Diabetes Prediction Machine Learning Project Using Python Streamlit
Last Updated :
21 Mar, 2024
In this article, we will demonstrate how to create a Diabetes Prediction Machine Learning Project using Python and Streamlit. Our primary objective is to build a user-friendly graphical interface using Streamlit, allowing users to input data for diabetes prediction. To achieve this, we will leverage a dataset as our backend, along with a generated .sav file to facilitate diabetes prediction.
Diabetes Prediction Machine Learning Project using Python Streamlit
Below are the steps by which we can make a Diabetes Prediction Machine Learning Project using Python Streamlit:
Step 1: Create a Virtual Environment
First, we need to create a virtual environment and enter into a virtualenv
virtualenv env
.\env\Scripts\activate.ps1
Note.
Step 2: Install Libraries
First, install all the essential libraries using the following commands. In a Diabetes prediction GUI built with Streamlit, these libraries serve critical roles. Numpy enables efficient data handling, Pickle5 is essential for saving and loading machine learning models, and Streamlit empowers the creation of simple web apps from data analysis scripts, making them accessible to users without the need for advanced web development skills.
pip install numpy
pip install pickle5
pip install streamlit
Step 3 : File Structure
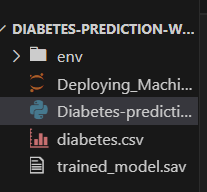
File Structure
Step 4: Writing Our Code
After installing all the libraries we write our coding part :
- Model Loading: The code loads a pre-trained machine learning model from a file named ‘trained_model.sav‘ using the
pickle.load
method. This model is stored in the variable loaded_model
and will be used for making predictions.
- Diabetes Prediction Function: The
diabetes_prediction
function is defined to make predictions based on user input. It takes a list of input data, converts it to a NumPy array, reshapes it, and then uses the loaded model to make a prediction. If the prediction is 0, it returns ‘Non Diabetic,’ otherwise, it returns ‘Diabetic’.
- Main Function: The
main
function is the core of the web application. It uses Streamlit to create a simple web interface for diabetes prediction. It displays input fields for various features related to diabetes, such as number of pregnancies, glucose level, blood pressure, and more. After entering the data, the user can click a “Predict” button to trigger the prediction.
- User Interface: The code defines the layout of the web app, takes user input for the diabetes-related features, calls the
diabetes_prediction
function with the user’s input, and displays the prediction result using the st.success
function. If the user clicks the “Predict” button, the result is shown, indicating whether the individual is predicted to be diabetic or non-diabetic.
Python3
import numpy as np
import pickle
import streamlit as st
loaded_model = pickle.load( open ( 'trained_model.sav' , 'rb' ))
def diabetes_prediction(input_data):
input_data_as_nparray = np.asarray(input_data)
input_data_reshaped = input_data_as_nparray.reshape( 1 , - 1 )
prediction = loaded_model.predict(input_data_reshaped)
if prediction = = 0 :
return 'Non Diabetic'
else :
return 'Diabetic'
def main():
st.title( 'Diabetes Prediction Web App' )
Pregnancies = st.text_input( 'No. of Pregnancies:' )
Glucose = st.text_input( 'Glucose level:' )
BloodPressure = st.text_input( 'Blood Pressure value:' )
SkinThickness = st.text_input( 'Skin thickness value:' )
Insulin = st.text_input( 'Insulin level:' )
BMI = st.text_input( 'BMI value:' )
DiabetesPedigreeFunction = st.text_input(
'Diabetes pedigree function value:' )
Age = st.text_input( 'Age:' )
diagnosis = ''
if st.button( 'Predict' ):
diagnosis = diabetes_prediction(
[Pregnancies, Glucose, BloodPressure, SkinThickness, Insulin, BMI, DiabetesPedigreeFunction, Age])
st.success(diagnosis)
if __name__ = = '__main__' :
main()
|
Step 5: Run the code
For run the code run the following command :
streamlit run "Your_.py_File_Path"
Output
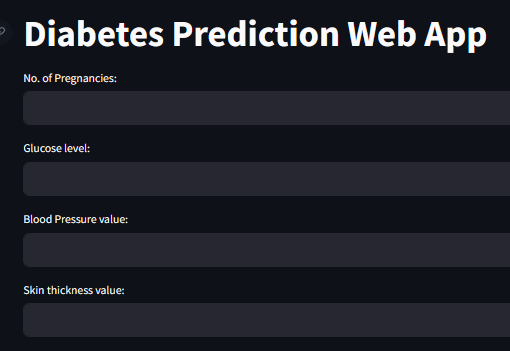
Video Demonstration
Share your thoughts in the comments
Please Login to comment...