views.home, name = "home" ),
]
|
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
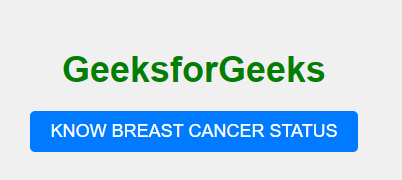
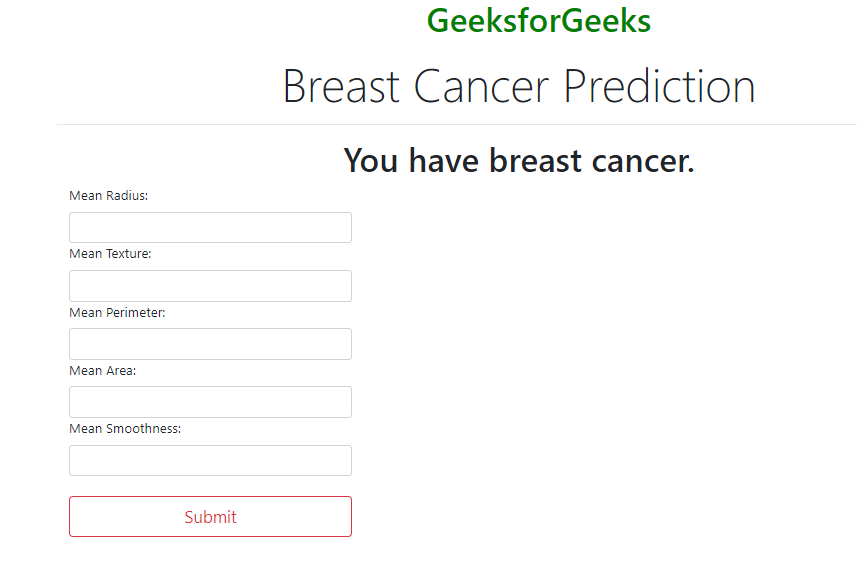
final output
The accuracy of this project is 80 % according to the using algorithm and data set.
Share your thoughts in the comments
Please Login to comment...